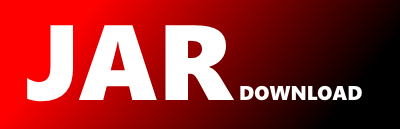
com.azure.resourcemanager.monitor.fluent.models.DataCollectionRuleResourceInner Maven / Gradle / Ivy
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.monitor.fluent.models;
import com.azure.core.annotation.Fluent;
import com.azure.core.management.Resource;
import com.azure.core.management.SystemData;
import com.azure.resourcemanager.monitor.models.DataCollectionRuleDataSources;
import com.azure.resourcemanager.monitor.models.DataCollectionRuleDestinations;
import com.azure.resourcemanager.monitor.models.DataCollectionRuleMetadata;
import com.azure.resourcemanager.monitor.models.DataFlow;
import com.azure.resourcemanager.monitor.models.KnownDataCollectionRuleProvisioningState;
import com.azure.resourcemanager.monitor.models.KnownDataCollectionRuleResourceKind;
import com.azure.resourcemanager.monitor.models.StreamDeclaration;
import com.fasterxml.jackson.annotation.JsonProperty;
import java.util.List;
import java.util.Map;
/** Definition of ARM tracked top level resource. */
@Fluent
public final class DataCollectionRuleResourceInner extends Resource {
/*
* Resource properties.
*/
@JsonProperty(value = "properties")
private DataCollectionRuleResourceProperties innerProperties;
/*
* The kind of the resource.
*/
@JsonProperty(value = "kind")
private KnownDataCollectionRuleResourceKind kind;
/*
* Resource entity tag (ETag).
*/
@JsonProperty(value = "etag", access = JsonProperty.Access.WRITE_ONLY)
private String etag;
/*
* Metadata pertaining to creation and last modification of the resource.
*/
@JsonProperty(value = "systemData", access = JsonProperty.Access.WRITE_ONLY)
private SystemData systemData;
/** Creates an instance of DataCollectionRuleResourceInner class. */
public DataCollectionRuleResourceInner() {
}
/**
* Get the innerProperties property: Resource properties.
*
* @return the innerProperties value.
*/
private DataCollectionRuleResourceProperties innerProperties() {
return this.innerProperties;
}
/**
* Get the kind property: The kind of the resource.
*
* @return the kind value.
*/
public KnownDataCollectionRuleResourceKind kind() {
return this.kind;
}
/**
* Set the kind property: The kind of the resource.
*
* @param kind the kind value to set.
* @return the DataCollectionRuleResourceInner object itself.
*/
public DataCollectionRuleResourceInner withKind(KnownDataCollectionRuleResourceKind kind) {
this.kind = kind;
return this;
}
/**
* Get the etag property: Resource entity tag (ETag).
*
* @return the etag value.
*/
public String etag() {
return this.etag;
}
/**
* Get the systemData property: Metadata pertaining to creation and last modification of the resource.
*
* @return the systemData value.
*/
public SystemData systemData() {
return this.systemData;
}
/** {@inheritDoc} */
@Override
public DataCollectionRuleResourceInner withLocation(String location) {
super.withLocation(location);
return this;
}
/** {@inheritDoc} */
@Override
public DataCollectionRuleResourceInner withTags(Map tags) {
super.withTags(tags);
return this;
}
/**
* Get the description property: Description of the data collection rule.
*
* @return the description value.
*/
public String description() {
return this.innerProperties() == null ? null : this.innerProperties().description();
}
/**
* Set the description property: Description of the data collection rule.
*
* @param description the description value to set.
* @return the DataCollectionRuleResourceInner object itself.
*/
public DataCollectionRuleResourceInner withDescription(String description) {
if (this.innerProperties() == null) {
this.innerProperties = new DataCollectionRuleResourceProperties();
}
this.innerProperties().withDescription(description);
return this;
}
/**
* Get the immutableId property: The immutable ID of this data collection rule. This property is READ-ONLY.
*
* @return the immutableId value.
*/
public String immutableId() {
return this.innerProperties() == null ? null : this.innerProperties().immutableId();
}
/**
* Get the dataCollectionEndpointId property: The resource ID of the data collection endpoint that this rule can be
* used with.
*
* @return the dataCollectionEndpointId value.
*/
public String dataCollectionEndpointId() {
return this.innerProperties() == null ? null : this.innerProperties().dataCollectionEndpointId();
}
/**
* Set the dataCollectionEndpointId property: The resource ID of the data collection endpoint that this rule can be
* used with.
*
* @param dataCollectionEndpointId the dataCollectionEndpointId value to set.
* @return the DataCollectionRuleResourceInner object itself.
*/
public DataCollectionRuleResourceInner withDataCollectionEndpointId(String dataCollectionEndpointId) {
if (this.innerProperties() == null) {
this.innerProperties = new DataCollectionRuleResourceProperties();
}
this.innerProperties().withDataCollectionEndpointId(dataCollectionEndpointId);
return this;
}
/**
* Get the metadata property: Metadata about the resource.
*
* @return the metadata value.
*/
public DataCollectionRuleMetadata metadata() {
return this.innerProperties() == null ? null : this.innerProperties().metadata();
}
/**
* Get the streamDeclarations property: Declaration of custom streams used in this rule.
*
* @return the streamDeclarations value.
*/
public Map streamDeclarations() {
return this.innerProperties() == null ? null : this.innerProperties().streamDeclarations();
}
/**
* Set the streamDeclarations property: Declaration of custom streams used in this rule.
*
* @param streamDeclarations the streamDeclarations value to set.
* @return the DataCollectionRuleResourceInner object itself.
*/
public DataCollectionRuleResourceInner withStreamDeclarations(Map streamDeclarations) {
if (this.innerProperties() == null) {
this.innerProperties = new DataCollectionRuleResourceProperties();
}
this.innerProperties().withStreamDeclarations(streamDeclarations);
return this;
}
/**
* Get the dataSources property: The specification of data sources. This property is optional and can be omitted if
* the rule is meant to be used via direct calls to the provisioned endpoint.
*
* @return the dataSources value.
*/
public DataCollectionRuleDataSources dataSources() {
return this.innerProperties() == null ? null : this.innerProperties().dataSources();
}
/**
* Set the dataSources property: The specification of data sources. This property is optional and can be omitted if
* the rule is meant to be used via direct calls to the provisioned endpoint.
*
* @param dataSources the dataSources value to set.
* @return the DataCollectionRuleResourceInner object itself.
*/
public DataCollectionRuleResourceInner withDataSources(DataCollectionRuleDataSources dataSources) {
if (this.innerProperties() == null) {
this.innerProperties = new DataCollectionRuleResourceProperties();
}
this.innerProperties().withDataSources(dataSources);
return this;
}
/**
* Get the destinations property: The specification of destinations.
*
* @return the destinations value.
*/
public DataCollectionRuleDestinations destinations() {
return this.innerProperties() == null ? null : this.innerProperties().destinations();
}
/**
* Set the destinations property: The specification of destinations.
*
* @param destinations the destinations value to set.
* @return the DataCollectionRuleResourceInner object itself.
*/
public DataCollectionRuleResourceInner withDestinations(DataCollectionRuleDestinations destinations) {
if (this.innerProperties() == null) {
this.innerProperties = new DataCollectionRuleResourceProperties();
}
this.innerProperties().withDestinations(destinations);
return this;
}
/**
* Get the dataFlows property: The specification of data flows.
*
* @return the dataFlows value.
*/
public List dataFlows() {
return this.innerProperties() == null ? null : this.innerProperties().dataFlows();
}
/**
* Set the dataFlows property: The specification of data flows.
*
* @param dataFlows the dataFlows value to set.
* @return the DataCollectionRuleResourceInner object itself.
*/
public DataCollectionRuleResourceInner withDataFlows(List dataFlows) {
if (this.innerProperties() == null) {
this.innerProperties = new DataCollectionRuleResourceProperties();
}
this.innerProperties().withDataFlows(dataFlows);
return this;
}
/**
* Get the provisioningState property: The resource provisioning state.
*
* @return the provisioningState value.
*/
public KnownDataCollectionRuleProvisioningState provisioningState() {
return this.innerProperties() == null ? null : this.innerProperties().provisioningState();
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (innerProperties() != null) {
innerProperties().validate();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy