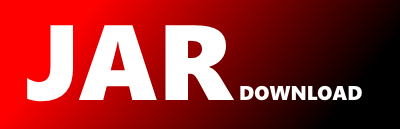
com.azure.resourcemanager.netapp.models.SubvolumeInfo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-netapp Show documentation
Show all versions of azure-resourcemanager-netapp Show documentation
This package contains Microsoft Azure SDK for NetAppFiles Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. Microsoft NetApp Files Azure Resource Provider specification. Package tag package-preview-2024-07-01-preview.
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.netapp.models;
import com.azure.core.management.SystemData;
import com.azure.core.util.Context;
import com.azure.resourcemanager.netapp.fluent.models.SubvolumeInfoInner;
/**
* An immutable client-side representation of SubvolumeInfo.
*/
public interface SubvolumeInfo {
/**
* Gets the id property: Fully qualified resource Id for the resource.
*
* @return the id value.
*/
String id();
/**
* Gets the name property: The name of the resource.
*
* @return the name value.
*/
String name();
/**
* Gets the type property: The type of the resource.
*
* @return the type value.
*/
String type();
/**
* Gets the systemData property: Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
* @return the systemData value.
*/
SystemData systemData();
/**
* Gets the path property: path
*
* Path to the subvolume.
*
* @return the path value.
*/
String path();
/**
* Gets the size property: size
*
* Truncate subvolume to the provided size in bytes.
*
* @return the size value.
*/
Long size();
/**
* Gets the parentPath property: name
*
* parent path to the subvolume.
*
* @return the parentPath value.
*/
String parentPath();
/**
* Gets the provisioningState property: Azure lifecycle management.
*
* @return the provisioningState value.
*/
String provisioningState();
/**
* Gets the name of the resource group.
*
* @return the name of the resource group.
*/
String resourceGroupName();
/**
* Gets the inner com.azure.resourcemanager.netapp.fluent.models.SubvolumeInfoInner object.
*
* @return the inner object.
*/
SubvolumeInfoInner innerModel();
/**
* The entirety of the SubvolumeInfo definition.
*/
interface Definition
extends DefinitionStages.Blank, DefinitionStages.WithParentResource, DefinitionStages.WithCreate {
}
/**
* The SubvolumeInfo definition stages.
*/
interface DefinitionStages {
/**
* The first stage of the SubvolumeInfo definition.
*/
interface Blank extends WithParentResource {
}
/**
* The stage of the SubvolumeInfo definition allowing to specify parent resource.
*/
interface WithParentResource {
/**
* Specifies resourceGroupName, accountName, poolName, volumeName.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @return the next definition stage.
*/
WithCreate withExistingVolume(String resourceGroupName, String accountName, String poolName,
String volumeName);
}
/**
* The stage of the SubvolumeInfo definition which contains all the minimum required properties for the resource
* to be created, but also allows for any other optional properties to be specified.
*/
interface WithCreate
extends DefinitionStages.WithPath, DefinitionStages.WithSize, DefinitionStages.WithParentPath {
/**
* Executes the create request.
*
* @return the created resource.
*/
SubvolumeInfo create();
/**
* Executes the create request.
*
* @param context The context to associate with this operation.
* @return the created resource.
*/
SubvolumeInfo create(Context context);
}
/**
* The stage of the SubvolumeInfo definition allowing to specify path.
*/
interface WithPath {
/**
* Specifies the path property: path
*
* Path to the subvolume.
*
* @param path path
*
* Path to the subvolume.
* @return the next definition stage.
*/
WithCreate withPath(String path);
}
/**
* The stage of the SubvolumeInfo definition allowing to specify size.
*/
interface WithSize {
/**
* Specifies the size property: size
*
* Truncate subvolume to the provided size in bytes.
*
* @param size size
*
* Truncate subvolume to the provided size in bytes.
* @return the next definition stage.
*/
WithCreate withSize(Long size);
}
/**
* The stage of the SubvolumeInfo definition allowing to specify parentPath.
*/
interface WithParentPath {
/**
* Specifies the parentPath property: name
*
* parent path to the subvolume.
*
* @param parentPath name
*
* parent path to the subvolume.
* @return the next definition stage.
*/
WithCreate withParentPath(String parentPath);
}
}
/**
* Begins update for the SubvolumeInfo resource.
*
* @return the stage of resource update.
*/
SubvolumeInfo.Update update();
/**
* The template for SubvolumeInfo update.
*/
interface Update extends UpdateStages.WithSize, UpdateStages.WithPath {
/**
* Executes the update request.
*
* @return the updated resource.
*/
SubvolumeInfo apply();
/**
* Executes the update request.
*
* @param context The context to associate with this operation.
* @return the updated resource.
*/
SubvolumeInfo apply(Context context);
}
/**
* The SubvolumeInfo update stages.
*/
interface UpdateStages {
/**
* The stage of the SubvolumeInfo update allowing to specify size.
*/
interface WithSize {
/**
* Specifies the size property: size
*
* Truncate subvolume to the provided size in bytes.
*
* @param size size
*
* Truncate subvolume to the provided size in bytes.
* @return the next definition stage.
*/
Update withSize(Long size);
}
/**
* The stage of the SubvolumeInfo update allowing to specify path.
*/
interface WithPath {
/**
* Specifies the path property: path
*
* path to the subvolume.
*
* @param path path
*
* path to the subvolume.
* @return the next definition stage.
*/
Update withPath(String path);
}
}
/**
* Refreshes the resource to sync with Azure.
*
* @return the refreshed resource.
*/
SubvolumeInfo refresh();
/**
* Refreshes the resource to sync with Azure.
*
* @param context The context to associate with this operation.
* @return the refreshed resource.
*/
SubvolumeInfo refresh(Context context);
/**
* Describe a subvolume
*
* Get details of the specified subvolume.
*
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return details of the specified subvolume.
*/
SubvolumeModel getMetadata();
/**
* Describe a subvolume
*
* Get details of the specified subvolume.
*
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return details of the specified subvolume.
*/
SubvolumeModel getMetadata(Context context);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy