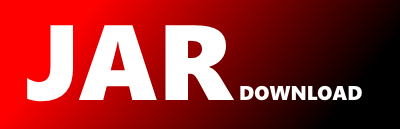
com.azure.resourcemanager.mysqlflexibleserver.models.DatabaseListResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-mysqlflexibleserver Show documentation
Show all versions of azure-resourcemanager-mysqlflexibleserver Show documentation
This package contains Microsoft Azure SDK for MySql Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. The Microsoft Azure management API provides create, read, update, and delete functionality for Azure MySQL resources including servers, databases, firewall rules, VNET rules, log files and configurations with new business model. Package tag package-flexibleserver-2021-05-01.
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.mysqlflexibleserver.models;
import com.azure.core.annotation.Fluent;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import com.azure.resourcemanager.mysqlflexibleserver.fluent.models.DatabaseInner;
import java.io.IOException;
import java.util.List;
/**
* A List of databases.
*/
@Fluent
public final class DatabaseListResult implements JsonSerializable {
/*
* The list of databases housed in a server
*/
private List value;
/*
* The link used to get the next page of operations.
*/
private String nextLink;
/**
* Creates an instance of DatabaseListResult class.
*/
public DatabaseListResult() {
}
/**
* Get the value property: The list of databases housed in a server.
*
* @return the value value.
*/
public List value() {
return this.value;
}
/**
* Set the value property: The list of databases housed in a server.
*
* @param value the value value to set.
* @return the DatabaseListResult object itself.
*/
public DatabaseListResult withValue(List value) {
this.value = value;
return this;
}
/**
* Get the nextLink property: The link used to get the next page of operations.
*
* @return the nextLink value.
*/
public String nextLink() {
return this.nextLink;
}
/**
* Set the nextLink property: The link used to get the next page of operations.
*
* @param nextLink the nextLink value to set.
* @return the DatabaseListResult object itself.
*/
public DatabaseListResult withNextLink(String nextLink) {
this.nextLink = nextLink;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (value() != null) {
value().forEach(e -> e.validate());
}
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeArrayField("value", this.value, (writer, element) -> writer.writeJson(element));
jsonWriter.writeStringField("nextLink", this.nextLink);
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of DatabaseListResult from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of DatabaseListResult if the JsonReader was pointing to an instance of it, or null if it was
* pointing to JSON null.
* @throws IOException If an error occurs while reading the DatabaseListResult.
*/
public static DatabaseListResult fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
DatabaseListResult deserializedDatabaseListResult = new DatabaseListResult();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("value".equals(fieldName)) {
List value = reader.readArray(reader1 -> DatabaseInner.fromJson(reader1));
deserializedDatabaseListResult.value = value;
} else if ("nextLink".equals(fieldName)) {
deserializedDatabaseListResult.nextLink = reader.getString();
} else {
reader.skipChildren();
}
}
return deserializedDatabaseListResult;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy