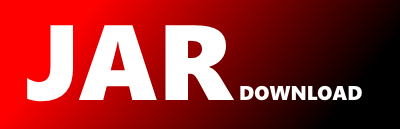
com.azure.resourcemanager.monitor.models.DataCollectionRule Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-monitor Show documentation
Show all versions of azure-resourcemanager-monitor Show documentation
This package contains Microsoft Azure Monitor SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.monitor.models;
import com.azure.core.annotation.Fluent;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
import java.util.List;
import java.util.Map;
/**
* Definition of what monitoring data to collect and where that data should be sent.
*/
@Fluent
public class DataCollectionRule implements JsonSerializable {
/*
* Description of the data collection rule.
*/
private String description;
/*
* The immutable ID of this data collection rule. This property is READ-ONLY.
*/
private String immutableId;
/*
* The resource ID of the data collection endpoint that this rule can be used with.
*/
private String dataCollectionEndpointId;
/*
* Metadata about the resource
*/
private DataCollectionRuleMetadata metadata;
/*
* Declaration of custom streams used in this rule.
*/
private Map streamDeclarations;
/*
* The specification of data sources.
* This property is optional and can be omitted if the rule is meant to be used via direct calls to the provisioned
* endpoint.
*/
private DataCollectionRuleDataSources dataSources;
/*
* The specification of destinations.
*/
private DataCollectionRuleDestinations destinations;
/*
* The specification of data flows.
*/
private List dataFlows;
/*
* The resource provisioning state.
*/
private KnownDataCollectionRuleProvisioningState provisioningState;
/**
* Creates an instance of DataCollectionRule class.
*/
public DataCollectionRule() {
}
/**
* Get the description property: Description of the data collection rule.
*
* @return the description value.
*/
public String description() {
return this.description;
}
/**
* Set the description property: Description of the data collection rule.
*
* @param description the description value to set.
* @return the DataCollectionRule object itself.
*/
public DataCollectionRule withDescription(String description) {
this.description = description;
return this;
}
/**
* Get the immutableId property: The immutable ID of this data collection rule. This property is READ-ONLY.
*
* @return the immutableId value.
*/
public String immutableId() {
return this.immutableId;
}
/**
* Set the immutableId property: The immutable ID of this data collection rule. This property is READ-ONLY.
*
* @param immutableId the immutableId value to set.
* @return the DataCollectionRule object itself.
*/
DataCollectionRule withImmutableId(String immutableId) {
this.immutableId = immutableId;
return this;
}
/**
* Get the dataCollectionEndpointId property: The resource ID of the data collection endpoint that this rule can be
* used with.
*
* @return the dataCollectionEndpointId value.
*/
public String dataCollectionEndpointId() {
return this.dataCollectionEndpointId;
}
/**
* Set the dataCollectionEndpointId property: The resource ID of the data collection endpoint that this rule can be
* used with.
*
* @param dataCollectionEndpointId the dataCollectionEndpointId value to set.
* @return the DataCollectionRule object itself.
*/
public DataCollectionRule withDataCollectionEndpointId(String dataCollectionEndpointId) {
this.dataCollectionEndpointId = dataCollectionEndpointId;
return this;
}
/**
* Get the metadata property: Metadata about the resource.
*
* @return the metadata value.
*/
public DataCollectionRuleMetadata metadata() {
return this.metadata;
}
/**
* Set the metadata property: Metadata about the resource.
*
* @param metadata the metadata value to set.
* @return the DataCollectionRule object itself.
*/
DataCollectionRule withMetadata(DataCollectionRuleMetadata metadata) {
this.metadata = metadata;
return this;
}
/**
* Get the streamDeclarations property: Declaration of custom streams used in this rule.
*
* @return the streamDeclarations value.
*/
public Map streamDeclarations() {
return this.streamDeclarations;
}
/**
* Set the streamDeclarations property: Declaration of custom streams used in this rule.
*
* @param streamDeclarations the streamDeclarations value to set.
* @return the DataCollectionRule object itself.
*/
public DataCollectionRule withStreamDeclarations(Map streamDeclarations) {
this.streamDeclarations = streamDeclarations;
return this;
}
/**
* Get the dataSources property: The specification of data sources.
* This property is optional and can be omitted if the rule is meant to be used via direct calls to the provisioned
* endpoint.
*
* @return the dataSources value.
*/
public DataCollectionRuleDataSources dataSources() {
return this.dataSources;
}
/**
* Set the dataSources property: The specification of data sources.
* This property is optional and can be omitted if the rule is meant to be used via direct calls to the provisioned
* endpoint.
*
* @param dataSources the dataSources value to set.
* @return the DataCollectionRule object itself.
*/
public DataCollectionRule withDataSources(DataCollectionRuleDataSources dataSources) {
this.dataSources = dataSources;
return this;
}
/**
* Get the destinations property: The specification of destinations.
*
* @return the destinations value.
*/
public DataCollectionRuleDestinations destinations() {
return this.destinations;
}
/**
* Set the destinations property: The specification of destinations.
*
* @param destinations the destinations value to set.
* @return the DataCollectionRule object itself.
*/
public DataCollectionRule withDestinations(DataCollectionRuleDestinations destinations) {
this.destinations = destinations;
return this;
}
/**
* Get the dataFlows property: The specification of data flows.
*
* @return the dataFlows value.
*/
public List dataFlows() {
return this.dataFlows;
}
/**
* Set the dataFlows property: The specification of data flows.
*
* @param dataFlows the dataFlows value to set.
* @return the DataCollectionRule object itself.
*/
public DataCollectionRule withDataFlows(List dataFlows) {
this.dataFlows = dataFlows;
return this;
}
/**
* Get the provisioningState property: The resource provisioning state.
*
* @return the provisioningState value.
*/
public KnownDataCollectionRuleProvisioningState provisioningState() {
return this.provisioningState;
}
/**
* Set the provisioningState property: The resource provisioning state.
*
* @param provisioningState the provisioningState value to set.
* @return the DataCollectionRule object itself.
*/
DataCollectionRule withProvisioningState(KnownDataCollectionRuleProvisioningState provisioningState) {
this.provisioningState = provisioningState;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (metadata() != null) {
metadata().validate();
}
if (streamDeclarations() != null) {
streamDeclarations().values().forEach(e -> {
if (e != null) {
e.validate();
}
});
}
if (dataSources() != null) {
dataSources().validate();
}
if (destinations() != null) {
destinations().validate();
}
if (dataFlows() != null) {
dataFlows().forEach(e -> e.validate());
}
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("description", this.description);
jsonWriter.writeStringField("dataCollectionEndpointId", this.dataCollectionEndpointId);
jsonWriter.writeMapField("streamDeclarations", this.streamDeclarations,
(writer, element) -> writer.writeJson(element));
jsonWriter.writeJsonField("dataSources", this.dataSources);
jsonWriter.writeJsonField("destinations", this.destinations);
jsonWriter.writeArrayField("dataFlows", this.dataFlows, (writer, element) -> writer.writeJson(element));
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of DataCollectionRule from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of DataCollectionRule if the JsonReader was pointing to an instance of it, or null if it was
* pointing to JSON null.
* @throws IOException If an error occurs while reading the DataCollectionRule.
*/
public static DataCollectionRule fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
DataCollectionRule deserializedDataCollectionRule = new DataCollectionRule();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("description".equals(fieldName)) {
deserializedDataCollectionRule.description = reader.getString();
} else if ("immutableId".equals(fieldName)) {
deserializedDataCollectionRule.immutableId = reader.getString();
} else if ("dataCollectionEndpointId".equals(fieldName)) {
deserializedDataCollectionRule.dataCollectionEndpointId = reader.getString();
} else if ("metadata".equals(fieldName)) {
deserializedDataCollectionRule.metadata = DataCollectionRuleMetadata.fromJson(reader);
} else if ("streamDeclarations".equals(fieldName)) {
Map streamDeclarations
= reader.readMap(reader1 -> StreamDeclaration.fromJson(reader1));
deserializedDataCollectionRule.streamDeclarations = streamDeclarations;
} else if ("dataSources".equals(fieldName)) {
deserializedDataCollectionRule.dataSources = DataCollectionRuleDataSources.fromJson(reader);
} else if ("destinations".equals(fieldName)) {
deserializedDataCollectionRule.destinations = DataCollectionRuleDestinations.fromJson(reader);
} else if ("dataFlows".equals(fieldName)) {
List dataFlows = reader.readArray(reader1 -> DataFlow.fromJson(reader1));
deserializedDataCollectionRule.dataFlows = dataFlows;
} else if ("provisioningState".equals(fieldName)) {
deserializedDataCollectionRule.provisioningState
= KnownDataCollectionRuleProvisioningState.fromString(reader.getString());
} else {
reader.skipChildren();
}
}
return deserializedDataCollectionRule;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy