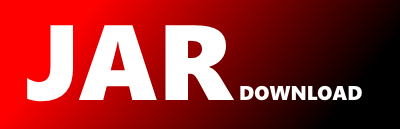
com.azure.resourcemanager.netapp.models.VolumePatch Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-netapp Show documentation
Show all versions of azure-resourcemanager-netapp Show documentation
This package contains Microsoft Azure SDK for NetAppFiles Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. Microsoft NetApp Files Azure Resource Provider specification. Package tag package-preview-2024-07-01-preview.
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.netapp.models;
import com.azure.core.annotation.Fluent;
import com.azure.core.management.Resource;
import com.azure.resourcemanager.netapp.fluent.models.VolumePatchProperties;
import com.fasterxml.jackson.annotation.JsonProperty;
import java.util.Map;
/**
* Volume patch resource.
*/
@Fluent
public final class VolumePatch extends Resource {
/*
* Patchable volume properties
*/
@JsonProperty(value = "properties")
private VolumePatchProperties innerProperties;
/**
* Creates an instance of VolumePatch class.
*/
public VolumePatch() {
}
/**
* Get the innerProperties property: Patchable volume properties.
*
* @return the innerProperties value.
*/
private VolumePatchProperties innerProperties() {
return this.innerProperties;
}
/**
* {@inheritDoc}
*/
@Override
public VolumePatch withLocation(String location) {
super.withLocation(location);
return this;
}
/**
* {@inheritDoc}
*/
@Override
public VolumePatch withTags(Map tags) {
super.withTags(tags);
return this;
}
/**
* Get the serviceLevel property: serviceLevel
*
* The service level of the file system.
*
* @return the serviceLevel value.
*/
public ServiceLevel serviceLevel() {
return this.innerProperties() == null ? null : this.innerProperties().serviceLevel();
}
/**
* Set the serviceLevel property: serviceLevel
*
* The service level of the file system.
*
* @param serviceLevel the serviceLevel value to set.
* @return the VolumePatch object itself.
*/
public VolumePatch withServiceLevel(ServiceLevel serviceLevel) {
if (this.innerProperties() == null) {
this.innerProperties = new VolumePatchProperties();
}
this.innerProperties().withServiceLevel(serviceLevel);
return this;
}
/**
* Get the usageThreshold property: usageThreshold
*
* Maximum storage quota allowed for a file system in bytes. This is a soft quota used for alerting only. Minimum
* size is 100 GiB. Upper limit is 100TiB, 500Tib for LargeVolume or 2400Tib for LargeVolume on exceptional basis.
* Specified in bytes.
*
* @return the usageThreshold value.
*/
public Long usageThreshold() {
return this.innerProperties() == null ? null : this.innerProperties().usageThreshold();
}
/**
* Set the usageThreshold property: usageThreshold
*
* Maximum storage quota allowed for a file system in bytes. This is a soft quota used for alerting only. Minimum
* size is 100 GiB. Upper limit is 100TiB, 500Tib for LargeVolume or 2400Tib for LargeVolume on exceptional basis.
* Specified in bytes.
*
* @param usageThreshold the usageThreshold value to set.
* @return the VolumePatch object itself.
*/
public VolumePatch withUsageThreshold(Long usageThreshold) {
if (this.innerProperties() == null) {
this.innerProperties = new VolumePatchProperties();
}
this.innerProperties().withUsageThreshold(usageThreshold);
return this;
}
/**
* Get the exportPolicy property: exportPolicy
*
* Set of export policy rules.
*
* @return the exportPolicy value.
*/
public VolumePatchPropertiesExportPolicy exportPolicy() {
return this.innerProperties() == null ? null : this.innerProperties().exportPolicy();
}
/**
* Set the exportPolicy property: exportPolicy
*
* Set of export policy rules.
*
* @param exportPolicy the exportPolicy value to set.
* @return the VolumePatch object itself.
*/
public VolumePatch withExportPolicy(VolumePatchPropertiesExportPolicy exportPolicy) {
if (this.innerProperties() == null) {
this.innerProperties = new VolumePatchProperties();
}
this.innerProperties().withExportPolicy(exportPolicy);
return this;
}
/**
* Get the throughputMibps property: Maximum throughput in MiB/s that can be achieved by this volume and this will
* be accepted as input only for manual qosType volume.
*
* @return the throughputMibps value.
*/
public Float throughputMibps() {
return this.innerProperties() == null ? null : this.innerProperties().throughputMibps();
}
/**
* Set the throughputMibps property: Maximum throughput in MiB/s that can be achieved by this volume and this will
* be accepted as input only for manual qosType volume.
*
* @param throughputMibps the throughputMibps value to set.
* @return the VolumePatch object itself.
*/
public VolumePatch withThroughputMibps(Float throughputMibps) {
if (this.innerProperties() == null) {
this.innerProperties = new VolumePatchProperties();
}
this.innerProperties().withThroughputMibps(throughputMibps);
return this;
}
/**
* Get the dataProtection property: DataProtection
*
* DataProtection type volumes include an object containing details of the replication.
*
* @return the dataProtection value.
*/
public VolumePatchPropertiesDataProtection dataProtection() {
return this.innerProperties() == null ? null : this.innerProperties().dataProtection();
}
/**
* Set the dataProtection property: DataProtection
*
* DataProtection type volumes include an object containing details of the replication.
*
* @param dataProtection the dataProtection value to set.
* @return the VolumePatch object itself.
*/
public VolumePatch withDataProtection(VolumePatchPropertiesDataProtection dataProtection) {
if (this.innerProperties() == null) {
this.innerProperties = new VolumePatchProperties();
}
this.innerProperties().withDataProtection(dataProtection);
return this;
}
/**
* Get the isDefaultQuotaEnabled property: Specifies if default quota is enabled for the volume.
*
* @return the isDefaultQuotaEnabled value.
*/
public Boolean isDefaultQuotaEnabled() {
return this.innerProperties() == null ? null : this.innerProperties().isDefaultQuotaEnabled();
}
/**
* Set the isDefaultQuotaEnabled property: Specifies if default quota is enabled for the volume.
*
* @param isDefaultQuotaEnabled the isDefaultQuotaEnabled value to set.
* @return the VolumePatch object itself.
*/
public VolumePatch withIsDefaultQuotaEnabled(Boolean isDefaultQuotaEnabled) {
if (this.innerProperties() == null) {
this.innerProperties = new VolumePatchProperties();
}
this.innerProperties().withIsDefaultQuotaEnabled(isDefaultQuotaEnabled);
return this;
}
/**
* Get the defaultUserQuotaInKiBs property: Default user quota for volume in KiBs. If isDefaultQuotaEnabled is set,
* the minimum value of 4 KiBs applies .
*
* @return the defaultUserQuotaInKiBs value.
*/
public Long defaultUserQuotaInKiBs() {
return this.innerProperties() == null ? null : this.innerProperties().defaultUserQuotaInKiBs();
}
/**
* Set the defaultUserQuotaInKiBs property: Default user quota for volume in KiBs. If isDefaultQuotaEnabled is set,
* the minimum value of 4 KiBs applies .
*
* @param defaultUserQuotaInKiBs the defaultUserQuotaInKiBs value to set.
* @return the VolumePatch object itself.
*/
public VolumePatch withDefaultUserQuotaInKiBs(Long defaultUserQuotaInKiBs) {
if (this.innerProperties() == null) {
this.innerProperties = new VolumePatchProperties();
}
this.innerProperties().withDefaultUserQuotaInKiBs(defaultUserQuotaInKiBs);
return this;
}
/**
* Get the defaultGroupQuotaInKiBs property: Default group quota for volume in KiBs. If isDefaultQuotaEnabled is
* set, the minimum value of 4 KiBs applies.
*
* @return the defaultGroupQuotaInKiBs value.
*/
public Long defaultGroupQuotaInKiBs() {
return this.innerProperties() == null ? null : this.innerProperties().defaultGroupQuotaInKiBs();
}
/**
* Set the defaultGroupQuotaInKiBs property: Default group quota for volume in KiBs. If isDefaultQuotaEnabled is
* set, the minimum value of 4 KiBs applies.
*
* @param defaultGroupQuotaInKiBs the defaultGroupQuotaInKiBs value to set.
* @return the VolumePatch object itself.
*/
public VolumePatch withDefaultGroupQuotaInKiBs(Long defaultGroupQuotaInKiBs) {
if (this.innerProperties() == null) {
this.innerProperties = new VolumePatchProperties();
}
this.innerProperties().withDefaultGroupQuotaInKiBs(defaultGroupQuotaInKiBs);
return this;
}
/**
* Get the unixPermissions property: UNIX permissions for NFS volume accepted in octal 4 digit format. First digit
* selects the set user ID(4), set group ID (2) and sticky (1) attributes. Second digit selects permission for the
* owner of the file: read (4), write (2) and execute (1). Third selects permissions for other users in the same
* group. the fourth for other users not in the group. 0755 - gives read/write/execute permissions to owner and
* read/execute to group and other users.
*
* @return the unixPermissions value.
*/
public String unixPermissions() {
return this.innerProperties() == null ? null : this.innerProperties().unixPermissions();
}
/**
* Set the unixPermissions property: UNIX permissions for NFS volume accepted in octal 4 digit format. First digit
* selects the set user ID(4), set group ID (2) and sticky (1) attributes. Second digit selects permission for the
* owner of the file: read (4), write (2) and execute (1). Third selects permissions for other users in the same
* group. the fourth for other users not in the group. 0755 - gives read/write/execute permissions to owner and
* read/execute to group and other users.
*
* @param unixPermissions the unixPermissions value to set.
* @return the VolumePatch object itself.
*/
public VolumePatch withUnixPermissions(String unixPermissions) {
if (this.innerProperties() == null) {
this.innerProperties = new VolumePatchProperties();
}
this.innerProperties().withUnixPermissions(unixPermissions);
return this;
}
/**
* Get the coolAccess property: Specifies whether Cool Access(tiering) is enabled for the volume.
*
* @return the coolAccess value.
*/
public Boolean coolAccess() {
return this.innerProperties() == null ? null : this.innerProperties().coolAccess();
}
/**
* Set the coolAccess property: Specifies whether Cool Access(tiering) is enabled for the volume.
*
* @param coolAccess the coolAccess value to set.
* @return the VolumePatch object itself.
*/
public VolumePatch withCoolAccess(Boolean coolAccess) {
if (this.innerProperties() == null) {
this.innerProperties = new VolumePatchProperties();
}
this.innerProperties().withCoolAccess(coolAccess);
return this;
}
/**
* Get the coolnessPeriod property: Specifies the number of days after which data that is not accessed by clients
* will be tiered.
*
* @return the coolnessPeriod value.
*/
public Integer coolnessPeriod() {
return this.innerProperties() == null ? null : this.innerProperties().coolnessPeriod();
}
/**
* Set the coolnessPeriod property: Specifies the number of days after which data that is not accessed by clients
* will be tiered.
*
* @param coolnessPeriod the coolnessPeriod value to set.
* @return the VolumePatch object itself.
*/
public VolumePatch withCoolnessPeriod(Integer coolnessPeriod) {
if (this.innerProperties() == null) {
this.innerProperties = new VolumePatchProperties();
}
this.innerProperties().withCoolnessPeriod(coolnessPeriod);
return this;
}
/**
* Get the coolAccessRetrievalPolicy property: coolAccessRetrievalPolicy determines the data retrieval behavior
* from the cool tier to standard storage based on the read pattern for cool access enabled volumes. The possible
* values for this field are:
* Default - Data will be pulled from cool tier to standard storage on random reads. This policy is the default.
* OnRead - All client-driven data read is pulled from cool tier to standard storage on both sequential and random
* reads.
* Never - No client-driven data is pulled from cool tier to standard storage.
*
* @return the coolAccessRetrievalPolicy value.
*/
public CoolAccessRetrievalPolicy coolAccessRetrievalPolicy() {
return this.innerProperties() == null ? null : this.innerProperties().coolAccessRetrievalPolicy();
}
/**
* Set the coolAccessRetrievalPolicy property: coolAccessRetrievalPolicy determines the data retrieval behavior
* from the cool tier to standard storage based on the read pattern for cool access enabled volumes. The possible
* values for this field are:
* Default - Data will be pulled from cool tier to standard storage on random reads. This policy is the default.
* OnRead - All client-driven data read is pulled from cool tier to standard storage on both sequential and random
* reads.
* Never - No client-driven data is pulled from cool tier to standard storage.
*
* @param coolAccessRetrievalPolicy the coolAccessRetrievalPolicy value to set.
* @return the VolumePatch object itself.
*/
public VolumePatch withCoolAccessRetrievalPolicy(CoolAccessRetrievalPolicy coolAccessRetrievalPolicy) {
if (this.innerProperties() == null) {
this.innerProperties = new VolumePatchProperties();
}
this.innerProperties().withCoolAccessRetrievalPolicy(coolAccessRetrievalPolicy);
return this;
}
/**
* Get the snapshotDirectoryVisible property: If enabled (true) the volume will contain a read-only snapshot
* directory which provides access to each of the volume's snapshots.
*
* @return the snapshotDirectoryVisible value.
*/
public Boolean snapshotDirectoryVisible() {
return this.innerProperties() == null ? null : this.innerProperties().snapshotDirectoryVisible();
}
/**
* Set the snapshotDirectoryVisible property: If enabled (true) the volume will contain a read-only snapshot
* directory which provides access to each of the volume's snapshots.
*
* @param snapshotDirectoryVisible the snapshotDirectoryVisible value to set.
* @return the VolumePatch object itself.
*/
public VolumePatch withSnapshotDirectoryVisible(Boolean snapshotDirectoryVisible) {
if (this.innerProperties() == null) {
this.innerProperties = new VolumePatchProperties();
}
this.innerProperties().withSnapshotDirectoryVisible(snapshotDirectoryVisible);
return this;
}
/**
* Get the smbAccessBasedEnumeration property: smbAccessBasedEnumeration
*
* Enables access-based enumeration share property for SMB Shares. Only applicable for SMB/DualProtocol volume.
*
* @return the smbAccessBasedEnumeration value.
*/
public SmbAccessBasedEnumeration smbAccessBasedEnumeration() {
return this.innerProperties() == null ? null : this.innerProperties().smbAccessBasedEnumeration();
}
/**
* Set the smbAccessBasedEnumeration property: smbAccessBasedEnumeration
*
* Enables access-based enumeration share property for SMB Shares. Only applicable for SMB/DualProtocol volume.
*
* @param smbAccessBasedEnumeration the smbAccessBasedEnumeration value to set.
* @return the VolumePatch object itself.
*/
public VolumePatch withSmbAccessBasedEnumeration(SmbAccessBasedEnumeration smbAccessBasedEnumeration) {
if (this.innerProperties() == null) {
this.innerProperties = new VolumePatchProperties();
}
this.innerProperties().withSmbAccessBasedEnumeration(smbAccessBasedEnumeration);
return this;
}
/**
* Get the smbNonBrowsable property: smbNonBrowsable
*
* Enables non-browsable property for SMB Shares. Only applicable for SMB/DualProtocol volume.
*
* @return the smbNonBrowsable value.
*/
public SmbNonBrowsable smbNonBrowsable() {
return this.innerProperties() == null ? null : this.innerProperties().smbNonBrowsable();
}
/**
* Set the smbNonBrowsable property: smbNonBrowsable
*
* Enables non-browsable property for SMB Shares. Only applicable for SMB/DualProtocol volume.
*
* @param smbNonBrowsable the smbNonBrowsable value to set.
* @return the VolumePatch object itself.
*/
public VolumePatch withSmbNonBrowsable(SmbNonBrowsable smbNonBrowsable) {
if (this.innerProperties() == null) {
this.innerProperties = new VolumePatchProperties();
}
this.innerProperties().withSmbNonBrowsable(smbNonBrowsable);
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (innerProperties() != null) {
innerProperties().validate();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy