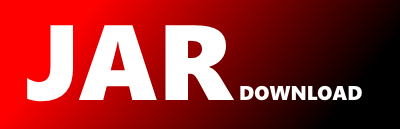
com.azure.resourcemanager.monitor.fluent.models.IncidentInner Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-monitor Show documentation
Show all versions of azure-resourcemanager-monitor Show documentation
This package contains Microsoft Azure Monitor SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.monitor.fluent.models;
import com.azure.core.annotation.Immutable;
import com.azure.core.util.CoreUtils;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
import java.time.OffsetDateTime;
/**
* An alert incident indicates the activation status of an alert rule.
*/
@Immutable
public final class IncidentInner implements JsonSerializable {
/*
* Incident name.
*/
private String name;
/*
* Rule name that is associated with the incident.
*/
private String ruleName;
/*
* A boolean to indicate whether the incident is active or resolved.
*/
private Boolean isActive;
/*
* The time at which the incident was activated in ISO8601 format.
*/
private OffsetDateTime activatedTime;
/*
* The time at which the incident was resolved in ISO8601 format. If null, it means the incident is still active.
*/
private OffsetDateTime resolvedTime;
/**
* Creates an instance of IncidentInner class.
*/
public IncidentInner() {
}
/**
* Get the name property: Incident name.
*
* @return the name value.
*/
public String name() {
return this.name;
}
/**
* Get the ruleName property: Rule name that is associated with the incident.
*
* @return the ruleName value.
*/
public String ruleName() {
return this.ruleName;
}
/**
* Get the isActive property: A boolean to indicate whether the incident is active or resolved.
*
* @return the isActive value.
*/
public Boolean isActive() {
return this.isActive;
}
/**
* Get the activatedTime property: The time at which the incident was activated in ISO8601 format.
*
* @return the activatedTime value.
*/
public OffsetDateTime activatedTime() {
return this.activatedTime;
}
/**
* Get the resolvedTime property: The time at which the incident was resolved in ISO8601 format. If null, it means
* the incident is still active.
*
* @return the resolvedTime value.
*/
public OffsetDateTime resolvedTime() {
return this.resolvedTime;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of IncidentInner from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of IncidentInner if the JsonReader was pointing to an instance of it, or null if it was
* pointing to JSON null.
* @throws IOException If an error occurs while reading the IncidentInner.
*/
public static IncidentInner fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
IncidentInner deserializedIncidentInner = new IncidentInner();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("name".equals(fieldName)) {
deserializedIncidentInner.name = reader.getString();
} else if ("ruleName".equals(fieldName)) {
deserializedIncidentInner.ruleName = reader.getString();
} else if ("isActive".equals(fieldName)) {
deserializedIncidentInner.isActive = reader.getNullable(JsonReader::getBoolean);
} else if ("activatedTime".equals(fieldName)) {
deserializedIncidentInner.activatedTime = reader
.getNullable(nonNullReader -> CoreUtils.parseBestOffsetDateTime(nonNullReader.getString()));
} else if ("resolvedTime".equals(fieldName)) {
deserializedIncidentInner.resolvedTime = reader
.getNullable(nonNullReader -> CoreUtils.parseBestOffsetDateTime(nonNullReader.getString()));
} else {
reader.skipChildren();
}
}
return deserializedIncidentInner;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy