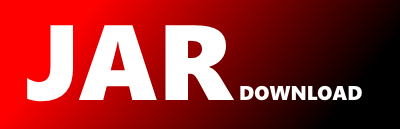
com.azure.resourcemanager.monitor.models.AccessModeSettings Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-monitor Show documentation
Show all versions of azure-resourcemanager-monitor Show documentation
This package contains Microsoft Azure Monitor SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.monitor.models;
import com.azure.core.annotation.Fluent;
import com.azure.core.util.logging.ClientLogger;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
import java.util.List;
/**
* Properties that define the scope private link mode settings.
*/
@Fluent
public final class AccessModeSettings implements JsonSerializable {
/*
* Specifies the default access mode of queries through associated private endpoints in scope. If not specified
* default value is 'Open'. You can override this default setting for a specific private endpoint connection by
* adding an exclusion in the 'exclusions' array.
*/
private AccessMode queryAccessMode;
/*
* Specifies the default access mode of ingestion through associated private endpoints in scope. If not specified
* default value is 'Open'. You can override this default setting for a specific private endpoint connection by
* adding an exclusion in the 'exclusions' array.
*/
private AccessMode ingestionAccessMode;
/*
* List of exclusions that override the default access mode settings for specific private endpoint connections.
*/
private List exclusions;
/**
* Creates an instance of AccessModeSettings class.
*/
public AccessModeSettings() {
}
/**
* Get the queryAccessMode property: Specifies the default access mode of queries through associated private
* endpoints in scope. If not specified default value is 'Open'. You can override this default setting for a
* specific private endpoint connection by adding an exclusion in the 'exclusions' array.
*
* @return the queryAccessMode value.
*/
public AccessMode queryAccessMode() {
return this.queryAccessMode;
}
/**
* Set the queryAccessMode property: Specifies the default access mode of queries through associated private
* endpoints in scope. If not specified default value is 'Open'. You can override this default setting for a
* specific private endpoint connection by adding an exclusion in the 'exclusions' array.
*
* @param queryAccessMode the queryAccessMode value to set.
* @return the AccessModeSettings object itself.
*/
public AccessModeSettings withQueryAccessMode(AccessMode queryAccessMode) {
this.queryAccessMode = queryAccessMode;
return this;
}
/**
* Get the ingestionAccessMode property: Specifies the default access mode of ingestion through associated private
* endpoints in scope. If not specified default value is 'Open'. You can override this default setting for a
* specific private endpoint connection by adding an exclusion in the 'exclusions' array.
*
* @return the ingestionAccessMode value.
*/
public AccessMode ingestionAccessMode() {
return this.ingestionAccessMode;
}
/**
* Set the ingestionAccessMode property: Specifies the default access mode of ingestion through associated private
* endpoints in scope. If not specified default value is 'Open'. You can override this default setting for a
* specific private endpoint connection by adding an exclusion in the 'exclusions' array.
*
* @param ingestionAccessMode the ingestionAccessMode value to set.
* @return the AccessModeSettings object itself.
*/
public AccessModeSettings withIngestionAccessMode(AccessMode ingestionAccessMode) {
this.ingestionAccessMode = ingestionAccessMode;
return this;
}
/**
* Get the exclusions property: List of exclusions that override the default access mode settings for specific
* private endpoint connections.
*
* @return the exclusions value.
*/
public List exclusions() {
return this.exclusions;
}
/**
* Set the exclusions property: List of exclusions that override the default access mode settings for specific
* private endpoint connections.
*
* @param exclusions the exclusions value to set.
* @return the AccessModeSettings object itself.
*/
public AccessModeSettings withExclusions(List exclusions) {
this.exclusions = exclusions;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (queryAccessMode() == null) {
throw LOGGER.atError()
.log(new IllegalArgumentException(
"Missing required property queryAccessMode in model AccessModeSettings"));
}
if (ingestionAccessMode() == null) {
throw LOGGER.atError()
.log(new IllegalArgumentException(
"Missing required property ingestionAccessMode in model AccessModeSettings"));
}
if (exclusions() != null) {
exclusions().forEach(e -> e.validate());
}
}
private static final ClientLogger LOGGER = new ClientLogger(AccessModeSettings.class);
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("queryAccessMode",
this.queryAccessMode == null ? null : this.queryAccessMode.toString());
jsonWriter.writeStringField("ingestionAccessMode",
this.ingestionAccessMode == null ? null : this.ingestionAccessMode.toString());
jsonWriter.writeArrayField("exclusions", this.exclusions, (writer, element) -> writer.writeJson(element));
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of AccessModeSettings from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of AccessModeSettings if the JsonReader was pointing to an instance of it, or null if it was
* pointing to JSON null.
* @throws IllegalStateException If the deserialized JSON object was missing any required properties.
* @throws IOException If an error occurs while reading the AccessModeSettings.
*/
public static AccessModeSettings fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
AccessModeSettings deserializedAccessModeSettings = new AccessModeSettings();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("queryAccessMode".equals(fieldName)) {
deserializedAccessModeSettings.queryAccessMode = AccessMode.fromString(reader.getString());
} else if ("ingestionAccessMode".equals(fieldName)) {
deserializedAccessModeSettings.ingestionAccessMode = AccessMode.fromString(reader.getString());
} else if ("exclusions".equals(fieldName)) {
List exclusions
= reader.readArray(reader1 -> AccessModeSettingsExclusion.fromJson(reader1));
deserializedAccessModeSettings.exclusions = exclusions;
} else {
reader.skipChildren();
}
}
return deserializedAccessModeSettings;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy