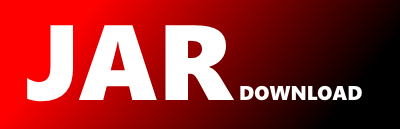
com.azure.resourcemanager.monitor.fluent.models.MetricAlertPropertiesPatch Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-monitor Show documentation
Show all versions of azure-resourcemanager-monitor Show documentation
This package contains Microsoft Azure Monitor SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.monitor.fluent.models;
import com.azure.core.annotation.Fluent;
import com.azure.core.util.CoreUtils;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import com.azure.resourcemanager.monitor.models.MetricAlertAction;
import com.azure.resourcemanager.monitor.models.MetricAlertCriteria;
import java.io.IOException;
import java.time.Duration;
import java.time.OffsetDateTime;
import java.util.List;
/**
* An alert rule properties for patch.
*/
@Fluent
public final class MetricAlertPropertiesPatch implements JsonSerializable {
/*
* the description of the metric alert that will be included in the alert email.
*/
private String description;
/*
* Alert severity {0, 1, 2, 3, 4}
*/
private Integer severity;
/*
* the flag that indicates whether the metric alert is enabled.
*/
private Boolean enabled;
/*
* the list of resource id's that this metric alert is scoped to.
*/
private List scopes;
/*
* how often the metric alert is evaluated represented in ISO 8601 duration format.
*/
private Duration evaluationFrequency;
/*
* the period of time (in ISO 8601 duration format) that is used to monitor alert activity based on the threshold.
*/
private Duration windowSize;
/*
* the resource type of the target resource(s) on which the alert is created/updated. Mandatory for
* MultipleResourceMultipleMetricCriteria.
*/
private String targetResourceType;
/*
* the region of the target resource(s) on which the alert is created/updated. Mandatory for
* MultipleResourceMultipleMetricCriteria.
*/
private String targetResourceRegion;
/*
* defines the specific alert criteria information.
*/
private MetricAlertCriteria criteria;
/*
* the flag that indicates whether the alert should be auto resolved or not. The default is true.
*/
private Boolean autoMitigate;
/*
* the array of actions that are performed when the alert rule becomes active, and when an alert condition is
* resolved.
*/
private List actions;
/*
* Last time the rule was updated in ISO8601 format.
*/
private OffsetDateTime lastUpdatedTime;
/*
* the value indicating whether this alert rule is migrated.
*/
private Boolean isMigrated;
/**
* Creates an instance of MetricAlertPropertiesPatch class.
*/
public MetricAlertPropertiesPatch() {
}
/**
* Get the description property: the description of the metric alert that will be included in the alert email.
*
* @return the description value.
*/
public String description() {
return this.description;
}
/**
* Set the description property: the description of the metric alert that will be included in the alert email.
*
* @param description the description value to set.
* @return the MetricAlertPropertiesPatch object itself.
*/
public MetricAlertPropertiesPatch withDescription(String description) {
this.description = description;
return this;
}
/**
* Get the severity property: Alert severity {0, 1, 2, 3, 4}.
*
* @return the severity value.
*/
public Integer severity() {
return this.severity;
}
/**
* Set the severity property: Alert severity {0, 1, 2, 3, 4}.
*
* @param severity the severity value to set.
* @return the MetricAlertPropertiesPatch object itself.
*/
public MetricAlertPropertiesPatch withSeverity(Integer severity) {
this.severity = severity;
return this;
}
/**
* Get the enabled property: the flag that indicates whether the metric alert is enabled.
*
* @return the enabled value.
*/
public Boolean enabled() {
return this.enabled;
}
/**
* Set the enabled property: the flag that indicates whether the metric alert is enabled.
*
* @param enabled the enabled value to set.
* @return the MetricAlertPropertiesPatch object itself.
*/
public MetricAlertPropertiesPatch withEnabled(Boolean enabled) {
this.enabled = enabled;
return this;
}
/**
* Get the scopes property: the list of resource id's that this metric alert is scoped to.
*
* @return the scopes value.
*/
public List scopes() {
return this.scopes;
}
/**
* Set the scopes property: the list of resource id's that this metric alert is scoped to.
*
* @param scopes the scopes value to set.
* @return the MetricAlertPropertiesPatch object itself.
*/
public MetricAlertPropertiesPatch withScopes(List scopes) {
this.scopes = scopes;
return this;
}
/**
* Get the evaluationFrequency property: how often the metric alert is evaluated represented in ISO 8601 duration
* format.
*
* @return the evaluationFrequency value.
*/
public Duration evaluationFrequency() {
return this.evaluationFrequency;
}
/**
* Set the evaluationFrequency property: how often the metric alert is evaluated represented in ISO 8601 duration
* format.
*
* @param evaluationFrequency the evaluationFrequency value to set.
* @return the MetricAlertPropertiesPatch object itself.
*/
public MetricAlertPropertiesPatch withEvaluationFrequency(Duration evaluationFrequency) {
this.evaluationFrequency = evaluationFrequency;
return this;
}
/**
* Get the windowSize property: the period of time (in ISO 8601 duration format) that is used to monitor alert
* activity based on the threshold.
*
* @return the windowSize value.
*/
public Duration windowSize() {
return this.windowSize;
}
/**
* Set the windowSize property: the period of time (in ISO 8601 duration format) that is used to monitor alert
* activity based on the threshold.
*
* @param windowSize the windowSize value to set.
* @return the MetricAlertPropertiesPatch object itself.
*/
public MetricAlertPropertiesPatch withWindowSize(Duration windowSize) {
this.windowSize = windowSize;
return this;
}
/**
* Get the targetResourceType property: the resource type of the target resource(s) on which the alert is
* created/updated. Mandatory for MultipleResourceMultipleMetricCriteria.
*
* @return the targetResourceType value.
*/
public String targetResourceType() {
return this.targetResourceType;
}
/**
* Set the targetResourceType property: the resource type of the target resource(s) on which the alert is
* created/updated. Mandatory for MultipleResourceMultipleMetricCriteria.
*
* @param targetResourceType the targetResourceType value to set.
* @return the MetricAlertPropertiesPatch object itself.
*/
public MetricAlertPropertiesPatch withTargetResourceType(String targetResourceType) {
this.targetResourceType = targetResourceType;
return this;
}
/**
* Get the targetResourceRegion property: the region of the target resource(s) on which the alert is
* created/updated. Mandatory for MultipleResourceMultipleMetricCriteria.
*
* @return the targetResourceRegion value.
*/
public String targetResourceRegion() {
return this.targetResourceRegion;
}
/**
* Set the targetResourceRegion property: the region of the target resource(s) on which the alert is
* created/updated. Mandatory for MultipleResourceMultipleMetricCriteria.
*
* @param targetResourceRegion the targetResourceRegion value to set.
* @return the MetricAlertPropertiesPatch object itself.
*/
public MetricAlertPropertiesPatch withTargetResourceRegion(String targetResourceRegion) {
this.targetResourceRegion = targetResourceRegion;
return this;
}
/**
* Get the criteria property: defines the specific alert criteria information.
*
* @return the criteria value.
*/
public MetricAlertCriteria criteria() {
return this.criteria;
}
/**
* Set the criteria property: defines the specific alert criteria information.
*
* @param criteria the criteria value to set.
* @return the MetricAlertPropertiesPatch object itself.
*/
public MetricAlertPropertiesPatch withCriteria(MetricAlertCriteria criteria) {
this.criteria = criteria;
return this;
}
/**
* Get the autoMitigate property: the flag that indicates whether the alert should be auto resolved or not. The
* default is true.
*
* @return the autoMitigate value.
*/
public Boolean autoMitigate() {
return this.autoMitigate;
}
/**
* Set the autoMitigate property: the flag that indicates whether the alert should be auto resolved or not. The
* default is true.
*
* @param autoMitigate the autoMitigate value to set.
* @return the MetricAlertPropertiesPatch object itself.
*/
public MetricAlertPropertiesPatch withAutoMitigate(Boolean autoMitigate) {
this.autoMitigate = autoMitigate;
return this;
}
/**
* Get the actions property: the array of actions that are performed when the alert rule becomes active, and when an
* alert condition is resolved.
*
* @return the actions value.
*/
public List actions() {
return this.actions;
}
/**
* Set the actions property: the array of actions that are performed when the alert rule becomes active, and when an
* alert condition is resolved.
*
* @param actions the actions value to set.
* @return the MetricAlertPropertiesPatch object itself.
*/
public MetricAlertPropertiesPatch withActions(List actions) {
this.actions = actions;
return this;
}
/**
* Get the lastUpdatedTime property: Last time the rule was updated in ISO8601 format.
*
* @return the lastUpdatedTime value.
*/
public OffsetDateTime lastUpdatedTime() {
return this.lastUpdatedTime;
}
/**
* Get the isMigrated property: the value indicating whether this alert rule is migrated.
*
* @return the isMigrated value.
*/
public Boolean isMigrated() {
return this.isMigrated;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (criteria() != null) {
criteria().validate();
}
if (actions() != null) {
actions().forEach(e -> e.validate());
}
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("description", this.description);
jsonWriter.writeNumberField("severity", this.severity);
jsonWriter.writeBooleanField("enabled", this.enabled);
jsonWriter.writeArrayField("scopes", this.scopes, (writer, element) -> writer.writeString(element));
jsonWriter.writeStringField("evaluationFrequency",
CoreUtils.durationToStringWithDays(this.evaluationFrequency));
jsonWriter.writeStringField("windowSize", CoreUtils.durationToStringWithDays(this.windowSize));
jsonWriter.writeStringField("targetResourceType", this.targetResourceType);
jsonWriter.writeStringField("targetResourceRegion", this.targetResourceRegion);
jsonWriter.writeJsonField("criteria", this.criteria);
jsonWriter.writeBooleanField("autoMitigate", this.autoMitigate);
jsonWriter.writeArrayField("actions", this.actions, (writer, element) -> writer.writeJson(element));
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of MetricAlertPropertiesPatch from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of MetricAlertPropertiesPatch if the JsonReader was pointing to an instance of it, or null if
* it was pointing to JSON null.
* @throws IOException If an error occurs while reading the MetricAlertPropertiesPatch.
*/
public static MetricAlertPropertiesPatch fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
MetricAlertPropertiesPatch deserializedMetricAlertPropertiesPatch = new MetricAlertPropertiesPatch();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("description".equals(fieldName)) {
deserializedMetricAlertPropertiesPatch.description = reader.getString();
} else if ("severity".equals(fieldName)) {
deserializedMetricAlertPropertiesPatch.severity = reader.getNullable(JsonReader::getInt);
} else if ("enabled".equals(fieldName)) {
deserializedMetricAlertPropertiesPatch.enabled = reader.getNullable(JsonReader::getBoolean);
} else if ("scopes".equals(fieldName)) {
List scopes = reader.readArray(reader1 -> reader1.getString());
deserializedMetricAlertPropertiesPatch.scopes = scopes;
} else if ("evaluationFrequency".equals(fieldName)) {
deserializedMetricAlertPropertiesPatch.evaluationFrequency
= reader.getNullable(nonNullReader -> Duration.parse(nonNullReader.getString()));
} else if ("windowSize".equals(fieldName)) {
deserializedMetricAlertPropertiesPatch.windowSize
= reader.getNullable(nonNullReader -> Duration.parse(nonNullReader.getString()));
} else if ("targetResourceType".equals(fieldName)) {
deserializedMetricAlertPropertiesPatch.targetResourceType = reader.getString();
} else if ("targetResourceRegion".equals(fieldName)) {
deserializedMetricAlertPropertiesPatch.targetResourceRegion = reader.getString();
} else if ("criteria".equals(fieldName)) {
deserializedMetricAlertPropertiesPatch.criteria = MetricAlertCriteria.fromJson(reader);
} else if ("autoMitigate".equals(fieldName)) {
deserializedMetricAlertPropertiesPatch.autoMitigate = reader.getNullable(JsonReader::getBoolean);
} else if ("actions".equals(fieldName)) {
List actions = reader.readArray(reader1 -> MetricAlertAction.fromJson(reader1));
deserializedMetricAlertPropertiesPatch.actions = actions;
} else if ("lastUpdatedTime".equals(fieldName)) {
deserializedMetricAlertPropertiesPatch.lastUpdatedTime = reader
.getNullable(nonNullReader -> CoreUtils.parseBestOffsetDateTime(nonNullReader.getString()));
} else if ("isMigrated".equals(fieldName)) {
deserializedMetricAlertPropertiesPatch.isMigrated = reader.getNullable(JsonReader::getBoolean);
} else {
reader.skipChildren();
}
}
return deserializedMetricAlertPropertiesPatch;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy