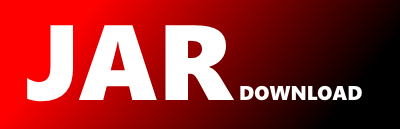
com.azure.resourcemanager.recoveryservicesbackup.models.AzureFileShareProtectionPolicy Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-recoveryservicesbackup Show documentation
Show all versions of azure-resourcemanager-recoveryservicesbackup Show documentation
This package contains Microsoft Azure SDK for RecoveryServicesBackup Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. Open API 2.0 Specs for Azure RecoveryServices Backup service. Package tag package-2024-04.
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.recoveryservicesbackup.models;
import com.azure.core.annotation.Fluent;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonTypeInfo;
import com.fasterxml.jackson.annotation.JsonTypeName;
import java.util.List;
/**
* AzureStorage backup policy.
*/
@JsonTypeInfo(use = JsonTypeInfo.Id.NAME, include = JsonTypeInfo.As.PROPERTY, property = "backupManagementType")
@JsonTypeName("AzureStorage")
@Fluent
public final class AzureFileShareProtectionPolicy extends ProtectionPolicy {
/*
* Type of workload for the backup management
*/
@JsonProperty(value = "workLoadType")
private WorkloadType workLoadType;
/*
* Backup schedule specified as part of backup policy.
*/
@JsonProperty(value = "schedulePolicy")
private SchedulePolicy schedulePolicy;
/*
* Retention policy with the details on backup copy retention ranges.
*/
@JsonProperty(value = "retentionPolicy")
private RetentionPolicy retentionPolicy;
/*
* Retention policy with the details on hardened backup copy retention ranges.
*/
@JsonProperty(value = "vaultRetentionPolicy")
private VaultRetentionPolicy vaultRetentionPolicy;
/*
* TimeZone optional input as string. For example: TimeZone = "Pacific Standard Time".
*/
@JsonProperty(value = "timeZone")
private String timeZone;
/**
* Creates an instance of AzureFileShareProtectionPolicy class.
*/
public AzureFileShareProtectionPolicy() {
}
/**
* Get the workLoadType property: Type of workload for the backup management.
*
* @return the workLoadType value.
*/
public WorkloadType workLoadType() {
return this.workLoadType;
}
/**
* Set the workLoadType property: Type of workload for the backup management.
*
* @param workLoadType the workLoadType value to set.
* @return the AzureFileShareProtectionPolicy object itself.
*/
public AzureFileShareProtectionPolicy withWorkLoadType(WorkloadType workLoadType) {
this.workLoadType = workLoadType;
return this;
}
/**
* Get the schedulePolicy property: Backup schedule specified as part of backup policy.
*
* @return the schedulePolicy value.
*/
public SchedulePolicy schedulePolicy() {
return this.schedulePolicy;
}
/**
* Set the schedulePolicy property: Backup schedule specified as part of backup policy.
*
* @param schedulePolicy the schedulePolicy value to set.
* @return the AzureFileShareProtectionPolicy object itself.
*/
public AzureFileShareProtectionPolicy withSchedulePolicy(SchedulePolicy schedulePolicy) {
this.schedulePolicy = schedulePolicy;
return this;
}
/**
* Get the retentionPolicy property: Retention policy with the details on backup copy retention ranges.
*
* @return the retentionPolicy value.
*/
public RetentionPolicy retentionPolicy() {
return this.retentionPolicy;
}
/**
* Set the retentionPolicy property: Retention policy with the details on backup copy retention ranges.
*
* @param retentionPolicy the retentionPolicy value to set.
* @return the AzureFileShareProtectionPolicy object itself.
*/
public AzureFileShareProtectionPolicy withRetentionPolicy(RetentionPolicy retentionPolicy) {
this.retentionPolicy = retentionPolicy;
return this;
}
/**
* Get the vaultRetentionPolicy property: Retention policy with the details on hardened backup copy retention
* ranges.
*
* @return the vaultRetentionPolicy value.
*/
public VaultRetentionPolicy vaultRetentionPolicy() {
return this.vaultRetentionPolicy;
}
/**
* Set the vaultRetentionPolicy property: Retention policy with the details on hardened backup copy retention
* ranges.
*
* @param vaultRetentionPolicy the vaultRetentionPolicy value to set.
* @return the AzureFileShareProtectionPolicy object itself.
*/
public AzureFileShareProtectionPolicy withVaultRetentionPolicy(VaultRetentionPolicy vaultRetentionPolicy) {
this.vaultRetentionPolicy = vaultRetentionPolicy;
return this;
}
/**
* Get the timeZone property: TimeZone optional input as string. For example: TimeZone = "Pacific Standard Time".
*
* @return the timeZone value.
*/
public String timeZone() {
return this.timeZone;
}
/**
* Set the timeZone property: TimeZone optional input as string. For example: TimeZone = "Pacific Standard Time".
*
* @param timeZone the timeZone value to set.
* @return the AzureFileShareProtectionPolicy object itself.
*/
public AzureFileShareProtectionPolicy withTimeZone(String timeZone) {
this.timeZone = timeZone;
return this;
}
/**
* {@inheritDoc}
*/
@Override
public AzureFileShareProtectionPolicy withProtectedItemsCount(Integer protectedItemsCount) {
super.withProtectedItemsCount(protectedItemsCount);
return this;
}
/**
* {@inheritDoc}
*/
@Override
public AzureFileShareProtectionPolicy
withResourceGuardOperationRequests(List resourceGuardOperationRequests) {
super.withResourceGuardOperationRequests(resourceGuardOperationRequests);
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
@Override
public void validate() {
super.validate();
if (schedulePolicy() != null) {
schedulePolicy().validate();
}
if (retentionPolicy() != null) {
retentionPolicy().validate();
}
if (vaultRetentionPolicy() != null) {
vaultRetentionPolicy().validate();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy