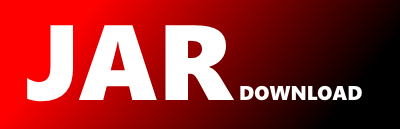
com.azure.resourcemanager.monitor.fluent.models.ScheduledQueryRuleResourceInner Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-monitor Show documentation
Show all versions of azure-resourcemanager-monitor Show documentation
This package contains Microsoft Azure Monitor SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.monitor.fluent.models;
import com.azure.core.annotation.Fluent;
import com.azure.core.management.Resource;
import com.azure.core.management.SystemData;
import com.azure.core.util.logging.ClientLogger;
import com.azure.json.JsonReader;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import com.azure.resourcemanager.monitor.models.Actions;
import com.azure.resourcemanager.monitor.models.AlertSeverity;
import com.azure.resourcemanager.monitor.models.Kind;
import com.azure.resourcemanager.monitor.models.ScheduledQueryRuleCriteria;
import java.io.IOException;
import java.time.Duration;
import java.util.List;
import java.util.Map;
/**
* The scheduled query rule resource.
*/
@Fluent
public final class ScheduledQueryRuleResourceInner extends Resource {
/*
* Indicates the type of scheduled query rule. The default is LogAlert.
*/
private Kind kind;
/*
* The etag field is *not* required. If it is provided in the response body, it must also be provided as a header
* per the normal etag convention. Entity tags are used for comparing two or more entities from the same requested
* resource. HTTP/1.1 uses entity tags in the etag (section 14.19), If-Match (section 14.24), If-None-Match (section
* 14.26), and If-Range (section 14.27) header fields.
*/
private String etag;
/*
* SystemData of ScheduledQueryRule.
*/
private SystemData systemData;
/*
* The rule properties of the resource.
*/
private ScheduledQueryRuleProperties innerProperties = new ScheduledQueryRuleProperties();
/*
* Fully qualified resource Id for the resource.
*/
private String id;
/*
* The name of the resource.
*/
private String name;
/*
* The type of the resource.
*/
private String type;
/**
* Creates an instance of ScheduledQueryRuleResourceInner class.
*/
public ScheduledQueryRuleResourceInner() {
}
/**
* Get the kind property: Indicates the type of scheduled query rule. The default is LogAlert.
*
* @return the kind value.
*/
public Kind kind() {
return this.kind;
}
/**
* Set the kind property: Indicates the type of scheduled query rule. The default is LogAlert.
*
* @param kind the kind value to set.
* @return the ScheduledQueryRuleResourceInner object itself.
*/
public ScheduledQueryRuleResourceInner withKind(Kind kind) {
this.kind = kind;
return this;
}
/**
* Get the etag property: The etag field is *not* required. If it is provided in the response body, it must also be
* provided as a header per the normal etag convention. Entity tags are used for comparing two or more entities from
* the same requested resource. HTTP/1.1 uses entity tags in the etag (section 14.19), If-Match (section 14.24),
* If-None-Match (section 14.26), and If-Range (section 14.27) header fields.
*
* @return the etag value.
*/
public String etag() {
return this.etag;
}
/**
* Get the systemData property: SystemData of ScheduledQueryRule.
*
* @return the systemData value.
*/
public SystemData systemData() {
return this.systemData;
}
/**
* Get the innerProperties property: The rule properties of the resource.
*
* @return the innerProperties value.
*/
private ScheduledQueryRuleProperties innerProperties() {
return this.innerProperties;
}
/**
* Get the id property: Fully qualified resource Id for the resource.
*
* @return the id value.
*/
@Override
public String id() {
return this.id;
}
/**
* Get the name property: The name of the resource.
*
* @return the name value.
*/
@Override
public String name() {
return this.name;
}
/**
* Get the type property: The type of the resource.
*
* @return the type value.
*/
@Override
public String type() {
return this.type;
}
/**
* {@inheritDoc}
*/
@Override
public ScheduledQueryRuleResourceInner withLocation(String location) {
super.withLocation(location);
return this;
}
/**
* {@inheritDoc}
*/
@Override
public ScheduledQueryRuleResourceInner withTags(Map tags) {
super.withTags(tags);
return this;
}
/**
* Get the createdWithApiVersion property: The api-version used when creating this alert rule.
*
* @return the createdWithApiVersion value.
*/
public String createdWithApiVersion() {
return this.innerProperties() == null ? null : this.innerProperties().createdWithApiVersion();
}
/**
* Get the isLegacyLogAnalyticsRule property: True if alert rule is legacy Log Analytic rule.
*
* @return the isLegacyLogAnalyticsRule value.
*/
public Boolean isLegacyLogAnalyticsRule() {
return this.innerProperties() == null ? null : this.innerProperties().isLegacyLogAnalyticsRule();
}
/**
* Get the description property: The description of the scheduled query rule.
*
* @return the description value.
*/
public String description() {
return this.innerProperties() == null ? null : this.innerProperties().description();
}
/**
* Set the description property: The description of the scheduled query rule.
*
* @param description the description value to set.
* @return the ScheduledQueryRuleResourceInner object itself.
*/
public ScheduledQueryRuleResourceInner withDescription(String description) {
if (this.innerProperties() == null) {
this.innerProperties = new ScheduledQueryRuleProperties();
}
this.innerProperties().withDescription(description);
return this;
}
/**
* Get the displayName property: The display name of the alert rule.
*
* @return the displayName value.
*/
public String displayName() {
return this.innerProperties() == null ? null : this.innerProperties().displayName();
}
/**
* Set the displayName property: The display name of the alert rule.
*
* @param displayName the displayName value to set.
* @return the ScheduledQueryRuleResourceInner object itself.
*/
public ScheduledQueryRuleResourceInner withDisplayName(String displayName) {
if (this.innerProperties() == null) {
this.innerProperties = new ScheduledQueryRuleProperties();
}
this.innerProperties().withDisplayName(displayName);
return this;
}
/**
* Get the severity property: Severity of the alert. Should be an integer between [0-4]. Value of 0 is severest.
* Relevant and required only for rules of the kind LogAlert.
*
* @return the severity value.
*/
public AlertSeverity severity() {
return this.innerProperties() == null ? null : this.innerProperties().severity();
}
/**
* Set the severity property: Severity of the alert. Should be an integer between [0-4]. Value of 0 is severest.
* Relevant and required only for rules of the kind LogAlert.
*
* @param severity the severity value to set.
* @return the ScheduledQueryRuleResourceInner object itself.
*/
public ScheduledQueryRuleResourceInner withSeverity(AlertSeverity severity) {
if (this.innerProperties() == null) {
this.innerProperties = new ScheduledQueryRuleProperties();
}
this.innerProperties().withSeverity(severity);
return this;
}
/**
* Get the enabled property: The flag which indicates whether this scheduled query rule is enabled. Value should be
* true or false.
*
* @return the enabled value.
*/
public Boolean enabled() {
return this.innerProperties() == null ? null : this.innerProperties().enabled();
}
/**
* Set the enabled property: The flag which indicates whether this scheduled query rule is enabled. Value should be
* true or false.
*
* @param enabled the enabled value to set.
* @return the ScheduledQueryRuleResourceInner object itself.
*/
public ScheduledQueryRuleResourceInner withEnabled(Boolean enabled) {
if (this.innerProperties() == null) {
this.innerProperties = new ScheduledQueryRuleProperties();
}
this.innerProperties().withEnabled(enabled);
return this;
}
/**
* Get the scopes property: The list of resource id's that this scheduled query rule is scoped to.
*
* @return the scopes value.
*/
public List scopes() {
return this.innerProperties() == null ? null : this.innerProperties().scopes();
}
/**
* Set the scopes property: The list of resource id's that this scheduled query rule is scoped to.
*
* @param scopes the scopes value to set.
* @return the ScheduledQueryRuleResourceInner object itself.
*/
public ScheduledQueryRuleResourceInner withScopes(List scopes) {
if (this.innerProperties() == null) {
this.innerProperties = new ScheduledQueryRuleProperties();
}
this.innerProperties().withScopes(scopes);
return this;
}
/**
* Get the evaluationFrequency property: How often the scheduled query rule is evaluated represented in ISO 8601
* duration format. Relevant and required only for rules of the kind LogAlert.
*
* @return the evaluationFrequency value.
*/
public Duration evaluationFrequency() {
return this.innerProperties() == null ? null : this.innerProperties().evaluationFrequency();
}
/**
* Set the evaluationFrequency property: How often the scheduled query rule is evaluated represented in ISO 8601
* duration format. Relevant and required only for rules of the kind LogAlert.
*
* @param evaluationFrequency the evaluationFrequency value to set.
* @return the ScheduledQueryRuleResourceInner object itself.
*/
public ScheduledQueryRuleResourceInner withEvaluationFrequency(Duration evaluationFrequency) {
if (this.innerProperties() == null) {
this.innerProperties = new ScheduledQueryRuleProperties();
}
this.innerProperties().withEvaluationFrequency(evaluationFrequency);
return this;
}
/**
* Get the windowSize property: The period of time (in ISO 8601 duration format) on which the Alert query will be
* executed (bin size). Relevant and required only for rules of the kind LogAlert.
*
* @return the windowSize value.
*/
public Duration windowSize() {
return this.innerProperties() == null ? null : this.innerProperties().windowSize();
}
/**
* Set the windowSize property: The period of time (in ISO 8601 duration format) on which the Alert query will be
* executed (bin size). Relevant and required only for rules of the kind LogAlert.
*
* @param windowSize the windowSize value to set.
* @return the ScheduledQueryRuleResourceInner object itself.
*/
public ScheduledQueryRuleResourceInner withWindowSize(Duration windowSize) {
if (this.innerProperties() == null) {
this.innerProperties = new ScheduledQueryRuleProperties();
}
this.innerProperties().withWindowSize(windowSize);
return this;
}
/**
* Get the overrideQueryTimeRange property: If specified then overrides the query time range (default is
* WindowSize*NumberOfEvaluationPeriods). Relevant only for rules of the kind LogAlert.
*
* @return the overrideQueryTimeRange value.
*/
public Duration overrideQueryTimeRange() {
return this.innerProperties() == null ? null : this.innerProperties().overrideQueryTimeRange();
}
/**
* Set the overrideQueryTimeRange property: If specified then overrides the query time range (default is
* WindowSize*NumberOfEvaluationPeriods). Relevant only for rules of the kind LogAlert.
*
* @param overrideQueryTimeRange the overrideQueryTimeRange value to set.
* @return the ScheduledQueryRuleResourceInner object itself.
*/
public ScheduledQueryRuleResourceInner withOverrideQueryTimeRange(Duration overrideQueryTimeRange) {
if (this.innerProperties() == null) {
this.innerProperties = new ScheduledQueryRuleProperties();
}
this.innerProperties().withOverrideQueryTimeRange(overrideQueryTimeRange);
return this;
}
/**
* Get the targetResourceTypes property: List of resource type of the target resource(s) on which the alert is
* created/updated. For example if the scope is a resource group and targetResourceTypes is
* Microsoft.Compute/virtualMachines, then a different alert will be fired for each virtual machine in the resource
* group which meet the alert criteria. Relevant only for rules of the kind LogAlert.
*
* @return the targetResourceTypes value.
*/
public List targetResourceTypes() {
return this.innerProperties() == null ? null : this.innerProperties().targetResourceTypes();
}
/**
* Set the targetResourceTypes property: List of resource type of the target resource(s) on which the alert is
* created/updated. For example if the scope is a resource group and targetResourceTypes is
* Microsoft.Compute/virtualMachines, then a different alert will be fired for each virtual machine in the resource
* group which meet the alert criteria. Relevant only for rules of the kind LogAlert.
*
* @param targetResourceTypes the targetResourceTypes value to set.
* @return the ScheduledQueryRuleResourceInner object itself.
*/
public ScheduledQueryRuleResourceInner withTargetResourceTypes(List targetResourceTypes) {
if (this.innerProperties() == null) {
this.innerProperties = new ScheduledQueryRuleProperties();
}
this.innerProperties().withTargetResourceTypes(targetResourceTypes);
return this;
}
/**
* Get the criteria property: The rule criteria that defines the conditions of the scheduled query rule.
*
* @return the criteria value.
*/
public ScheduledQueryRuleCriteria criteria() {
return this.innerProperties() == null ? null : this.innerProperties().criteria();
}
/**
* Set the criteria property: The rule criteria that defines the conditions of the scheduled query rule.
*
* @param criteria the criteria value to set.
* @return the ScheduledQueryRuleResourceInner object itself.
*/
public ScheduledQueryRuleResourceInner withCriteria(ScheduledQueryRuleCriteria criteria) {
if (this.innerProperties() == null) {
this.innerProperties = new ScheduledQueryRuleProperties();
}
this.innerProperties().withCriteria(criteria);
return this;
}
/**
* Get the muteActionsDuration property: Mute actions for the chosen period of time (in ISO 8601 duration format)
* after the alert is fired. Relevant only for rules of the kind LogAlert.
*
* @return the muteActionsDuration value.
*/
public Duration muteActionsDuration() {
return this.innerProperties() == null ? null : this.innerProperties().muteActionsDuration();
}
/**
* Set the muteActionsDuration property: Mute actions for the chosen period of time (in ISO 8601 duration format)
* after the alert is fired. Relevant only for rules of the kind LogAlert.
*
* @param muteActionsDuration the muteActionsDuration value to set.
* @return the ScheduledQueryRuleResourceInner object itself.
*/
public ScheduledQueryRuleResourceInner withMuteActionsDuration(Duration muteActionsDuration) {
if (this.innerProperties() == null) {
this.innerProperties = new ScheduledQueryRuleProperties();
}
this.innerProperties().withMuteActionsDuration(muteActionsDuration);
return this;
}
/**
* Get the actions property: Actions to invoke when the alert fires.
*
* @return the actions value.
*/
public Actions actions() {
return this.innerProperties() == null ? null : this.innerProperties().actions();
}
/**
* Set the actions property: Actions to invoke when the alert fires.
*
* @param actions the actions value to set.
* @return the ScheduledQueryRuleResourceInner object itself.
*/
public ScheduledQueryRuleResourceInner withActions(Actions actions) {
if (this.innerProperties() == null) {
this.innerProperties = new ScheduledQueryRuleProperties();
}
this.innerProperties().withActions(actions);
return this;
}
/**
* Get the isWorkspaceAlertsStorageConfigured property: The flag which indicates whether this scheduled query rule
* has been configured to be stored in the customer's storage. The default is false.
*
* @return the isWorkspaceAlertsStorageConfigured value.
*/
public Boolean isWorkspaceAlertsStorageConfigured() {
return this.innerProperties() == null ? null : this.innerProperties().isWorkspaceAlertsStorageConfigured();
}
/**
* Get the checkWorkspaceAlertsStorageConfigured property: The flag which indicates whether this scheduled query
* rule should be stored in the customer's storage. The default is false. Relevant only for rules of the kind
* LogAlert.
*
* @return the checkWorkspaceAlertsStorageConfigured value.
*/
public Boolean checkWorkspaceAlertsStorageConfigured() {
return this.innerProperties() == null ? null : this.innerProperties().checkWorkspaceAlertsStorageConfigured();
}
/**
* Set the checkWorkspaceAlertsStorageConfigured property: The flag which indicates whether this scheduled query
* rule should be stored in the customer's storage. The default is false. Relevant only for rules of the kind
* LogAlert.
*
* @param checkWorkspaceAlertsStorageConfigured the checkWorkspaceAlertsStorageConfigured value to set.
* @return the ScheduledQueryRuleResourceInner object itself.
*/
public ScheduledQueryRuleResourceInner
withCheckWorkspaceAlertsStorageConfigured(Boolean checkWorkspaceAlertsStorageConfigured) {
if (this.innerProperties() == null) {
this.innerProperties = new ScheduledQueryRuleProperties();
}
this.innerProperties().withCheckWorkspaceAlertsStorageConfigured(checkWorkspaceAlertsStorageConfigured);
return this;
}
/**
* Get the skipQueryValidation property: The flag which indicates whether the provided query should be validated or
* not. The default is false. Relevant only for rules of the kind LogAlert.
*
* @return the skipQueryValidation value.
*/
public Boolean skipQueryValidation() {
return this.innerProperties() == null ? null : this.innerProperties().skipQueryValidation();
}
/**
* Set the skipQueryValidation property: The flag which indicates whether the provided query should be validated or
* not. The default is false. Relevant only for rules of the kind LogAlert.
*
* @param skipQueryValidation the skipQueryValidation value to set.
* @return the ScheduledQueryRuleResourceInner object itself.
*/
public ScheduledQueryRuleResourceInner withSkipQueryValidation(Boolean skipQueryValidation) {
if (this.innerProperties() == null) {
this.innerProperties = new ScheduledQueryRuleProperties();
}
this.innerProperties().withSkipQueryValidation(skipQueryValidation);
return this;
}
/**
* Get the autoMitigate property: The flag that indicates whether the alert should be automatically resolved or not.
* The default is true. Relevant only for rules of the kind LogAlert.
*
* @return the autoMitigate value.
*/
public Boolean autoMitigate() {
return this.innerProperties() == null ? null : this.innerProperties().autoMitigate();
}
/**
* Set the autoMitigate property: The flag that indicates whether the alert should be automatically resolved or not.
* The default is true. Relevant only for rules of the kind LogAlert.
*
* @param autoMitigate the autoMitigate value to set.
* @return the ScheduledQueryRuleResourceInner object itself.
*/
public ScheduledQueryRuleResourceInner withAutoMitigate(Boolean autoMitigate) {
if (this.innerProperties() == null) {
this.innerProperties = new ScheduledQueryRuleProperties();
}
this.innerProperties().withAutoMitigate(autoMitigate);
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (innerProperties() == null) {
throw LOGGER.atError()
.log(new IllegalArgumentException(
"Missing required property innerProperties in model ScheduledQueryRuleResourceInner"));
} else {
innerProperties().validate();
}
}
private static final ClientLogger LOGGER = new ClientLogger(ScheduledQueryRuleResourceInner.class);
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("location", location());
jsonWriter.writeMapField("tags", tags(), (writer, element) -> writer.writeString(element));
jsonWriter.writeJsonField("properties", this.innerProperties);
jsonWriter.writeStringField("kind", this.kind == null ? null : this.kind.toString());
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of ScheduledQueryRuleResourceInner from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of ScheduledQueryRuleResourceInner if the JsonReader was pointing to an instance of it, or
* null if it was pointing to JSON null.
* @throws IllegalStateException If the deserialized JSON object was missing any required properties.
* @throws IOException If an error occurs while reading the ScheduledQueryRuleResourceInner.
*/
public static ScheduledQueryRuleResourceInner fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
ScheduledQueryRuleResourceInner deserializedScheduledQueryRuleResourceInner
= new ScheduledQueryRuleResourceInner();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("id".equals(fieldName)) {
deserializedScheduledQueryRuleResourceInner.id = reader.getString();
} else if ("name".equals(fieldName)) {
deserializedScheduledQueryRuleResourceInner.name = reader.getString();
} else if ("type".equals(fieldName)) {
deserializedScheduledQueryRuleResourceInner.type = reader.getString();
} else if ("location".equals(fieldName)) {
deserializedScheduledQueryRuleResourceInner.withLocation(reader.getString());
} else if ("tags".equals(fieldName)) {
Map tags = reader.readMap(reader1 -> reader1.getString());
deserializedScheduledQueryRuleResourceInner.withTags(tags);
} else if ("properties".equals(fieldName)) {
deserializedScheduledQueryRuleResourceInner.innerProperties
= ScheduledQueryRuleProperties.fromJson(reader);
} else if ("kind".equals(fieldName)) {
deserializedScheduledQueryRuleResourceInner.kind = Kind.fromString(reader.getString());
} else if ("etag".equals(fieldName)) {
deserializedScheduledQueryRuleResourceInner.etag = reader.getString();
} else if ("systemData".equals(fieldName)) {
deserializedScheduledQueryRuleResourceInner.systemData = SystemData.fromJson(reader);
} else {
reader.skipChildren();
}
}
return deserializedScheduledQueryRuleResourceInner;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy