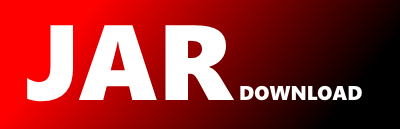
com.azure.resourcemanager.newrelicobservability.fluent.models.MetricRulesInner Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-newrelicobservability Show documentation
Show all versions of azure-resourcemanager-newrelicobservability Show documentation
This package contains Microsoft Azure SDK for NewRelicObservability Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. Package tag package-2024-01-01.
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.newrelicobservability.fluent.models;
import com.azure.core.annotation.Fluent;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import com.azure.resourcemanager.newrelicobservability.models.FilteringTag;
import com.azure.resourcemanager.newrelicobservability.models.SendMetricsStatus;
import java.io.IOException;
import java.util.List;
/**
* Set of rules for sending metrics for the Monitor resource.
*/
@Fluent
public final class MetricRulesInner implements JsonSerializable {
/*
* Flag specifying if metrics should be sent for the Monitor resource.
*/
private SendMetricsStatus sendMetrics;
/*
* List of filtering tags to be used for capturing metrics.
*/
private List filteringTags;
/*
* User Email
*/
private String userEmail;
/**
* Creates an instance of MetricRulesInner class.
*/
public MetricRulesInner() {
}
/**
* Get the sendMetrics property: Flag specifying if metrics should be sent for the Monitor resource.
*
* @return the sendMetrics value.
*/
public SendMetricsStatus sendMetrics() {
return this.sendMetrics;
}
/**
* Set the sendMetrics property: Flag specifying if metrics should be sent for the Monitor resource.
*
* @param sendMetrics the sendMetrics value to set.
* @return the MetricRulesInner object itself.
*/
public MetricRulesInner withSendMetrics(SendMetricsStatus sendMetrics) {
this.sendMetrics = sendMetrics;
return this;
}
/**
* Get the filteringTags property: List of filtering tags to be used for capturing metrics.
*
* @return the filteringTags value.
*/
public List filteringTags() {
return this.filteringTags;
}
/**
* Set the filteringTags property: List of filtering tags to be used for capturing metrics.
*
* @param filteringTags the filteringTags value to set.
* @return the MetricRulesInner object itself.
*/
public MetricRulesInner withFilteringTags(List filteringTags) {
this.filteringTags = filteringTags;
return this;
}
/**
* Get the userEmail property: User Email.
*
* @return the userEmail value.
*/
public String userEmail() {
return this.userEmail;
}
/**
* Set the userEmail property: User Email.
*
* @param userEmail the userEmail value to set.
* @return the MetricRulesInner object itself.
*/
public MetricRulesInner withUserEmail(String userEmail) {
this.userEmail = userEmail;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (filteringTags() != null) {
filteringTags().forEach(e -> e.validate());
}
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("sendMetrics", this.sendMetrics == null ? null : this.sendMetrics.toString());
jsonWriter.writeArrayField("filteringTags", this.filteringTags, (writer, element) -> writer.writeJson(element));
jsonWriter.writeStringField("userEmail", this.userEmail);
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of MetricRulesInner from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of MetricRulesInner if the JsonReader was pointing to an instance of it, or null if it was
* pointing to JSON null.
* @throws IOException If an error occurs while reading the MetricRulesInner.
*/
public static MetricRulesInner fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
MetricRulesInner deserializedMetricRulesInner = new MetricRulesInner();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("sendMetrics".equals(fieldName)) {
deserializedMetricRulesInner.sendMetrics = SendMetricsStatus.fromString(reader.getString());
} else if ("filteringTags".equals(fieldName)) {
List filteringTags = reader.readArray(reader1 -> FilteringTag.fromJson(reader1));
deserializedMetricRulesInner.filteringTags = filteringTags;
} else if ("userEmail".equals(fieldName)) {
deserializedMetricRulesInner.userEmail = reader.getString();
} else {
reader.skipChildren();
}
}
return deserializedMetricRulesInner;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy