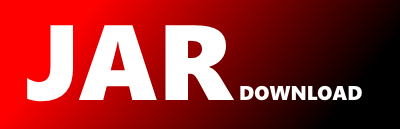
com.azure.resourcemanager.migrationdiscoverysap.implementation.ServerInstancesImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-migrationdiscoverysap Show documentation
Show all versions of azure-resourcemanager-migrationdiscoverysap Show documentation
This package contains Microsoft Azure SDK for MigrationDiscoverySap Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. Migration Discovery SAP Client. Package tag package-preview-2023-10.
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.migrationdiscoverysap.implementation;
import com.azure.core.http.rest.PagedIterable;
import com.azure.core.http.rest.Response;
import com.azure.core.http.rest.SimpleResponse;
import com.azure.core.util.Context;
import com.azure.core.util.logging.ClientLogger;
import com.azure.resourcemanager.migrationdiscoverysap.fluent.ServerInstancesClient;
import com.azure.resourcemanager.migrationdiscoverysap.fluent.models.ServerInstanceInner;
import com.azure.resourcemanager.migrationdiscoverysap.models.ServerInstance;
import com.azure.resourcemanager.migrationdiscoverysap.models.ServerInstances;
public final class ServerInstancesImpl implements ServerInstances {
private static final ClientLogger LOGGER = new ClientLogger(ServerInstancesImpl.class);
private final ServerInstancesClient innerClient;
private final com.azure.resourcemanager.migrationdiscoverysap.MigrationDiscoverySapManager serviceManager;
public ServerInstancesImpl(ServerInstancesClient innerClient,
com.azure.resourcemanager.migrationdiscoverysap.MigrationDiscoverySapManager serviceManager) {
this.innerClient = innerClient;
this.serviceManager = serviceManager;
}
public PagedIterable listBySapInstance(String resourceGroupName, String sapDiscoverySiteName,
String sapInstanceName) {
PagedIterable inner
= this.serviceClient().listBySapInstance(resourceGroupName, sapDiscoverySiteName, sapInstanceName);
return ResourceManagerUtils.mapPage(inner, inner1 -> new ServerInstanceImpl(inner1, this.manager()));
}
public PagedIterable listBySapInstance(String resourceGroupName, String sapDiscoverySiteName,
String sapInstanceName, Context context) {
PagedIterable inner
= this.serviceClient().listBySapInstance(resourceGroupName, sapDiscoverySiteName, sapInstanceName, context);
return ResourceManagerUtils.mapPage(inner, inner1 -> new ServerInstanceImpl(inner1, this.manager()));
}
public Response getWithResponse(String resourceGroupName, String sapDiscoverySiteName,
String sapInstanceName, String serverInstanceName, Context context) {
Response inner = this.serviceClient().getWithResponse(resourceGroupName,
sapDiscoverySiteName, sapInstanceName, serverInstanceName, context);
if (inner != null) {
return new SimpleResponse<>(inner.getRequest(), inner.getStatusCode(), inner.getHeaders(),
new ServerInstanceImpl(inner.getValue(), this.manager()));
} else {
return null;
}
}
public ServerInstance get(String resourceGroupName, String sapDiscoverySiteName, String sapInstanceName,
String serverInstanceName) {
ServerInstanceInner inner
= this.serviceClient().get(resourceGroupName, sapDiscoverySiteName, sapInstanceName, serverInstanceName);
if (inner != null) {
return new ServerInstanceImpl(inner, this.manager());
} else {
return null;
}
}
public void delete(String resourceGroupName, String sapDiscoverySiteName, String sapInstanceName,
String serverInstanceName) {
this.serviceClient().delete(resourceGroupName, sapDiscoverySiteName, sapInstanceName, serverInstanceName);
}
public void delete(String resourceGroupName, String sapDiscoverySiteName, String sapInstanceName,
String serverInstanceName, Context context) {
this.serviceClient().delete(resourceGroupName, sapDiscoverySiteName, sapInstanceName, serverInstanceName,
context);
}
public ServerInstance getById(String id) {
String resourceGroupName = ResourceManagerUtils.getValueFromIdByName(id, "resourceGroups");
if (resourceGroupName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'resourceGroups'.", id)));
}
String sapDiscoverySiteName = ResourceManagerUtils.getValueFromIdByName(id, "sapDiscoverySites");
if (sapDiscoverySiteName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'sapDiscoverySites'.", id)));
}
String sapInstanceName = ResourceManagerUtils.getValueFromIdByName(id, "sapInstances");
if (sapInstanceName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'sapInstances'.", id)));
}
String serverInstanceName = ResourceManagerUtils.getValueFromIdByName(id, "serverInstances");
if (serverInstanceName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'serverInstances'.", id)));
}
return this
.getWithResponse(resourceGroupName, sapDiscoverySiteName, sapInstanceName, serverInstanceName, Context.NONE)
.getValue();
}
public Response getByIdWithResponse(String id, Context context) {
String resourceGroupName = ResourceManagerUtils.getValueFromIdByName(id, "resourceGroups");
if (resourceGroupName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'resourceGroups'.", id)));
}
String sapDiscoverySiteName = ResourceManagerUtils.getValueFromIdByName(id, "sapDiscoverySites");
if (sapDiscoverySiteName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'sapDiscoverySites'.", id)));
}
String sapInstanceName = ResourceManagerUtils.getValueFromIdByName(id, "sapInstances");
if (sapInstanceName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'sapInstances'.", id)));
}
String serverInstanceName = ResourceManagerUtils.getValueFromIdByName(id, "serverInstances");
if (serverInstanceName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'serverInstances'.", id)));
}
return this.getWithResponse(resourceGroupName, sapDiscoverySiteName, sapInstanceName, serverInstanceName,
context);
}
public void deleteById(String id) {
String resourceGroupName = ResourceManagerUtils.getValueFromIdByName(id, "resourceGroups");
if (resourceGroupName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'resourceGroups'.", id)));
}
String sapDiscoverySiteName = ResourceManagerUtils.getValueFromIdByName(id, "sapDiscoverySites");
if (sapDiscoverySiteName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'sapDiscoverySites'.", id)));
}
String sapInstanceName = ResourceManagerUtils.getValueFromIdByName(id, "sapInstances");
if (sapInstanceName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'sapInstances'.", id)));
}
String serverInstanceName = ResourceManagerUtils.getValueFromIdByName(id, "serverInstances");
if (serverInstanceName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'serverInstances'.", id)));
}
this.delete(resourceGroupName, sapDiscoverySiteName, sapInstanceName, serverInstanceName, Context.NONE);
}
public void deleteByIdWithResponse(String id, Context context) {
String resourceGroupName = ResourceManagerUtils.getValueFromIdByName(id, "resourceGroups");
if (resourceGroupName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'resourceGroups'.", id)));
}
String sapDiscoverySiteName = ResourceManagerUtils.getValueFromIdByName(id, "sapDiscoverySites");
if (sapDiscoverySiteName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'sapDiscoverySites'.", id)));
}
String sapInstanceName = ResourceManagerUtils.getValueFromIdByName(id, "sapInstances");
if (sapInstanceName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'sapInstances'.", id)));
}
String serverInstanceName = ResourceManagerUtils.getValueFromIdByName(id, "serverInstances");
if (serverInstanceName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'serverInstances'.", id)));
}
this.delete(resourceGroupName, sapDiscoverySiteName, sapInstanceName, serverInstanceName, context);
}
private ServerInstancesClient serviceClient() {
return this.innerClient;
}
private com.azure.resourcemanager.migrationdiscoverysap.MigrationDiscoverySapManager manager() {
return this.serviceManager;
}
public ServerInstanceImpl define(String name) {
return new ServerInstanceImpl(name, this.manager());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy