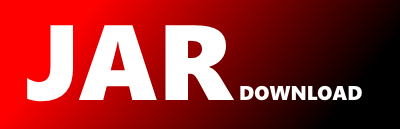
com.azure.resourcemanager.monitor.fluent.models.VMInsightsOnboardingStatusProperties Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-monitor Show documentation
Show all versions of azure-resourcemanager-monitor Show documentation
This package contains Microsoft Azure Monitor SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.monitor.fluent.models;
import com.azure.core.annotation.Fluent;
import com.azure.core.util.logging.ClientLogger;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import com.azure.resourcemanager.monitor.models.DataContainer;
import com.azure.resourcemanager.monitor.models.DataStatus;
import com.azure.resourcemanager.monitor.models.OnboardingStatus;
import java.io.IOException;
import java.util.List;
/**
* Resource properties.
*/
@Fluent
public final class VMInsightsOnboardingStatusProperties
implements JsonSerializable {
/*
* Azure Resource Manager identifier of the resource whose onboarding status is being represented.
*/
private String resourceId;
/*
* The onboarding status for the resource. Note that, a higher level scope, e.g., resource group or subscription, is
* considered onboarded if at least one resource under it is onboarded.
*/
private OnboardingStatus onboardingStatus;
/*
* The status of VM Insights data from the resource. When reported as `present` the data array will contain
* information about the data containers to which data for the specified resource is being routed.
*/
private DataStatus dataStatus;
/*
* Containers that currently store VM Insights data for the specified resource.
*/
private List data;
/**
* Creates an instance of VMInsightsOnboardingStatusProperties class.
*/
public VMInsightsOnboardingStatusProperties() {
}
/**
* Get the resourceId property: Azure Resource Manager identifier of the resource whose onboarding status is being
* represented.
*
* @return the resourceId value.
*/
public String resourceId() {
return this.resourceId;
}
/**
* Set the resourceId property: Azure Resource Manager identifier of the resource whose onboarding status is being
* represented.
*
* @param resourceId the resourceId value to set.
* @return the VMInsightsOnboardingStatusProperties object itself.
*/
public VMInsightsOnboardingStatusProperties withResourceId(String resourceId) {
this.resourceId = resourceId;
return this;
}
/**
* Get the onboardingStatus property: The onboarding status for the resource. Note that, a higher level scope, e.g.,
* resource group or subscription, is considered onboarded if at least one resource under it is onboarded.
*
* @return the onboardingStatus value.
*/
public OnboardingStatus onboardingStatus() {
return this.onboardingStatus;
}
/**
* Set the onboardingStatus property: The onboarding status for the resource. Note that, a higher level scope, e.g.,
* resource group or subscription, is considered onboarded if at least one resource under it is onboarded.
*
* @param onboardingStatus the onboardingStatus value to set.
* @return the VMInsightsOnboardingStatusProperties object itself.
*/
public VMInsightsOnboardingStatusProperties withOnboardingStatus(OnboardingStatus onboardingStatus) {
this.onboardingStatus = onboardingStatus;
return this;
}
/**
* Get the dataStatus property: The status of VM Insights data from the resource. When reported as `present` the
* data array will contain information about the data containers to which data for the specified resource is being
* routed.
*
* @return the dataStatus value.
*/
public DataStatus dataStatus() {
return this.dataStatus;
}
/**
* Set the dataStatus property: The status of VM Insights data from the resource. When reported as `present` the
* data array will contain information about the data containers to which data for the specified resource is being
* routed.
*
* @param dataStatus the dataStatus value to set.
* @return the VMInsightsOnboardingStatusProperties object itself.
*/
public VMInsightsOnboardingStatusProperties withDataStatus(DataStatus dataStatus) {
this.dataStatus = dataStatus;
return this;
}
/**
* Get the data property: Containers that currently store VM Insights data for the specified resource.
*
* @return the data value.
*/
public List data() {
return this.data;
}
/**
* Set the data property: Containers that currently store VM Insights data for the specified resource.
*
* @param data the data value to set.
* @return the VMInsightsOnboardingStatusProperties object itself.
*/
public VMInsightsOnboardingStatusProperties withData(List data) {
this.data = data;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (resourceId() == null) {
throw LOGGER.atError()
.log(new IllegalArgumentException(
"Missing required property resourceId in model VMInsightsOnboardingStatusProperties"));
}
if (onboardingStatus() == null) {
throw LOGGER.atError()
.log(new IllegalArgumentException(
"Missing required property onboardingStatus in model VMInsightsOnboardingStatusProperties"));
}
if (dataStatus() == null) {
throw LOGGER.atError()
.log(new IllegalArgumentException(
"Missing required property dataStatus in model VMInsightsOnboardingStatusProperties"));
}
if (data() != null) {
data().forEach(e -> e.validate());
}
}
private static final ClientLogger LOGGER = new ClientLogger(VMInsightsOnboardingStatusProperties.class);
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("resourceId", this.resourceId);
jsonWriter.writeStringField("onboardingStatus",
this.onboardingStatus == null ? null : this.onboardingStatus.toString());
jsonWriter.writeStringField("dataStatus", this.dataStatus == null ? null : this.dataStatus.toString());
jsonWriter.writeArrayField("data", this.data, (writer, element) -> writer.writeJson(element));
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of VMInsightsOnboardingStatusProperties from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of VMInsightsOnboardingStatusProperties if the JsonReader was pointing to an instance of it,
* or null if it was pointing to JSON null.
* @throws IllegalStateException If the deserialized JSON object was missing any required properties.
* @throws IOException If an error occurs while reading the VMInsightsOnboardingStatusProperties.
*/
public static VMInsightsOnboardingStatusProperties fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
VMInsightsOnboardingStatusProperties deserializedVMInsightsOnboardingStatusProperties
= new VMInsightsOnboardingStatusProperties();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("resourceId".equals(fieldName)) {
deserializedVMInsightsOnboardingStatusProperties.resourceId = reader.getString();
} else if ("onboardingStatus".equals(fieldName)) {
deserializedVMInsightsOnboardingStatusProperties.onboardingStatus
= OnboardingStatus.fromString(reader.getString());
} else if ("dataStatus".equals(fieldName)) {
deserializedVMInsightsOnboardingStatusProperties.dataStatus
= DataStatus.fromString(reader.getString());
} else if ("data".equals(fieldName)) {
List data = reader.readArray(reader1 -> DataContainer.fromJson(reader1));
deserializedVMInsightsOnboardingStatusProperties.data = data;
} else {
reader.skipChildren();
}
}
return deserializedVMInsightsOnboardingStatusProperties;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy