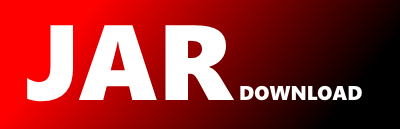
com.azure.resourcemanager.mediaservices.models.Video Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-mediaservices Show documentation
Show all versions of azure-resourcemanager-mediaservices Show documentation
This package contains Microsoft Azure SDK for MediaServices Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. This Swagger was generated by the API Framework. Package tag package-account-2023-01.
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.mediaservices.models;
import com.azure.core.annotation.Fluent;
import com.azure.core.util.CoreUtils;
import com.azure.json.JsonReader;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
import java.time.Duration;
/**
* Describes the basic properties for encoding the input video.
*/
@Fluent
public class Video extends Codec {
/*
* The discriminator for derived types.
*/
private String odataType = "#Microsoft.Media.Video";
/*
* The distance between two key frames. The value should be non-zero in the range [0.5, 20] seconds, specified in
* ISO 8601 format. The default is 2 seconds(PT2S). Note that this setting is ignored if VideoSyncMode.Passthrough
* is set, where the KeyFrameInterval value will follow the input source setting.
*/
private Duration keyFrameInterval;
/*
* The resizing mode - how the input video will be resized to fit the desired output resolution(s). Default is
* AutoSize
*/
private StretchMode stretchMode;
/*
* The Video Sync Mode
*/
private VideoSyncMode syncMode;
/**
* Creates an instance of Video class.
*/
public Video() {
}
/**
* Get the odataType property: The discriminator for derived types.
*
* @return the odataType value.
*/
@Override
public String odataType() {
return this.odataType;
}
/**
* Get the keyFrameInterval property: The distance between two key frames. The value should be non-zero in the range
* [0.5, 20] seconds, specified in ISO 8601 format. The default is 2 seconds(PT2S). Note that this setting is
* ignored if VideoSyncMode.Passthrough is set, where the KeyFrameInterval value will follow the input source
* setting.
*
* @return the keyFrameInterval value.
*/
public Duration keyFrameInterval() {
return this.keyFrameInterval;
}
/**
* Set the keyFrameInterval property: The distance between two key frames. The value should be non-zero in the range
* [0.5, 20] seconds, specified in ISO 8601 format. The default is 2 seconds(PT2S). Note that this setting is
* ignored if VideoSyncMode.Passthrough is set, where the KeyFrameInterval value will follow the input source
* setting.
*
* @param keyFrameInterval the keyFrameInterval value to set.
* @return the Video object itself.
*/
public Video withKeyFrameInterval(Duration keyFrameInterval) {
this.keyFrameInterval = keyFrameInterval;
return this;
}
/**
* Get the stretchMode property: The resizing mode - how the input video will be resized to fit the desired output
* resolution(s). Default is AutoSize.
*
* @return the stretchMode value.
*/
public StretchMode stretchMode() {
return this.stretchMode;
}
/**
* Set the stretchMode property: The resizing mode - how the input video will be resized to fit the desired output
* resolution(s). Default is AutoSize.
*
* @param stretchMode the stretchMode value to set.
* @return the Video object itself.
*/
public Video withStretchMode(StretchMode stretchMode) {
this.stretchMode = stretchMode;
return this;
}
/**
* Get the syncMode property: The Video Sync Mode.
*
* @return the syncMode value.
*/
public VideoSyncMode syncMode() {
return this.syncMode;
}
/**
* Set the syncMode property: The Video Sync Mode.
*
* @param syncMode the syncMode value to set.
* @return the Video object itself.
*/
public Video withSyncMode(VideoSyncMode syncMode) {
this.syncMode = syncMode;
return this;
}
/**
* {@inheritDoc}
*/
@Override
public Video withLabel(String label) {
super.withLabel(label);
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
@Override
public void validate() {
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("label", label());
jsonWriter.writeStringField("@odata.type", this.odataType);
jsonWriter.writeStringField("keyFrameInterval", CoreUtils.durationToStringWithDays(this.keyFrameInterval));
jsonWriter.writeStringField("stretchMode", this.stretchMode == null ? null : this.stretchMode.toString());
jsonWriter.writeStringField("syncMode", this.syncMode == null ? null : this.syncMode.toString());
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of Video from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of Video if the JsonReader was pointing to an instance of it, or null if it was pointing to
* JSON null.
* @throws IOException If an error occurs while reading the Video.
*/
public static Video fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
String discriminatorValue = null;
try (JsonReader readerToUse = reader.bufferObject()) {
readerToUse.nextToken(); // Prepare for reading
while (readerToUse.nextToken() != JsonToken.END_OBJECT) {
String fieldName = readerToUse.getFieldName();
readerToUse.nextToken();
if ("@odata.type".equals(fieldName)) {
discriminatorValue = readerToUse.getString();
break;
} else {
readerToUse.skipChildren();
}
}
// Use the discriminator value to determine which subtype should be deserialized.
if ("#Microsoft.Media.H265Video".equals(discriminatorValue)) {
return H265Video.fromJson(readerToUse.reset());
} else if ("#Microsoft.Media.Image".equals(discriminatorValue)) {
return Image.fromJsonKnownDiscriminator(readerToUse.reset());
} else if ("#Microsoft.Media.JpgImage".equals(discriminatorValue)) {
return JpgImage.fromJson(readerToUse.reset());
} else if ("#Microsoft.Media.PngImage".equals(discriminatorValue)) {
return PngImage.fromJson(readerToUse.reset());
} else if ("#Microsoft.Media.H264Video".equals(discriminatorValue)) {
return H264Video.fromJson(readerToUse.reset());
} else {
return fromJsonKnownDiscriminator(readerToUse.reset());
}
}
});
}
static Video fromJsonKnownDiscriminator(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
Video deserializedVideo = new Video();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("label".equals(fieldName)) {
deserializedVideo.withLabel(reader.getString());
} else if ("@odata.type".equals(fieldName)) {
deserializedVideo.odataType = reader.getString();
} else if ("keyFrameInterval".equals(fieldName)) {
deserializedVideo.keyFrameInterval
= reader.getNullable(nonNullReader -> Duration.parse(nonNullReader.getString()));
} else if ("stretchMode".equals(fieldName)) {
deserializedVideo.stretchMode = StretchMode.fromString(reader.getString());
} else if ("syncMode".equals(fieldName)) {
deserializedVideo.syncMode = VideoSyncMode.fromString(reader.getString());
} else {
reader.skipChildren();
}
}
return deserializedVideo;
});
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy