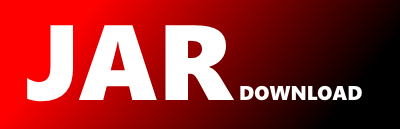
com.azure.resourcemanager.monitor.models.Condition Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-monitor Show documentation
Show all versions of azure-resourcemanager-monitor Show documentation
This package contains Microsoft Azure Monitor SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.monitor.models;
import com.azure.core.annotation.Fluent;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
import java.util.List;
/**
* A condition of the scheduled query rule.
*/
@Fluent
public final class Condition implements JsonSerializable {
/*
* Log query alert
*/
private String query;
/*
* Aggregation type. Relevant and required only for rules of the kind LogAlert.
*/
private TimeAggregation timeAggregation;
/*
* The column containing the metric measure number. Relevant only for rules of the kind LogAlert.
*/
private String metricMeasureColumn;
/*
* The column containing the resource id. The content of the column must be a uri formatted as resource id. Relevant
* only for rules of the kind LogAlert.
*/
private String resourceIdColumn;
/*
* List of Dimensions conditions
*/
private List dimensions;
/*
* The criteria operator. Relevant and required only for rules of the kind LogAlert.
*/
private ConditionOperator operator;
/*
* the criteria threshold value that activates the alert. Relevant and required only for rules of the kind LogAlert.
*/
private Double threshold;
/*
* The minimum number of violations required within the selected lookback time window required to raise an alert.
* Relevant only for rules of the kind LogAlert.
*/
private ConditionFailingPeriods failingPeriods;
/*
* The name of the metric to be sent. Relevant and required only for rules of the kind LogToMetric.
*/
private String metricName;
/**
* Creates an instance of Condition class.
*/
public Condition() {
}
/**
* Get the query property: Log query alert.
*
* @return the query value.
*/
public String query() {
return this.query;
}
/**
* Set the query property: Log query alert.
*
* @param query the query value to set.
* @return the Condition object itself.
*/
public Condition withQuery(String query) {
this.query = query;
return this;
}
/**
* Get the timeAggregation property: Aggregation type. Relevant and required only for rules of the kind LogAlert.
*
* @return the timeAggregation value.
*/
public TimeAggregation timeAggregation() {
return this.timeAggregation;
}
/**
* Set the timeAggregation property: Aggregation type. Relevant and required only for rules of the kind LogAlert.
*
* @param timeAggregation the timeAggregation value to set.
* @return the Condition object itself.
*/
public Condition withTimeAggregation(TimeAggregation timeAggregation) {
this.timeAggregation = timeAggregation;
return this;
}
/**
* Get the metricMeasureColumn property: The column containing the metric measure number. Relevant only for rules of
* the kind LogAlert.
*
* @return the metricMeasureColumn value.
*/
public String metricMeasureColumn() {
return this.metricMeasureColumn;
}
/**
* Set the metricMeasureColumn property: The column containing the metric measure number. Relevant only for rules of
* the kind LogAlert.
*
* @param metricMeasureColumn the metricMeasureColumn value to set.
* @return the Condition object itself.
*/
public Condition withMetricMeasureColumn(String metricMeasureColumn) {
this.metricMeasureColumn = metricMeasureColumn;
return this;
}
/**
* Get the resourceIdColumn property: The column containing the resource id. The content of the column must be a uri
* formatted as resource id. Relevant only for rules of the kind LogAlert.
*
* @return the resourceIdColumn value.
*/
public String resourceIdColumn() {
return this.resourceIdColumn;
}
/**
* Set the resourceIdColumn property: The column containing the resource id. The content of the column must be a uri
* formatted as resource id. Relevant only for rules of the kind LogAlert.
*
* @param resourceIdColumn the resourceIdColumn value to set.
* @return the Condition object itself.
*/
public Condition withResourceIdColumn(String resourceIdColumn) {
this.resourceIdColumn = resourceIdColumn;
return this;
}
/**
* Get the dimensions property: List of Dimensions conditions.
*
* @return the dimensions value.
*/
public List dimensions() {
return this.dimensions;
}
/**
* Set the dimensions property: List of Dimensions conditions.
*
* @param dimensions the dimensions value to set.
* @return the Condition object itself.
*/
public Condition withDimensions(List dimensions) {
this.dimensions = dimensions;
return this;
}
/**
* Get the operator property: The criteria operator. Relevant and required only for rules of the kind LogAlert.
*
* @return the operator value.
*/
public ConditionOperator operator() {
return this.operator;
}
/**
* Set the operator property: The criteria operator. Relevant and required only for rules of the kind LogAlert.
*
* @param operator the operator value to set.
* @return the Condition object itself.
*/
public Condition withOperator(ConditionOperator operator) {
this.operator = operator;
return this;
}
/**
* Get the threshold property: the criteria threshold value that activates the alert. Relevant and required only for
* rules of the kind LogAlert.
*
* @return the threshold value.
*/
public Double threshold() {
return this.threshold;
}
/**
* Set the threshold property: the criteria threshold value that activates the alert. Relevant and required only for
* rules of the kind LogAlert.
*
* @param threshold the threshold value to set.
* @return the Condition object itself.
*/
public Condition withThreshold(Double threshold) {
this.threshold = threshold;
return this;
}
/**
* Get the failingPeriods property: The minimum number of violations required within the selected lookback time
* window required to raise an alert. Relevant only for rules of the kind LogAlert.
*
* @return the failingPeriods value.
*/
public ConditionFailingPeriods failingPeriods() {
return this.failingPeriods;
}
/**
* Set the failingPeriods property: The minimum number of violations required within the selected lookback time
* window required to raise an alert. Relevant only for rules of the kind LogAlert.
*
* @param failingPeriods the failingPeriods value to set.
* @return the Condition object itself.
*/
public Condition withFailingPeriods(ConditionFailingPeriods failingPeriods) {
this.failingPeriods = failingPeriods;
return this;
}
/**
* Get the metricName property: The name of the metric to be sent. Relevant and required only for rules of the kind
* LogToMetric.
*
* @return the metricName value.
*/
public String metricName() {
return this.metricName;
}
/**
* Set the metricName property: The name of the metric to be sent. Relevant and required only for rules of the kind
* LogToMetric.
*
* @param metricName the metricName value to set.
* @return the Condition object itself.
*/
public Condition withMetricName(String metricName) {
this.metricName = metricName;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (dimensions() != null) {
dimensions().forEach(e -> e.validate());
}
if (failingPeriods() != null) {
failingPeriods().validate();
}
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("query", this.query);
jsonWriter.writeStringField("timeAggregation",
this.timeAggregation == null ? null : this.timeAggregation.toString());
jsonWriter.writeStringField("metricMeasureColumn", this.metricMeasureColumn);
jsonWriter.writeStringField("resourceIdColumn", this.resourceIdColumn);
jsonWriter.writeArrayField("dimensions", this.dimensions, (writer, element) -> writer.writeJson(element));
jsonWriter.writeStringField("operator", this.operator == null ? null : this.operator.toString());
jsonWriter.writeNumberField("threshold", this.threshold);
jsonWriter.writeJsonField("failingPeriods", this.failingPeriods);
jsonWriter.writeStringField("metricName", this.metricName);
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of Condition from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of Condition if the JsonReader was pointing to an instance of it, or null if it was pointing
* to JSON null.
* @throws IOException If an error occurs while reading the Condition.
*/
public static Condition fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
Condition deserializedCondition = new Condition();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("query".equals(fieldName)) {
deserializedCondition.query = reader.getString();
} else if ("timeAggregation".equals(fieldName)) {
deserializedCondition.timeAggregation = TimeAggregation.fromString(reader.getString());
} else if ("metricMeasureColumn".equals(fieldName)) {
deserializedCondition.metricMeasureColumn = reader.getString();
} else if ("resourceIdColumn".equals(fieldName)) {
deserializedCondition.resourceIdColumn = reader.getString();
} else if ("dimensions".equals(fieldName)) {
List dimensions = reader.readArray(reader1 -> Dimension.fromJson(reader1));
deserializedCondition.dimensions = dimensions;
} else if ("operator".equals(fieldName)) {
deserializedCondition.operator = ConditionOperator.fromString(reader.getString());
} else if ("threshold".equals(fieldName)) {
deserializedCondition.threshold = reader.getNullable(JsonReader::getDouble);
} else if ("failingPeriods".equals(fieldName)) {
deserializedCondition.failingPeriods = ConditionFailingPeriods.fromJson(reader);
} else if ("metricName".equals(fieldName)) {
deserializedCondition.metricName = reader.getString();
} else {
reader.skipChildren();
}
}
return deserializedCondition;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy