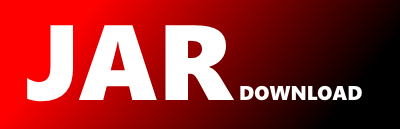
com.azure.resourcemanager.orbital.fluent.SpacecraftsClient Maven / Gradle / Ivy
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.orbital.fluent;
import com.azure.core.annotation.ReturnType;
import com.azure.core.annotation.ServiceMethod;
import com.azure.core.http.rest.PagedIterable;
import com.azure.core.http.rest.Response;
import com.azure.core.management.polling.PollResult;
import com.azure.core.util.Context;
import com.azure.core.util.polling.SyncPoller;
import com.azure.resourcemanager.orbital.fluent.models.AvailableContactsInner;
import com.azure.resourcemanager.orbital.fluent.models.SpacecraftInner;
import com.azure.resourcemanager.orbital.models.ContactParameters;
import com.azure.resourcemanager.orbital.models.TagsObject;
/**
* An instance of this class provides access to all the operations defined in SpacecraftsClient.
*/
public interface SpacecraftsClient {
/**
* Returns list of spacecrafts by subscription.
*
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return response for the ListSpacecrafts API service call as paginated response with {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
PagedIterable list();
/**
* Returns list of spacecrafts by subscription.
*
* @param skiptoken An opaque string that the resource provider uses to skip over previously-returned results. This
* is used when a previous list operation call returned a partial result. If a previous response contains a nextLink
* element, the value of the nextLink element will include a skiptoken parameter that specifies a starting point to
* use for subsequent calls.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return response for the ListSpacecrafts API service call as paginated response with {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
PagedIterable list(String skiptoken, Context context);
/**
* Returns list of spacecrafts by resource group.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return response for the ListSpacecrafts API service call as paginated response with {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
PagedIterable listByResourceGroup(String resourceGroupName);
/**
* Returns list of spacecrafts by resource group.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param skiptoken An opaque string that the resource provider uses to skip over previously-returned results. This
* is used when a previous list operation call returned a partial result. If a previous response contains a nextLink
* element, the value of the nextLink element will include a skiptoken parameter that specifies a starting point to
* use for subsequent calls.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return response for the ListSpacecrafts API service call as paginated response with {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
PagedIterable listByResourceGroup(String resourceGroupName, String skiptoken, Context context);
/**
* Gets the specified spacecraft in a specified resource group.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param spacecraftName Spacecraft ID.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the specified spacecraft in a specified resource group along with {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Response getByResourceGroupWithResponse(String resourceGroupName, String spacecraftName,
Context context);
/**
* Gets the specified spacecraft in a specified resource group.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param spacecraftName Spacecraft ID.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the specified spacecraft in a specified resource group.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
SpacecraftInner getByResourceGroup(String resourceGroupName, String spacecraftName);
/**
* Creates or updates a spacecraft resource.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param spacecraftName Spacecraft ID.
* @param parameters The parameters to provide for the created spacecraft.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of customer creates a spacecraft resource to schedule a contact.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, SpacecraftInner> beginCreateOrUpdate(String resourceGroupName,
String spacecraftName, SpacecraftInner parameters);
/**
* Creates or updates a spacecraft resource.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param spacecraftName Spacecraft ID.
* @param parameters The parameters to provide for the created spacecraft.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of customer creates a spacecraft resource to schedule a contact.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, SpacecraftInner> beginCreateOrUpdate(String resourceGroupName,
String spacecraftName, SpacecraftInner parameters, Context context);
/**
* Creates or updates a spacecraft resource.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param spacecraftName Spacecraft ID.
* @param parameters The parameters to provide for the created spacecraft.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return customer creates a spacecraft resource to schedule a contact.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
SpacecraftInner createOrUpdate(String resourceGroupName, String spacecraftName, SpacecraftInner parameters);
/**
* Creates or updates a spacecraft resource.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param spacecraftName Spacecraft ID.
* @param parameters The parameters to provide for the created spacecraft.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return customer creates a spacecraft resource to schedule a contact.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
SpacecraftInner createOrUpdate(String resourceGroupName, String spacecraftName, SpacecraftInner parameters,
Context context);
/**
* Deletes a specified spacecraft resource.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param spacecraftName Spacecraft ID.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, Void> beginDelete(String resourceGroupName, String spacecraftName);
/**
* Deletes a specified spacecraft resource.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param spacecraftName Spacecraft ID.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, Void> beginDelete(String resourceGroupName, String spacecraftName, Context context);
/**
* Deletes a specified spacecraft resource.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param spacecraftName Spacecraft ID.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
void delete(String resourceGroupName, String spacecraftName);
/**
* Deletes a specified spacecraft resource.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param spacecraftName Spacecraft ID.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
void delete(String resourceGroupName, String spacecraftName, Context context);
/**
* Updates the specified spacecraft tags.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param spacecraftName Spacecraft ID.
* @param parameters Parameters supplied to update spacecraft tags.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of customer creates a spacecraft resource to schedule a contact.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, SpacecraftInner> beginUpdateTags(String resourceGroupName,
String spacecraftName, TagsObject parameters);
/**
* Updates the specified spacecraft tags.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param spacecraftName Spacecraft ID.
* @param parameters Parameters supplied to update spacecraft tags.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of customer creates a spacecraft resource to schedule a contact.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, SpacecraftInner> beginUpdateTags(String resourceGroupName,
String spacecraftName, TagsObject parameters, Context context);
/**
* Updates the specified spacecraft tags.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param spacecraftName Spacecraft ID.
* @param parameters Parameters supplied to update spacecraft tags.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return customer creates a spacecraft resource to schedule a contact.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
SpacecraftInner updateTags(String resourceGroupName, String spacecraftName, TagsObject parameters);
/**
* Updates the specified spacecraft tags.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param spacecraftName Spacecraft ID.
* @param parameters Parameters supplied to update spacecraft tags.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return customer creates a spacecraft resource to schedule a contact.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
SpacecraftInner updateTags(String resourceGroupName, String spacecraftName, TagsObject parameters, Context context);
/**
* Returns list of available contacts. A contact is available if the spacecraft is visible from the ground station
* for more than the minimum viable contact duration provided in the contact profile.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param spacecraftName Spacecraft ID.
* @param parameters The parameters to provide for the contacts.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return response for the ListAvailableContacts API service call as paginated response with {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
PagedIterable listAvailableContacts(String resourceGroupName, String spacecraftName,
ContactParameters parameters);
/**
* Returns list of available contacts. A contact is available if the spacecraft is visible from the ground station
* for more than the minimum viable contact duration provided in the contact profile.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param spacecraftName Spacecraft ID.
* @param parameters The parameters to provide for the contacts.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return response for the ListAvailableContacts API service call as paginated response with {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
PagedIterable listAvailableContacts(String resourceGroupName, String spacecraftName,
ContactParameters parameters, Context context);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy