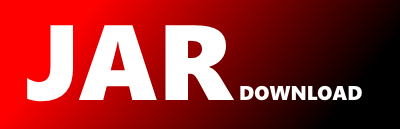
com.azure.resourcemanager.recoveryservicesbackup.fluent.models.BackupStatusResponseInner Maven / Gradle / Ivy
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.recoveryservicesbackup.fluent.models;
import com.azure.core.annotation.Fluent;
import com.azure.resourcemanager.recoveryservicesbackup.models.AcquireStorageAccountLock;
import com.azure.resourcemanager.recoveryservicesbackup.models.FabricName;
import com.azure.resourcemanager.recoveryservicesbackup.models.ProtectionStatus;
import com.fasterxml.jackson.annotation.JsonProperty;
/**
* BackupStatus response.
*/
@Fluent
public final class BackupStatusResponseInner {
/*
* Specifies whether the container is registered or not
*/
@JsonProperty(value = "protectionStatus")
private ProtectionStatus protectionStatus;
/*
* Specifies the arm resource id of the vault
*/
@JsonProperty(value = "vaultId")
private String vaultId;
/*
* Specifies the fabric name - Azure or AD
*/
@JsonProperty(value = "fabricName")
private FabricName fabricName;
/*
* Specifies the product specific container name. E.g. iaasvmcontainer;iaasvmcontainer;csname;vmname.
*/
@JsonProperty(value = "containerName")
private String containerName;
/*
* Specifies the product specific ds name. E.g. vm;iaasvmcontainer;csname;vmname.
*/
@JsonProperty(value = "protectedItemName")
private String protectedItemName;
/*
* ErrorCode in case of intent failed
*/
@JsonProperty(value = "errorCode")
private String errorCode;
/*
* ErrorMessage in case of intent failed.
*/
@JsonProperty(value = "errorMessage")
private String errorMessage;
/*
* Specifies the policy name which is used for protection
*/
@JsonProperty(value = "policyName")
private String policyName;
/*
* Container registration status
*/
@JsonProperty(value = "registrationStatus")
private String registrationStatus;
/*
* Number of protected items
*/
@JsonProperty(value = "protectedItemsCount")
private Integer protectedItemsCount;
/*
* Specifies whether the storage account lock has been acquired or not
*/
@JsonProperty(value = "acquireStorageAccountLock")
private AcquireStorageAccountLock acquireStorageAccountLock;
/**
* Creates an instance of BackupStatusResponseInner class.
*/
public BackupStatusResponseInner() {
}
/**
* Get the protectionStatus property: Specifies whether the container is registered or not.
*
* @return the protectionStatus value.
*/
public ProtectionStatus protectionStatus() {
return this.protectionStatus;
}
/**
* Set the protectionStatus property: Specifies whether the container is registered or not.
*
* @param protectionStatus the protectionStatus value to set.
* @return the BackupStatusResponseInner object itself.
*/
public BackupStatusResponseInner withProtectionStatus(ProtectionStatus protectionStatus) {
this.protectionStatus = protectionStatus;
return this;
}
/**
* Get the vaultId property: Specifies the arm resource id of the vault.
*
* @return the vaultId value.
*/
public String vaultId() {
return this.vaultId;
}
/**
* Set the vaultId property: Specifies the arm resource id of the vault.
*
* @param vaultId the vaultId value to set.
* @return the BackupStatusResponseInner object itself.
*/
public BackupStatusResponseInner withVaultId(String vaultId) {
this.vaultId = vaultId;
return this;
}
/**
* Get the fabricName property: Specifies the fabric name - Azure or AD.
*
* @return the fabricName value.
*/
public FabricName fabricName() {
return this.fabricName;
}
/**
* Set the fabricName property: Specifies the fabric name - Azure or AD.
*
* @param fabricName the fabricName value to set.
* @return the BackupStatusResponseInner object itself.
*/
public BackupStatusResponseInner withFabricName(FabricName fabricName) {
this.fabricName = fabricName;
return this;
}
/**
* Get the containerName property: Specifies the product specific container name. E.g.
* iaasvmcontainer;iaasvmcontainer;csname;vmname.
*
* @return the containerName value.
*/
public String containerName() {
return this.containerName;
}
/**
* Set the containerName property: Specifies the product specific container name. E.g.
* iaasvmcontainer;iaasvmcontainer;csname;vmname.
*
* @param containerName the containerName value to set.
* @return the BackupStatusResponseInner object itself.
*/
public BackupStatusResponseInner withContainerName(String containerName) {
this.containerName = containerName;
return this;
}
/**
* Get the protectedItemName property: Specifies the product specific ds name. E.g.
* vm;iaasvmcontainer;csname;vmname.
*
* @return the protectedItemName value.
*/
public String protectedItemName() {
return this.protectedItemName;
}
/**
* Set the protectedItemName property: Specifies the product specific ds name. E.g.
* vm;iaasvmcontainer;csname;vmname.
*
* @param protectedItemName the protectedItemName value to set.
* @return the BackupStatusResponseInner object itself.
*/
public BackupStatusResponseInner withProtectedItemName(String protectedItemName) {
this.protectedItemName = protectedItemName;
return this;
}
/**
* Get the errorCode property: ErrorCode in case of intent failed.
*
* @return the errorCode value.
*/
public String errorCode() {
return this.errorCode;
}
/**
* Set the errorCode property: ErrorCode in case of intent failed.
*
* @param errorCode the errorCode value to set.
* @return the BackupStatusResponseInner object itself.
*/
public BackupStatusResponseInner withErrorCode(String errorCode) {
this.errorCode = errorCode;
return this;
}
/**
* Get the errorMessage property: ErrorMessage in case of intent failed.
*
* @return the errorMessage value.
*/
public String errorMessage() {
return this.errorMessage;
}
/**
* Set the errorMessage property: ErrorMessage in case of intent failed.
*
* @param errorMessage the errorMessage value to set.
* @return the BackupStatusResponseInner object itself.
*/
public BackupStatusResponseInner withErrorMessage(String errorMessage) {
this.errorMessage = errorMessage;
return this;
}
/**
* Get the policyName property: Specifies the policy name which is used for protection.
*
* @return the policyName value.
*/
public String policyName() {
return this.policyName;
}
/**
* Set the policyName property: Specifies the policy name which is used for protection.
*
* @param policyName the policyName value to set.
* @return the BackupStatusResponseInner object itself.
*/
public BackupStatusResponseInner withPolicyName(String policyName) {
this.policyName = policyName;
return this;
}
/**
* Get the registrationStatus property: Container registration status.
*
* @return the registrationStatus value.
*/
public String registrationStatus() {
return this.registrationStatus;
}
/**
* Set the registrationStatus property: Container registration status.
*
* @param registrationStatus the registrationStatus value to set.
* @return the BackupStatusResponseInner object itself.
*/
public BackupStatusResponseInner withRegistrationStatus(String registrationStatus) {
this.registrationStatus = registrationStatus;
return this;
}
/**
* Get the protectedItemsCount property: Number of protected items.
*
* @return the protectedItemsCount value.
*/
public Integer protectedItemsCount() {
return this.protectedItemsCount;
}
/**
* Set the protectedItemsCount property: Number of protected items.
*
* @param protectedItemsCount the protectedItemsCount value to set.
* @return the BackupStatusResponseInner object itself.
*/
public BackupStatusResponseInner withProtectedItemsCount(Integer protectedItemsCount) {
this.protectedItemsCount = protectedItemsCount;
return this;
}
/**
* Get the acquireStorageAccountLock property: Specifies whether the storage account lock has been acquired or not.
*
* @return the acquireStorageAccountLock value.
*/
public AcquireStorageAccountLock acquireStorageAccountLock() {
return this.acquireStorageAccountLock;
}
/**
* Set the acquireStorageAccountLock property: Specifies whether the storage account lock has been acquired or not.
*
* @param acquireStorageAccountLock the acquireStorageAccountLock value to set.
* @return the BackupStatusResponseInner object itself.
*/
public BackupStatusResponseInner
withAcquireStorageAccountLock(AcquireStorageAccountLock acquireStorageAccountLock) {
this.acquireStorageAccountLock = acquireStorageAccountLock;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy