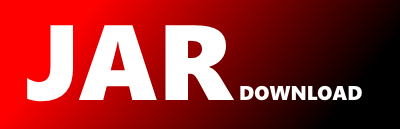
com.azure.resourcemanager.netapp.models.Accounts Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-netapp Show documentation
Show all versions of azure-resourcemanager-netapp Show documentation
This package contains Microsoft Azure SDK for NetAppFiles Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. Microsoft NetApp Files Azure Resource Provider specification. Package tag package-preview-2024-07-01-preview.
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.netapp.models;
import com.azure.core.http.rest.PagedIterable;
import com.azure.core.http.rest.Response;
import com.azure.core.util.Context;
/**
* Resource collection API of Accounts.
*/
public interface Accounts {
/**
* Describe all NetApp Accounts in a subscription
*
* List and describe all NetApp accounts in the subscription.
*
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return list of NetApp account resources as paginated response with {@link PagedIterable}.
*/
PagedIterable list();
/**
* Describe all NetApp Accounts in a subscription
*
* List and describe all NetApp accounts in the subscription.
*
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return list of NetApp account resources as paginated response with {@link PagedIterable}.
*/
PagedIterable list(Context context);
/**
* Describe all NetApp Accounts in a resource group
*
* List and describe all NetApp accounts in the resource group.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return list of NetApp account resources as paginated response with {@link PagedIterable}.
*/
PagedIterable listByResourceGroup(String resourceGroupName);
/**
* Describe all NetApp Accounts in a resource group
*
* List and describe all NetApp accounts in the resource group.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return list of NetApp account resources as paginated response with {@link PagedIterable}.
*/
PagedIterable listByResourceGroup(String resourceGroupName, Context context);
/**
* Describe a NetApp Account
*
* Get the NetApp account.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the NetApp account along with {@link Response}.
*/
Response getByResourceGroupWithResponse(String resourceGroupName, String accountName,
Context context);
/**
* Describe a NetApp Account
*
* Get the NetApp account.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the NetApp account.
*/
NetAppAccount getByResourceGroup(String resourceGroupName, String accountName);
/**
* Delete a NetApp account
*
* Delete the specified NetApp account.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
void deleteByResourceGroup(String resourceGroupName, String accountName);
/**
* Delete a NetApp account
*
* Delete the specified NetApp account.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
void delete(String resourceGroupName, String accountName, Context context);
/**
* Renew identity credentials
*
* Renew identity credentials that are used to authenticate to key vault, for customer-managed key encryption. If
* encryption.identity.principalId does not match identity.principalId, running this operation will fix it.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
void renewCredentials(String resourceGroupName, String accountName);
/**
* Renew identity credentials
*
* Renew identity credentials that are used to authenticate to key vault, for customer-managed key encryption. If
* encryption.identity.principalId does not match identity.principalId, running this operation will fix it.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
void renewCredentials(String resourceGroupName, String accountName, Context context);
/**
* Transition volumes encryption from PMK to CMK.
*
* Transitions all volumes in a VNet to a different encryption key source (Microsoft-managed key or Azure Key
* Vault). Operation fails if targeted volumes share encryption sibling set with volumes from another account.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
void transitionToCmk(String resourceGroupName, String accountName);
/**
* Transition volumes encryption from PMK to CMK.
*
* Transitions all volumes in a VNet to a different encryption key source (Microsoft-managed key or Azure Key
* Vault). Operation fails if targeted volumes share encryption sibling set with volumes from another account.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param body The required parameters to perform encryption transition.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
void transitionToCmk(String resourceGroupName, String accountName, EncryptionTransitionRequest body,
Context context);
/**
* Get information about how volumes under NetApp account are encrypted.
*
* Contains data from encryption.keyVaultProperties as well as information about which private endpoint is used by
* each encryption sibling set. Response from this endpoint can be modified and used as request body for POST
* request.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
void getChangeKeyVaultInformation(String resourceGroupName, String accountName);
/**
* Get information about how volumes under NetApp account are encrypted.
*
* Contains data from encryption.keyVaultProperties as well as information about which private endpoint is used by
* each encryption sibling set. Response from this endpoint can be modified and used as request body for POST
* request.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
void getChangeKeyVaultInformation(String resourceGroupName, String accountName, Context context);
/**
* Change Key Vault/Managed HSM that is used for encryption of volumes under NetApp account.
*
* Affects existing volumes that are encrypted with Key Vault/Managed HSM, and new volumes. Supports HSM to Key
* Vault, Key Vault to HSM, HSM to HSM and Key Vault to Key Vault.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
void changeKeyVault(String resourceGroupName, String accountName);
/**
* Change Key Vault/Managed HSM that is used for encryption of volumes under NetApp account.
*
* Affects existing volumes that are encrypted with Key Vault/Managed HSM, and new volumes. Supports HSM to Key
* Vault, Key Vault to HSM, HSM to HSM and Key Vault to Key Vault.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName The name of the NetApp account.
* @param body The required parameters to perform encryption migration.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
void changeKeyVault(String resourceGroupName, String accountName, ChangeKeyVault body, Context context);
/**
* Describe a NetApp Account
*
* Get the NetApp account.
*
* @param id the resource ID.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the NetApp account along with {@link Response}.
*/
NetAppAccount getById(String id);
/**
* Describe a NetApp Account
*
* Get the NetApp account.
*
* @param id the resource ID.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the NetApp account along with {@link Response}.
*/
Response getByIdWithResponse(String id, Context context);
/**
* Delete a NetApp account
*
* Delete the specified NetApp account.
*
* @param id the resource ID.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
void deleteById(String id);
/**
* Delete a NetApp account
*
* Delete the specified NetApp account.
*
* @param id the resource ID.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
void deleteByIdWithResponse(String id, Context context);
/**
* Begins definition for a new NetAppAccount resource.
*
* @param name resource name.
* @return the first stage of the new NetAppAccount definition.
*/
NetAppAccount.DefinitionStages.Blank define(String name);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy