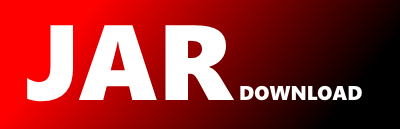
com.azure.resourcemanager.mediaservices.models.Overlay Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-mediaservices Show documentation
Show all versions of azure-resourcemanager-mediaservices Show documentation
This package contains Microsoft Azure SDK for MediaServices Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. This Swagger was generated by the API Framework. Package tag package-account-2023-01.
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.mediaservices.models;
import com.azure.core.annotation.Fluent;
import com.azure.core.util.CoreUtils;
import com.azure.core.util.logging.ClientLogger;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
import java.time.Duration;
/**
* Base type for all overlays - image, audio or video.
*/
@Fluent
public class Overlay implements JsonSerializable {
/*
* The discriminator for derived types.
*/
private String odataType = "Overlay";
/*
* The label of the job input which is to be used as an overlay. The Input must specify exactly one file. You can
* specify an image file in JPG, PNG, GIF or BMP format, or an audio file (such as a WAV, MP3, WMA or M4A file), or
* a video file. See https://aka.ms/mesformats for the complete list of supported audio and video file formats.
*/
private String inputLabel;
/*
* The start position, with reference to the input video, at which the overlay starts. The value should be in ISO
* 8601 format. For example, PT05S to start the overlay at 5 seconds into the input video. If not specified the
* overlay starts from the beginning of the input video.
*/
private Duration start;
/*
* The end position, with reference to the input video, at which the overlay ends. The value should be in ISO 8601
* format. For example, PT30S to end the overlay at 30 seconds into the input video. If not specified or the value
* is greater than the input video duration, the overlay will be applied until the end of the input video if the
* overlay media duration is greater than the input video duration, else the overlay will last as long as the
* overlay media duration.
*/
private Duration end;
/*
* The duration over which the overlay fades in onto the input video. The value should be in ISO 8601 duration
* format. If not specified the default behavior is to have no fade in (same as PT0S).
*/
private Duration fadeInDuration;
/*
* The duration over which the overlay fades out of the input video. The value should be in ISO 8601 duration
* format. If not specified the default behavior is to have no fade out (same as PT0S).
*/
private Duration fadeOutDuration;
/*
* The gain level of audio in the overlay. The value should be in the range [0, 1.0]. The default is 1.0.
*/
private Double audioGainLevel;
/**
* Creates an instance of Overlay class.
*/
public Overlay() {
}
/**
* Get the odataType property: The discriminator for derived types.
*
* @return the odataType value.
*/
public String odataType() {
return this.odataType;
}
/**
* Get the inputLabel property: The label of the job input which is to be used as an overlay. The Input must specify
* exactly one file. You can specify an image file in JPG, PNG, GIF or BMP format, or an audio file (such as a WAV,
* MP3, WMA or M4A file), or a video file. See https://aka.ms/mesformats for the complete list of supported audio
* and video file formats.
*
* @return the inputLabel value.
*/
public String inputLabel() {
return this.inputLabel;
}
/**
* Set the inputLabel property: The label of the job input which is to be used as an overlay. The Input must specify
* exactly one file. You can specify an image file in JPG, PNG, GIF or BMP format, or an audio file (such as a WAV,
* MP3, WMA or M4A file), or a video file. See https://aka.ms/mesformats for the complete list of supported audio
* and video file formats.
*
* @param inputLabel the inputLabel value to set.
* @return the Overlay object itself.
*/
public Overlay withInputLabel(String inputLabel) {
this.inputLabel = inputLabel;
return this;
}
/**
* Get the start property: The start position, with reference to the input video, at which the overlay starts. The
* value should be in ISO 8601 format. For example, PT05S to start the overlay at 5 seconds into the input video. If
* not specified the overlay starts from the beginning of the input video.
*
* @return the start value.
*/
public Duration start() {
return this.start;
}
/**
* Set the start property: The start position, with reference to the input video, at which the overlay starts. The
* value should be in ISO 8601 format. For example, PT05S to start the overlay at 5 seconds into the input video. If
* not specified the overlay starts from the beginning of the input video.
*
* @param start the start value to set.
* @return the Overlay object itself.
*/
public Overlay withStart(Duration start) {
this.start = start;
return this;
}
/**
* Get the end property: The end position, with reference to the input video, at which the overlay ends. The value
* should be in ISO 8601 format. For example, PT30S to end the overlay at 30 seconds into the input video. If not
* specified or the value is greater than the input video duration, the overlay will be applied until the end of the
* input video if the overlay media duration is greater than the input video duration, else the overlay will last as
* long as the overlay media duration.
*
* @return the end value.
*/
public Duration end() {
return this.end;
}
/**
* Set the end property: The end position, with reference to the input video, at which the overlay ends. The value
* should be in ISO 8601 format. For example, PT30S to end the overlay at 30 seconds into the input video. If not
* specified or the value is greater than the input video duration, the overlay will be applied until the end of the
* input video if the overlay media duration is greater than the input video duration, else the overlay will last as
* long as the overlay media duration.
*
* @param end the end value to set.
* @return the Overlay object itself.
*/
public Overlay withEnd(Duration end) {
this.end = end;
return this;
}
/**
* Get the fadeInDuration property: The duration over which the overlay fades in onto the input video. The value
* should be in ISO 8601 duration format. If not specified the default behavior is to have no fade in (same as
* PT0S).
*
* @return the fadeInDuration value.
*/
public Duration fadeInDuration() {
return this.fadeInDuration;
}
/**
* Set the fadeInDuration property: The duration over which the overlay fades in onto the input video. The value
* should be in ISO 8601 duration format. If not specified the default behavior is to have no fade in (same as
* PT0S).
*
* @param fadeInDuration the fadeInDuration value to set.
* @return the Overlay object itself.
*/
public Overlay withFadeInDuration(Duration fadeInDuration) {
this.fadeInDuration = fadeInDuration;
return this;
}
/**
* Get the fadeOutDuration property: The duration over which the overlay fades out of the input video. The value
* should be in ISO 8601 duration format. If not specified the default behavior is to have no fade out (same as
* PT0S).
*
* @return the fadeOutDuration value.
*/
public Duration fadeOutDuration() {
return this.fadeOutDuration;
}
/**
* Set the fadeOutDuration property: The duration over which the overlay fades out of the input video. The value
* should be in ISO 8601 duration format. If not specified the default behavior is to have no fade out (same as
* PT0S).
*
* @param fadeOutDuration the fadeOutDuration value to set.
* @return the Overlay object itself.
*/
public Overlay withFadeOutDuration(Duration fadeOutDuration) {
this.fadeOutDuration = fadeOutDuration;
return this;
}
/**
* Get the audioGainLevel property: The gain level of audio in the overlay. The value should be in the range [0,
* 1.0]. The default is 1.0.
*
* @return the audioGainLevel value.
*/
public Double audioGainLevel() {
return this.audioGainLevel;
}
/**
* Set the audioGainLevel property: The gain level of audio in the overlay. The value should be in the range [0,
* 1.0]. The default is 1.0.
*
* @param audioGainLevel the audioGainLevel value to set.
* @return the Overlay object itself.
*/
public Overlay withAudioGainLevel(Double audioGainLevel) {
this.audioGainLevel = audioGainLevel;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (inputLabel() == null) {
throw LOGGER.atError()
.log(new IllegalArgumentException("Missing required property inputLabel in model Overlay"));
}
}
private static final ClientLogger LOGGER = new ClientLogger(Overlay.class);
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("inputLabel", this.inputLabel);
jsonWriter.writeStringField("@odata.type", this.odataType);
jsonWriter.writeStringField("start", CoreUtils.durationToStringWithDays(this.start));
jsonWriter.writeStringField("end", CoreUtils.durationToStringWithDays(this.end));
jsonWriter.writeStringField("fadeInDuration", CoreUtils.durationToStringWithDays(this.fadeInDuration));
jsonWriter.writeStringField("fadeOutDuration", CoreUtils.durationToStringWithDays(this.fadeOutDuration));
jsonWriter.writeNumberField("audioGainLevel", this.audioGainLevel);
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of Overlay from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of Overlay if the JsonReader was pointing to an instance of it, or null if it was pointing to
* JSON null.
* @throws IllegalStateException If the deserialized JSON object was missing any required properties.
* @throws IOException If an error occurs while reading the Overlay.
*/
public static Overlay fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
String discriminatorValue = null;
try (JsonReader readerToUse = reader.bufferObject()) {
readerToUse.nextToken(); // Prepare for reading
while (readerToUse.nextToken() != JsonToken.END_OBJECT) {
String fieldName = readerToUse.getFieldName();
readerToUse.nextToken();
if ("@odata.type".equals(fieldName)) {
discriminatorValue = readerToUse.getString();
break;
} else {
readerToUse.skipChildren();
}
}
// Use the discriminator value to determine which subtype should be deserialized.
if ("#Microsoft.Media.AudioOverlay".equals(discriminatorValue)) {
return AudioOverlay.fromJson(readerToUse.reset());
} else if ("#Microsoft.Media.VideoOverlay".equals(discriminatorValue)) {
return VideoOverlay.fromJson(readerToUse.reset());
} else {
return fromJsonKnownDiscriminator(readerToUse.reset());
}
}
});
}
static Overlay fromJsonKnownDiscriminator(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
Overlay deserializedOverlay = new Overlay();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("inputLabel".equals(fieldName)) {
deserializedOverlay.inputLabel = reader.getString();
} else if ("@odata.type".equals(fieldName)) {
deserializedOverlay.odataType = reader.getString();
} else if ("start".equals(fieldName)) {
deserializedOverlay.start
= reader.getNullable(nonNullReader -> Duration.parse(nonNullReader.getString()));
} else if ("end".equals(fieldName)) {
deserializedOverlay.end
= reader.getNullable(nonNullReader -> Duration.parse(nonNullReader.getString()));
} else if ("fadeInDuration".equals(fieldName)) {
deserializedOverlay.fadeInDuration
= reader.getNullable(nonNullReader -> Duration.parse(nonNullReader.getString()));
} else if ("fadeOutDuration".equals(fieldName)) {
deserializedOverlay.fadeOutDuration
= reader.getNullable(nonNullReader -> Duration.parse(nonNullReader.getString()));
} else if ("audioGainLevel".equals(fieldName)) {
deserializedOverlay.audioGainLevel = reader.getNullable(JsonReader::getDouble);
} else {
reader.skipChildren();
}
}
return deserializedOverlay;
});
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy