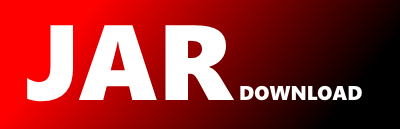
com.azure.resourcemanager.notificationhubs.fluent.models.PnsCredentialsProperties Maven / Gradle / Ivy
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.notificationhubs.fluent.models;
import com.azure.core.annotation.Fluent;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import com.azure.resourcemanager.notificationhubs.models.AdmCredential;
import com.azure.resourcemanager.notificationhubs.models.ApnsCredential;
import com.azure.resourcemanager.notificationhubs.models.BaiduCredential;
import com.azure.resourcemanager.notificationhubs.models.GcmCredential;
import com.azure.resourcemanager.notificationhubs.models.MpnsCredential;
import com.azure.resourcemanager.notificationhubs.models.WnsCredential;
import java.io.IOException;
/**
* Description of a NotificationHub PNS Credentials.
*/
@Fluent
public final class PnsCredentialsProperties implements JsonSerializable {
/*
* The ApnsCredential of the created NotificationHub
*/
private ApnsCredential apnsCredential;
/*
* The WnsCredential of the created NotificationHub
*/
private WnsCredential wnsCredential;
/*
* The GcmCredential of the created NotificationHub
*/
private GcmCredential gcmCredential;
/*
* The MpnsCredential of the created NotificationHub
*/
private MpnsCredential mpnsCredential;
/*
* The AdmCredential of the created NotificationHub
*/
private AdmCredential admCredential;
/*
* The BaiduCredential of the created NotificationHub
*/
private BaiduCredential baiduCredential;
/**
* Creates an instance of PnsCredentialsProperties class.
*/
public PnsCredentialsProperties() {
}
/**
* Get the apnsCredential property: The ApnsCredential of the created NotificationHub.
*
* @return the apnsCredential value.
*/
public ApnsCredential apnsCredential() {
return this.apnsCredential;
}
/**
* Set the apnsCredential property: The ApnsCredential of the created NotificationHub.
*
* @param apnsCredential the apnsCredential value to set.
* @return the PnsCredentialsProperties object itself.
*/
public PnsCredentialsProperties withApnsCredential(ApnsCredential apnsCredential) {
this.apnsCredential = apnsCredential;
return this;
}
/**
* Get the wnsCredential property: The WnsCredential of the created NotificationHub.
*
* @return the wnsCredential value.
*/
public WnsCredential wnsCredential() {
return this.wnsCredential;
}
/**
* Set the wnsCredential property: The WnsCredential of the created NotificationHub.
*
* @param wnsCredential the wnsCredential value to set.
* @return the PnsCredentialsProperties object itself.
*/
public PnsCredentialsProperties withWnsCredential(WnsCredential wnsCredential) {
this.wnsCredential = wnsCredential;
return this;
}
/**
* Get the gcmCredential property: The GcmCredential of the created NotificationHub.
*
* @return the gcmCredential value.
*/
public GcmCredential gcmCredential() {
return this.gcmCredential;
}
/**
* Set the gcmCredential property: The GcmCredential of the created NotificationHub.
*
* @param gcmCredential the gcmCredential value to set.
* @return the PnsCredentialsProperties object itself.
*/
public PnsCredentialsProperties withGcmCredential(GcmCredential gcmCredential) {
this.gcmCredential = gcmCredential;
return this;
}
/**
* Get the mpnsCredential property: The MpnsCredential of the created NotificationHub.
*
* @return the mpnsCredential value.
*/
public MpnsCredential mpnsCredential() {
return this.mpnsCredential;
}
/**
* Set the mpnsCredential property: The MpnsCredential of the created NotificationHub.
*
* @param mpnsCredential the mpnsCredential value to set.
* @return the PnsCredentialsProperties object itself.
*/
public PnsCredentialsProperties withMpnsCredential(MpnsCredential mpnsCredential) {
this.mpnsCredential = mpnsCredential;
return this;
}
/**
* Get the admCredential property: The AdmCredential of the created NotificationHub.
*
* @return the admCredential value.
*/
public AdmCredential admCredential() {
return this.admCredential;
}
/**
* Set the admCredential property: The AdmCredential of the created NotificationHub.
*
* @param admCredential the admCredential value to set.
* @return the PnsCredentialsProperties object itself.
*/
public PnsCredentialsProperties withAdmCredential(AdmCredential admCredential) {
this.admCredential = admCredential;
return this;
}
/**
* Get the baiduCredential property: The BaiduCredential of the created NotificationHub.
*
* @return the baiduCredential value.
*/
public BaiduCredential baiduCredential() {
return this.baiduCredential;
}
/**
* Set the baiduCredential property: The BaiduCredential of the created NotificationHub.
*
* @param baiduCredential the baiduCredential value to set.
* @return the PnsCredentialsProperties object itself.
*/
public PnsCredentialsProperties withBaiduCredential(BaiduCredential baiduCredential) {
this.baiduCredential = baiduCredential;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (apnsCredential() != null) {
apnsCredential().validate();
}
if (wnsCredential() != null) {
wnsCredential().validate();
}
if (gcmCredential() != null) {
gcmCredential().validate();
}
if (mpnsCredential() != null) {
mpnsCredential().validate();
}
if (admCredential() != null) {
admCredential().validate();
}
if (baiduCredential() != null) {
baiduCredential().validate();
}
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeJsonField("apnsCredential", this.apnsCredential);
jsonWriter.writeJsonField("wnsCredential", this.wnsCredential);
jsonWriter.writeJsonField("gcmCredential", this.gcmCredential);
jsonWriter.writeJsonField("mpnsCredential", this.mpnsCredential);
jsonWriter.writeJsonField("admCredential", this.admCredential);
jsonWriter.writeJsonField("baiduCredential", this.baiduCredential);
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of PnsCredentialsProperties from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of PnsCredentialsProperties if the JsonReader was pointing to an instance of it, or null if
* it was pointing to JSON null.
* @throws IOException If an error occurs while reading the PnsCredentialsProperties.
*/
public static PnsCredentialsProperties fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
PnsCredentialsProperties deserializedPnsCredentialsProperties = new PnsCredentialsProperties();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("apnsCredential".equals(fieldName)) {
deserializedPnsCredentialsProperties.apnsCredential = ApnsCredential.fromJson(reader);
} else if ("wnsCredential".equals(fieldName)) {
deserializedPnsCredentialsProperties.wnsCredential = WnsCredential.fromJson(reader);
} else if ("gcmCredential".equals(fieldName)) {
deserializedPnsCredentialsProperties.gcmCredential = GcmCredential.fromJson(reader);
} else if ("mpnsCredential".equals(fieldName)) {
deserializedPnsCredentialsProperties.mpnsCredential = MpnsCredential.fromJson(reader);
} else if ("admCredential".equals(fieldName)) {
deserializedPnsCredentialsProperties.admCredential = AdmCredential.fromJson(reader);
} else if ("baiduCredential".equals(fieldName)) {
deserializedPnsCredentialsProperties.baiduCredential = BaiduCredential.fromJson(reader);
} else {
reader.skipChildren();
}
}
return deserializedPnsCredentialsProperties;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy