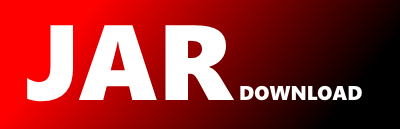
com.azure.resourcemanager.recoveryservicesbackup.fluent.models.PreValidateEnableBackupResponseInner Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-recoveryservicesbackup Show documentation
Show all versions of azure-resourcemanager-recoveryservicesbackup Show documentation
This package contains Microsoft Azure SDK for RecoveryServicesBackup Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. Open API 2.0 Specs for Azure RecoveryServices Backup service. Package tag package-2024-04.
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.recoveryservicesbackup.fluent.models;
import com.azure.core.annotation.Fluent;
import com.azure.resourcemanager.recoveryservicesbackup.models.ValidationStatus;
import com.fasterxml.jackson.annotation.JsonProperty;
/**
* Response contract for enable backup validation request.
*/
@Fluent
public final class PreValidateEnableBackupResponseInner {
/*
* Validation Status
*/
@JsonProperty(value = "status")
private ValidationStatus status;
/*
* Response error code
*/
@JsonProperty(value = "errorCode")
private String errorCode;
/*
* Response error message
*/
@JsonProperty(value = "errorMessage")
private String errorMessage;
/*
* Recommended action for user
*/
@JsonProperty(value = "recommendation")
private String recommendation;
/*
* Specifies the product specific container name. E.g. iaasvmcontainer;iaasvmcontainer;rgname;vmname. This is
* required
* for portal
*/
@JsonProperty(value = "containerName")
private String containerName;
/*
* Specifies the product specific ds name. E.g. vm;iaasvmcontainer;rgname;vmname. This is required for portal
*/
@JsonProperty(value = "protectedItemName")
private String protectedItemName;
/**
* Creates an instance of PreValidateEnableBackupResponseInner class.
*/
public PreValidateEnableBackupResponseInner() {
}
/**
* Get the status property: Validation Status.
*
* @return the status value.
*/
public ValidationStatus status() {
return this.status;
}
/**
* Set the status property: Validation Status.
*
* @param status the status value to set.
* @return the PreValidateEnableBackupResponseInner object itself.
*/
public PreValidateEnableBackupResponseInner withStatus(ValidationStatus status) {
this.status = status;
return this;
}
/**
* Get the errorCode property: Response error code.
*
* @return the errorCode value.
*/
public String errorCode() {
return this.errorCode;
}
/**
* Set the errorCode property: Response error code.
*
* @param errorCode the errorCode value to set.
* @return the PreValidateEnableBackupResponseInner object itself.
*/
public PreValidateEnableBackupResponseInner withErrorCode(String errorCode) {
this.errorCode = errorCode;
return this;
}
/**
* Get the errorMessage property: Response error message.
*
* @return the errorMessage value.
*/
public String errorMessage() {
return this.errorMessage;
}
/**
* Set the errorMessage property: Response error message.
*
* @param errorMessage the errorMessage value to set.
* @return the PreValidateEnableBackupResponseInner object itself.
*/
public PreValidateEnableBackupResponseInner withErrorMessage(String errorMessage) {
this.errorMessage = errorMessage;
return this;
}
/**
* Get the recommendation property: Recommended action for user.
*
* @return the recommendation value.
*/
public String recommendation() {
return this.recommendation;
}
/**
* Set the recommendation property: Recommended action for user.
*
* @param recommendation the recommendation value to set.
* @return the PreValidateEnableBackupResponseInner object itself.
*/
public PreValidateEnableBackupResponseInner withRecommendation(String recommendation) {
this.recommendation = recommendation;
return this;
}
/**
* Get the containerName property: Specifies the product specific container name. E.g.
* iaasvmcontainer;iaasvmcontainer;rgname;vmname. This is required
* for portal.
*
* @return the containerName value.
*/
public String containerName() {
return this.containerName;
}
/**
* Set the containerName property: Specifies the product specific container name. E.g.
* iaasvmcontainer;iaasvmcontainer;rgname;vmname. This is required
* for portal.
*
* @param containerName the containerName value to set.
* @return the PreValidateEnableBackupResponseInner object itself.
*/
public PreValidateEnableBackupResponseInner withContainerName(String containerName) {
this.containerName = containerName;
return this;
}
/**
* Get the protectedItemName property: Specifies the product specific ds name. E.g.
* vm;iaasvmcontainer;rgname;vmname. This is required for portal.
*
* @return the protectedItemName value.
*/
public String protectedItemName() {
return this.protectedItemName;
}
/**
* Set the protectedItemName property: Specifies the product specific ds name. E.g.
* vm;iaasvmcontainer;rgname;vmname. This is required for portal.
*
* @param protectedItemName the protectedItemName value to set.
* @return the PreValidateEnableBackupResponseInner object itself.
*/
public PreValidateEnableBackupResponseInner withProtectedItemName(String protectedItemName) {
this.protectedItemName = protectedItemName;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy