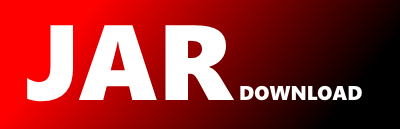
com.azure.resourcemanager.orbital.models.Contact Maven / Gradle / Ivy
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.orbital.models;
import com.azure.core.management.SystemData;
import com.azure.core.util.Context;
import com.azure.resourcemanager.orbital.fluent.models.ContactInner;
import java.time.OffsetDateTime;
/**
* An immutable client-side representation of Contact.
*/
public interface Contact {
/**
* Gets the id property: Fully qualified resource Id for the resource.
*
* @return the id value.
*/
String id();
/**
* Gets the name property: The name of the resource.
*
* @return the name value.
*/
String name();
/**
* Gets the type property: The type of the resource.
*
* @return the type value.
*/
String type();
/**
* Gets the systemData property: Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
* @return the systemData value.
*/
SystemData systemData();
/**
* Gets the provisioningState property: The current state of the resource's creation, deletion, or modification.
*
* @return the provisioningState value.
*/
ContactsPropertiesProvisioningState provisioningState();
/**
* Gets the status property: Status of a contact.
*
* @return the status value.
*/
ContactsStatus status();
/**
* Gets the reservationStartTime property: Reservation start time of a contact (ISO 8601 UTC standard).
*
* @return the reservationStartTime value.
*/
OffsetDateTime reservationStartTime();
/**
* Gets the reservationEndTime property: Reservation end time of a contact (ISO 8601 UTC standard).
*
* @return the reservationEndTime value.
*/
OffsetDateTime reservationEndTime();
/**
* Gets the rxStartTime property: Receive start time of a contact (ISO 8601 UTC standard).
*
* @return the rxStartTime value.
*/
OffsetDateTime rxStartTime();
/**
* Gets the rxEndTime property: Receive end time of a contact (ISO 8601 UTC standard).
*
* @return the rxEndTime value.
*/
OffsetDateTime rxEndTime();
/**
* Gets the txStartTime property: Transmit start time of a contact (ISO 8601 UTC standard).
*
* @return the txStartTime value.
*/
OffsetDateTime txStartTime();
/**
* Gets the txEndTime property: Transmit end time of a contact (ISO 8601 UTC standard).
*
* @return the txEndTime value.
*/
OffsetDateTime txEndTime();
/**
* Gets the errorMessage property: Any error message while scheduling a contact.
*
* @return the errorMessage value.
*/
String errorMessage();
/**
* Gets the maximumElevationDegrees property: Maximum elevation of the antenna during the contact in decimal
* degrees.
*
* @return the maximumElevationDegrees value.
*/
Float maximumElevationDegrees();
/**
* Gets the startAzimuthDegrees property: Azimuth of the antenna at the start of the contact in decimal degrees.
*
* @return the startAzimuthDegrees value.
*/
Float startAzimuthDegrees();
/**
* Gets the endAzimuthDegrees property: Azimuth of the antenna at the end of the contact in decimal degrees.
*
* @return the endAzimuthDegrees value.
*/
Float endAzimuthDegrees();
/**
* Gets the groundStationName property: Azure Ground Station name.
*
* @return the groundStationName value.
*/
String groundStationName();
/**
* Gets the startElevationDegrees property: Spacecraft elevation above the horizon at contact start.
*
* @return the startElevationDegrees value.
*/
Float startElevationDegrees();
/**
* Gets the endElevationDegrees property: Spacecraft elevation above the horizon at contact end.
*
* @return the endElevationDegrees value.
*/
Float endElevationDegrees();
/**
* Gets the antennaConfiguration property: The configuration associated with the allocated antenna.
*
* @return the antennaConfiguration value.
*/
ContactsPropertiesAntennaConfiguration antennaConfiguration();
/**
* Gets the contactProfile property: The reference to the contact profile resource.
*
* @return the contactProfile value.
*/
ContactsPropertiesContactProfile contactProfile();
/**
* Gets the inner com.azure.resourcemanager.orbital.fluent.models.ContactInner object.
*
* @return the inner object.
*/
ContactInner innerModel();
/**
* The entirety of the Contact definition.
*/
interface Definition extends DefinitionStages.Blank, DefinitionStages.WithParentResource,
DefinitionStages.WithReservationStartTime, DefinitionStages.WithReservationEndTime,
DefinitionStages.WithGroundStationName, DefinitionStages.WithContactProfile, DefinitionStages.WithCreate {
}
/**
* The Contact definition stages.
*/
interface DefinitionStages {
/**
* The first stage of the Contact definition.
*/
interface Blank extends WithParentResource {
}
/**
* The stage of the Contact definition allowing to specify parent resource.
*/
interface WithParentResource {
/**
* Specifies resourceGroupName, spacecraftName.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param spacecraftName Spacecraft ID.
* @return the next definition stage.
*/
WithReservationStartTime withExistingSpacecraft(String resourceGroupName, String spacecraftName);
}
/**
* The stage of the Contact definition allowing to specify reservationStartTime.
*/
interface WithReservationStartTime {
/**
* Specifies the reservationStartTime property: Reservation start time of a contact (ISO 8601 UTC
* standard)..
*
* @param reservationStartTime Reservation start time of a contact (ISO 8601 UTC standard).
* @return the next definition stage.
*/
WithReservationEndTime withReservationStartTime(OffsetDateTime reservationStartTime);
}
/**
* The stage of the Contact definition allowing to specify reservationEndTime.
*/
interface WithReservationEndTime {
/**
* Specifies the reservationEndTime property: Reservation end time of a contact (ISO 8601 UTC standard)..
*
* @param reservationEndTime Reservation end time of a contact (ISO 8601 UTC standard).
* @return the next definition stage.
*/
WithGroundStationName withReservationEndTime(OffsetDateTime reservationEndTime);
}
/**
* The stage of the Contact definition allowing to specify groundStationName.
*/
interface WithGroundStationName {
/**
* Specifies the groundStationName property: Azure Ground Station name..
*
* @param groundStationName Azure Ground Station name.
* @return the next definition stage.
*/
WithContactProfile withGroundStationName(String groundStationName);
}
/**
* The stage of the Contact definition allowing to specify contactProfile.
*/
interface WithContactProfile {
/**
* Specifies the contactProfile property: The reference to the contact profile resource..
*
* @param contactProfile The reference to the contact profile resource.
* @return the next definition stage.
*/
WithCreate withContactProfile(ContactsPropertiesContactProfile contactProfile);
}
/**
* The stage of the Contact definition which contains all the minimum required properties for the resource to be
* created, but also allows for any other optional properties to be specified.
*/
interface WithCreate extends DefinitionStages.WithProvisioningState {
/**
* Executes the create request.
*
* @return the created resource.
*/
Contact create();
/**
* Executes the create request.
*
* @param context The context to associate with this operation.
* @return the created resource.
*/
Contact create(Context context);
}
/**
* The stage of the Contact definition allowing to specify provisioningState.
*/
interface WithProvisioningState {
/**
* Specifies the provisioningState property: The current state of the resource's creation, deletion, or
* modification..
*
* @param provisioningState The current state of the resource's creation, deletion, or modification.
* @return the next definition stage.
*/
WithCreate withProvisioningState(ContactsPropertiesProvisioningState provisioningState);
}
}
/**
* Refreshes the resource to sync with Azure.
*
* @return the refreshed resource.
*/
Contact refresh();
/**
* Refreshes the resource to sync with Azure.
*
* @param context The context to associate with this operation.
* @return the refreshed resource.
*/
Contact refresh(Context context);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy