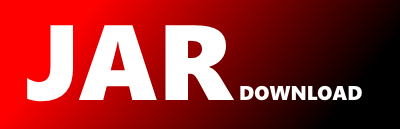
com.azure.resourcemanager.mysqlflexibleserver.implementation.CheckNameAvailabilitiesClientImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-mysqlflexibleserver Show documentation
Show all versions of azure-resourcemanager-mysqlflexibleserver Show documentation
This package contains Microsoft Azure SDK for MySql Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. The Microsoft Azure management API provides create, read, update, and delete functionality for Azure MySQL resources including servers, databases, firewall rules, VNET rules, log files and configurations with new business model. Package tag package-flexibleserver-2021-05-01.
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.mysqlflexibleserver.implementation;
import com.azure.core.annotation.BodyParam;
import com.azure.core.annotation.ExpectedResponses;
import com.azure.core.annotation.HeaderParam;
import com.azure.core.annotation.Headers;
import com.azure.core.annotation.Host;
import com.azure.core.annotation.HostParam;
import com.azure.core.annotation.PathParam;
import com.azure.core.annotation.Post;
import com.azure.core.annotation.QueryParam;
import com.azure.core.annotation.ReturnType;
import com.azure.core.annotation.ServiceInterface;
import com.azure.core.annotation.ServiceMethod;
import com.azure.core.annotation.UnexpectedResponseExceptionType;
import com.azure.core.http.rest.Response;
import com.azure.core.http.rest.RestProxy;
import com.azure.core.management.exception.ManagementException;
import com.azure.core.util.Context;
import com.azure.core.util.FluxUtil;
import com.azure.resourcemanager.mysqlflexibleserver.fluent.CheckNameAvailabilitiesClient;
import com.azure.resourcemanager.mysqlflexibleserver.fluent.models.NameAvailabilityInner;
import com.azure.resourcemanager.mysqlflexibleserver.models.NameAvailabilityRequest;
import reactor.core.publisher.Mono;
/**
* An instance of this class provides access to all the operations defined in CheckNameAvailabilitiesClient.
*/
public final class CheckNameAvailabilitiesClientImpl implements CheckNameAvailabilitiesClient {
/**
* The proxy service used to perform REST calls.
*/
private final CheckNameAvailabilitiesService service;
/**
* The service client containing this operation class.
*/
private final MySqlManagementClientImpl client;
/**
* Initializes an instance of CheckNameAvailabilitiesClientImpl.
*
* @param client the instance of the service client containing this operation class.
*/
CheckNameAvailabilitiesClientImpl(MySqlManagementClientImpl client) {
this.service = RestProxy.create(CheckNameAvailabilitiesService.class, client.getHttpPipeline(),
client.getSerializerAdapter());
this.client = client;
}
/**
* The interface defining all the services for MySqlManagementClientCheckNameAvailabilities to be used by the proxy
* service to perform REST calls.
*/
@Host("{$host}")
@ServiceInterface(name = "MySqlManagementClien")
public interface CheckNameAvailabilitiesService {
@Headers({ "Content-Type: application/json" })
@Post("/subscriptions/{subscriptionId}/providers/Microsoft.DBforMySQL/locations/{locationName}/checkNameAvailability")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono> execute(@HostParam("$host") String endpoint,
@QueryParam("api-version") String apiVersion, @PathParam("subscriptionId") String subscriptionId,
@PathParam("locationName") String locationName,
@BodyParam("application/json") NameAvailabilityRequest nameAvailabilityRequest,
@HeaderParam("Accept") String accept, Context context);
}
/**
* Check the availability of name for server.
*
* @param locationName The name of the location.
* @param nameAvailabilityRequest The required parameters for checking if server name is available.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return represents a resource name availability along with {@link Response} on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono> executeWithResponseAsync(String locationName,
NameAvailabilityRequest nameAvailabilityRequest) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (locationName == null) {
return Mono.error(new IllegalArgumentException("Parameter locationName is required and cannot be null."));
}
if (nameAvailabilityRequest == null) {
return Mono.error(
new IllegalArgumentException("Parameter nameAvailabilityRequest is required and cannot be null."));
} else {
nameAvailabilityRequest.validate();
}
final String accept = "application/json";
return FluxUtil
.withContext(context -> service.execute(this.client.getEndpoint(), this.client.getApiVersion(),
this.client.getSubscriptionId(), locationName, nameAvailabilityRequest, accept, context))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* Check the availability of name for server.
*
* @param locationName The name of the location.
* @param nameAvailabilityRequest The required parameters for checking if server name is available.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return represents a resource name availability along with {@link Response} on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono> executeWithResponseAsync(String locationName,
NameAvailabilityRequest nameAvailabilityRequest, Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (locationName == null) {
return Mono.error(new IllegalArgumentException("Parameter locationName is required and cannot be null."));
}
if (nameAvailabilityRequest == null) {
return Mono.error(
new IllegalArgumentException("Parameter nameAvailabilityRequest is required and cannot be null."));
} else {
nameAvailabilityRequest.validate();
}
final String accept = "application/json";
context = this.client.mergeContext(context);
return service.execute(this.client.getEndpoint(), this.client.getApiVersion(), this.client.getSubscriptionId(),
locationName, nameAvailabilityRequest, accept, context);
}
/**
* Check the availability of name for server.
*
* @param locationName The name of the location.
* @param nameAvailabilityRequest The required parameters for checking if server name is available.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return represents a resource name availability on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono executeAsync(String locationName,
NameAvailabilityRequest nameAvailabilityRequest) {
return executeWithResponseAsync(locationName, nameAvailabilityRequest)
.flatMap(res -> Mono.justOrEmpty(res.getValue()));
}
/**
* Check the availability of name for server.
*
* @param locationName The name of the location.
* @param nameAvailabilityRequest The required parameters for checking if server name is available.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return represents a resource name availability along with {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Response executeWithResponse(String locationName,
NameAvailabilityRequest nameAvailabilityRequest, Context context) {
return executeWithResponseAsync(locationName, nameAvailabilityRequest, context).block();
}
/**
* Check the availability of name for server.
*
* @param locationName The name of the location.
* @param nameAvailabilityRequest The required parameters for checking if server name is available.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return represents a resource name availability.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public NameAvailabilityInner execute(String locationName, NameAvailabilityRequest nameAvailabilityRequest) {
return executeWithResponse(locationName, nameAvailabilityRequest, Context.NONE).getValue();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy