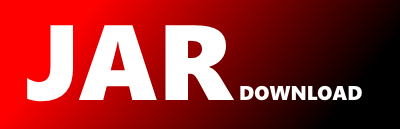
com.azure.resourcemanager.netapp.implementation.VolumesClientImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-netapp Show documentation
Show all versions of azure-resourcemanager-netapp Show documentation
This package contains Microsoft Azure SDK for NetAppFiles Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. Microsoft NetApp Files Azure Resource Provider specification. Package tag package-preview-2024-07-01-preview.
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.netapp.implementation;
import com.azure.core.annotation.BodyParam;
import com.azure.core.annotation.Delete;
import com.azure.core.annotation.ExpectedResponses;
import com.azure.core.annotation.Get;
import com.azure.core.annotation.HeaderParam;
import com.azure.core.annotation.Headers;
import com.azure.core.annotation.Host;
import com.azure.core.annotation.HostParam;
import com.azure.core.annotation.Patch;
import com.azure.core.annotation.PathParam;
import com.azure.core.annotation.Post;
import com.azure.core.annotation.Put;
import com.azure.core.annotation.QueryParam;
import com.azure.core.annotation.ReturnType;
import com.azure.core.annotation.ServiceInterface;
import com.azure.core.annotation.ServiceMethod;
import com.azure.core.annotation.UnexpectedResponseExceptionType;
import com.azure.core.http.rest.PagedFlux;
import com.azure.core.http.rest.PagedIterable;
import com.azure.core.http.rest.PagedResponse;
import com.azure.core.http.rest.PagedResponseBase;
import com.azure.core.http.rest.Response;
import com.azure.core.http.rest.RestProxy;
import com.azure.core.management.exception.ManagementException;
import com.azure.core.management.polling.PollResult;
import com.azure.core.util.Context;
import com.azure.core.util.FluxUtil;
import com.azure.core.util.polling.PollerFlux;
import com.azure.core.util.polling.SyncPoller;
import com.azure.resourcemanager.netapp.fluent.VolumesClient;
import com.azure.resourcemanager.netapp.fluent.models.ClusterPeerCommandResponseInner;
import com.azure.resourcemanager.netapp.fluent.models.GetGroupIdListForLdapUserResponseInner;
import com.azure.resourcemanager.netapp.fluent.models.ListQuotaReportResponseInner;
import com.azure.resourcemanager.netapp.fluent.models.ReplicationInner;
import com.azure.resourcemanager.netapp.fluent.models.ReplicationStatusInner;
import com.azure.resourcemanager.netapp.fluent.models.SvmPeerCommandResponseInner;
import com.azure.resourcemanager.netapp.fluent.models.VolumeInner;
import com.azure.resourcemanager.netapp.models.AuthorizeRequest;
import com.azure.resourcemanager.netapp.models.BreakFileLocksRequest;
import com.azure.resourcemanager.netapp.models.BreakReplicationRequest;
import com.azure.resourcemanager.netapp.models.GetGroupIdListForLdapUserRequest;
import com.azure.resourcemanager.netapp.models.ListReplications;
import com.azure.resourcemanager.netapp.models.PeerClusterForVolumeMigrationRequest;
import com.azure.resourcemanager.netapp.models.PoolChangeRequest;
import com.azure.resourcemanager.netapp.models.ReestablishReplicationRequest;
import com.azure.resourcemanager.netapp.models.RelocateVolumeRequest;
import com.azure.resourcemanager.netapp.models.VolumeList;
import com.azure.resourcemanager.netapp.models.VolumePatch;
import com.azure.resourcemanager.netapp.models.VolumeRevert;
import java.nio.ByteBuffer;
import reactor.core.publisher.Flux;
import reactor.core.publisher.Mono;
/**
* An instance of this class provides access to all the operations defined in VolumesClient.
*/
public final class VolumesClientImpl implements VolumesClient {
/**
* The proxy service used to perform REST calls.
*/
private final VolumesService service;
/**
* The service client containing this operation class.
*/
private final NetAppManagementClientImpl client;
/**
* Initializes an instance of VolumesClientImpl.
*
* @param client the instance of the service client containing this operation class.
*/
VolumesClientImpl(NetAppManagementClientImpl client) {
this.service = RestProxy.create(VolumesService.class, client.getHttpPipeline(), client.getSerializerAdapter());
this.client = client;
}
/**
* The interface defining all the services for NetAppManagementClientVolumes to be used by the proxy service to
* perform REST calls.
*/
@Host("{$host}")
@ServiceInterface(name = "NetAppManagementClie")
public interface VolumesService {
@Headers({ "Content-Type: application/json" })
@Get("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.NetApp/netAppAccounts/{accountName}/capacityPools/{poolName}/volumes")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono> list(@HostParam("$host") String endpoint,
@PathParam("subscriptionId") String subscriptionId,
@PathParam("resourceGroupName") String resourceGroupName, @PathParam("accountName") String accountName,
@PathParam("poolName") String poolName, @QueryParam("api-version") String apiVersion,
@HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Get("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.NetApp/netAppAccounts/{accountName}/capacityPools/{poolName}/volumes/{volumeName}")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono> get(@HostParam("$host") String endpoint,
@PathParam("subscriptionId") String subscriptionId,
@PathParam("resourceGroupName") String resourceGroupName, @PathParam("accountName") String accountName,
@PathParam("poolName") String poolName, @PathParam("volumeName") String volumeName,
@QueryParam("api-version") String apiVersion, @HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Put("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.NetApp/netAppAccounts/{accountName}/capacityPools/{poolName}/volumes/{volumeName}")
@ExpectedResponses({ 200, 201, 202 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono>> createOrUpdate(@HostParam("$host") String endpoint,
@PathParam("subscriptionId") String subscriptionId,
@PathParam("resourceGroupName") String resourceGroupName, @PathParam("accountName") String accountName,
@PathParam("poolName") String poolName, @PathParam("volumeName") String volumeName,
@QueryParam("api-version") String apiVersion, @BodyParam("application/json") VolumeInner body,
@HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Patch("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.NetApp/netAppAccounts/{accountName}/capacityPools/{poolName}/volumes/{volumeName}")
@ExpectedResponses({ 200, 202 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono>> update(@HostParam("$host") String endpoint,
@PathParam("subscriptionId") String subscriptionId,
@PathParam("resourceGroupName") String resourceGroupName, @PathParam("accountName") String accountName,
@PathParam("poolName") String poolName, @PathParam("volumeName") String volumeName,
@QueryParam("api-version") String apiVersion, @BodyParam("application/json") VolumePatch body,
@HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Delete("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.NetApp/netAppAccounts/{accountName}/capacityPools/{poolName}/volumes/{volumeName}")
@ExpectedResponses({ 202, 204 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono>> delete(@HostParam("$host") String endpoint,
@PathParam("subscriptionId") String subscriptionId,
@PathParam("resourceGroupName") String resourceGroupName, @PathParam("accountName") String accountName,
@PathParam("poolName") String poolName, @PathParam("volumeName") String volumeName,
@QueryParam("forceDelete") Boolean forceDelete, @QueryParam("api-version") String apiVersion,
@HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Post("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.NetApp/netAppAccounts/{accountName}/capacityPools/{poolName}/volumes/{volumeName}/populateAvailabilityZone")
@ExpectedResponses({ 200, 202 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono>> populateAvailabilityZone(@HostParam("$host") String endpoint,
@PathParam("subscriptionId") String subscriptionId,
@PathParam("resourceGroupName") String resourceGroupName, @PathParam("accountName") String accountName,
@PathParam("poolName") String poolName, @PathParam("volumeName") String volumeName,
@QueryParam("api-version") String apiVersion, @HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Post("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.NetApp/netAppAccounts/{accountName}/capacityPools/{poolName}/volumes/{volumeName}/revert")
@ExpectedResponses({ 200, 202 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono>> revert(@HostParam("$host") String endpoint,
@PathParam("subscriptionId") String subscriptionId,
@PathParam("resourceGroupName") String resourceGroupName, @PathParam("accountName") String accountName,
@PathParam("poolName") String poolName, @PathParam("volumeName") String volumeName,
@QueryParam("api-version") String apiVersion, @BodyParam("application/json") VolumeRevert body,
@HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Post("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.NetApp/netAppAccounts/{accountName}/capacityPools/{poolName}/volumes/{volumeName}/resetCifsPassword")
@ExpectedResponses({ 202 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono>> resetCifsPassword(@HostParam("$host") String endpoint,
@PathParam("subscriptionId") String subscriptionId,
@PathParam("resourceGroupName") String resourceGroupName, @PathParam("accountName") String accountName,
@PathParam("poolName") String poolName, @PathParam("volumeName") String volumeName,
@QueryParam("api-version") String apiVersion, @HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Post("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.NetApp/netAppAccounts/{accountName}/capacityPools/{poolName}/volumes/{volumeName}/splitCloneFromParent")
@ExpectedResponses({ 202 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono>> splitCloneFromParent(@HostParam("$host") String endpoint,
@PathParam("subscriptionId") String subscriptionId,
@PathParam("resourceGroupName") String resourceGroupName, @PathParam("accountName") String accountName,
@PathParam("poolName") String poolName, @PathParam("volumeName") String volumeName,
@QueryParam("api-version") String apiVersion, @HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Post("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.NetApp/netAppAccounts/{accountName}/capacityPools/{poolName}/volumes/{volumeName}/breakFileLocks")
@ExpectedResponses({ 200, 202 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono>> breakFileLocks(@HostParam("$host") String endpoint,
@PathParam("subscriptionId") String subscriptionId,
@PathParam("resourceGroupName") String resourceGroupName, @PathParam("accountName") String accountName,
@PathParam("poolName") String poolName, @PathParam("volumeName") String volumeName,
@QueryParam("api-version") String apiVersion, @BodyParam("application/json") BreakFileLocksRequest body,
@HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Post("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.NetApp/netAppAccounts/{accountName}/capacityPools/{poolName}/volumes/{volumeName}/getGroupIdListForLdapUser")
@ExpectedResponses({ 200, 202 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono>> listGetGroupIdListForLdapUser(@HostParam("$host") String endpoint,
@PathParam("subscriptionId") String subscriptionId,
@PathParam("resourceGroupName") String resourceGroupName, @PathParam("accountName") String accountName,
@PathParam("poolName") String poolName, @PathParam("volumeName") String volumeName,
@QueryParam("api-version") String apiVersion,
@BodyParam("application/json") GetGroupIdListForLdapUserRequest body, @HeaderParam("Accept") String accept,
Context context);
@Headers({ "Content-Type: application/json" })
@Post("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.NetApp/netAppAccounts/{accountName}/capacityPools/{poolName}/volumes/{volumeName}/listQuotaReport")
@ExpectedResponses({ 200, 202 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono>> listQuotaReport(@HostParam("$host") String endpoint,
@PathParam("subscriptionId") String subscriptionId,
@PathParam("resourceGroupName") String resourceGroupName, @PathParam("accountName") String accountName,
@PathParam("poolName") String poolName, @PathParam("volumeName") String volumeName,
@QueryParam("api-version") String apiVersion, @HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Post("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.NetApp/netAppAccounts/{accountName}/capacityPools/{poolName}/volumes/{volumeName}/breakReplication")
@ExpectedResponses({ 200, 202 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono>> breakReplication(@HostParam("$host") String endpoint,
@PathParam("subscriptionId") String subscriptionId,
@PathParam("resourceGroupName") String resourceGroupName, @PathParam("accountName") String accountName,
@PathParam("poolName") String poolName, @PathParam("volumeName") String volumeName,
@QueryParam("api-version") String apiVersion, @BodyParam("application/json") BreakReplicationRequest body,
@HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Post("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.NetApp/netAppAccounts/{accountName}/capacityPools/{poolName}/volumes/{volumeName}/reestablishReplication")
@ExpectedResponses({ 202 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono>> reestablishReplication(@HostParam("$host") String endpoint,
@PathParam("subscriptionId") String subscriptionId,
@PathParam("resourceGroupName") String resourceGroupName, @PathParam("accountName") String accountName,
@PathParam("poolName") String poolName, @PathParam("volumeName") String volumeName,
@QueryParam("api-version") String apiVersion,
@BodyParam("application/json") ReestablishReplicationRequest body, @HeaderParam("Accept") String accept,
Context context);
@Headers({ "Content-Type: application/json" })
@Get("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.NetApp/netAppAccounts/{accountName}/capacityPools/{poolName}/volumes/{volumeName}/replicationStatus")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono> replicationStatus(@HostParam("$host") String endpoint,
@PathParam("subscriptionId") String subscriptionId,
@PathParam("resourceGroupName") String resourceGroupName, @PathParam("accountName") String accountName,
@PathParam("poolName") String poolName, @PathParam("volumeName") String volumeName,
@QueryParam("api-version") String apiVersion, @HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Post("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.NetApp/netAppAccounts/{accountName}/capacityPools/{poolName}/volumes/{volumeName}/listReplications")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono> listReplications(@HostParam("$host") String endpoint,
@PathParam("subscriptionId") String subscriptionId,
@PathParam("resourceGroupName") String resourceGroupName, @PathParam("accountName") String accountName,
@PathParam("poolName") String poolName, @PathParam("volumeName") String volumeName,
@QueryParam("api-version") String apiVersion, @HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Post("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.NetApp/netAppAccounts/{accountName}/capacityPools/{poolName}/volumes/{volumeName}/resyncReplication")
@ExpectedResponses({ 200, 202 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono>> resyncReplication(@HostParam("$host") String endpoint,
@PathParam("subscriptionId") String subscriptionId,
@PathParam("resourceGroupName") String resourceGroupName, @PathParam("accountName") String accountName,
@PathParam("poolName") String poolName, @PathParam("volumeName") String volumeName,
@QueryParam("api-version") String apiVersion, @HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Post("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.NetApp/netAppAccounts/{accountName}/capacityPools/{poolName}/volumes/{volumeName}/deleteReplication")
@ExpectedResponses({ 200, 202 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono>> deleteReplication(@HostParam("$host") String endpoint,
@PathParam("subscriptionId") String subscriptionId,
@PathParam("resourceGroupName") String resourceGroupName, @PathParam("accountName") String accountName,
@PathParam("poolName") String poolName, @PathParam("volumeName") String volumeName,
@QueryParam("api-version") String apiVersion, @HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Post("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.NetApp/netAppAccounts/{accountName}/capacityPools/{poolName}/volumes/{volumeName}/authorizeReplication")
@ExpectedResponses({ 200, 202 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono>> authorizeReplication(@HostParam("$host") String endpoint,
@PathParam("subscriptionId") String subscriptionId,
@PathParam("resourceGroupName") String resourceGroupName, @PathParam("accountName") String accountName,
@PathParam("poolName") String poolName, @PathParam("volumeName") String volumeName,
@QueryParam("api-version") String apiVersion, @BodyParam("application/json") AuthorizeRequest body,
@HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Post("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.NetApp/netAppAccounts/{accountName}/capacityPools/{poolName}/volumes/{volumeName}/reinitializeReplication")
@ExpectedResponses({ 200, 202 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono>> reInitializeReplication(@HostParam("$host") String endpoint,
@PathParam("subscriptionId") String subscriptionId,
@PathParam("resourceGroupName") String resourceGroupName, @PathParam("accountName") String accountName,
@PathParam("poolName") String poolName, @PathParam("volumeName") String volumeName,
@QueryParam("api-version") String apiVersion, @HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Post("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.NetApp/netAppAccounts/{accountName}/capacityPools/{poolName}/volumes/{volumeName}/peerExternalCluster")
@ExpectedResponses({ 200, 202 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono>> peerExternalCluster(@HostParam("$host") String endpoint,
@PathParam("subscriptionId") String subscriptionId,
@PathParam("resourceGroupName") String resourceGroupName, @PathParam("accountName") String accountName,
@PathParam("poolName") String poolName, @PathParam("volumeName") String volumeName,
@QueryParam("api-version") String apiVersion,
@BodyParam("application/json") PeerClusterForVolumeMigrationRequest body,
@HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Post("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.NetApp/netAppAccounts/{accountName}/capacityPools/{poolName}/volumes/{volumeName}/authorizeExternalReplication")
@ExpectedResponses({ 200, 202 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono>> authorizeExternalReplication(@HostParam("$host") String endpoint,
@PathParam("subscriptionId") String subscriptionId,
@PathParam("resourceGroupName") String resourceGroupName, @PathParam("accountName") String accountName,
@PathParam("poolName") String poolName, @PathParam("volumeName") String volumeName,
@QueryParam("api-version") String apiVersion, @HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Post("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.NetApp/netAppAccounts/{accountName}/capacityPools/{poolName}/volumes/{volumeName}/finalizeExternalReplication")
@ExpectedResponses({ 202 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono>> finalizeExternalReplication(@HostParam("$host") String endpoint,
@PathParam("subscriptionId") String subscriptionId,
@PathParam("resourceGroupName") String resourceGroupName, @PathParam("accountName") String accountName,
@PathParam("poolName") String poolName, @PathParam("volumeName") String volumeName,
@QueryParam("api-version") String apiVersion, @HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Post("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.NetApp/netAppAccounts/{accountName}/capacityPools/{poolName}/volumes/{volumeName}/performReplicationTransfer")
@ExpectedResponses({ 202 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono>> performReplicationTransfer(@HostParam("$host") String endpoint,
@PathParam("subscriptionId") String subscriptionId,
@PathParam("resourceGroupName") String resourceGroupName, @PathParam("accountName") String accountName,
@PathParam("poolName") String poolName, @PathParam("volumeName") String volumeName,
@QueryParam("api-version") String apiVersion, @HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Post("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.NetApp/netAppAccounts/{accountName}/capacityPools/{poolName}/volumes/{volumeName}/poolChange")
@ExpectedResponses({ 200, 202 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono>> poolChange(@HostParam("$host") String endpoint,
@PathParam("subscriptionId") String subscriptionId,
@PathParam("resourceGroupName") String resourceGroupName, @PathParam("accountName") String accountName,
@PathParam("poolName") String poolName, @PathParam("volumeName") String volumeName,
@QueryParam("api-version") String apiVersion, @BodyParam("application/json") PoolChangeRequest body,
@HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Post("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.NetApp/netAppAccounts/{accountName}/capacityPools/{poolName}/volumes/{volumeName}/relocate")
@ExpectedResponses({ 200, 202 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono>> relocate(@HostParam("$host") String endpoint,
@PathParam("subscriptionId") String subscriptionId,
@PathParam("resourceGroupName") String resourceGroupName, @PathParam("accountName") String accountName,
@PathParam("poolName") String poolName, @PathParam("volumeName") String volumeName,
@QueryParam("api-version") String apiVersion, @BodyParam("application/json") RelocateVolumeRequest body,
@HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Post("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.NetApp/netAppAccounts/{accountName}/capacityPools/{poolName}/volumes/{volumeName}/finalizeRelocation")
@ExpectedResponses({ 200, 202 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono>> finalizeRelocation(@HostParam("$host") String endpoint,
@PathParam("subscriptionId") String subscriptionId,
@PathParam("resourceGroupName") String resourceGroupName, @PathParam("accountName") String accountName,
@PathParam("poolName") String poolName, @PathParam("volumeName") String volumeName,
@QueryParam("api-version") String apiVersion, @HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Post("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.NetApp/netAppAccounts/{accountName}/capacityPools/{poolName}/volumes/{volumeName}/revertRelocation")
@ExpectedResponses({ 200, 202 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono>> revertRelocation(@HostParam("$host") String endpoint,
@PathParam("subscriptionId") String subscriptionId,
@PathParam("resourceGroupName") String resourceGroupName, @PathParam("accountName") String accountName,
@PathParam("poolName") String poolName, @PathParam("volumeName") String volumeName,
@QueryParam("api-version") String apiVersion, @HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Get("{nextLink}")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono> listNext(@PathParam(value = "nextLink", encoded = true) String nextLink,
@HostParam("$host") String endpoint, @HeaderParam("Accept") String accept, Context context);
}
/**
* Describe all volumes
*
* List all volumes within the capacity pool.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return list of volume resources along with {@link PagedResponse} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono> listSinglePageAsync(String resourceGroupName, String accountName,
String poolName) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (accountName == null) {
return Mono.error(new IllegalArgumentException("Parameter accountName is required and cannot be null."));
}
if (poolName == null) {
return Mono.error(new IllegalArgumentException("Parameter poolName is required and cannot be null."));
}
final String accept = "application/json";
return FluxUtil
.withContext(context -> service.list(this.client.getEndpoint(), this.client.getSubscriptionId(),
resourceGroupName, accountName, poolName, this.client.getApiVersion(), accept, context))
.>map(res -> new PagedResponseBase<>(res.getRequest(), res.getStatusCode(),
res.getHeaders(), res.getValue().value(), res.getValue().nextLink(), null))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* Describe all volumes
*
* List all volumes within the capacity pool.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return list of volume resources along with {@link PagedResponse} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono> listSinglePageAsync(String resourceGroupName, String accountName,
String poolName, Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (accountName == null) {
return Mono.error(new IllegalArgumentException("Parameter accountName is required and cannot be null."));
}
if (poolName == null) {
return Mono.error(new IllegalArgumentException("Parameter poolName is required and cannot be null."));
}
final String accept = "application/json";
context = this.client.mergeContext(context);
return service
.list(this.client.getEndpoint(), this.client.getSubscriptionId(), resourceGroupName, accountName, poolName,
this.client.getApiVersion(), accept, context)
.map(res -> new PagedResponseBase<>(res.getRequest(), res.getStatusCode(), res.getHeaders(),
res.getValue().value(), res.getValue().nextLink(), null));
}
/**
* Describe all volumes
*
* List all volumes within the capacity pool.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return list of volume resources as paginated response with {@link PagedFlux}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
private PagedFlux listAsync(String resourceGroupName, String accountName, String poolName) {
return new PagedFlux<>(() -> listSinglePageAsync(resourceGroupName, accountName, poolName),
nextLink -> listNextSinglePageAsync(nextLink));
}
/**
* Describe all volumes
*
* List all volumes within the capacity pool.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return list of volume resources as paginated response with {@link PagedFlux}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
private PagedFlux listAsync(String resourceGroupName, String accountName, String poolName,
Context context) {
return new PagedFlux<>(() -> listSinglePageAsync(resourceGroupName, accountName, poolName, context),
nextLink -> listNextSinglePageAsync(nextLink, context));
}
/**
* Describe all volumes
*
* List all volumes within the capacity pool.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return list of volume resources as paginated response with {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
public PagedIterable list(String resourceGroupName, String accountName, String poolName) {
return new PagedIterable<>(listAsync(resourceGroupName, accountName, poolName));
}
/**
* Describe all volumes
*
* List all volumes within the capacity pool.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return list of volume resources as paginated response with {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
public PagedIterable list(String resourceGroupName, String accountName, String poolName,
Context context) {
return new PagedIterable<>(listAsync(resourceGroupName, accountName, poolName, context));
}
/**
* Describe a volume
*
* Get the details of the specified volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the details of the specified volume along with {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono> getWithResponseAsync(String resourceGroupName, String accountName,
String poolName, String volumeName) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (accountName == null) {
return Mono.error(new IllegalArgumentException("Parameter accountName is required and cannot be null."));
}
if (poolName == null) {
return Mono.error(new IllegalArgumentException("Parameter poolName is required and cannot be null."));
}
if (volumeName == null) {
return Mono.error(new IllegalArgumentException("Parameter volumeName is required and cannot be null."));
}
final String accept = "application/json";
return FluxUtil
.withContext(context -> service.get(this.client.getEndpoint(), this.client.getSubscriptionId(),
resourceGroupName, accountName, poolName, volumeName, this.client.getApiVersion(), accept, context))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* Describe a volume
*
* Get the details of the specified volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the details of the specified volume along with {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono> getWithResponseAsync(String resourceGroupName, String accountName,
String poolName, String volumeName, Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (accountName == null) {
return Mono.error(new IllegalArgumentException("Parameter accountName is required and cannot be null."));
}
if (poolName == null) {
return Mono.error(new IllegalArgumentException("Parameter poolName is required and cannot be null."));
}
if (volumeName == null) {
return Mono.error(new IllegalArgumentException("Parameter volumeName is required and cannot be null."));
}
final String accept = "application/json";
context = this.client.mergeContext(context);
return service.get(this.client.getEndpoint(), this.client.getSubscriptionId(), resourceGroupName, accountName,
poolName, volumeName, this.client.getApiVersion(), accept, context);
}
/**
* Describe a volume
*
* Get the details of the specified volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the details of the specified volume on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono getAsync(String resourceGroupName, String accountName, String poolName,
String volumeName) {
return getWithResponseAsync(resourceGroupName, accountName, poolName, volumeName)
.flatMap(res -> Mono.justOrEmpty(res.getValue()));
}
/**
* Describe a volume
*
* Get the details of the specified volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the details of the specified volume along with {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Response getWithResponse(String resourceGroupName, String accountName, String poolName,
String volumeName, Context context) {
return getWithResponseAsync(resourceGroupName, accountName, poolName, volumeName, context).block();
}
/**
* Describe a volume
*
* Get the details of the specified volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the details of the specified volume.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public VolumeInner get(String resourceGroupName, String accountName, String poolName, String volumeName) {
return getWithResponse(resourceGroupName, accountName, poolName, volumeName, Context.NONE).getValue();
}
/**
* Create or Update a volume
*
* Create or update the specified volume within the capacity pool.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param body Volume object supplied in the body of the operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return volume resource along with {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono>> createOrUpdateWithResponseAsync(String resourceGroupName,
String accountName, String poolName, String volumeName, VolumeInner body) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (accountName == null) {
return Mono.error(new IllegalArgumentException("Parameter accountName is required and cannot be null."));
}
if (poolName == null) {
return Mono.error(new IllegalArgumentException("Parameter poolName is required and cannot be null."));
}
if (volumeName == null) {
return Mono.error(new IllegalArgumentException("Parameter volumeName is required and cannot be null."));
}
if (body == null) {
return Mono.error(new IllegalArgumentException("Parameter body is required and cannot be null."));
} else {
body.validate();
}
final String accept = "application/json";
return FluxUtil
.withContext(context -> service.createOrUpdate(this.client.getEndpoint(), this.client.getSubscriptionId(),
resourceGroupName, accountName, poolName, volumeName, this.client.getApiVersion(), body, accept,
context))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* Create or Update a volume
*
* Create or update the specified volume within the capacity pool.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param body Volume object supplied in the body of the operation.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return volume resource along with {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono>> createOrUpdateWithResponseAsync(String resourceGroupName,
String accountName, String poolName, String volumeName, VolumeInner body, Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (accountName == null) {
return Mono.error(new IllegalArgumentException("Parameter accountName is required and cannot be null."));
}
if (poolName == null) {
return Mono.error(new IllegalArgumentException("Parameter poolName is required and cannot be null."));
}
if (volumeName == null) {
return Mono.error(new IllegalArgumentException("Parameter volumeName is required and cannot be null."));
}
if (body == null) {
return Mono.error(new IllegalArgumentException("Parameter body is required and cannot be null."));
} else {
body.validate();
}
final String accept = "application/json";
context = this.client.mergeContext(context);
return service.createOrUpdate(this.client.getEndpoint(), this.client.getSubscriptionId(), resourceGroupName,
accountName, poolName, volumeName, this.client.getApiVersion(), body, accept, context);
}
/**
* Create or Update a volume
*
* Create or update the specified volume within the capacity pool.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param body Volume object supplied in the body of the operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of volume resource.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
private PollerFlux, VolumeInner> beginCreateOrUpdateAsync(String resourceGroupName,
String accountName, String poolName, String volumeName, VolumeInner body) {
Mono>> mono
= createOrUpdateWithResponseAsync(resourceGroupName, accountName, poolName, volumeName, body);
return this.client.getLroResult(mono, this.client.getHttpPipeline(),
VolumeInner.class, VolumeInner.class, this.client.getContext());
}
/**
* Create or Update a volume
*
* Create or update the specified volume within the capacity pool.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param body Volume object supplied in the body of the operation.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of volume resource.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
private PollerFlux, VolumeInner> beginCreateOrUpdateAsync(String resourceGroupName,
String accountName, String poolName, String volumeName, VolumeInner body, Context context) {
context = this.client.mergeContext(context);
Mono>> mono
= createOrUpdateWithResponseAsync(resourceGroupName, accountName, poolName, volumeName, body, context);
return this.client.getLroResult(mono, this.client.getHttpPipeline(),
VolumeInner.class, VolumeInner.class, context);
}
/**
* Create or Update a volume
*
* Create or update the specified volume within the capacity pool.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param body Volume object supplied in the body of the operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of volume resource.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, VolumeInner> beginCreateOrUpdate(String resourceGroupName,
String accountName, String poolName, String volumeName, VolumeInner body) {
return this.beginCreateOrUpdateAsync(resourceGroupName, accountName, poolName, volumeName, body)
.getSyncPoller();
}
/**
* Create or Update a volume
*
* Create or update the specified volume within the capacity pool.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param body Volume object supplied in the body of the operation.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of volume resource.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, VolumeInner> beginCreateOrUpdate(String resourceGroupName,
String accountName, String poolName, String volumeName, VolumeInner body, Context context) {
return this.beginCreateOrUpdateAsync(resourceGroupName, accountName, poolName, volumeName, body, context)
.getSyncPoller();
}
/**
* Create or Update a volume
*
* Create or update the specified volume within the capacity pool.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param body Volume object supplied in the body of the operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return volume resource on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono createOrUpdateAsync(String resourceGroupName, String accountName, String poolName,
String volumeName, VolumeInner body) {
return beginCreateOrUpdateAsync(resourceGroupName, accountName, poolName, volumeName, body).last()
.flatMap(this.client::getLroFinalResultOrError);
}
/**
* Create or Update a volume
*
* Create or update the specified volume within the capacity pool.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param body Volume object supplied in the body of the operation.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return volume resource on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono createOrUpdateAsync(String resourceGroupName, String accountName, String poolName,
String volumeName, VolumeInner body, Context context) {
return beginCreateOrUpdateAsync(resourceGroupName, accountName, poolName, volumeName, body, context).last()
.flatMap(this.client::getLroFinalResultOrError);
}
/**
* Create or Update a volume
*
* Create or update the specified volume within the capacity pool.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param body Volume object supplied in the body of the operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return volume resource.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public VolumeInner createOrUpdate(String resourceGroupName, String accountName, String poolName, String volumeName,
VolumeInner body) {
return createOrUpdateAsync(resourceGroupName, accountName, poolName, volumeName, body).block();
}
/**
* Create or Update a volume
*
* Create or update the specified volume within the capacity pool.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param body Volume object supplied in the body of the operation.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return volume resource.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public VolumeInner createOrUpdate(String resourceGroupName, String accountName, String poolName, String volumeName,
VolumeInner body, Context context) {
return createOrUpdateAsync(resourceGroupName, accountName, poolName, volumeName, body, context).block();
}
/**
* Update a volume
*
* Patch the specified volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param body Volume object supplied in the body of the operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return volume resource along with {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono>> updateWithResponseAsync(String resourceGroupName, String accountName,
String poolName, String volumeName, VolumePatch body) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (accountName == null) {
return Mono.error(new IllegalArgumentException("Parameter accountName is required and cannot be null."));
}
if (poolName == null) {
return Mono.error(new IllegalArgumentException("Parameter poolName is required and cannot be null."));
}
if (volumeName == null) {
return Mono.error(new IllegalArgumentException("Parameter volumeName is required and cannot be null."));
}
if (body == null) {
return Mono.error(new IllegalArgumentException("Parameter body is required and cannot be null."));
} else {
body.validate();
}
final String accept = "application/json";
return FluxUtil
.withContext(
context -> service.update(this.client.getEndpoint(), this.client.getSubscriptionId(), resourceGroupName,
accountName, poolName, volumeName, this.client.getApiVersion(), body, accept, context))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* Update a volume
*
* Patch the specified volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param body Volume object supplied in the body of the operation.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return volume resource along with {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono>> updateWithResponseAsync(String resourceGroupName, String accountName,
String poolName, String volumeName, VolumePatch body, Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (accountName == null) {
return Mono.error(new IllegalArgumentException("Parameter accountName is required and cannot be null."));
}
if (poolName == null) {
return Mono.error(new IllegalArgumentException("Parameter poolName is required and cannot be null."));
}
if (volumeName == null) {
return Mono.error(new IllegalArgumentException("Parameter volumeName is required and cannot be null."));
}
if (body == null) {
return Mono.error(new IllegalArgumentException("Parameter body is required and cannot be null."));
} else {
body.validate();
}
final String accept = "application/json";
context = this.client.mergeContext(context);
return service.update(this.client.getEndpoint(), this.client.getSubscriptionId(), resourceGroupName,
accountName, poolName, volumeName, this.client.getApiVersion(), body, accept, context);
}
/**
* Update a volume
*
* Patch the specified volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param body Volume object supplied in the body of the operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of volume resource.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
private PollerFlux, VolumeInner> beginUpdateAsync(String resourceGroupName,
String accountName, String poolName, String volumeName, VolumePatch body) {
Mono>> mono
= updateWithResponseAsync(resourceGroupName, accountName, poolName, volumeName, body);
return this.client.getLroResult(mono, this.client.getHttpPipeline(),
VolumeInner.class, VolumeInner.class, this.client.getContext());
}
/**
* Update a volume
*
* Patch the specified volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param body Volume object supplied in the body of the operation.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of volume resource.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
private PollerFlux, VolumeInner> beginUpdateAsync(String resourceGroupName,
String accountName, String poolName, String volumeName, VolumePatch body, Context context) {
context = this.client.mergeContext(context);
Mono>> mono
= updateWithResponseAsync(resourceGroupName, accountName, poolName, volumeName, body, context);
return this.client.getLroResult(mono, this.client.getHttpPipeline(),
VolumeInner.class, VolumeInner.class, context);
}
/**
* Update a volume
*
* Patch the specified volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param body Volume object supplied in the body of the operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of volume resource.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, VolumeInner> beginUpdate(String resourceGroupName, String accountName,
String poolName, String volumeName, VolumePatch body) {
return this.beginUpdateAsync(resourceGroupName, accountName, poolName, volumeName, body).getSyncPoller();
}
/**
* Update a volume
*
* Patch the specified volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param body Volume object supplied in the body of the operation.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of volume resource.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, VolumeInner> beginUpdate(String resourceGroupName, String accountName,
String poolName, String volumeName, VolumePatch body, Context context) {
return this.beginUpdateAsync(resourceGroupName, accountName, poolName, volumeName, body, context)
.getSyncPoller();
}
/**
* Update a volume
*
* Patch the specified volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param body Volume object supplied in the body of the operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return volume resource on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono updateAsync(String resourceGroupName, String accountName, String poolName,
String volumeName, VolumePatch body) {
return beginUpdateAsync(resourceGroupName, accountName, poolName, volumeName, body).last()
.flatMap(this.client::getLroFinalResultOrError);
}
/**
* Update a volume
*
* Patch the specified volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param body Volume object supplied in the body of the operation.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return volume resource on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono updateAsync(String resourceGroupName, String accountName, String poolName,
String volumeName, VolumePatch body, Context context) {
return beginUpdateAsync(resourceGroupName, accountName, poolName, volumeName, body, context).last()
.flatMap(this.client::getLroFinalResultOrError);
}
/**
* Update a volume
*
* Patch the specified volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param body Volume object supplied in the body of the operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return volume resource.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public VolumeInner update(String resourceGroupName, String accountName, String poolName, String volumeName,
VolumePatch body) {
return updateAsync(resourceGroupName, accountName, poolName, volumeName, body).block();
}
/**
* Update a volume
*
* Patch the specified volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param body Volume object supplied in the body of the operation.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return volume resource.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public VolumeInner update(String resourceGroupName, String accountName, String poolName, String volumeName,
VolumePatch body, Context context) {
return updateAsync(resourceGroupName, accountName, poolName, volumeName, body, context).block();
}
/**
* Delete a volume
*
* Delete the specified volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param forceDelete An option to force delete the volume. Will cleanup resources connected to the particular
* volume.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono>> deleteWithResponseAsync(String resourceGroupName, String accountName,
String poolName, String volumeName, Boolean forceDelete) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (accountName == null) {
return Mono.error(new IllegalArgumentException("Parameter accountName is required and cannot be null."));
}
if (poolName == null) {
return Mono.error(new IllegalArgumentException("Parameter poolName is required and cannot be null."));
}
if (volumeName == null) {
return Mono.error(new IllegalArgumentException("Parameter volumeName is required and cannot be null."));
}
final String accept = "application/json";
return FluxUtil
.withContext(
context -> service.delete(this.client.getEndpoint(), this.client.getSubscriptionId(), resourceGroupName,
accountName, poolName, volumeName, forceDelete, this.client.getApiVersion(), accept, context))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* Delete a volume
*
* Delete the specified volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param forceDelete An option to force delete the volume. Will cleanup resources connected to the particular
* volume.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono>> deleteWithResponseAsync(String resourceGroupName, String accountName,
String poolName, String volumeName, Boolean forceDelete, Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (accountName == null) {
return Mono.error(new IllegalArgumentException("Parameter accountName is required and cannot be null."));
}
if (poolName == null) {
return Mono.error(new IllegalArgumentException("Parameter poolName is required and cannot be null."));
}
if (volumeName == null) {
return Mono.error(new IllegalArgumentException("Parameter volumeName is required and cannot be null."));
}
final String accept = "application/json";
context = this.client.mergeContext(context);
return service.delete(this.client.getEndpoint(), this.client.getSubscriptionId(), resourceGroupName,
accountName, poolName, volumeName, forceDelete, this.client.getApiVersion(), accept, context);
}
/**
* Delete a volume
*
* Delete the specified volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param forceDelete An option to force delete the volume. Will cleanup resources connected to the particular
* volume.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
private PollerFlux, Void> beginDeleteAsync(String resourceGroupName, String accountName,
String poolName, String volumeName, Boolean forceDelete) {
Mono>> mono
= deleteWithResponseAsync(resourceGroupName, accountName, poolName, volumeName, forceDelete);
return this.client.getLroResult(mono, this.client.getHttpPipeline(), Void.class, Void.class,
this.client.getContext());
}
/**
* Delete a volume
*
* Delete the specified volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
private PollerFlux, Void> beginDeleteAsync(String resourceGroupName, String accountName,
String poolName, String volumeName) {
final Boolean forceDelete = null;
Mono>> mono
= deleteWithResponseAsync(resourceGroupName, accountName, poolName, volumeName, forceDelete);
return this.client.getLroResult(mono, this.client.getHttpPipeline(), Void.class, Void.class,
this.client.getContext());
}
/**
* Delete a volume
*
* Delete the specified volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param forceDelete An option to force delete the volume. Will cleanup resources connected to the particular
* volume.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
private PollerFlux, Void> beginDeleteAsync(String resourceGroupName, String accountName,
String poolName, String volumeName, Boolean forceDelete, Context context) {
context = this.client.mergeContext(context);
Mono>> mono
= deleteWithResponseAsync(resourceGroupName, accountName, poolName, volumeName, forceDelete, context);
return this.client.getLroResult(mono, this.client.getHttpPipeline(), Void.class, Void.class,
context);
}
/**
* Delete a volume
*
* Delete the specified volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, Void> beginDelete(String resourceGroupName, String accountName, String poolName,
String volumeName) {
final Boolean forceDelete = null;
return this.beginDeleteAsync(resourceGroupName, accountName, poolName, volumeName, forceDelete).getSyncPoller();
}
/**
* Delete a volume
*
* Delete the specified volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param forceDelete An option to force delete the volume. Will cleanup resources connected to the particular
* volume.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, Void> beginDelete(String resourceGroupName, String accountName, String poolName,
String volumeName, Boolean forceDelete, Context context) {
return this.beginDeleteAsync(resourceGroupName, accountName, poolName, volumeName, forceDelete, context)
.getSyncPoller();
}
/**
* Delete a volume
*
* Delete the specified volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param forceDelete An option to force delete the volume. Will cleanup resources connected to the particular
* volume.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono deleteAsync(String resourceGroupName, String accountName, String poolName, String volumeName,
Boolean forceDelete) {
return beginDeleteAsync(resourceGroupName, accountName, poolName, volumeName, forceDelete).last()
.flatMap(this.client::getLroFinalResultOrError);
}
/**
* Delete a volume
*
* Delete the specified volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono deleteAsync(String resourceGroupName, String accountName, String poolName, String volumeName) {
final Boolean forceDelete = null;
return beginDeleteAsync(resourceGroupName, accountName, poolName, volumeName, forceDelete).last()
.flatMap(this.client::getLroFinalResultOrError);
}
/**
* Delete a volume
*
* Delete the specified volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param forceDelete An option to force delete the volume. Will cleanup resources connected to the particular
* volume.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono deleteAsync(String resourceGroupName, String accountName, String poolName, String volumeName,
Boolean forceDelete, Context context) {
return beginDeleteAsync(resourceGroupName, accountName, poolName, volumeName, forceDelete, context).last()
.flatMap(this.client::getLroFinalResultOrError);
}
/**
* Delete a volume
*
* Delete the specified volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public void delete(String resourceGroupName, String accountName, String poolName, String volumeName) {
final Boolean forceDelete = null;
deleteAsync(resourceGroupName, accountName, poolName, volumeName, forceDelete).block();
}
/**
* Delete a volume
*
* Delete the specified volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param forceDelete An option to force delete the volume. Will cleanup resources connected to the particular
* volume.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public void delete(String resourceGroupName, String accountName, String poolName, String volumeName,
Boolean forceDelete, Context context) {
deleteAsync(resourceGroupName, accountName, poolName, volumeName, forceDelete, context).block();
}
/**
* Populate Availability Zone
*
* This operation will populate availability zone information for a volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return volume resource along with {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono>> populateAvailabilityZoneWithResponseAsync(String resourceGroupName,
String accountName, String poolName, String volumeName) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (accountName == null) {
return Mono.error(new IllegalArgumentException("Parameter accountName is required and cannot be null."));
}
if (poolName == null) {
return Mono.error(new IllegalArgumentException("Parameter poolName is required and cannot be null."));
}
if (volumeName == null) {
return Mono.error(new IllegalArgumentException("Parameter volumeName is required and cannot be null."));
}
final String accept = "application/json";
return FluxUtil
.withContext(
context -> service.populateAvailabilityZone(this.client.getEndpoint(), this.client.getSubscriptionId(),
resourceGroupName, accountName, poolName, volumeName, this.client.getApiVersion(), accept, context))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* Populate Availability Zone
*
* This operation will populate availability zone information for a volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return volume resource along with {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono>> populateAvailabilityZoneWithResponseAsync(String resourceGroupName,
String accountName, String poolName, String volumeName, Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (accountName == null) {
return Mono.error(new IllegalArgumentException("Parameter accountName is required and cannot be null."));
}
if (poolName == null) {
return Mono.error(new IllegalArgumentException("Parameter poolName is required and cannot be null."));
}
if (volumeName == null) {
return Mono.error(new IllegalArgumentException("Parameter volumeName is required and cannot be null."));
}
final String accept = "application/json";
context = this.client.mergeContext(context);
return service.populateAvailabilityZone(this.client.getEndpoint(), this.client.getSubscriptionId(),
resourceGroupName, accountName, poolName, volumeName, this.client.getApiVersion(), accept, context);
}
/**
* Populate Availability Zone
*
* This operation will populate availability zone information for a volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of volume resource.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
private PollerFlux, VolumeInner> beginPopulateAvailabilityZoneAsync(
String resourceGroupName, String accountName, String poolName, String volumeName) {
Mono>> mono
= populateAvailabilityZoneWithResponseAsync(resourceGroupName, accountName, poolName, volumeName);
return this.client.getLroResult(mono, this.client.getHttpPipeline(),
VolumeInner.class, VolumeInner.class, this.client.getContext());
}
/**
* Populate Availability Zone
*
* This operation will populate availability zone information for a volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of volume resource.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
private PollerFlux, VolumeInner> beginPopulateAvailabilityZoneAsync(
String resourceGroupName, String accountName, String poolName, String volumeName, Context context) {
context = this.client.mergeContext(context);
Mono>> mono
= populateAvailabilityZoneWithResponseAsync(resourceGroupName, accountName, poolName, volumeName, context);
return this.client.getLroResult(mono, this.client.getHttpPipeline(),
VolumeInner.class, VolumeInner.class, context);
}
/**
* Populate Availability Zone
*
* This operation will populate availability zone information for a volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of volume resource.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, VolumeInner> beginPopulateAvailabilityZone(String resourceGroupName,
String accountName, String poolName, String volumeName) {
return this.beginPopulateAvailabilityZoneAsync(resourceGroupName, accountName, poolName, volumeName)
.getSyncPoller();
}
/**
* Populate Availability Zone
*
* This operation will populate availability zone information for a volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of volume resource.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, VolumeInner> beginPopulateAvailabilityZone(String resourceGroupName,
String accountName, String poolName, String volumeName, Context context) {
return this.beginPopulateAvailabilityZoneAsync(resourceGroupName, accountName, poolName, volumeName, context)
.getSyncPoller();
}
/**
* Populate Availability Zone
*
* This operation will populate availability zone information for a volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return volume resource on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono populateAvailabilityZoneAsync(String resourceGroupName, String accountName,
String poolName, String volumeName) {
return beginPopulateAvailabilityZoneAsync(resourceGroupName, accountName, poolName, volumeName).last()
.flatMap(this.client::getLroFinalResultOrError);
}
/**
* Populate Availability Zone
*
* This operation will populate availability zone information for a volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return volume resource on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono populateAvailabilityZoneAsync(String resourceGroupName, String accountName,
String poolName, String volumeName, Context context) {
return beginPopulateAvailabilityZoneAsync(resourceGroupName, accountName, poolName, volumeName, context).last()
.flatMap(this.client::getLroFinalResultOrError);
}
/**
* Populate Availability Zone
*
* This operation will populate availability zone information for a volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return volume resource.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public VolumeInner populateAvailabilityZone(String resourceGroupName, String accountName, String poolName,
String volumeName) {
return populateAvailabilityZoneAsync(resourceGroupName, accountName, poolName, volumeName).block();
}
/**
* Populate Availability Zone
*
* This operation will populate availability zone information for a volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return volume resource.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public VolumeInner populateAvailabilityZone(String resourceGroupName, String accountName, String poolName,
String volumeName, Context context) {
return populateAvailabilityZoneAsync(resourceGroupName, accountName, poolName, volumeName, context).block();
}
/**
* Revert a volume to one of its snapshots
*
* Revert a volume to the snapshot specified in the body.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param body Object for snapshot to revert supplied in the body of the operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono>> revertWithResponseAsync(String resourceGroupName, String accountName,
String poolName, String volumeName, VolumeRevert body) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (accountName == null) {
return Mono.error(new IllegalArgumentException("Parameter accountName is required and cannot be null."));
}
if (poolName == null) {
return Mono.error(new IllegalArgumentException("Parameter poolName is required and cannot be null."));
}
if (volumeName == null) {
return Mono.error(new IllegalArgumentException("Parameter volumeName is required and cannot be null."));
}
if (body == null) {
return Mono.error(new IllegalArgumentException("Parameter body is required and cannot be null."));
} else {
body.validate();
}
final String accept = "application/json";
return FluxUtil
.withContext(
context -> service.revert(this.client.getEndpoint(), this.client.getSubscriptionId(), resourceGroupName,
accountName, poolName, volumeName, this.client.getApiVersion(), body, accept, context))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* Revert a volume to one of its snapshots
*
* Revert a volume to the snapshot specified in the body.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param body Object for snapshot to revert supplied in the body of the operation.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono>> revertWithResponseAsync(String resourceGroupName, String accountName,
String poolName, String volumeName, VolumeRevert body, Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (accountName == null) {
return Mono.error(new IllegalArgumentException("Parameter accountName is required and cannot be null."));
}
if (poolName == null) {
return Mono.error(new IllegalArgumentException("Parameter poolName is required and cannot be null."));
}
if (volumeName == null) {
return Mono.error(new IllegalArgumentException("Parameter volumeName is required and cannot be null."));
}
if (body == null) {
return Mono.error(new IllegalArgumentException("Parameter body is required and cannot be null."));
} else {
body.validate();
}
final String accept = "application/json";
context = this.client.mergeContext(context);
return service.revert(this.client.getEndpoint(), this.client.getSubscriptionId(), resourceGroupName,
accountName, poolName, volumeName, this.client.getApiVersion(), body, accept, context);
}
/**
* Revert a volume to one of its snapshots
*
* Revert a volume to the snapshot specified in the body.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param body Object for snapshot to revert supplied in the body of the operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
private PollerFlux, Void> beginRevertAsync(String resourceGroupName, String accountName,
String poolName, String volumeName, VolumeRevert body) {
Mono>> mono
= revertWithResponseAsync(resourceGroupName, accountName, poolName, volumeName, body);
return this.client.getLroResult(mono, this.client.getHttpPipeline(), Void.class, Void.class,
this.client.getContext());
}
/**
* Revert a volume to one of its snapshots
*
* Revert a volume to the snapshot specified in the body.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param body Object for snapshot to revert supplied in the body of the operation.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
private PollerFlux, Void> beginRevertAsync(String resourceGroupName, String accountName,
String poolName, String volumeName, VolumeRevert body, Context context) {
context = this.client.mergeContext(context);
Mono>> mono
= revertWithResponseAsync(resourceGroupName, accountName, poolName, volumeName, body, context);
return this.client.getLroResult(mono, this.client.getHttpPipeline(), Void.class, Void.class,
context);
}
/**
* Revert a volume to one of its snapshots
*
* Revert a volume to the snapshot specified in the body.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param body Object for snapshot to revert supplied in the body of the operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, Void> beginRevert(String resourceGroupName, String accountName, String poolName,
String volumeName, VolumeRevert body) {
return this.beginRevertAsync(resourceGroupName, accountName, poolName, volumeName, body).getSyncPoller();
}
/**
* Revert a volume to one of its snapshots
*
* Revert a volume to the snapshot specified in the body.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param body Object for snapshot to revert supplied in the body of the operation.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, Void> beginRevert(String resourceGroupName, String accountName, String poolName,
String volumeName, VolumeRevert body, Context context) {
return this.beginRevertAsync(resourceGroupName, accountName, poolName, volumeName, body, context)
.getSyncPoller();
}
/**
* Revert a volume to one of its snapshots
*
* Revert a volume to the snapshot specified in the body.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param body Object for snapshot to revert supplied in the body of the operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono revertAsync(String resourceGroupName, String accountName, String poolName, String volumeName,
VolumeRevert body) {
return beginRevertAsync(resourceGroupName, accountName, poolName, volumeName, body).last()
.flatMap(this.client::getLroFinalResultOrError);
}
/**
* Revert a volume to one of its snapshots
*
* Revert a volume to the snapshot specified in the body.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param body Object for snapshot to revert supplied in the body of the operation.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono revertAsync(String resourceGroupName, String accountName, String poolName, String volumeName,
VolumeRevert body, Context context) {
return beginRevertAsync(resourceGroupName, accountName, poolName, volumeName, body, context).last()
.flatMap(this.client::getLroFinalResultOrError);
}
/**
* Revert a volume to one of its snapshots
*
* Revert a volume to the snapshot specified in the body.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param body Object for snapshot to revert supplied in the body of the operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public void revert(String resourceGroupName, String accountName, String poolName, String volumeName,
VolumeRevert body) {
revertAsync(resourceGroupName, accountName, poolName, volumeName, body).block();
}
/**
* Revert a volume to one of its snapshots
*
* Revert a volume to the snapshot specified in the body.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param body Object for snapshot to revert supplied in the body of the operation.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public void revert(String resourceGroupName, String accountName, String poolName, String volumeName,
VolumeRevert body, Context context) {
revertAsync(resourceGroupName, accountName, poolName, volumeName, body, context).block();
}
/**
* Reset cifs password
*
* Reset cifs password from volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono>> resetCifsPasswordWithResponseAsync(String resourceGroupName,
String accountName, String poolName, String volumeName) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (accountName == null) {
return Mono.error(new IllegalArgumentException("Parameter accountName is required and cannot be null."));
}
if (poolName == null) {
return Mono.error(new IllegalArgumentException("Parameter poolName is required and cannot be null."));
}
if (volumeName == null) {
return Mono.error(new IllegalArgumentException("Parameter volumeName is required and cannot be null."));
}
final String accept = "application/json";
return FluxUtil
.withContext(
context -> service.resetCifsPassword(this.client.getEndpoint(), this.client.getSubscriptionId(),
resourceGroupName, accountName, poolName, volumeName, this.client.getApiVersion(), accept, context))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* Reset cifs password
*
* Reset cifs password from volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono>> resetCifsPasswordWithResponseAsync(String resourceGroupName,
String accountName, String poolName, String volumeName, Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (accountName == null) {
return Mono.error(new IllegalArgumentException("Parameter accountName is required and cannot be null."));
}
if (poolName == null) {
return Mono.error(new IllegalArgumentException("Parameter poolName is required and cannot be null."));
}
if (volumeName == null) {
return Mono.error(new IllegalArgumentException("Parameter volumeName is required and cannot be null."));
}
final String accept = "application/json";
context = this.client.mergeContext(context);
return service.resetCifsPassword(this.client.getEndpoint(), this.client.getSubscriptionId(), resourceGroupName,
accountName, poolName, volumeName, this.client.getApiVersion(), accept, context);
}
/**
* Reset cifs password
*
* Reset cifs password from volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
private PollerFlux, Void> beginResetCifsPasswordAsync(String resourceGroupName, String accountName,
String poolName, String volumeName) {
Mono>> mono
= resetCifsPasswordWithResponseAsync(resourceGroupName, accountName, poolName, volumeName);
return this.client.getLroResult(mono, this.client.getHttpPipeline(), Void.class, Void.class,
this.client.getContext());
}
/**
* Reset cifs password
*
* Reset cifs password from volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
private PollerFlux, Void> beginResetCifsPasswordAsync(String resourceGroupName, String accountName,
String poolName, String volumeName, Context context) {
context = this.client.mergeContext(context);
Mono>> mono
= resetCifsPasswordWithResponseAsync(resourceGroupName, accountName, poolName, volumeName, context);
return this.client.getLroResult(mono, this.client.getHttpPipeline(), Void.class, Void.class,
context);
}
/**
* Reset cifs password
*
* Reset cifs password from volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, Void> beginResetCifsPassword(String resourceGroupName, String accountName,
String poolName, String volumeName) {
return this.beginResetCifsPasswordAsync(resourceGroupName, accountName, poolName, volumeName).getSyncPoller();
}
/**
* Reset cifs password
*
* Reset cifs password from volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, Void> beginResetCifsPassword(String resourceGroupName, String accountName,
String poolName, String volumeName, Context context) {
return this.beginResetCifsPasswordAsync(resourceGroupName, accountName, poolName, volumeName, context)
.getSyncPoller();
}
/**
* Reset cifs password
*
* Reset cifs password from volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono resetCifsPasswordAsync(String resourceGroupName, String accountName, String poolName,
String volumeName) {
return beginResetCifsPasswordAsync(resourceGroupName, accountName, poolName, volumeName).last()
.flatMap(this.client::getLroFinalResultOrError);
}
/**
* Reset cifs password
*
* Reset cifs password from volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono resetCifsPasswordAsync(String resourceGroupName, String accountName, String poolName,
String volumeName, Context context) {
return beginResetCifsPasswordAsync(resourceGroupName, accountName, poolName, volumeName, context).last()
.flatMap(this.client::getLroFinalResultOrError);
}
/**
* Reset cifs password
*
* Reset cifs password from volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public void resetCifsPassword(String resourceGroupName, String accountName, String poolName, String volumeName) {
resetCifsPasswordAsync(resourceGroupName, accountName, poolName, volumeName).block();
}
/**
* Reset cifs password
*
* Reset cifs password from volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public void resetCifsPassword(String resourceGroupName, String accountName, String poolName, String volumeName,
Context context) {
resetCifsPasswordAsync(resourceGroupName, accountName, poolName, volumeName, context).block();
}
/**
* Split clone from parent volume
*
* Split operation to convert clone volume to an independent volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono>> splitCloneFromParentWithResponseAsync(String resourceGroupName,
String accountName, String poolName, String volumeName) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (accountName == null) {
return Mono.error(new IllegalArgumentException("Parameter accountName is required and cannot be null."));
}
if (poolName == null) {
return Mono.error(new IllegalArgumentException("Parameter poolName is required and cannot be null."));
}
if (volumeName == null) {
return Mono.error(new IllegalArgumentException("Parameter volumeName is required and cannot be null."));
}
final String accept = "application/json";
return FluxUtil
.withContext(
context -> service.splitCloneFromParent(this.client.getEndpoint(), this.client.getSubscriptionId(),
resourceGroupName, accountName, poolName, volumeName, this.client.getApiVersion(), accept, context))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* Split clone from parent volume
*
* Split operation to convert clone volume to an independent volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono>> splitCloneFromParentWithResponseAsync(String resourceGroupName,
String accountName, String poolName, String volumeName, Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (accountName == null) {
return Mono.error(new IllegalArgumentException("Parameter accountName is required and cannot be null."));
}
if (poolName == null) {
return Mono.error(new IllegalArgumentException("Parameter poolName is required and cannot be null."));
}
if (volumeName == null) {
return Mono.error(new IllegalArgumentException("Parameter volumeName is required and cannot be null."));
}
final String accept = "application/json";
context = this.client.mergeContext(context);
return service.splitCloneFromParent(this.client.getEndpoint(), this.client.getSubscriptionId(),
resourceGroupName, accountName, poolName, volumeName, this.client.getApiVersion(), accept, context);
}
/**
* Split clone from parent volume
*
* Split operation to convert clone volume to an independent volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
private PollerFlux, Void> beginSplitCloneFromParentAsync(String resourceGroupName,
String accountName, String poolName, String volumeName) {
Mono>> mono
= splitCloneFromParentWithResponseAsync(resourceGroupName, accountName, poolName, volumeName);
return this.client.getLroResult(mono, this.client.getHttpPipeline(), Void.class, Void.class,
this.client.getContext());
}
/**
* Split clone from parent volume
*
* Split operation to convert clone volume to an independent volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
private PollerFlux, Void> beginSplitCloneFromParentAsync(String resourceGroupName,
String accountName, String poolName, String volumeName, Context context) {
context = this.client.mergeContext(context);
Mono>> mono
= splitCloneFromParentWithResponseAsync(resourceGroupName, accountName, poolName, volumeName, context);
return this.client.getLroResult(mono, this.client.getHttpPipeline(), Void.class, Void.class,
context);
}
/**
* Split clone from parent volume
*
* Split operation to convert clone volume to an independent volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, Void> beginSplitCloneFromParent(String resourceGroupName, String accountName,
String poolName, String volumeName) {
return this.beginSplitCloneFromParentAsync(resourceGroupName, accountName, poolName, volumeName)
.getSyncPoller();
}
/**
* Split clone from parent volume
*
* Split operation to convert clone volume to an independent volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, Void> beginSplitCloneFromParent(String resourceGroupName, String accountName,
String poolName, String volumeName, Context context) {
return this.beginSplitCloneFromParentAsync(resourceGroupName, accountName, poolName, volumeName, context)
.getSyncPoller();
}
/**
* Split clone from parent volume
*
* Split operation to convert clone volume to an independent volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono splitCloneFromParentAsync(String resourceGroupName, String accountName, String poolName,
String volumeName) {
return beginSplitCloneFromParentAsync(resourceGroupName, accountName, poolName, volumeName).last()
.flatMap(this.client::getLroFinalResultOrError);
}
/**
* Split clone from parent volume
*
* Split operation to convert clone volume to an independent volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono splitCloneFromParentAsync(String resourceGroupName, String accountName, String poolName,
String volumeName, Context context) {
return beginSplitCloneFromParentAsync(resourceGroupName, accountName, poolName, volumeName, context).last()
.flatMap(this.client::getLroFinalResultOrError);
}
/**
* Split clone from parent volume
*
* Split operation to convert clone volume to an independent volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public void splitCloneFromParent(String resourceGroupName, String accountName, String poolName, String volumeName) {
splitCloneFromParentAsync(resourceGroupName, accountName, poolName, volumeName).block();
}
/**
* Split clone from parent volume
*
* Split operation to convert clone volume to an independent volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public void splitCloneFromParent(String resourceGroupName, String accountName, String poolName, String volumeName,
Context context) {
splitCloneFromParentAsync(resourceGroupName, accountName, poolName, volumeName, context).block();
}
/**
* Break file locks
*
* Break all the file locks on a volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param body Optional body to provide the ability to clear file locks with selected options.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono>> breakFileLocksWithResponseAsync(String resourceGroupName,
String accountName, String poolName, String volumeName, BreakFileLocksRequest body) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (accountName == null) {
return Mono.error(new IllegalArgumentException("Parameter accountName is required and cannot be null."));
}
if (poolName == null) {
return Mono.error(new IllegalArgumentException("Parameter poolName is required and cannot be null."));
}
if (volumeName == null) {
return Mono.error(new IllegalArgumentException("Parameter volumeName is required and cannot be null."));
}
if (body != null) {
body.validate();
}
final String accept = "application/json";
return FluxUtil
.withContext(context -> service.breakFileLocks(this.client.getEndpoint(), this.client.getSubscriptionId(),
resourceGroupName, accountName, poolName, volumeName, this.client.getApiVersion(), body, accept,
context))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* Break file locks
*
* Break all the file locks on a volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param body Optional body to provide the ability to clear file locks with selected options.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono>> breakFileLocksWithResponseAsync(String resourceGroupName,
String accountName, String poolName, String volumeName, BreakFileLocksRequest body, Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (accountName == null) {
return Mono.error(new IllegalArgumentException("Parameter accountName is required and cannot be null."));
}
if (poolName == null) {
return Mono.error(new IllegalArgumentException("Parameter poolName is required and cannot be null."));
}
if (volumeName == null) {
return Mono.error(new IllegalArgumentException("Parameter volumeName is required and cannot be null."));
}
if (body != null) {
body.validate();
}
final String accept = "application/json";
context = this.client.mergeContext(context);
return service.breakFileLocks(this.client.getEndpoint(), this.client.getSubscriptionId(), resourceGroupName,
accountName, poolName, volumeName, this.client.getApiVersion(), body, accept, context);
}
/**
* Break file locks
*
* Break all the file locks on a volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param body Optional body to provide the ability to clear file locks with selected options.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
private PollerFlux, Void> beginBreakFileLocksAsync(String resourceGroupName, String accountName,
String poolName, String volumeName, BreakFileLocksRequest body) {
Mono>> mono
= breakFileLocksWithResponseAsync(resourceGroupName, accountName, poolName, volumeName, body);
return this.client.getLroResult(mono, this.client.getHttpPipeline(), Void.class, Void.class,
this.client.getContext());
}
/**
* Break file locks
*
* Break all the file locks on a volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
private PollerFlux, Void> beginBreakFileLocksAsync(String resourceGroupName, String accountName,
String poolName, String volumeName) {
final BreakFileLocksRequest body = null;
Mono>> mono
= breakFileLocksWithResponseAsync(resourceGroupName, accountName, poolName, volumeName, body);
return this.client.getLroResult(mono, this.client.getHttpPipeline(), Void.class, Void.class,
this.client.getContext());
}
/**
* Break file locks
*
* Break all the file locks on a volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param body Optional body to provide the ability to clear file locks with selected options.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
private PollerFlux, Void> beginBreakFileLocksAsync(String resourceGroupName, String accountName,
String poolName, String volumeName, BreakFileLocksRequest body, Context context) {
context = this.client.mergeContext(context);
Mono>> mono
= breakFileLocksWithResponseAsync(resourceGroupName, accountName, poolName, volumeName, body, context);
return this.client.getLroResult(mono, this.client.getHttpPipeline(), Void.class, Void.class,
context);
}
/**
* Break file locks
*
* Break all the file locks on a volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, Void> beginBreakFileLocks(String resourceGroupName, String accountName,
String poolName, String volumeName) {
final BreakFileLocksRequest body = null;
return this.beginBreakFileLocksAsync(resourceGroupName, accountName, poolName, volumeName, body)
.getSyncPoller();
}
/**
* Break file locks
*
* Break all the file locks on a volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param body Optional body to provide the ability to clear file locks with selected options.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, Void> beginBreakFileLocks(String resourceGroupName, String accountName,
String poolName, String volumeName, BreakFileLocksRequest body, Context context) {
return this.beginBreakFileLocksAsync(resourceGroupName, accountName, poolName, volumeName, body, context)
.getSyncPoller();
}
/**
* Break file locks
*
* Break all the file locks on a volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param body Optional body to provide the ability to clear file locks with selected options.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono breakFileLocksAsync(String resourceGroupName, String accountName, String poolName,
String volumeName, BreakFileLocksRequest body) {
return beginBreakFileLocksAsync(resourceGroupName, accountName, poolName, volumeName, body).last()
.flatMap(this.client::getLroFinalResultOrError);
}
/**
* Break file locks
*
* Break all the file locks on a volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono breakFileLocksAsync(String resourceGroupName, String accountName, String poolName,
String volumeName) {
final BreakFileLocksRequest body = null;
return beginBreakFileLocksAsync(resourceGroupName, accountName, poolName, volumeName, body).last()
.flatMap(this.client::getLroFinalResultOrError);
}
/**
* Break file locks
*
* Break all the file locks on a volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param body Optional body to provide the ability to clear file locks with selected options.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono breakFileLocksAsync(String resourceGroupName, String accountName, String poolName,
String volumeName, BreakFileLocksRequest body, Context context) {
return beginBreakFileLocksAsync(resourceGroupName, accountName, poolName, volumeName, body, context).last()
.flatMap(this.client::getLroFinalResultOrError);
}
/**
* Break file locks
*
* Break all the file locks on a volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public void breakFileLocks(String resourceGroupName, String accountName, String poolName, String volumeName) {
final BreakFileLocksRequest body = null;
breakFileLocksAsync(resourceGroupName, accountName, poolName, volumeName, body).block();
}
/**
* Break file locks
*
* Break all the file locks on a volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param body Optional body to provide the ability to clear file locks with selected options.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public void breakFileLocks(String resourceGroupName, String accountName, String poolName, String volumeName,
BreakFileLocksRequest body, Context context) {
breakFileLocksAsync(resourceGroupName, accountName, poolName, volumeName, body, context).block();
}
/**
* Get Group Id List for LDAP User
*
* Returns the list of group Ids for a specific LDAP User.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param body Returns group Id list for a specific LDAP user.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return group Id list for Ldap user along with {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono>> listGetGroupIdListForLdapUserWithResponseAsync(String resourceGroupName,
String accountName, String poolName, String volumeName, GetGroupIdListForLdapUserRequest body) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (accountName == null) {
return Mono.error(new IllegalArgumentException("Parameter accountName is required and cannot be null."));
}
if (poolName == null) {
return Mono.error(new IllegalArgumentException("Parameter poolName is required and cannot be null."));
}
if (volumeName == null) {
return Mono.error(new IllegalArgumentException("Parameter volumeName is required and cannot be null."));
}
if (body == null) {
return Mono.error(new IllegalArgumentException("Parameter body is required and cannot be null."));
} else {
body.validate();
}
final String accept = "application/json";
return FluxUtil
.withContext(context -> service.listGetGroupIdListForLdapUser(this.client.getEndpoint(),
this.client.getSubscriptionId(), resourceGroupName, accountName, poolName, volumeName,
this.client.getApiVersion(), body, accept, context))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* Get Group Id List for LDAP User
*
* Returns the list of group Ids for a specific LDAP User.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param body Returns group Id list for a specific LDAP user.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return group Id list for Ldap user along with {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono>> listGetGroupIdListForLdapUserWithResponseAsync(String resourceGroupName,
String accountName, String poolName, String volumeName, GetGroupIdListForLdapUserRequest body,
Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (accountName == null) {
return Mono.error(new IllegalArgumentException("Parameter accountName is required and cannot be null."));
}
if (poolName == null) {
return Mono.error(new IllegalArgumentException("Parameter poolName is required and cannot be null."));
}
if (volumeName == null) {
return Mono.error(new IllegalArgumentException("Parameter volumeName is required and cannot be null."));
}
if (body == null) {
return Mono.error(new IllegalArgumentException("Parameter body is required and cannot be null."));
} else {
body.validate();
}
final String accept = "application/json";
context = this.client.mergeContext(context);
return service.listGetGroupIdListForLdapUser(this.client.getEndpoint(), this.client.getSubscriptionId(),
resourceGroupName, accountName, poolName, volumeName, this.client.getApiVersion(), body, accept, context);
}
/**
* Get Group Id List for LDAP User
*
* Returns the list of group Ids for a specific LDAP User.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param body Returns group Id list for a specific LDAP user.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of group Id list for Ldap user.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
private PollerFlux, GetGroupIdListForLdapUserResponseInner>
beginListGetGroupIdListForLdapUserAsync(String resourceGroupName, String accountName, String poolName,
String volumeName, GetGroupIdListForLdapUserRequest body) {
Mono>> mono = listGetGroupIdListForLdapUserWithResponseAsync(resourceGroupName,
accountName, poolName, volumeName, body);
return this.client.getLroResult(
mono, this.client.getHttpPipeline(), GetGroupIdListForLdapUserResponseInner.class,
GetGroupIdListForLdapUserResponseInner.class, this.client.getContext());
}
/**
* Get Group Id List for LDAP User
*
* Returns the list of group Ids for a specific LDAP User.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param body Returns group Id list for a specific LDAP user.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of group Id list for Ldap user.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
private PollerFlux, GetGroupIdListForLdapUserResponseInner>
beginListGetGroupIdListForLdapUserAsync(String resourceGroupName, String accountName, String poolName,
String volumeName, GetGroupIdListForLdapUserRequest body, Context context) {
context = this.client.mergeContext(context);
Mono>> mono = listGetGroupIdListForLdapUserWithResponseAsync(resourceGroupName,
accountName, poolName, volumeName, body, context);
return this.client.getLroResult(
mono, this.client.getHttpPipeline(), GetGroupIdListForLdapUserResponseInner.class,
GetGroupIdListForLdapUserResponseInner.class, context);
}
/**
* Get Group Id List for LDAP User
*
* Returns the list of group Ids for a specific LDAP User.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param body Returns group Id list for a specific LDAP user.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of group Id list for Ldap user.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, GetGroupIdListForLdapUserResponseInner>
beginListGetGroupIdListForLdapUser(String resourceGroupName, String accountName, String poolName,
String volumeName, GetGroupIdListForLdapUserRequest body) {
return this.beginListGetGroupIdListForLdapUserAsync(resourceGroupName, accountName, poolName, volumeName, body)
.getSyncPoller();
}
/**
* Get Group Id List for LDAP User
*
* Returns the list of group Ids for a specific LDAP User.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param body Returns group Id list for a specific LDAP user.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of group Id list for Ldap user.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, GetGroupIdListForLdapUserResponseInner>
beginListGetGroupIdListForLdapUser(String resourceGroupName, String accountName, String poolName,
String volumeName, GetGroupIdListForLdapUserRequest body, Context context) {
return this
.beginListGetGroupIdListForLdapUserAsync(resourceGroupName, accountName, poolName, volumeName, body,
context)
.getSyncPoller();
}
/**
* Get Group Id List for LDAP User
*
* Returns the list of group Ids for a specific LDAP User.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param body Returns group Id list for a specific LDAP user.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return group Id list for Ldap user on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono listGetGroupIdListForLdapUserAsync(String resourceGroupName,
String accountName, String poolName, String volumeName, GetGroupIdListForLdapUserRequest body) {
return beginListGetGroupIdListForLdapUserAsync(resourceGroupName, accountName, poolName, volumeName, body)
.last()
.flatMap(this.client::getLroFinalResultOrError);
}
/**
* Get Group Id List for LDAP User
*
* Returns the list of group Ids for a specific LDAP User.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param body Returns group Id list for a specific LDAP user.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return group Id list for Ldap user on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono listGetGroupIdListForLdapUserAsync(String resourceGroupName,
String accountName, String poolName, String volumeName, GetGroupIdListForLdapUserRequest body,
Context context) {
return beginListGetGroupIdListForLdapUserAsync(resourceGroupName, accountName, poolName, volumeName, body,
context).last().flatMap(this.client::getLroFinalResultOrError);
}
/**
* Get Group Id List for LDAP User
*
* Returns the list of group Ids for a specific LDAP User.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param body Returns group Id list for a specific LDAP user.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return group Id list for Ldap user.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public GetGroupIdListForLdapUserResponseInner listGetGroupIdListForLdapUser(String resourceGroupName,
String accountName, String poolName, String volumeName, GetGroupIdListForLdapUserRequest body) {
return listGetGroupIdListForLdapUserAsync(resourceGroupName, accountName, poolName, volumeName, body).block();
}
/**
* Get Group Id List for LDAP User
*
* Returns the list of group Ids for a specific LDAP User.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param body Returns group Id list for a specific LDAP user.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return group Id list for Ldap user.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public GetGroupIdListForLdapUserResponseInner listGetGroupIdListForLdapUser(String resourceGroupName,
String accountName, String poolName, String volumeName, GetGroupIdListForLdapUserRequest body,
Context context) {
return listGetGroupIdListForLdapUserAsync(resourceGroupName, accountName, poolName, volumeName, body, context)
.block();
}
/**
* Lists Quota Report for the volume
*
* Returns report of quotas for the volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return quota Report for volume along with {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono>> listQuotaReportWithResponseAsync(String resourceGroupName,
String accountName, String poolName, String volumeName) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (accountName == null) {
return Mono.error(new IllegalArgumentException("Parameter accountName is required and cannot be null."));
}
if (poolName == null) {
return Mono.error(new IllegalArgumentException("Parameter poolName is required and cannot be null."));
}
if (volumeName == null) {
return Mono.error(new IllegalArgumentException("Parameter volumeName is required and cannot be null."));
}
final String accept = "application/json";
return FluxUtil
.withContext(context -> service.listQuotaReport(this.client.getEndpoint(), this.client.getSubscriptionId(),
resourceGroupName, accountName, poolName, volumeName, this.client.getApiVersion(), accept, context))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* Lists Quota Report for the volume
*
* Returns report of quotas for the volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return quota Report for volume along with {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono>> listQuotaReportWithResponseAsync(String resourceGroupName,
String accountName, String poolName, String volumeName, Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (accountName == null) {
return Mono.error(new IllegalArgumentException("Parameter accountName is required and cannot be null."));
}
if (poolName == null) {
return Mono.error(new IllegalArgumentException("Parameter poolName is required and cannot be null."));
}
if (volumeName == null) {
return Mono.error(new IllegalArgumentException("Parameter volumeName is required and cannot be null."));
}
final String accept = "application/json";
context = this.client.mergeContext(context);
return service.listQuotaReport(this.client.getEndpoint(), this.client.getSubscriptionId(), resourceGroupName,
accountName, poolName, volumeName, this.client.getApiVersion(), accept, context);
}
/**
* Lists Quota Report for the volume
*
* Returns report of quotas for the volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of quota Report for volume.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
private PollerFlux, ListQuotaReportResponseInner>
beginListQuotaReportAsync(String resourceGroupName, String accountName, String poolName, String volumeName) {
Mono>> mono
= listQuotaReportWithResponseAsync(resourceGroupName, accountName, poolName, volumeName);
return this.client.getLroResult(mono,
this.client.getHttpPipeline(), ListQuotaReportResponseInner.class, ListQuotaReportResponseInner.class,
this.client.getContext());
}
/**
* Lists Quota Report for the volume
*
* Returns report of quotas for the volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of quota Report for volume.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
private PollerFlux, ListQuotaReportResponseInner>
beginListQuotaReportAsync(String resourceGroupName, String accountName, String poolName, String volumeName,
Context context) {
context = this.client.mergeContext(context);
Mono>> mono
= listQuotaReportWithResponseAsync(resourceGroupName, accountName, poolName, volumeName, context);
return this.client.getLroResult(mono,
this.client.getHttpPipeline(), ListQuotaReportResponseInner.class, ListQuotaReportResponseInner.class,
context);
}
/**
* Lists Quota Report for the volume
*
* Returns report of quotas for the volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of quota Report for volume.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, ListQuotaReportResponseInner>
beginListQuotaReport(String resourceGroupName, String accountName, String poolName, String volumeName) {
return this.beginListQuotaReportAsync(resourceGroupName, accountName, poolName, volumeName).getSyncPoller();
}
/**
* Lists Quota Report for the volume
*
* Returns report of quotas for the volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of quota Report for volume.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, ListQuotaReportResponseInner> beginListQuotaReport(
String resourceGroupName, String accountName, String poolName, String volumeName, Context context) {
return this.beginListQuotaReportAsync(resourceGroupName, accountName, poolName, volumeName, context)
.getSyncPoller();
}
/**
* Lists Quota Report for the volume
*
* Returns report of quotas for the volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return quota Report for volume on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono listQuotaReportAsync(String resourceGroupName, String accountName,
String poolName, String volumeName) {
return beginListQuotaReportAsync(resourceGroupName, accountName, poolName, volumeName).last()
.flatMap(this.client::getLroFinalResultOrError);
}
/**
* Lists Quota Report for the volume
*
* Returns report of quotas for the volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return quota Report for volume on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono listQuotaReportAsync(String resourceGroupName, String accountName,
String poolName, String volumeName, Context context) {
return beginListQuotaReportAsync(resourceGroupName, accountName, poolName, volumeName, context).last()
.flatMap(this.client::getLroFinalResultOrError);
}
/**
* Lists Quota Report for the volume
*
* Returns report of quotas for the volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return quota Report for volume.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public ListQuotaReportResponseInner listQuotaReport(String resourceGroupName, String accountName, String poolName,
String volumeName) {
return listQuotaReportAsync(resourceGroupName, accountName, poolName, volumeName).block();
}
/**
* Lists Quota Report for the volume
*
* Returns report of quotas for the volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return quota Report for volume.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public ListQuotaReportResponseInner listQuotaReport(String resourceGroupName, String accountName, String poolName,
String volumeName, Context context) {
return listQuotaReportAsync(resourceGroupName, accountName, poolName, volumeName, context).block();
}
/**
* Break volume replication
*
* Break the replication connection on the destination volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param body Optional body to force break the replication.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono>> breakReplicationWithResponseAsync(String resourceGroupName,
String accountName, String poolName, String volumeName, BreakReplicationRequest body) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (accountName == null) {
return Mono.error(new IllegalArgumentException("Parameter accountName is required and cannot be null."));
}
if (poolName == null) {
return Mono.error(new IllegalArgumentException("Parameter poolName is required and cannot be null."));
}
if (volumeName == null) {
return Mono.error(new IllegalArgumentException("Parameter volumeName is required and cannot be null."));
}
if (body != null) {
body.validate();
}
final String accept = "application/json";
return FluxUtil
.withContext(context -> service.breakReplication(this.client.getEndpoint(), this.client.getSubscriptionId(),
resourceGroupName, accountName, poolName, volumeName, this.client.getApiVersion(), body, accept,
context))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* Break volume replication
*
* Break the replication connection on the destination volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param body Optional body to force break the replication.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono>> breakReplicationWithResponseAsync(String resourceGroupName,
String accountName, String poolName, String volumeName, BreakReplicationRequest body, Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (accountName == null) {
return Mono.error(new IllegalArgumentException("Parameter accountName is required and cannot be null."));
}
if (poolName == null) {
return Mono.error(new IllegalArgumentException("Parameter poolName is required and cannot be null."));
}
if (volumeName == null) {
return Mono.error(new IllegalArgumentException("Parameter volumeName is required and cannot be null."));
}
if (body != null) {
body.validate();
}
final String accept = "application/json";
context = this.client.mergeContext(context);
return service.breakReplication(this.client.getEndpoint(), this.client.getSubscriptionId(), resourceGroupName,
accountName, poolName, volumeName, this.client.getApiVersion(), body, accept, context);
}
/**
* Break volume replication
*
* Break the replication connection on the destination volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param body Optional body to force break the replication.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
private PollerFlux, Void> beginBreakReplicationAsync(String resourceGroupName, String accountName,
String poolName, String volumeName, BreakReplicationRequest body) {
Mono>> mono
= breakReplicationWithResponseAsync(resourceGroupName, accountName, poolName, volumeName, body);
return this.client.getLroResult(mono, this.client.getHttpPipeline(), Void.class, Void.class,
this.client.getContext());
}
/**
* Break volume replication
*
* Break the replication connection on the destination volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
private PollerFlux, Void> beginBreakReplicationAsync(String resourceGroupName, String accountName,
String poolName, String volumeName) {
final BreakReplicationRequest body = null;
Mono>> mono
= breakReplicationWithResponseAsync(resourceGroupName, accountName, poolName, volumeName, body);
return this.client.getLroResult(mono, this.client.getHttpPipeline(), Void.class, Void.class,
this.client.getContext());
}
/**
* Break volume replication
*
* Break the replication connection on the destination volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param body Optional body to force break the replication.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
private PollerFlux, Void> beginBreakReplicationAsync(String resourceGroupName, String accountName,
String poolName, String volumeName, BreakReplicationRequest body, Context context) {
context = this.client.mergeContext(context);
Mono>> mono
= breakReplicationWithResponseAsync(resourceGroupName, accountName, poolName, volumeName, body, context);
return this.client.getLroResult(mono, this.client.getHttpPipeline(), Void.class, Void.class,
context);
}
/**
* Break volume replication
*
* Break the replication connection on the destination volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, Void> beginBreakReplication(String resourceGroupName, String accountName,
String poolName, String volumeName) {
final BreakReplicationRequest body = null;
return this.beginBreakReplicationAsync(resourceGroupName, accountName, poolName, volumeName, body)
.getSyncPoller();
}
/**
* Break volume replication
*
* Break the replication connection on the destination volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param body Optional body to force break the replication.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, Void> beginBreakReplication(String resourceGroupName, String accountName,
String poolName, String volumeName, BreakReplicationRequest body, Context context) {
return this.beginBreakReplicationAsync(resourceGroupName, accountName, poolName, volumeName, body, context)
.getSyncPoller();
}
/**
* Break volume replication
*
* Break the replication connection on the destination volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param body Optional body to force break the replication.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono breakReplicationAsync(String resourceGroupName, String accountName, String poolName,
String volumeName, BreakReplicationRequest body) {
return beginBreakReplicationAsync(resourceGroupName, accountName, poolName, volumeName, body).last()
.flatMap(this.client::getLroFinalResultOrError);
}
/**
* Break volume replication
*
* Break the replication connection on the destination volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono breakReplicationAsync(String resourceGroupName, String accountName, String poolName,
String volumeName) {
final BreakReplicationRequest body = null;
return beginBreakReplicationAsync(resourceGroupName, accountName, poolName, volumeName, body).last()
.flatMap(this.client::getLroFinalResultOrError);
}
/**
* Break volume replication
*
* Break the replication connection on the destination volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param body Optional body to force break the replication.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono breakReplicationAsync(String resourceGroupName, String accountName, String poolName,
String volumeName, BreakReplicationRequest body, Context context) {
return beginBreakReplicationAsync(resourceGroupName, accountName, poolName, volumeName, body, context).last()
.flatMap(this.client::getLroFinalResultOrError);
}
/**
* Break volume replication
*
* Break the replication connection on the destination volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public void breakReplication(String resourceGroupName, String accountName, String poolName, String volumeName) {
final BreakReplicationRequest body = null;
breakReplicationAsync(resourceGroupName, accountName, poolName, volumeName, body).block();
}
/**
* Break volume replication
*
* Break the replication connection on the destination volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param body Optional body to force break the replication.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public void breakReplication(String resourceGroupName, String accountName, String poolName, String volumeName,
BreakReplicationRequest body, Context context) {
breakReplicationAsync(resourceGroupName, accountName, poolName, volumeName, body, context).block();
}
/**
* Re-establish volume replication
*
* Re-establish a previously deleted replication between 2 volumes that have a common ad-hoc or policy-based
* snapshots.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param body body for the id of the source volume.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono>> reestablishReplicationWithResponseAsync(String resourceGroupName,
String accountName, String poolName, String volumeName, ReestablishReplicationRequest body) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (accountName == null) {
return Mono.error(new IllegalArgumentException("Parameter accountName is required and cannot be null."));
}
if (poolName == null) {
return Mono.error(new IllegalArgumentException("Parameter poolName is required and cannot be null."));
}
if (volumeName == null) {
return Mono.error(new IllegalArgumentException("Parameter volumeName is required and cannot be null."));
}
if (body == null) {
return Mono.error(new IllegalArgumentException("Parameter body is required and cannot be null."));
} else {
body.validate();
}
final String accept = "application/json";
return FluxUtil
.withContext(context -> service.reestablishReplication(this.client.getEndpoint(),
this.client.getSubscriptionId(), resourceGroupName, accountName, poolName, volumeName,
this.client.getApiVersion(), body, accept, context))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* Re-establish volume replication
*
* Re-establish a previously deleted replication between 2 volumes that have a common ad-hoc or policy-based
* snapshots.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param body body for the id of the source volume.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono>> reestablishReplicationWithResponseAsync(String resourceGroupName,
String accountName, String poolName, String volumeName, ReestablishReplicationRequest body, Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (accountName == null) {
return Mono.error(new IllegalArgumentException("Parameter accountName is required and cannot be null."));
}
if (poolName == null) {
return Mono.error(new IllegalArgumentException("Parameter poolName is required and cannot be null."));
}
if (volumeName == null) {
return Mono.error(new IllegalArgumentException("Parameter volumeName is required and cannot be null."));
}
if (body == null) {
return Mono.error(new IllegalArgumentException("Parameter body is required and cannot be null."));
} else {
body.validate();
}
final String accept = "application/json";
context = this.client.mergeContext(context);
return service.reestablishReplication(this.client.getEndpoint(), this.client.getSubscriptionId(),
resourceGroupName, accountName, poolName, volumeName, this.client.getApiVersion(), body, accept, context);
}
/**
* Re-establish volume replication
*
* Re-establish a previously deleted replication between 2 volumes that have a common ad-hoc or policy-based
* snapshots.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param body body for the id of the source volume.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
private PollerFlux, Void> beginReestablishReplicationAsync(String resourceGroupName,
String accountName, String poolName, String volumeName, ReestablishReplicationRequest body) {
Mono>> mono
= reestablishReplicationWithResponseAsync(resourceGroupName, accountName, poolName, volumeName, body);
return this.client.getLroResult(mono, this.client.getHttpPipeline(), Void.class, Void.class,
this.client.getContext());
}
/**
* Re-establish volume replication
*
* Re-establish a previously deleted replication between 2 volumes that have a common ad-hoc or policy-based
* snapshots.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param body body for the id of the source volume.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
private PollerFlux, Void> beginReestablishReplicationAsync(String resourceGroupName,
String accountName, String poolName, String volumeName, ReestablishReplicationRequest body, Context context) {
context = this.client.mergeContext(context);
Mono>> mono = reestablishReplicationWithResponseAsync(resourceGroupName, accountName,
poolName, volumeName, body, context);
return this.client.getLroResult(mono, this.client.getHttpPipeline(), Void.class, Void.class,
context);
}
/**
* Re-establish volume replication
*
* Re-establish a previously deleted replication between 2 volumes that have a common ad-hoc or policy-based
* snapshots.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param body body for the id of the source volume.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, Void> beginReestablishReplication(String resourceGroupName, String accountName,
String poolName, String volumeName, ReestablishReplicationRequest body) {
return this.beginReestablishReplicationAsync(resourceGroupName, accountName, poolName, volumeName, body)
.getSyncPoller();
}
/**
* Re-establish volume replication
*
* Re-establish a previously deleted replication between 2 volumes that have a common ad-hoc or policy-based
* snapshots.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param body body for the id of the source volume.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, Void> beginReestablishReplication(String resourceGroupName, String accountName,
String poolName, String volumeName, ReestablishReplicationRequest body, Context context) {
return this
.beginReestablishReplicationAsync(resourceGroupName, accountName, poolName, volumeName, body, context)
.getSyncPoller();
}
/**
* Re-establish volume replication
*
* Re-establish a previously deleted replication between 2 volumes that have a common ad-hoc or policy-based
* snapshots.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param body body for the id of the source volume.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono reestablishReplicationAsync(String resourceGroupName, String accountName, String poolName,
String volumeName, ReestablishReplicationRequest body) {
return beginReestablishReplicationAsync(resourceGroupName, accountName, poolName, volumeName, body).last()
.flatMap(this.client::getLroFinalResultOrError);
}
/**
* Re-establish volume replication
*
* Re-establish a previously deleted replication between 2 volumes that have a common ad-hoc or policy-based
* snapshots.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param body body for the id of the source volume.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono reestablishReplicationAsync(String resourceGroupName, String accountName, String poolName,
String volumeName, ReestablishReplicationRequest body, Context context) {
return beginReestablishReplicationAsync(resourceGroupName, accountName, poolName, volumeName, body, context)
.last()
.flatMap(this.client::getLroFinalResultOrError);
}
/**
* Re-establish volume replication
*
* Re-establish a previously deleted replication between 2 volumes that have a common ad-hoc or policy-based
* snapshots.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param body body for the id of the source volume.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public void reestablishReplication(String resourceGroupName, String accountName, String poolName, String volumeName,
ReestablishReplicationRequest body) {
reestablishReplicationAsync(resourceGroupName, accountName, poolName, volumeName, body).block();
}
/**
* Re-establish volume replication
*
* Re-establish a previously deleted replication between 2 volumes that have a common ad-hoc or policy-based
* snapshots.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param body body for the id of the source volume.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public void reestablishReplication(String resourceGroupName, String accountName, String poolName, String volumeName,
ReestablishReplicationRequest body, Context context) {
reestablishReplicationAsync(resourceGroupName, accountName, poolName, volumeName, body, context).block();
}
/**
* Get volume replication status
*
* Get the status of the replication.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the status of the replication along with {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono> replicationStatusWithResponseAsync(String resourceGroupName,
String accountName, String poolName, String volumeName) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (accountName == null) {
return Mono.error(new IllegalArgumentException("Parameter accountName is required and cannot be null."));
}
if (poolName == null) {
return Mono.error(new IllegalArgumentException("Parameter poolName is required and cannot be null."));
}
if (volumeName == null) {
return Mono.error(new IllegalArgumentException("Parameter volumeName is required and cannot be null."));
}
final String accept = "application/json";
return FluxUtil
.withContext(
context -> service.replicationStatus(this.client.getEndpoint(), this.client.getSubscriptionId(),
resourceGroupName, accountName, poolName, volumeName, this.client.getApiVersion(), accept, context))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* Get volume replication status
*
* Get the status of the replication.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the status of the replication along with {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono> replicationStatusWithResponseAsync(String resourceGroupName,
String accountName, String poolName, String volumeName, Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (accountName == null) {
return Mono.error(new IllegalArgumentException("Parameter accountName is required and cannot be null."));
}
if (poolName == null) {
return Mono.error(new IllegalArgumentException("Parameter poolName is required and cannot be null."));
}
if (volumeName == null) {
return Mono.error(new IllegalArgumentException("Parameter volumeName is required and cannot be null."));
}
final String accept = "application/json";
context = this.client.mergeContext(context);
return service.replicationStatus(this.client.getEndpoint(), this.client.getSubscriptionId(), resourceGroupName,
accountName, poolName, volumeName, this.client.getApiVersion(), accept, context);
}
/**
* Get volume replication status
*
* Get the status of the replication.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the status of the replication on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono replicationStatusAsync(String resourceGroupName, String accountName,
String poolName, String volumeName) {
return replicationStatusWithResponseAsync(resourceGroupName, accountName, poolName, volumeName)
.flatMap(res -> Mono.justOrEmpty(res.getValue()));
}
/**
* Get volume replication status
*
* Get the status of the replication.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the status of the replication along with {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Response replicationStatusWithResponse(String resourceGroupName, String accountName,
String poolName, String volumeName, Context context) {
return replicationStatusWithResponseAsync(resourceGroupName, accountName, poolName, volumeName, context)
.block();
}
/**
* Get volume replication status
*
* Get the status of the replication.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the status of the replication.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public ReplicationStatusInner replicationStatus(String resourceGroupName, String accountName, String poolName,
String volumeName) {
return replicationStatusWithResponse(resourceGroupName, accountName, poolName, volumeName, Context.NONE)
.getValue();
}
/**
* List replications for volume
*
* List all replications for a specified volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return list Replications along with {@link PagedResponse} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono> listReplicationsSinglePageAsync(String resourceGroupName,
String accountName, String poolName, String volumeName) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (accountName == null) {
return Mono.error(new IllegalArgumentException("Parameter accountName is required and cannot be null."));
}
if (poolName == null) {
return Mono.error(new IllegalArgumentException("Parameter poolName is required and cannot be null."));
}
if (volumeName == null) {
return Mono.error(new IllegalArgumentException("Parameter volumeName is required and cannot be null."));
}
final String accept = "application/json";
return FluxUtil
.withContext(context -> service.listReplications(this.client.getEndpoint(), this.client.getSubscriptionId(),
resourceGroupName, accountName, poolName, volumeName, this.client.getApiVersion(), accept, context))
.>map(res -> new PagedResponseBase<>(res.getRequest(), res.getStatusCode(),
res.getHeaders(), res.getValue().value(), null, null))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* List replications for volume
*
* List all replications for a specified volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return list Replications along with {@link PagedResponse} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono> listReplicationsSinglePageAsync(String resourceGroupName,
String accountName, String poolName, String volumeName, Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (accountName == null) {
return Mono.error(new IllegalArgumentException("Parameter accountName is required and cannot be null."));
}
if (poolName == null) {
return Mono.error(new IllegalArgumentException("Parameter poolName is required and cannot be null."));
}
if (volumeName == null) {
return Mono.error(new IllegalArgumentException("Parameter volumeName is required and cannot be null."));
}
final String accept = "application/json";
context = this.client.mergeContext(context);
return service
.listReplications(this.client.getEndpoint(), this.client.getSubscriptionId(), resourceGroupName,
accountName, poolName, volumeName, this.client.getApiVersion(), accept, context)
.map(res -> new PagedResponseBase<>(res.getRequest(), res.getStatusCode(), res.getHeaders(),
res.getValue().value(), null, null));
}
/**
* List replications for volume
*
* List all replications for a specified volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return list Replications as paginated response with {@link PagedFlux}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
private PagedFlux listReplicationsAsync(String resourceGroupName, String accountName,
String poolName, String volumeName) {
return new PagedFlux<>(
() -> listReplicationsSinglePageAsync(resourceGroupName, accountName, poolName, volumeName));
}
/**
* List replications for volume
*
* List all replications for a specified volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return list Replications as paginated response with {@link PagedFlux}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
private PagedFlux listReplicationsAsync(String resourceGroupName, String accountName,
String poolName, String volumeName, Context context) {
return new PagedFlux<>(
() -> listReplicationsSinglePageAsync(resourceGroupName, accountName, poolName, volumeName, context));
}
/**
* List replications for volume
*
* List all replications for a specified volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return list Replications as paginated response with {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
public PagedIterable listReplications(String resourceGroupName, String accountName,
String poolName, String volumeName) {
return new PagedIterable<>(listReplicationsAsync(resourceGroupName, accountName, poolName, volumeName));
}
/**
* List replications for volume
*
* List all replications for a specified volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return list Replications as paginated response with {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
public PagedIterable listReplications(String resourceGroupName, String accountName,
String poolName, String volumeName, Context context) {
return new PagedIterable<>(
listReplicationsAsync(resourceGroupName, accountName, poolName, volumeName, context));
}
/**
* Resync volume replication
*
* Resync the connection on the destination volume. If the operation is ran on the source volume it will
* reverse-resync the connection and sync from destination to source.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono>> resyncReplicationWithResponseAsync(String resourceGroupName,
String accountName, String poolName, String volumeName) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (accountName == null) {
return Mono.error(new IllegalArgumentException("Parameter accountName is required and cannot be null."));
}
if (poolName == null) {
return Mono.error(new IllegalArgumentException("Parameter poolName is required and cannot be null."));
}
if (volumeName == null) {
return Mono.error(new IllegalArgumentException("Parameter volumeName is required and cannot be null."));
}
final String accept = "application/json";
return FluxUtil
.withContext(
context -> service.resyncReplication(this.client.getEndpoint(), this.client.getSubscriptionId(),
resourceGroupName, accountName, poolName, volumeName, this.client.getApiVersion(), accept, context))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* Resync volume replication
*
* Resync the connection on the destination volume. If the operation is ran on the source volume it will
* reverse-resync the connection and sync from destination to source.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono>> resyncReplicationWithResponseAsync(String resourceGroupName,
String accountName, String poolName, String volumeName, Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (accountName == null) {
return Mono.error(new IllegalArgumentException("Parameter accountName is required and cannot be null."));
}
if (poolName == null) {
return Mono.error(new IllegalArgumentException("Parameter poolName is required and cannot be null."));
}
if (volumeName == null) {
return Mono.error(new IllegalArgumentException("Parameter volumeName is required and cannot be null."));
}
final String accept = "application/json";
context = this.client.mergeContext(context);
return service.resyncReplication(this.client.getEndpoint(), this.client.getSubscriptionId(), resourceGroupName,
accountName, poolName, volumeName, this.client.getApiVersion(), accept, context);
}
/**
* Resync volume replication
*
* Resync the connection on the destination volume. If the operation is ran on the source volume it will
* reverse-resync the connection and sync from destination to source.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
private PollerFlux, Void> beginResyncReplicationAsync(String resourceGroupName, String accountName,
String poolName, String volumeName) {
Mono>> mono
= resyncReplicationWithResponseAsync(resourceGroupName, accountName, poolName, volumeName);
return this.client.getLroResult(mono, this.client.getHttpPipeline(), Void.class, Void.class,
this.client.getContext());
}
/**
* Resync volume replication
*
* Resync the connection on the destination volume. If the operation is ran on the source volume it will
* reverse-resync the connection and sync from destination to source.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
private PollerFlux, Void> beginResyncReplicationAsync(String resourceGroupName, String accountName,
String poolName, String volumeName, Context context) {
context = this.client.mergeContext(context);
Mono>> mono
= resyncReplicationWithResponseAsync(resourceGroupName, accountName, poolName, volumeName, context);
return this.client.getLroResult(mono, this.client.getHttpPipeline(), Void.class, Void.class,
context);
}
/**
* Resync volume replication
*
* Resync the connection on the destination volume. If the operation is ran on the source volume it will
* reverse-resync the connection and sync from destination to source.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, Void> beginResyncReplication(String resourceGroupName, String accountName,
String poolName, String volumeName) {
return this.beginResyncReplicationAsync(resourceGroupName, accountName, poolName, volumeName).getSyncPoller();
}
/**
* Resync volume replication
*
* Resync the connection on the destination volume. If the operation is ran on the source volume it will
* reverse-resync the connection and sync from destination to source.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, Void> beginResyncReplication(String resourceGroupName, String accountName,
String poolName, String volumeName, Context context) {
return this.beginResyncReplicationAsync(resourceGroupName, accountName, poolName, volumeName, context)
.getSyncPoller();
}
/**
* Resync volume replication
*
* Resync the connection on the destination volume. If the operation is ran on the source volume it will
* reverse-resync the connection and sync from destination to source.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono resyncReplicationAsync(String resourceGroupName, String accountName, String poolName,
String volumeName) {
return beginResyncReplicationAsync(resourceGroupName, accountName, poolName, volumeName).last()
.flatMap(this.client::getLroFinalResultOrError);
}
/**
* Resync volume replication
*
* Resync the connection on the destination volume. If the operation is ran on the source volume it will
* reverse-resync the connection and sync from destination to source.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono resyncReplicationAsync(String resourceGroupName, String accountName, String poolName,
String volumeName, Context context) {
return beginResyncReplicationAsync(resourceGroupName, accountName, poolName, volumeName, context).last()
.flatMap(this.client::getLroFinalResultOrError);
}
/**
* Resync volume replication
*
* Resync the connection on the destination volume. If the operation is ran on the source volume it will
* reverse-resync the connection and sync from destination to source.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public void resyncReplication(String resourceGroupName, String accountName, String poolName, String volumeName) {
resyncReplicationAsync(resourceGroupName, accountName, poolName, volumeName).block();
}
/**
* Resync volume replication
*
* Resync the connection on the destination volume. If the operation is ran on the source volume it will
* reverse-resync the connection and sync from destination to source.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public void resyncReplication(String resourceGroupName, String accountName, String poolName, String volumeName,
Context context) {
resyncReplicationAsync(resourceGroupName, accountName, poolName, volumeName, context).block();
}
/**
* Delete volume replication
*
* Delete the replication connection on the destination volume, and send release to the source replication.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono>> deleteReplicationWithResponseAsync(String resourceGroupName,
String accountName, String poolName, String volumeName) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (accountName == null) {
return Mono.error(new IllegalArgumentException("Parameter accountName is required and cannot be null."));
}
if (poolName == null) {
return Mono.error(new IllegalArgumentException("Parameter poolName is required and cannot be null."));
}
if (volumeName == null) {
return Mono.error(new IllegalArgumentException("Parameter volumeName is required and cannot be null."));
}
final String accept = "application/json";
return FluxUtil
.withContext(
context -> service.deleteReplication(this.client.getEndpoint(), this.client.getSubscriptionId(),
resourceGroupName, accountName, poolName, volumeName, this.client.getApiVersion(), accept, context))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* Delete volume replication
*
* Delete the replication connection on the destination volume, and send release to the source replication.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono>> deleteReplicationWithResponseAsync(String resourceGroupName,
String accountName, String poolName, String volumeName, Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (accountName == null) {
return Mono.error(new IllegalArgumentException("Parameter accountName is required and cannot be null."));
}
if (poolName == null) {
return Mono.error(new IllegalArgumentException("Parameter poolName is required and cannot be null."));
}
if (volumeName == null) {
return Mono.error(new IllegalArgumentException("Parameter volumeName is required and cannot be null."));
}
final String accept = "application/json";
context = this.client.mergeContext(context);
return service.deleteReplication(this.client.getEndpoint(), this.client.getSubscriptionId(), resourceGroupName,
accountName, poolName, volumeName, this.client.getApiVersion(), accept, context);
}
/**
* Delete volume replication
*
* Delete the replication connection on the destination volume, and send release to the source replication.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
private PollerFlux, Void> beginDeleteReplicationAsync(String resourceGroupName, String accountName,
String poolName, String volumeName) {
Mono>> mono
= deleteReplicationWithResponseAsync(resourceGroupName, accountName, poolName, volumeName);
return this.client.getLroResult(mono, this.client.getHttpPipeline(), Void.class, Void.class,
this.client.getContext());
}
/**
* Delete volume replication
*
* Delete the replication connection on the destination volume, and send release to the source replication.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
private PollerFlux, Void> beginDeleteReplicationAsync(String resourceGroupName, String accountName,
String poolName, String volumeName, Context context) {
context = this.client.mergeContext(context);
Mono>> mono
= deleteReplicationWithResponseAsync(resourceGroupName, accountName, poolName, volumeName, context);
return this.client.getLroResult(mono, this.client.getHttpPipeline(), Void.class, Void.class,
context);
}
/**
* Delete volume replication
*
* Delete the replication connection on the destination volume, and send release to the source replication.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, Void> beginDeleteReplication(String resourceGroupName, String accountName,
String poolName, String volumeName) {
return this.beginDeleteReplicationAsync(resourceGroupName, accountName, poolName, volumeName).getSyncPoller();
}
/**
* Delete volume replication
*
* Delete the replication connection on the destination volume, and send release to the source replication.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, Void> beginDeleteReplication(String resourceGroupName, String accountName,
String poolName, String volumeName, Context context) {
return this.beginDeleteReplicationAsync(resourceGroupName, accountName, poolName, volumeName, context)
.getSyncPoller();
}
/**
* Delete volume replication
*
* Delete the replication connection on the destination volume, and send release to the source replication.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono deleteReplicationAsync(String resourceGroupName, String accountName, String poolName,
String volumeName) {
return beginDeleteReplicationAsync(resourceGroupName, accountName, poolName, volumeName).last()
.flatMap(this.client::getLroFinalResultOrError);
}
/**
* Delete volume replication
*
* Delete the replication connection on the destination volume, and send release to the source replication.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono deleteReplicationAsync(String resourceGroupName, String accountName, String poolName,
String volumeName, Context context) {
return beginDeleteReplicationAsync(resourceGroupName, accountName, poolName, volumeName, context).last()
.flatMap(this.client::getLroFinalResultOrError);
}
/**
* Delete volume replication
*
* Delete the replication connection on the destination volume, and send release to the source replication.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public void deleteReplication(String resourceGroupName, String accountName, String poolName, String volumeName) {
deleteReplicationAsync(resourceGroupName, accountName, poolName, volumeName).block();
}
/**
* Delete volume replication
*
* Delete the replication connection on the destination volume, and send release to the source replication.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public void deleteReplication(String resourceGroupName, String accountName, String poolName, String volumeName,
Context context) {
deleteReplicationAsync(resourceGroupName, accountName, poolName, volumeName, context).block();
}
/**
* Authorize source volume replication
*
* Authorize the replication connection on the source volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param body Authorize request object supplied in the body of the operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono>> authorizeReplicationWithResponseAsync(String resourceGroupName,
String accountName, String poolName, String volumeName, AuthorizeRequest body) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (accountName == null) {
return Mono.error(new IllegalArgumentException("Parameter accountName is required and cannot be null."));
}
if (poolName == null) {
return Mono.error(new IllegalArgumentException("Parameter poolName is required and cannot be null."));
}
if (volumeName == null) {
return Mono.error(new IllegalArgumentException("Parameter volumeName is required and cannot be null."));
}
if (body == null) {
return Mono.error(new IllegalArgumentException("Parameter body is required and cannot be null."));
} else {
body.validate();
}
final String accept = "application/json";
return FluxUtil
.withContext(context -> service.authorizeReplication(this.client.getEndpoint(),
this.client.getSubscriptionId(), resourceGroupName, accountName, poolName, volumeName,
this.client.getApiVersion(), body, accept, context))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* Authorize source volume replication
*
* Authorize the replication connection on the source volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param body Authorize request object supplied in the body of the operation.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono>> authorizeReplicationWithResponseAsync(String resourceGroupName,
String accountName, String poolName, String volumeName, AuthorizeRequest body, Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (accountName == null) {
return Mono.error(new IllegalArgumentException("Parameter accountName is required and cannot be null."));
}
if (poolName == null) {
return Mono.error(new IllegalArgumentException("Parameter poolName is required and cannot be null."));
}
if (volumeName == null) {
return Mono.error(new IllegalArgumentException("Parameter volumeName is required and cannot be null."));
}
if (body == null) {
return Mono.error(new IllegalArgumentException("Parameter body is required and cannot be null."));
} else {
body.validate();
}
final String accept = "application/json";
context = this.client.mergeContext(context);
return service.authorizeReplication(this.client.getEndpoint(), this.client.getSubscriptionId(),
resourceGroupName, accountName, poolName, volumeName, this.client.getApiVersion(), body, accept, context);
}
/**
* Authorize source volume replication
*
* Authorize the replication connection on the source volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param body Authorize request object supplied in the body of the operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
private PollerFlux, Void> beginAuthorizeReplicationAsync(String resourceGroupName,
String accountName, String poolName, String volumeName, AuthorizeRequest body) {
Mono>> mono
= authorizeReplicationWithResponseAsync(resourceGroupName, accountName, poolName, volumeName, body);
return this.client.getLroResult(mono, this.client.getHttpPipeline(), Void.class, Void.class,
this.client.getContext());
}
/**
* Authorize source volume replication
*
* Authorize the replication connection on the source volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param body Authorize request object supplied in the body of the operation.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
private PollerFlux, Void> beginAuthorizeReplicationAsync(String resourceGroupName,
String accountName, String poolName, String volumeName, AuthorizeRequest body, Context context) {
context = this.client.mergeContext(context);
Mono>> mono = authorizeReplicationWithResponseAsync(resourceGroupName, accountName,
poolName, volumeName, body, context);
return this.client.getLroResult(mono, this.client.getHttpPipeline(), Void.class, Void.class,
context);
}
/**
* Authorize source volume replication
*
* Authorize the replication connection on the source volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param body Authorize request object supplied in the body of the operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, Void> beginAuthorizeReplication(String resourceGroupName, String accountName,
String poolName, String volumeName, AuthorizeRequest body) {
return this.beginAuthorizeReplicationAsync(resourceGroupName, accountName, poolName, volumeName, body)
.getSyncPoller();
}
/**
* Authorize source volume replication
*
* Authorize the replication connection on the source volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param body Authorize request object supplied in the body of the operation.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, Void> beginAuthorizeReplication(String resourceGroupName, String accountName,
String poolName, String volumeName, AuthorizeRequest body, Context context) {
return this.beginAuthorizeReplicationAsync(resourceGroupName, accountName, poolName, volumeName, body, context)
.getSyncPoller();
}
/**
* Authorize source volume replication
*
* Authorize the replication connection on the source volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param body Authorize request object supplied in the body of the operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono authorizeReplicationAsync(String resourceGroupName, String accountName, String poolName,
String volumeName, AuthorizeRequest body) {
return beginAuthorizeReplicationAsync(resourceGroupName, accountName, poolName, volumeName, body).last()
.flatMap(this.client::getLroFinalResultOrError);
}
/**
* Authorize source volume replication
*
* Authorize the replication connection on the source volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param body Authorize request object supplied in the body of the operation.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono authorizeReplicationAsync(String resourceGroupName, String accountName, String poolName,
String volumeName, AuthorizeRequest body, Context context) {
return beginAuthorizeReplicationAsync(resourceGroupName, accountName, poolName, volumeName, body, context)
.last()
.flatMap(this.client::getLroFinalResultOrError);
}
/**
* Authorize source volume replication
*
* Authorize the replication connection on the source volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param body Authorize request object supplied in the body of the operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public void authorizeReplication(String resourceGroupName, String accountName, String poolName, String volumeName,
AuthorizeRequest body) {
authorizeReplicationAsync(resourceGroupName, accountName, poolName, volumeName, body).block();
}
/**
* Authorize source volume replication
*
* Authorize the replication connection on the source volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param body Authorize request object supplied in the body of the operation.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public void authorizeReplication(String resourceGroupName, String accountName, String poolName, String volumeName,
AuthorizeRequest body, Context context) {
authorizeReplicationAsync(resourceGroupName, accountName, poolName, volumeName, body, context).block();
}
/**
* ReInitialize volume replication
*
* Re-Initializes the replication connection on the destination volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono>> reInitializeReplicationWithResponseAsync(String resourceGroupName,
String accountName, String poolName, String volumeName) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (accountName == null) {
return Mono.error(new IllegalArgumentException("Parameter accountName is required and cannot be null."));
}
if (poolName == null) {
return Mono.error(new IllegalArgumentException("Parameter poolName is required and cannot be null."));
}
if (volumeName == null) {
return Mono.error(new IllegalArgumentException("Parameter volumeName is required and cannot be null."));
}
final String accept = "application/json";
return FluxUtil
.withContext(
context -> service.reInitializeReplication(this.client.getEndpoint(), this.client.getSubscriptionId(),
resourceGroupName, accountName, poolName, volumeName, this.client.getApiVersion(), accept, context))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* ReInitialize volume replication
*
* Re-Initializes the replication connection on the destination volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono>> reInitializeReplicationWithResponseAsync(String resourceGroupName,
String accountName, String poolName, String volumeName, Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (accountName == null) {
return Mono.error(new IllegalArgumentException("Parameter accountName is required and cannot be null."));
}
if (poolName == null) {
return Mono.error(new IllegalArgumentException("Parameter poolName is required and cannot be null."));
}
if (volumeName == null) {
return Mono.error(new IllegalArgumentException("Parameter volumeName is required and cannot be null."));
}
final String accept = "application/json";
context = this.client.mergeContext(context);
return service.reInitializeReplication(this.client.getEndpoint(), this.client.getSubscriptionId(),
resourceGroupName, accountName, poolName, volumeName, this.client.getApiVersion(), accept, context);
}
/**
* ReInitialize volume replication
*
* Re-Initializes the replication connection on the destination volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
private PollerFlux, Void> beginReInitializeReplicationAsync(String resourceGroupName,
String accountName, String poolName, String volumeName) {
Mono>> mono
= reInitializeReplicationWithResponseAsync(resourceGroupName, accountName, poolName, volumeName);
return this.client.getLroResult(mono, this.client.getHttpPipeline(), Void.class, Void.class,
this.client.getContext());
}
/**
* ReInitialize volume replication
*
* Re-Initializes the replication connection on the destination volume.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param poolName The name of the capacity pool.
* @param volumeName The name of the volume.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
private PollerFlux, Void> beginReInitializeReplicationAsync(String resourceGroupName,
String accountName, String poolName, String volumeName, Context context) {
context = this.client.mergeContext(context);
Mono>> mono
= reInitializeReplicationWithResponseAsync(resourceGroupName, accountName, poolName, volumeName, context);
return this.client.