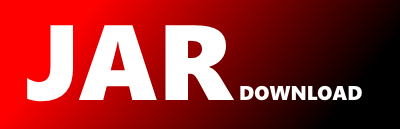
com.azure.resourcemanager.mysqlflexibleserver.implementation.DatabasesImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-mysqlflexibleserver Show documentation
Show all versions of azure-resourcemanager-mysqlflexibleserver Show documentation
This package contains Microsoft Azure SDK for MySql Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. The Microsoft Azure management API provides create, read, update, and delete functionality for Azure MySQL resources including servers, databases, firewall rules, VNET rules, log files and configurations with new business model. Package tag package-flexibleserver-2021-05-01.
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.mysqlflexibleserver.implementation;
import com.azure.core.http.rest.PagedIterable;
import com.azure.core.http.rest.Response;
import com.azure.core.http.rest.SimpleResponse;
import com.azure.core.util.Context;
import com.azure.core.util.logging.ClientLogger;
import com.azure.resourcemanager.mysqlflexibleserver.fluent.DatabasesClient;
import com.azure.resourcemanager.mysqlflexibleserver.fluent.models.DatabaseInner;
import com.azure.resourcemanager.mysqlflexibleserver.models.Database;
import com.azure.resourcemanager.mysqlflexibleserver.models.Databases;
public final class DatabasesImpl implements Databases {
private static final ClientLogger LOGGER = new ClientLogger(DatabasesImpl.class);
private final DatabasesClient innerClient;
private final com.azure.resourcemanager.mysqlflexibleserver.MySqlManager serviceManager;
public DatabasesImpl(DatabasesClient innerClient,
com.azure.resourcemanager.mysqlflexibleserver.MySqlManager serviceManager) {
this.innerClient = innerClient;
this.serviceManager = serviceManager;
}
public void delete(String resourceGroupName, String serverName, String databaseName) {
this.serviceClient().delete(resourceGroupName, serverName, databaseName);
}
public void delete(String resourceGroupName, String serverName, String databaseName, Context context) {
this.serviceClient().delete(resourceGroupName, serverName, databaseName, context);
}
public Response getWithResponse(String resourceGroupName, String serverName, String databaseName,
Context context) {
Response inner
= this.serviceClient().getWithResponse(resourceGroupName, serverName, databaseName, context);
if (inner != null) {
return new SimpleResponse<>(inner.getRequest(), inner.getStatusCode(), inner.getHeaders(),
new DatabaseImpl(inner.getValue(), this.manager()));
} else {
return null;
}
}
public Database get(String resourceGroupName, String serverName, String databaseName) {
DatabaseInner inner = this.serviceClient().get(resourceGroupName, serverName, databaseName);
if (inner != null) {
return new DatabaseImpl(inner, this.manager());
} else {
return null;
}
}
public PagedIterable listByServer(String resourceGroupName, String serverName) {
PagedIterable inner = this.serviceClient().listByServer(resourceGroupName, serverName);
return ResourceManagerUtils.mapPage(inner, inner1 -> new DatabaseImpl(inner1, this.manager()));
}
public PagedIterable listByServer(String resourceGroupName, String serverName, Context context) {
PagedIterable inner = this.serviceClient().listByServer(resourceGroupName, serverName, context);
return ResourceManagerUtils.mapPage(inner, inner1 -> new DatabaseImpl(inner1, this.manager()));
}
public Database getById(String id) {
String resourceGroupName = ResourceManagerUtils.getValueFromIdByName(id, "resourceGroups");
if (resourceGroupName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'resourceGroups'.", id)));
}
String serverName = ResourceManagerUtils.getValueFromIdByName(id, "flexibleServers");
if (serverName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'flexibleServers'.", id)));
}
String databaseName = ResourceManagerUtils.getValueFromIdByName(id, "databases");
if (databaseName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'databases'.", id)));
}
return this.getWithResponse(resourceGroupName, serverName, databaseName, Context.NONE).getValue();
}
public Response getByIdWithResponse(String id, Context context) {
String resourceGroupName = ResourceManagerUtils.getValueFromIdByName(id, "resourceGroups");
if (resourceGroupName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'resourceGroups'.", id)));
}
String serverName = ResourceManagerUtils.getValueFromIdByName(id, "flexibleServers");
if (serverName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'flexibleServers'.", id)));
}
String databaseName = ResourceManagerUtils.getValueFromIdByName(id, "databases");
if (databaseName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'databases'.", id)));
}
return this.getWithResponse(resourceGroupName, serverName, databaseName, context);
}
public void deleteById(String id) {
String resourceGroupName = ResourceManagerUtils.getValueFromIdByName(id, "resourceGroups");
if (resourceGroupName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'resourceGroups'.", id)));
}
String serverName = ResourceManagerUtils.getValueFromIdByName(id, "flexibleServers");
if (serverName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'flexibleServers'.", id)));
}
String databaseName = ResourceManagerUtils.getValueFromIdByName(id, "databases");
if (databaseName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'databases'.", id)));
}
this.delete(resourceGroupName, serverName, databaseName, Context.NONE);
}
public void deleteByIdWithResponse(String id, Context context) {
String resourceGroupName = ResourceManagerUtils.getValueFromIdByName(id, "resourceGroups");
if (resourceGroupName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'resourceGroups'.", id)));
}
String serverName = ResourceManagerUtils.getValueFromIdByName(id, "flexibleServers");
if (serverName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'flexibleServers'.", id)));
}
String databaseName = ResourceManagerUtils.getValueFromIdByName(id, "databases");
if (databaseName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'databases'.", id)));
}
this.delete(resourceGroupName, serverName, databaseName, context);
}
private DatabasesClient serviceClient() {
return this.innerClient;
}
private com.azure.resourcemanager.mysqlflexibleserver.MySqlManager manager() {
return this.serviceManager;
}
public DatabaseImpl define(String name) {
return new DatabaseImpl(name, this.manager());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy