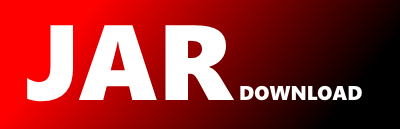
com.azure.resourcemanager.monitor.fluent.models.EventDataInner Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-monitor Show documentation
Show all versions of azure-resourcemanager-monitor Show documentation
This package contains Microsoft Azure Monitor SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.monitor.fluent.models;
import com.azure.core.annotation.Immutable;
import com.azure.core.util.CoreUtils;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import com.azure.resourcemanager.monitor.models.EventLevel;
import com.azure.resourcemanager.monitor.models.HttpRequestInfo;
import com.azure.resourcemanager.monitor.models.SenderAuthorization;
import java.io.IOException;
import java.time.OffsetDateTime;
import java.util.Map;
/**
* The Azure event log entries are of type EventData.
*/
@Immutable
public final class EventDataInner implements JsonSerializable {
/*
* The sender authorization information.
*/
private SenderAuthorization authorization;
/*
* key value pairs to identify ARM permissions.
*/
private Map claims;
/*
* the email address of the user who has performed the operation, the UPN claim or SPN claim based on availability.
*/
private String caller;
/*
* the description of the event.
*/
private String description;
/*
* the Id of this event as required by ARM for RBAC. It contains the EventDataID and a timestamp information.
*/
private String id;
/*
* the event data Id. This is a unique identifier for an event.
*/
private String eventDataId;
/*
* the correlation Id, usually a GUID in the string format. The correlation Id is shared among the events that
* belong to the same uber operation.
*/
private String correlationId;
/*
* the event name. This value should not be confused with OperationName. For practical purposes, OperationName might
* be more appealing to end users.
*/
private LocalizableStringInner eventName;
/*
* the event category.
*/
private LocalizableStringInner category;
/*
* the HTTP request info. Usually includes the 'clientRequestId', 'clientIpAddress' (IP address of the user who
* initiated the event) and 'method' (HTTP method e.g. PUT).
*/
private HttpRequestInfo httpRequest;
/*
* the event level
*/
private EventLevel level;
/*
* the resource group name of the impacted resource.
*/
private String resourceGroupName;
/*
* the resource provider name of the impacted resource.
*/
private LocalizableStringInner resourceProviderName;
/*
* the resource uri that uniquely identifies the resource that caused this event.
*/
private String resourceId;
/*
* the resource type
*/
private LocalizableStringInner resourceType;
/*
* It is usually a GUID shared among the events corresponding to single operation. This value should not be confused
* with EventName.
*/
private String operationId;
/*
* the operation name.
*/
private LocalizableStringInner operationName;
/*
* the set of pairs (usually a Dictionary) that includes details about the event.
*/
private Map properties;
/*
* a string describing the status of the operation. Some typical values are: Started, In progress, Succeeded,
* Failed, Resolved.
*/
private LocalizableStringInner status;
/*
* the event sub status. Most of the time, when included, this captures the HTTP status code of the REST call.
* Common values are: OK (HTTP Status Code: 200), Created (HTTP Status Code: 201), Accepted (HTTP Status Code: 202),
* No Content (HTTP Status Code: 204), Bad Request(HTTP Status Code: 400), Not Found (HTTP Status Code: 404),
* Conflict (HTTP Status Code: 409), Internal Server Error (HTTP Status Code: 500), Service Unavailable (HTTP Status
* Code:503), Gateway Timeout (HTTP Status Code: 504)
*/
private LocalizableStringInner subStatus;
/*
* the timestamp of when the event was generated by the Azure service processing the request corresponding the
* event. It in ISO 8601 format.
*/
private OffsetDateTime eventTimestamp;
/*
* the timestamp of when the event became available for querying via this API. It is in ISO 8601 format. This value
* should not be confused eventTimestamp. As there might be a delay between the occurrence time of the event, and
* the time that the event is submitted to the Azure logging infrastructure.
*/
private OffsetDateTime submissionTimestamp;
/*
* the Azure subscription Id usually a GUID.
*/
private String subscriptionId;
/*
* the Azure tenant Id
*/
private String tenantId;
/**
* Creates an instance of EventDataInner class.
*/
public EventDataInner() {
}
/**
* Get the authorization property: The sender authorization information.
*
* @return the authorization value.
*/
public SenderAuthorization authorization() {
return this.authorization;
}
/**
* Get the claims property: key value pairs to identify ARM permissions.
*
* @return the claims value.
*/
public Map claims() {
return this.claims;
}
/**
* Get the caller property: the email address of the user who has performed the operation, the UPN claim or SPN
* claim based on availability.
*
* @return the caller value.
*/
public String caller() {
return this.caller;
}
/**
* Get the description property: the description of the event.
*
* @return the description value.
*/
public String description() {
return this.description;
}
/**
* Get the id property: the Id of this event as required by ARM for RBAC. It contains the EventDataID and a
* timestamp information.
*
* @return the id value.
*/
public String id() {
return this.id;
}
/**
* Get the eventDataId property: the event data Id. This is a unique identifier for an event.
*
* @return the eventDataId value.
*/
public String eventDataId() {
return this.eventDataId;
}
/**
* Get the correlationId property: the correlation Id, usually a GUID in the string format. The correlation Id is
* shared among the events that belong to the same uber operation.
*
* @return the correlationId value.
*/
public String correlationId() {
return this.correlationId;
}
/**
* Get the eventName property: the event name. This value should not be confused with OperationName. For practical
* purposes, OperationName might be more appealing to end users.
*
* @return the eventName value.
*/
public LocalizableStringInner eventName() {
return this.eventName;
}
/**
* Get the category property: the event category.
*
* @return the category value.
*/
public LocalizableStringInner category() {
return this.category;
}
/**
* Get the httpRequest property: the HTTP request info. Usually includes the 'clientRequestId', 'clientIpAddress'
* (IP address of the user who initiated the event) and 'method' (HTTP method e.g. PUT).
*
* @return the httpRequest value.
*/
public HttpRequestInfo httpRequest() {
return this.httpRequest;
}
/**
* Get the level property: the event level.
*
* @return the level value.
*/
public EventLevel level() {
return this.level;
}
/**
* Get the resourceGroupName property: the resource group name of the impacted resource.
*
* @return the resourceGroupName value.
*/
public String resourceGroupName() {
return this.resourceGroupName;
}
/**
* Get the resourceProviderName property: the resource provider name of the impacted resource.
*
* @return the resourceProviderName value.
*/
public LocalizableStringInner resourceProviderName() {
return this.resourceProviderName;
}
/**
* Get the resourceId property: the resource uri that uniquely identifies the resource that caused this event.
*
* @return the resourceId value.
*/
public String resourceId() {
return this.resourceId;
}
/**
* Get the resourceType property: the resource type.
*
* @return the resourceType value.
*/
public LocalizableStringInner resourceType() {
return this.resourceType;
}
/**
* Get the operationId property: It is usually a GUID shared among the events corresponding to single operation.
* This value should not be confused with EventName.
*
* @return the operationId value.
*/
public String operationId() {
return this.operationId;
}
/**
* Get the operationName property: the operation name.
*
* @return the operationName value.
*/
public LocalizableStringInner operationName() {
return this.operationName;
}
/**
* Get the properties property: the set of <Key, Value> pairs (usually a Dictionary<String, String>)
* that includes details about the event.
*
* @return the properties value.
*/
public Map properties() {
return this.properties;
}
/**
* Get the status property: a string describing the status of the operation. Some typical values are: Started, In
* progress, Succeeded, Failed, Resolved.
*
* @return the status value.
*/
public LocalizableStringInner status() {
return this.status;
}
/**
* Get the subStatus property: the event sub status. Most of the time, when included, this captures the HTTP status
* code of the REST call. Common values are: OK (HTTP Status Code: 200), Created (HTTP Status Code: 201), Accepted
* (HTTP Status Code: 202), No Content (HTTP Status Code: 204), Bad Request(HTTP Status Code: 400), Not Found (HTTP
* Status Code: 404), Conflict (HTTP Status Code: 409), Internal Server Error (HTTP Status Code: 500), Service
* Unavailable (HTTP Status Code:503), Gateway Timeout (HTTP Status Code: 504).
*
* @return the subStatus value.
*/
public LocalizableStringInner subStatus() {
return this.subStatus;
}
/**
* Get the eventTimestamp property: the timestamp of when the event was generated by the Azure service processing
* the request corresponding the event. It in ISO 8601 format.
*
* @return the eventTimestamp value.
*/
public OffsetDateTime eventTimestamp() {
return this.eventTimestamp;
}
/**
* Get the submissionTimestamp property: the timestamp of when the event became available for querying via this API.
* It is in ISO 8601 format. This value should not be confused eventTimestamp. As there might be a delay between the
* occurrence time of the event, and the time that the event is submitted to the Azure logging infrastructure.
*
* @return the submissionTimestamp value.
*/
public OffsetDateTime submissionTimestamp() {
return this.submissionTimestamp;
}
/**
* Get the subscriptionId property: the Azure subscription Id usually a GUID.
*
* @return the subscriptionId value.
*/
public String subscriptionId() {
return this.subscriptionId;
}
/**
* Get the tenantId property: the Azure tenant Id.
*
* @return the tenantId value.
*/
public String tenantId() {
return this.tenantId;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (authorization() != null) {
authorization().validate();
}
if (eventName() != null) {
eventName().validate();
}
if (category() != null) {
category().validate();
}
if (httpRequest() != null) {
httpRequest().validate();
}
if (resourceProviderName() != null) {
resourceProviderName().validate();
}
if (resourceType() != null) {
resourceType().validate();
}
if (operationName() != null) {
operationName().validate();
}
if (status() != null) {
status().validate();
}
if (subStatus() != null) {
subStatus().validate();
}
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of EventDataInner from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of EventDataInner if the JsonReader was pointing to an instance of it, or null if it was
* pointing to JSON null.
* @throws IOException If an error occurs while reading the EventDataInner.
*/
public static EventDataInner fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
EventDataInner deserializedEventDataInner = new EventDataInner();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("authorization".equals(fieldName)) {
deserializedEventDataInner.authorization = SenderAuthorization.fromJson(reader);
} else if ("claims".equals(fieldName)) {
Map claims = reader.readMap(reader1 -> reader1.getString());
deserializedEventDataInner.claims = claims;
} else if ("caller".equals(fieldName)) {
deserializedEventDataInner.caller = reader.getString();
} else if ("description".equals(fieldName)) {
deserializedEventDataInner.description = reader.getString();
} else if ("id".equals(fieldName)) {
deserializedEventDataInner.id = reader.getString();
} else if ("eventDataId".equals(fieldName)) {
deserializedEventDataInner.eventDataId = reader.getString();
} else if ("correlationId".equals(fieldName)) {
deserializedEventDataInner.correlationId = reader.getString();
} else if ("eventName".equals(fieldName)) {
deserializedEventDataInner.eventName = LocalizableStringInner.fromJson(reader);
} else if ("category".equals(fieldName)) {
deserializedEventDataInner.category = LocalizableStringInner.fromJson(reader);
} else if ("httpRequest".equals(fieldName)) {
deserializedEventDataInner.httpRequest = HttpRequestInfo.fromJson(reader);
} else if ("level".equals(fieldName)) {
deserializedEventDataInner.level = EventLevel.fromString(reader.getString());
} else if ("resourceGroupName".equals(fieldName)) {
deserializedEventDataInner.resourceGroupName = reader.getString();
} else if ("resourceProviderName".equals(fieldName)) {
deserializedEventDataInner.resourceProviderName = LocalizableStringInner.fromJson(reader);
} else if ("resourceId".equals(fieldName)) {
deserializedEventDataInner.resourceId = reader.getString();
} else if ("resourceType".equals(fieldName)) {
deserializedEventDataInner.resourceType = LocalizableStringInner.fromJson(reader);
} else if ("operationId".equals(fieldName)) {
deserializedEventDataInner.operationId = reader.getString();
} else if ("operationName".equals(fieldName)) {
deserializedEventDataInner.operationName = LocalizableStringInner.fromJson(reader);
} else if ("properties".equals(fieldName)) {
Map properties = reader.readMap(reader1 -> reader1.getString());
deserializedEventDataInner.properties = properties;
} else if ("status".equals(fieldName)) {
deserializedEventDataInner.status = LocalizableStringInner.fromJson(reader);
} else if ("subStatus".equals(fieldName)) {
deserializedEventDataInner.subStatus = LocalizableStringInner.fromJson(reader);
} else if ("eventTimestamp".equals(fieldName)) {
deserializedEventDataInner.eventTimestamp = reader
.getNullable(nonNullReader -> CoreUtils.parseBestOffsetDateTime(nonNullReader.getString()));
} else if ("submissionTimestamp".equals(fieldName)) {
deserializedEventDataInner.submissionTimestamp = reader
.getNullable(nonNullReader -> CoreUtils.parseBestOffsetDateTime(nonNullReader.getString()));
} else if ("subscriptionId".equals(fieldName)) {
deserializedEventDataInner.subscriptionId = reader.getString();
} else if ("tenantId".equals(fieldName)) {
deserializedEventDataInner.tenantId = reader.getString();
} else {
reader.skipChildren();
}
}
return deserializedEventDataInner;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy