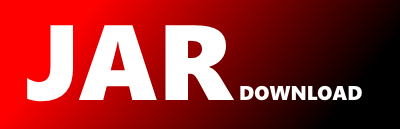
com.azure.resourcemanager.network.fluent.models.VpnConnectionProperties Maven / Gradle / Ivy
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.network.fluent.models;
import com.azure.core.annotation.Fluent;
import com.azure.core.management.SubResource;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import com.azure.resourcemanager.network.models.IpsecPolicy;
import com.azure.resourcemanager.network.models.ProvisioningState;
import com.azure.resourcemanager.network.models.RoutingConfiguration;
import com.azure.resourcemanager.network.models.TrafficSelectorPolicy;
import com.azure.resourcemanager.network.models.VirtualNetworkGatewayConnectionProtocol;
import com.azure.resourcemanager.network.models.VpnConnectionStatus;
import java.io.IOException;
import java.util.List;
/**
* Parameters for VpnConnection.
*/
@Fluent
public final class VpnConnectionProperties implements JsonSerializable {
/*
* Id of the connected vpn site.
*/
private SubResource remoteVpnSite;
/*
* Routing weight for vpn connection.
*/
private Integer routingWeight;
/*
* DPD timeout in seconds for vpn connection.
*/
private Integer dpdTimeoutSeconds;
/*
* The connection status.
*/
private VpnConnectionStatus connectionStatus;
/*
* Connection protocol used for this connection.
*/
private VirtualNetworkGatewayConnectionProtocol vpnConnectionProtocolType;
/*
* Ingress bytes transferred.
*/
private Long ingressBytesTransferred;
/*
* Egress bytes transferred.
*/
private Long egressBytesTransferred;
/*
* Expected bandwidth in MBPS.
*/
private Integer connectionBandwidth;
/*
* SharedKey for the vpn connection.
*/
private String sharedKey;
/*
* EnableBgp flag.
*/
private Boolean enableBgp;
/*
* Enable policy-based traffic selectors.
*/
private Boolean usePolicyBasedTrafficSelectors;
/*
* The IPSec Policies to be considered by this connection.
*/
private List ipsecPolicies;
/*
* The Traffic Selector Policies to be considered by this connection.
*/
private List trafficSelectorPolicies;
/*
* EnableBgp flag.
*/
private Boolean enableRateLimiting;
/*
* Enable internet security.
*/
private Boolean enableInternetSecurity;
/*
* Use local azure ip to initiate connection.
*/
private Boolean useLocalAzureIpAddress;
/*
* The provisioning state of the VPN connection resource.
*/
private ProvisioningState provisioningState;
/*
* List of all vpn site link connections to the gateway.
*/
private List vpnLinkConnections;
/*
* The Routing Configuration indicating the associated and propagated route tables on this connection.
*/
private RoutingConfiguration routingConfiguration;
/**
* Creates an instance of VpnConnectionProperties class.
*/
public VpnConnectionProperties() {
}
/**
* Get the remoteVpnSite property: Id of the connected vpn site.
*
* @return the remoteVpnSite value.
*/
public SubResource remoteVpnSite() {
return this.remoteVpnSite;
}
/**
* Set the remoteVpnSite property: Id of the connected vpn site.
*
* @param remoteVpnSite the remoteVpnSite value to set.
* @return the VpnConnectionProperties object itself.
*/
public VpnConnectionProperties withRemoteVpnSite(SubResource remoteVpnSite) {
this.remoteVpnSite = remoteVpnSite;
return this;
}
/**
* Get the routingWeight property: Routing weight for vpn connection.
*
* @return the routingWeight value.
*/
public Integer routingWeight() {
return this.routingWeight;
}
/**
* Set the routingWeight property: Routing weight for vpn connection.
*
* @param routingWeight the routingWeight value to set.
* @return the VpnConnectionProperties object itself.
*/
public VpnConnectionProperties withRoutingWeight(Integer routingWeight) {
this.routingWeight = routingWeight;
return this;
}
/**
* Get the dpdTimeoutSeconds property: DPD timeout in seconds for vpn connection.
*
* @return the dpdTimeoutSeconds value.
*/
public Integer dpdTimeoutSeconds() {
return this.dpdTimeoutSeconds;
}
/**
* Set the dpdTimeoutSeconds property: DPD timeout in seconds for vpn connection.
*
* @param dpdTimeoutSeconds the dpdTimeoutSeconds value to set.
* @return the VpnConnectionProperties object itself.
*/
public VpnConnectionProperties withDpdTimeoutSeconds(Integer dpdTimeoutSeconds) {
this.dpdTimeoutSeconds = dpdTimeoutSeconds;
return this;
}
/**
* Get the connectionStatus property: The connection status.
*
* @return the connectionStatus value.
*/
public VpnConnectionStatus connectionStatus() {
return this.connectionStatus;
}
/**
* Get the vpnConnectionProtocolType property: Connection protocol used for this connection.
*
* @return the vpnConnectionProtocolType value.
*/
public VirtualNetworkGatewayConnectionProtocol vpnConnectionProtocolType() {
return this.vpnConnectionProtocolType;
}
/**
* Set the vpnConnectionProtocolType property: Connection protocol used for this connection.
*
* @param vpnConnectionProtocolType the vpnConnectionProtocolType value to set.
* @return the VpnConnectionProperties object itself.
*/
public VpnConnectionProperties
withVpnConnectionProtocolType(VirtualNetworkGatewayConnectionProtocol vpnConnectionProtocolType) {
this.vpnConnectionProtocolType = vpnConnectionProtocolType;
return this;
}
/**
* Get the ingressBytesTransferred property: Ingress bytes transferred.
*
* @return the ingressBytesTransferred value.
*/
public Long ingressBytesTransferred() {
return this.ingressBytesTransferred;
}
/**
* Get the egressBytesTransferred property: Egress bytes transferred.
*
* @return the egressBytesTransferred value.
*/
public Long egressBytesTransferred() {
return this.egressBytesTransferred;
}
/**
* Get the connectionBandwidth property: Expected bandwidth in MBPS.
*
* @return the connectionBandwidth value.
*/
public Integer connectionBandwidth() {
return this.connectionBandwidth;
}
/**
* Set the connectionBandwidth property: Expected bandwidth in MBPS.
*
* @param connectionBandwidth the connectionBandwidth value to set.
* @return the VpnConnectionProperties object itself.
*/
public VpnConnectionProperties withConnectionBandwidth(Integer connectionBandwidth) {
this.connectionBandwidth = connectionBandwidth;
return this;
}
/**
* Get the sharedKey property: SharedKey for the vpn connection.
*
* @return the sharedKey value.
*/
public String sharedKey() {
return this.sharedKey;
}
/**
* Set the sharedKey property: SharedKey for the vpn connection.
*
* @param sharedKey the sharedKey value to set.
* @return the VpnConnectionProperties object itself.
*/
public VpnConnectionProperties withSharedKey(String sharedKey) {
this.sharedKey = sharedKey;
return this;
}
/**
* Get the enableBgp property: EnableBgp flag.
*
* @return the enableBgp value.
*/
public Boolean enableBgp() {
return this.enableBgp;
}
/**
* Set the enableBgp property: EnableBgp flag.
*
* @param enableBgp the enableBgp value to set.
* @return the VpnConnectionProperties object itself.
*/
public VpnConnectionProperties withEnableBgp(Boolean enableBgp) {
this.enableBgp = enableBgp;
return this;
}
/**
* Get the usePolicyBasedTrafficSelectors property: Enable policy-based traffic selectors.
*
* @return the usePolicyBasedTrafficSelectors value.
*/
public Boolean usePolicyBasedTrafficSelectors() {
return this.usePolicyBasedTrafficSelectors;
}
/**
* Set the usePolicyBasedTrafficSelectors property: Enable policy-based traffic selectors.
*
* @param usePolicyBasedTrafficSelectors the usePolicyBasedTrafficSelectors value to set.
* @return the VpnConnectionProperties object itself.
*/
public VpnConnectionProperties withUsePolicyBasedTrafficSelectors(Boolean usePolicyBasedTrafficSelectors) {
this.usePolicyBasedTrafficSelectors = usePolicyBasedTrafficSelectors;
return this;
}
/**
* Get the ipsecPolicies property: The IPSec Policies to be considered by this connection.
*
* @return the ipsecPolicies value.
*/
public List ipsecPolicies() {
return this.ipsecPolicies;
}
/**
* Set the ipsecPolicies property: The IPSec Policies to be considered by this connection.
*
* @param ipsecPolicies the ipsecPolicies value to set.
* @return the VpnConnectionProperties object itself.
*/
public VpnConnectionProperties withIpsecPolicies(List ipsecPolicies) {
this.ipsecPolicies = ipsecPolicies;
return this;
}
/**
* Get the trafficSelectorPolicies property: The Traffic Selector Policies to be considered by this connection.
*
* @return the trafficSelectorPolicies value.
*/
public List trafficSelectorPolicies() {
return this.trafficSelectorPolicies;
}
/**
* Set the trafficSelectorPolicies property: The Traffic Selector Policies to be considered by this connection.
*
* @param trafficSelectorPolicies the trafficSelectorPolicies value to set.
* @return the VpnConnectionProperties object itself.
*/
public VpnConnectionProperties withTrafficSelectorPolicies(List trafficSelectorPolicies) {
this.trafficSelectorPolicies = trafficSelectorPolicies;
return this;
}
/**
* Get the enableRateLimiting property: EnableBgp flag.
*
* @return the enableRateLimiting value.
*/
public Boolean enableRateLimiting() {
return this.enableRateLimiting;
}
/**
* Set the enableRateLimiting property: EnableBgp flag.
*
* @param enableRateLimiting the enableRateLimiting value to set.
* @return the VpnConnectionProperties object itself.
*/
public VpnConnectionProperties withEnableRateLimiting(Boolean enableRateLimiting) {
this.enableRateLimiting = enableRateLimiting;
return this;
}
/**
* Get the enableInternetSecurity property: Enable internet security.
*
* @return the enableInternetSecurity value.
*/
public Boolean enableInternetSecurity() {
return this.enableInternetSecurity;
}
/**
* Set the enableInternetSecurity property: Enable internet security.
*
* @param enableInternetSecurity the enableInternetSecurity value to set.
* @return the VpnConnectionProperties object itself.
*/
public VpnConnectionProperties withEnableInternetSecurity(Boolean enableInternetSecurity) {
this.enableInternetSecurity = enableInternetSecurity;
return this;
}
/**
* Get the useLocalAzureIpAddress property: Use local azure ip to initiate connection.
*
* @return the useLocalAzureIpAddress value.
*/
public Boolean useLocalAzureIpAddress() {
return this.useLocalAzureIpAddress;
}
/**
* Set the useLocalAzureIpAddress property: Use local azure ip to initiate connection.
*
* @param useLocalAzureIpAddress the useLocalAzureIpAddress value to set.
* @return the VpnConnectionProperties object itself.
*/
public VpnConnectionProperties withUseLocalAzureIpAddress(Boolean useLocalAzureIpAddress) {
this.useLocalAzureIpAddress = useLocalAzureIpAddress;
return this;
}
/**
* Get the provisioningState property: The provisioning state of the VPN connection resource.
*
* @return the provisioningState value.
*/
public ProvisioningState provisioningState() {
return this.provisioningState;
}
/**
* Get the vpnLinkConnections property: List of all vpn site link connections to the gateway.
*
* @return the vpnLinkConnections value.
*/
public List vpnLinkConnections() {
return this.vpnLinkConnections;
}
/**
* Set the vpnLinkConnections property: List of all vpn site link connections to the gateway.
*
* @param vpnLinkConnections the vpnLinkConnections value to set.
* @return the VpnConnectionProperties object itself.
*/
public VpnConnectionProperties withVpnLinkConnections(List vpnLinkConnections) {
this.vpnLinkConnections = vpnLinkConnections;
return this;
}
/**
* Get the routingConfiguration property: The Routing Configuration indicating the associated and propagated route
* tables on this connection.
*
* @return the routingConfiguration value.
*/
public RoutingConfiguration routingConfiguration() {
return this.routingConfiguration;
}
/**
* Set the routingConfiguration property: The Routing Configuration indicating the associated and propagated route
* tables on this connection.
*
* @param routingConfiguration the routingConfiguration value to set.
* @return the VpnConnectionProperties object itself.
*/
public VpnConnectionProperties withRoutingConfiguration(RoutingConfiguration routingConfiguration) {
this.routingConfiguration = routingConfiguration;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (ipsecPolicies() != null) {
ipsecPolicies().forEach(e -> e.validate());
}
if (trafficSelectorPolicies() != null) {
trafficSelectorPolicies().forEach(e -> e.validate());
}
if (vpnLinkConnections() != null) {
vpnLinkConnections().forEach(e -> e.validate());
}
if (routingConfiguration() != null) {
routingConfiguration().validate();
}
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeJsonField("remoteVpnSite", this.remoteVpnSite);
jsonWriter.writeNumberField("routingWeight", this.routingWeight);
jsonWriter.writeNumberField("dpdTimeoutSeconds", this.dpdTimeoutSeconds);
jsonWriter.writeStringField("vpnConnectionProtocolType",
this.vpnConnectionProtocolType == null ? null : this.vpnConnectionProtocolType.toString());
jsonWriter.writeNumberField("connectionBandwidth", this.connectionBandwidth);
jsonWriter.writeStringField("sharedKey", this.sharedKey);
jsonWriter.writeBooleanField("enableBgp", this.enableBgp);
jsonWriter.writeBooleanField("usePolicyBasedTrafficSelectors", this.usePolicyBasedTrafficSelectors);
jsonWriter.writeArrayField("ipsecPolicies", this.ipsecPolicies, (writer, element) -> writer.writeJson(element));
jsonWriter.writeArrayField("trafficSelectorPolicies", this.trafficSelectorPolicies,
(writer, element) -> writer.writeJson(element));
jsonWriter.writeBooleanField("enableRateLimiting", this.enableRateLimiting);
jsonWriter.writeBooleanField("enableInternetSecurity", this.enableInternetSecurity);
jsonWriter.writeBooleanField("useLocalAzureIpAddress", this.useLocalAzureIpAddress);
jsonWriter.writeArrayField("vpnLinkConnections", this.vpnLinkConnections,
(writer, element) -> writer.writeJson(element));
jsonWriter.writeJsonField("routingConfiguration", this.routingConfiguration);
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of VpnConnectionProperties from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of VpnConnectionProperties if the JsonReader was pointing to an instance of it, or null if it
* was pointing to JSON null.
* @throws IOException If an error occurs while reading the VpnConnectionProperties.
*/
public static VpnConnectionProperties fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
VpnConnectionProperties deserializedVpnConnectionProperties = new VpnConnectionProperties();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("remoteVpnSite".equals(fieldName)) {
deserializedVpnConnectionProperties.remoteVpnSite = SubResource.fromJson(reader);
} else if ("routingWeight".equals(fieldName)) {
deserializedVpnConnectionProperties.routingWeight = reader.getNullable(JsonReader::getInt);
} else if ("dpdTimeoutSeconds".equals(fieldName)) {
deserializedVpnConnectionProperties.dpdTimeoutSeconds = reader.getNullable(JsonReader::getInt);
} else if ("connectionStatus".equals(fieldName)) {
deserializedVpnConnectionProperties.connectionStatus
= VpnConnectionStatus.fromString(reader.getString());
} else if ("vpnConnectionProtocolType".equals(fieldName)) {
deserializedVpnConnectionProperties.vpnConnectionProtocolType
= VirtualNetworkGatewayConnectionProtocol.fromString(reader.getString());
} else if ("ingressBytesTransferred".equals(fieldName)) {
deserializedVpnConnectionProperties.ingressBytesTransferred
= reader.getNullable(JsonReader::getLong);
} else if ("egressBytesTransferred".equals(fieldName)) {
deserializedVpnConnectionProperties.egressBytesTransferred
= reader.getNullable(JsonReader::getLong);
} else if ("connectionBandwidth".equals(fieldName)) {
deserializedVpnConnectionProperties.connectionBandwidth = reader.getNullable(JsonReader::getInt);
} else if ("sharedKey".equals(fieldName)) {
deserializedVpnConnectionProperties.sharedKey = reader.getString();
} else if ("enableBgp".equals(fieldName)) {
deserializedVpnConnectionProperties.enableBgp = reader.getNullable(JsonReader::getBoolean);
} else if ("usePolicyBasedTrafficSelectors".equals(fieldName)) {
deserializedVpnConnectionProperties.usePolicyBasedTrafficSelectors
= reader.getNullable(JsonReader::getBoolean);
} else if ("ipsecPolicies".equals(fieldName)) {
List ipsecPolicies = reader.readArray(reader1 -> IpsecPolicy.fromJson(reader1));
deserializedVpnConnectionProperties.ipsecPolicies = ipsecPolicies;
} else if ("trafficSelectorPolicies".equals(fieldName)) {
List trafficSelectorPolicies
= reader.readArray(reader1 -> TrafficSelectorPolicy.fromJson(reader1));
deserializedVpnConnectionProperties.trafficSelectorPolicies = trafficSelectorPolicies;
} else if ("enableRateLimiting".equals(fieldName)) {
deserializedVpnConnectionProperties.enableRateLimiting = reader.getNullable(JsonReader::getBoolean);
} else if ("enableInternetSecurity".equals(fieldName)) {
deserializedVpnConnectionProperties.enableInternetSecurity
= reader.getNullable(JsonReader::getBoolean);
} else if ("useLocalAzureIpAddress".equals(fieldName)) {
deserializedVpnConnectionProperties.useLocalAzureIpAddress
= reader.getNullable(JsonReader::getBoolean);
} else if ("provisioningState".equals(fieldName)) {
deserializedVpnConnectionProperties.provisioningState
= ProvisioningState.fromString(reader.getString());
} else if ("vpnLinkConnections".equals(fieldName)) {
List vpnLinkConnections
= reader.readArray(reader1 -> VpnSiteLinkConnectionInner.fromJson(reader1));
deserializedVpnConnectionProperties.vpnLinkConnections = vpnLinkConnections;
} else if ("routingConfiguration".equals(fieldName)) {
deserializedVpnConnectionProperties.routingConfiguration = RoutingConfiguration.fromJson(reader);
} else {
reader.skipChildren();
}
}
return deserializedVpnConnectionProperties;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy