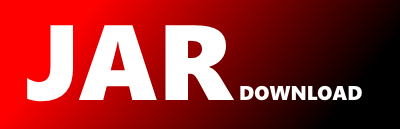
com.azure.resourcemanager.migrationdiscoverysap.models.ServerInstanceProperties Maven / Gradle / Ivy
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.migrationdiscoverysap.models;
import com.azure.core.annotation.Immutable;
import com.fasterxml.jackson.annotation.JsonProperty;
/**
* Defines the SAP Instance properties.
*/
@Immutable
public final class ServerInstanceProperties {
/*
* This is the Virtual Machine Name of the SAP system. Add all the virtual machines attached to an SAP system which
* you wish to migrate to Azure. Keeping this not equal to ID as for single tier all InstanceTypes would be on same
* server, leading to multiple resources with same servername.
*/
@JsonProperty(value = "serverName", access = JsonProperty.Access.WRITE_ONLY)
private String serverName;
/*
* Defines the type SAP instance on this server instance.
*/
@JsonProperty(value = "sapInstanceType", access = JsonProperty.Access.WRITE_ONLY)
private SapInstanceType sapInstanceType;
/*
* This is the Instance SID for ASCS/AP/DB instance. An SAP system with HANA database for example could have a
* different SID for database Instance than that of ASCS instance.
*/
@JsonProperty(value = "instanceSid", access = JsonProperty.Access.WRITE_ONLY)
private String instanceSid;
/*
* This is the SAP Application Component; e.g. SAP S/4HANA 2022, SAP ERP ENHANCE PACKAGE.
*/
@JsonProperty(value = "sapProduct", access = JsonProperty.Access.WRITE_ONLY)
private String sapProduct;
/*
* Provide the product version of the SAP product.
*/
@JsonProperty(value = "sapProductVersion", access = JsonProperty.Access.WRITE_ONLY)
private String sapProductVersion;
/*
* This is Operating System on which the host server is running.
*/
@JsonProperty(value = "operatingSystem", access = JsonProperty.Access.WRITE_ONLY)
private OperatingSystem operatingSystem;
/*
* Configuration data for this server instance.
*/
@JsonProperty(value = "configurationData", access = JsonProperty.Access.WRITE_ONLY)
private ConfigurationData configurationData;
/*
* Configuration data for this server instance.
*/
@JsonProperty(value = "performanceData", access = JsonProperty.Access.WRITE_ONLY)
private PerformanceData performanceData;
/*
* Defines the provisioning states.
*/
@JsonProperty(value = "provisioningState", access = JsonProperty.Access.WRITE_ONLY)
private ProvisioningState provisioningState;
/*
* Defines the errors related to SAP Instance resource.
*/
@JsonProperty(value = "errors", access = JsonProperty.Access.WRITE_ONLY)
private SapMigrateError errors;
/**
* Creates an instance of ServerInstanceProperties class.
*/
public ServerInstanceProperties() {
}
/**
* Get the serverName property: This is the Virtual Machine Name of the SAP system. Add all the virtual machines
* attached to an SAP system which you wish to migrate to Azure. Keeping this not equal to ID as for single tier
* all InstanceTypes would be on same server, leading to multiple resources with same servername.
*
* @return the serverName value.
*/
public String serverName() {
return this.serverName;
}
/**
* Get the sapInstanceType property: Defines the type SAP instance on this server instance.
*
* @return the sapInstanceType value.
*/
public SapInstanceType sapInstanceType() {
return this.sapInstanceType;
}
/**
* Get the instanceSid property: This is the Instance SID for ASCS/AP/DB instance. An SAP system with HANA
* database for example could have a different SID for database Instance than that of ASCS instance.
*
* @return the instanceSid value.
*/
public String instanceSid() {
return this.instanceSid;
}
/**
* Get the sapProduct property: This is the SAP Application Component; e.g. SAP S/4HANA 2022, SAP ERP ENHANCE
* PACKAGE.
*
* @return the sapProduct value.
*/
public String sapProduct() {
return this.sapProduct;
}
/**
* Get the sapProductVersion property: Provide the product version of the SAP product.
*
* @return the sapProductVersion value.
*/
public String sapProductVersion() {
return this.sapProductVersion;
}
/**
* Get the operatingSystem property: This is Operating System on which the host server is running.
*
* @return the operatingSystem value.
*/
public OperatingSystem operatingSystem() {
return this.operatingSystem;
}
/**
* Get the configurationData property: Configuration data for this server instance.
*
* @return the configurationData value.
*/
public ConfigurationData configurationData() {
return this.configurationData;
}
/**
* Get the performanceData property: Configuration data for this server instance.
*
* @return the performanceData value.
*/
public PerformanceData performanceData() {
return this.performanceData;
}
/**
* Get the provisioningState property: Defines the provisioning states.
*
* @return the provisioningState value.
*/
public ProvisioningState provisioningState() {
return this.provisioningState;
}
/**
* Get the errors property: Defines the errors related to SAP Instance resource.
*
* @return the errors value.
*/
public SapMigrateError errors() {
return this.errors;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (configurationData() != null) {
configurationData().validate();
}
if (performanceData() != null) {
performanceData().validate();
}
if (errors() != null) {
errors().validate();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy