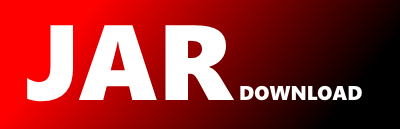
com.azure.resourcemanager.monitor.models.AccessModeSettingsExclusion Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-monitor Show documentation
Show all versions of azure-resourcemanager-monitor Show documentation
This package contains Microsoft Azure Monitor SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.monitor.models;
import com.azure.core.annotation.Fluent;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
/**
* Properties that define the scope private link mode settings exclusion item. This setting applies to a specific
* private endpoint connection and overrides the default settings for that private endpoint connection.
*/
@Fluent
public final class AccessModeSettingsExclusion implements JsonSerializable {
/*
* The private endpoint connection name associated to the private endpoint on which we want to apply the specific
* access mode settings.
*/
private String privateEndpointConnectionName;
/*
* Specifies the access mode of queries through the specified private endpoint connection in the exclusion.
*/
private AccessMode queryAccessMode;
/*
* Specifies the access mode of ingestion through the specified private endpoint connection in the exclusion.
*/
private AccessMode ingestionAccessMode;
/**
* Creates an instance of AccessModeSettingsExclusion class.
*/
public AccessModeSettingsExclusion() {
}
/**
* Get the privateEndpointConnectionName property: The private endpoint connection name associated to the private
* endpoint on which we want to apply the specific access mode settings.
*
* @return the privateEndpointConnectionName value.
*/
public String privateEndpointConnectionName() {
return this.privateEndpointConnectionName;
}
/**
* Set the privateEndpointConnectionName property: The private endpoint connection name associated to the private
* endpoint on which we want to apply the specific access mode settings.
*
* @param privateEndpointConnectionName the privateEndpointConnectionName value to set.
* @return the AccessModeSettingsExclusion object itself.
*/
public AccessModeSettingsExclusion withPrivateEndpointConnectionName(String privateEndpointConnectionName) {
this.privateEndpointConnectionName = privateEndpointConnectionName;
return this;
}
/**
* Get the queryAccessMode property: Specifies the access mode of queries through the specified private endpoint
* connection in the exclusion.
*
* @return the queryAccessMode value.
*/
public AccessMode queryAccessMode() {
return this.queryAccessMode;
}
/**
* Set the queryAccessMode property: Specifies the access mode of queries through the specified private endpoint
* connection in the exclusion.
*
* @param queryAccessMode the queryAccessMode value to set.
* @return the AccessModeSettingsExclusion object itself.
*/
public AccessModeSettingsExclusion withQueryAccessMode(AccessMode queryAccessMode) {
this.queryAccessMode = queryAccessMode;
return this;
}
/**
* Get the ingestionAccessMode property: Specifies the access mode of ingestion through the specified private
* endpoint connection in the exclusion.
*
* @return the ingestionAccessMode value.
*/
public AccessMode ingestionAccessMode() {
return this.ingestionAccessMode;
}
/**
* Set the ingestionAccessMode property: Specifies the access mode of ingestion through the specified private
* endpoint connection in the exclusion.
*
* @param ingestionAccessMode the ingestionAccessMode value to set.
* @return the AccessModeSettingsExclusion object itself.
*/
public AccessModeSettingsExclusion withIngestionAccessMode(AccessMode ingestionAccessMode) {
this.ingestionAccessMode = ingestionAccessMode;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("privateEndpointConnectionName", this.privateEndpointConnectionName);
jsonWriter.writeStringField("queryAccessMode",
this.queryAccessMode == null ? null : this.queryAccessMode.toString());
jsonWriter.writeStringField("ingestionAccessMode",
this.ingestionAccessMode == null ? null : this.ingestionAccessMode.toString());
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of AccessModeSettingsExclusion from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of AccessModeSettingsExclusion if the JsonReader was pointing to an instance of it, or null
* if it was pointing to JSON null.
* @throws IOException If an error occurs while reading the AccessModeSettingsExclusion.
*/
public static AccessModeSettingsExclusion fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
AccessModeSettingsExclusion deserializedAccessModeSettingsExclusion = new AccessModeSettingsExclusion();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("privateEndpointConnectionName".equals(fieldName)) {
deserializedAccessModeSettingsExclusion.privateEndpointConnectionName = reader.getString();
} else if ("queryAccessMode".equals(fieldName)) {
deserializedAccessModeSettingsExclusion.queryAccessMode = AccessMode.fromString(reader.getString());
} else if ("ingestionAccessMode".equals(fieldName)) {
deserializedAccessModeSettingsExclusion.ingestionAccessMode
= AccessMode.fromString(reader.getString());
} else {
reader.skipChildren();
}
}
return deserializedAccessModeSettingsExclusion;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy