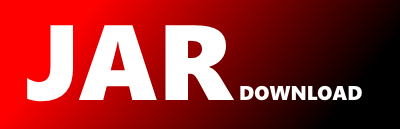
com.azure.resourcemanager.mediaservices.models.VideoAnalyzerPreset Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-mediaservices Show documentation
Show all versions of azure-resourcemanager-mediaservices Show documentation
This package contains Microsoft Azure SDK for MediaServices Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. This Swagger was generated by the API Framework. Package tag package-account-2023-01.
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.mediaservices.models;
import com.azure.core.annotation.Fluent;
import com.azure.json.JsonReader;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
import java.util.Map;
/**
* A video analyzer preset that extracts insights (rich metadata) from both audio and video, and outputs a JSON format
* file.
*/
@Fluent
public final class VideoAnalyzerPreset extends AudioAnalyzerPreset {
/*
* The discriminator for derived types.
*/
private String odataType = "#Microsoft.Media.VideoAnalyzerPreset";
/*
* Defines the type of insights that you want the service to generate. The allowed values are 'AudioInsightsOnly',
* 'VideoInsightsOnly', and 'AllInsights'. The default is AllInsights. If you set this to AllInsights and the input
* is audio only, then only audio insights are generated. Similarly if the input is video only, then only video
* insights are generated. It is recommended that you not use AudioInsightsOnly if you expect some of your inputs to
* be video only; or use VideoInsightsOnly if you expect some of your inputs to be audio only. Your Jobs in such
* conditions would error out.
*/
private InsightsType insightsToExtract;
/**
* Creates an instance of VideoAnalyzerPreset class.
*/
public VideoAnalyzerPreset() {
}
/**
* Get the odataType property: The discriminator for derived types.
*
* @return the odataType value.
*/
@Override
public String odataType() {
return this.odataType;
}
/**
* Get the insightsToExtract property: Defines the type of insights that you want the service to generate. The
* allowed values are 'AudioInsightsOnly', 'VideoInsightsOnly', and 'AllInsights'. The default is AllInsights. If
* you set this to AllInsights and the input is audio only, then only audio insights are generated. Similarly if the
* input is video only, then only video insights are generated. It is recommended that you not use AudioInsightsOnly
* if you expect some of your inputs to be video only; or use VideoInsightsOnly if you expect some of your inputs to
* be audio only. Your Jobs in such conditions would error out.
*
* @return the insightsToExtract value.
*/
public InsightsType insightsToExtract() {
return this.insightsToExtract;
}
/**
* Set the insightsToExtract property: Defines the type of insights that you want the service to generate. The
* allowed values are 'AudioInsightsOnly', 'VideoInsightsOnly', and 'AllInsights'. The default is AllInsights. If
* you set this to AllInsights and the input is audio only, then only audio insights are generated. Similarly if the
* input is video only, then only video insights are generated. It is recommended that you not use AudioInsightsOnly
* if you expect some of your inputs to be video only; or use VideoInsightsOnly if you expect some of your inputs to
* be audio only. Your Jobs in such conditions would error out.
*
* @param insightsToExtract the insightsToExtract value to set.
* @return the VideoAnalyzerPreset object itself.
*/
public VideoAnalyzerPreset withInsightsToExtract(InsightsType insightsToExtract) {
this.insightsToExtract = insightsToExtract;
return this;
}
/**
* {@inheritDoc}
*/
@Override
public VideoAnalyzerPreset withAudioLanguage(String audioLanguage) {
super.withAudioLanguage(audioLanguage);
return this;
}
/**
* {@inheritDoc}
*/
@Override
public VideoAnalyzerPreset withMode(AudioAnalysisMode mode) {
super.withMode(mode);
return this;
}
/**
* {@inheritDoc}
*/
@Override
public VideoAnalyzerPreset withExperimentalOptions(Map experimentalOptions) {
super.withExperimentalOptions(experimentalOptions);
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
@Override
public void validate() {
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("audioLanguage", audioLanguage());
jsonWriter.writeStringField("mode", mode() == null ? null : mode().toString());
jsonWriter.writeMapField("experimentalOptions", experimentalOptions(),
(writer, element) -> writer.writeString(element));
jsonWriter.writeStringField("@odata.type", this.odataType);
jsonWriter.writeStringField("insightsToExtract",
this.insightsToExtract == null ? null : this.insightsToExtract.toString());
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of VideoAnalyzerPreset from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of VideoAnalyzerPreset if the JsonReader was pointing to an instance of it, or null if it was
* pointing to JSON null.
* @throws IOException If an error occurs while reading the VideoAnalyzerPreset.
*/
public static VideoAnalyzerPreset fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
VideoAnalyzerPreset deserializedVideoAnalyzerPreset = new VideoAnalyzerPreset();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("audioLanguage".equals(fieldName)) {
deserializedVideoAnalyzerPreset.withAudioLanguage(reader.getString());
} else if ("mode".equals(fieldName)) {
deserializedVideoAnalyzerPreset.withMode(AudioAnalysisMode.fromString(reader.getString()));
} else if ("experimentalOptions".equals(fieldName)) {
Map experimentalOptions = reader.readMap(reader1 -> reader1.getString());
deserializedVideoAnalyzerPreset.withExperimentalOptions(experimentalOptions);
} else if ("@odata.type".equals(fieldName)) {
deserializedVideoAnalyzerPreset.odataType = reader.getString();
} else if ("insightsToExtract".equals(fieldName)) {
deserializedVideoAnalyzerPreset.insightsToExtract = InsightsType.fromString(reader.getString());
} else {
reader.skipChildren();
}
}
return deserializedVideoAnalyzerPreset;
});
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy