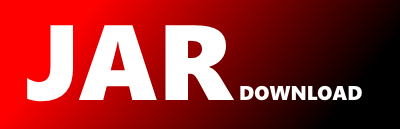
com.azure.resourcemanager.mysqlflexibleserver.fluent.models.ConfigurationProperties Maven / Gradle / Ivy
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.mysqlflexibleserver.fluent.models;
import com.azure.core.annotation.Fluent;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import com.azure.resourcemanager.mysqlflexibleserver.models.ConfigurationSource;
import com.azure.resourcemanager.mysqlflexibleserver.models.IsConfigPendingRestart;
import com.azure.resourcemanager.mysqlflexibleserver.models.IsDynamicConfig;
import com.azure.resourcemanager.mysqlflexibleserver.models.IsReadOnly;
import java.io.IOException;
/**
* The properties of a configuration.
*/
@Fluent
public final class ConfigurationProperties implements JsonSerializable {
/*
* Value of the configuration.
*/
private String value;
/*
* Description of the configuration.
*/
private String description;
/*
* Default value of the configuration.
*/
private String defaultValue;
/*
* Data type of the configuration.
*/
private String dataType;
/*
* Allowed values of the configuration.
*/
private String allowedValues;
/*
* Source of the configuration.
*/
private ConfigurationSource source;
/*
* If is the configuration read only.
*/
private IsReadOnly isReadOnly;
/*
* If is the configuration pending restart or not.
*/
private IsConfigPendingRestart isConfigPendingRestart;
/*
* If is the configuration dynamic.
*/
private IsDynamicConfig isDynamicConfig;
/**
* Creates an instance of ConfigurationProperties class.
*/
public ConfigurationProperties() {
}
/**
* Get the value property: Value of the configuration.
*
* @return the value value.
*/
public String value() {
return this.value;
}
/**
* Set the value property: Value of the configuration.
*
* @param value the value value to set.
* @return the ConfigurationProperties object itself.
*/
public ConfigurationProperties withValue(String value) {
this.value = value;
return this;
}
/**
* Get the description property: Description of the configuration.
*
* @return the description value.
*/
public String description() {
return this.description;
}
/**
* Get the defaultValue property: Default value of the configuration.
*
* @return the defaultValue value.
*/
public String defaultValue() {
return this.defaultValue;
}
/**
* Get the dataType property: Data type of the configuration.
*
* @return the dataType value.
*/
public String dataType() {
return this.dataType;
}
/**
* Get the allowedValues property: Allowed values of the configuration.
*
* @return the allowedValues value.
*/
public String allowedValues() {
return this.allowedValues;
}
/**
* Get the source property: Source of the configuration.
*
* @return the source value.
*/
public ConfigurationSource source() {
return this.source;
}
/**
* Set the source property: Source of the configuration.
*
* @param source the source value to set.
* @return the ConfigurationProperties object itself.
*/
public ConfigurationProperties withSource(ConfigurationSource source) {
this.source = source;
return this;
}
/**
* Get the isReadOnly property: If is the configuration read only.
*
* @return the isReadOnly value.
*/
public IsReadOnly isReadOnly() {
return this.isReadOnly;
}
/**
* Get the isConfigPendingRestart property: If is the configuration pending restart or not.
*
* @return the isConfigPendingRestart value.
*/
public IsConfigPendingRestart isConfigPendingRestart() {
return this.isConfigPendingRestart;
}
/**
* Get the isDynamicConfig property: If is the configuration dynamic.
*
* @return the isDynamicConfig value.
*/
public IsDynamicConfig isDynamicConfig() {
return this.isDynamicConfig;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("value", this.value);
jsonWriter.writeStringField("source", this.source == null ? null : this.source.toString());
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of ConfigurationProperties from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of ConfigurationProperties if the JsonReader was pointing to an instance of it, or null if it
* was pointing to JSON null.
* @throws IOException If an error occurs while reading the ConfigurationProperties.
*/
public static ConfigurationProperties fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
ConfigurationProperties deserializedConfigurationProperties = new ConfigurationProperties();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("value".equals(fieldName)) {
deserializedConfigurationProperties.value = reader.getString();
} else if ("description".equals(fieldName)) {
deserializedConfigurationProperties.description = reader.getString();
} else if ("defaultValue".equals(fieldName)) {
deserializedConfigurationProperties.defaultValue = reader.getString();
} else if ("dataType".equals(fieldName)) {
deserializedConfigurationProperties.dataType = reader.getString();
} else if ("allowedValues".equals(fieldName)) {
deserializedConfigurationProperties.allowedValues = reader.getString();
} else if ("source".equals(fieldName)) {
deserializedConfigurationProperties.source = ConfigurationSource.fromString(reader.getString());
} else if ("isReadOnly".equals(fieldName)) {
deserializedConfigurationProperties.isReadOnly = IsReadOnly.fromString(reader.getString());
} else if ("isConfigPendingRestart".equals(fieldName)) {
deserializedConfigurationProperties.isConfigPendingRestart
= IsConfigPendingRestart.fromString(reader.getString());
} else if ("isDynamicConfig".equals(fieldName)) {
deserializedConfigurationProperties.isDynamicConfig
= IsDynamicConfig.fromString(reader.getString());
} else {
reader.skipChildren();
}
}
return deserializedConfigurationProperties;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy