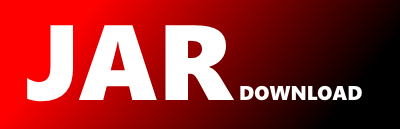
com.azure.resourcemanager.recoveryservicesbackup.models.AzureVmWorkloadProtectableItem Maven / Gradle / Ivy
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.recoveryservicesbackup.models;
import com.azure.core.annotation.Fluent;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonSubTypes;
import com.fasterxml.jackson.annotation.JsonTypeInfo;
import com.fasterxml.jackson.annotation.JsonTypeName;
/**
* Azure VM workload-specific protectable item.
*/
@JsonTypeInfo(
use = JsonTypeInfo.Id.NAME,
include = JsonTypeInfo.As.PROPERTY,
property = "protectableItemType",
defaultImpl = AzureVmWorkloadProtectableItem.class)
@JsonTypeName("AzureVmWorkloadProtectableItem")
@JsonSubTypes({
@JsonSubTypes.Type(name = "SAPAseSystem", value = AzureVmWorkloadSapAseSystemProtectableItem.class),
@JsonSubTypes.Type(name = "SAPHanaDatabase", value = AzureVmWorkloadSapHanaDatabaseProtectableItem.class),
@JsonSubTypes.Type(name = "SAPHanaSystem", value = AzureVmWorkloadSapHanaSystemProtectableItem.class),
@JsonSubTypes.Type(name = "SAPHanaDBInstance", value = AzureVmWorkloadSapHanaDBInstance.class),
@JsonSubTypes.Type(name = "HanaHSRContainer", value = AzureVmWorkloadSapHanaHsr.class),
@JsonSubTypes.Type(
name = "SQLAvailabilityGroupContainer",
value = AzureVmWorkloadSqlAvailabilityGroupProtectableItem.class),
@JsonSubTypes.Type(name = "SQLDataBase", value = AzureVmWorkloadSqlDatabaseProtectableItem.class),
@JsonSubTypes.Type(name = "SQLInstance", value = AzureVmWorkloadSqlInstanceProtectableItem.class) })
@Fluent
public class AzureVmWorkloadProtectableItem extends WorkloadProtectableItem {
/*
* Name for instance or AG
*/
@JsonProperty(value = "parentName")
private String parentName;
/*
* Parent Unique Name is added to provide the service formatted URI Name of the Parent
* Only Applicable for data bases where the parent would be either Instance or a SQL AG.
*/
@JsonProperty(value = "parentUniqueName")
private String parentUniqueName;
/*
* Host/Cluster Name for instance or AG
*/
@JsonProperty(value = "serverName")
private String serverName;
/*
* Indicates if protectable item is auto-protectable
*/
@JsonProperty(value = "isAutoProtectable")
private Boolean isAutoProtectable;
/*
* Indicates if protectable item is auto-protected
*/
@JsonProperty(value = "isAutoProtected")
private Boolean isAutoProtected;
/*
* For instance or AG, indicates number of DB's present
*/
@JsonProperty(value = "subinquireditemcount")
private Integer subinquireditemcount;
/*
* For instance or AG, indicates number of DB's to be protected
*/
@JsonProperty(value = "subprotectableitemcount")
private Integer subprotectableitemcount;
/*
* Pre-backup validation for protectable objects
*/
@JsonProperty(value = "prebackupvalidation")
private PreBackupValidation prebackupvalidation;
/*
* Indicates if item is protectable
*/
@JsonProperty(value = "isProtectable")
private Boolean isProtectable;
/**
* Creates an instance of AzureVmWorkloadProtectableItem class.
*/
public AzureVmWorkloadProtectableItem() {
}
/**
* Get the parentName property: Name for instance or AG.
*
* @return the parentName value.
*/
public String parentName() {
return this.parentName;
}
/**
* Set the parentName property: Name for instance or AG.
*
* @param parentName the parentName value to set.
* @return the AzureVmWorkloadProtectableItem object itself.
*/
public AzureVmWorkloadProtectableItem withParentName(String parentName) {
this.parentName = parentName;
return this;
}
/**
* Get the parentUniqueName property: Parent Unique Name is added to provide the service formatted URI Name of the
* Parent
* Only Applicable for data bases where the parent would be either Instance or a SQL AG.
*
* @return the parentUniqueName value.
*/
public String parentUniqueName() {
return this.parentUniqueName;
}
/**
* Set the parentUniqueName property: Parent Unique Name is added to provide the service formatted URI Name of the
* Parent
* Only Applicable for data bases where the parent would be either Instance or a SQL AG.
*
* @param parentUniqueName the parentUniqueName value to set.
* @return the AzureVmWorkloadProtectableItem object itself.
*/
public AzureVmWorkloadProtectableItem withParentUniqueName(String parentUniqueName) {
this.parentUniqueName = parentUniqueName;
return this;
}
/**
* Get the serverName property: Host/Cluster Name for instance or AG.
*
* @return the serverName value.
*/
public String serverName() {
return this.serverName;
}
/**
* Set the serverName property: Host/Cluster Name for instance or AG.
*
* @param serverName the serverName value to set.
* @return the AzureVmWorkloadProtectableItem object itself.
*/
public AzureVmWorkloadProtectableItem withServerName(String serverName) {
this.serverName = serverName;
return this;
}
/**
* Get the isAutoProtectable property: Indicates if protectable item is auto-protectable.
*
* @return the isAutoProtectable value.
*/
public Boolean isAutoProtectable() {
return this.isAutoProtectable;
}
/**
* Set the isAutoProtectable property: Indicates if protectable item is auto-protectable.
*
* @param isAutoProtectable the isAutoProtectable value to set.
* @return the AzureVmWorkloadProtectableItem object itself.
*/
public AzureVmWorkloadProtectableItem withIsAutoProtectable(Boolean isAutoProtectable) {
this.isAutoProtectable = isAutoProtectable;
return this;
}
/**
* Get the isAutoProtected property: Indicates if protectable item is auto-protected.
*
* @return the isAutoProtected value.
*/
public Boolean isAutoProtected() {
return this.isAutoProtected;
}
/**
* Set the isAutoProtected property: Indicates if protectable item is auto-protected.
*
* @param isAutoProtected the isAutoProtected value to set.
* @return the AzureVmWorkloadProtectableItem object itself.
*/
public AzureVmWorkloadProtectableItem withIsAutoProtected(Boolean isAutoProtected) {
this.isAutoProtected = isAutoProtected;
return this;
}
/**
* Get the subinquireditemcount property: For instance or AG, indicates number of DB's present.
*
* @return the subinquireditemcount value.
*/
public Integer subinquireditemcount() {
return this.subinquireditemcount;
}
/**
* Set the subinquireditemcount property: For instance or AG, indicates number of DB's present.
*
* @param subinquireditemcount the subinquireditemcount value to set.
* @return the AzureVmWorkloadProtectableItem object itself.
*/
public AzureVmWorkloadProtectableItem withSubinquireditemcount(Integer subinquireditemcount) {
this.subinquireditemcount = subinquireditemcount;
return this;
}
/**
* Get the subprotectableitemcount property: For instance or AG, indicates number of DB's to be protected.
*
* @return the subprotectableitemcount value.
*/
public Integer subprotectableitemcount() {
return this.subprotectableitemcount;
}
/**
* Set the subprotectableitemcount property: For instance or AG, indicates number of DB's to be protected.
*
* @param subprotectableitemcount the subprotectableitemcount value to set.
* @return the AzureVmWorkloadProtectableItem object itself.
*/
public AzureVmWorkloadProtectableItem withSubprotectableitemcount(Integer subprotectableitemcount) {
this.subprotectableitemcount = subprotectableitemcount;
return this;
}
/**
* Get the prebackupvalidation property: Pre-backup validation for protectable objects.
*
* @return the prebackupvalidation value.
*/
public PreBackupValidation prebackupvalidation() {
return this.prebackupvalidation;
}
/**
* Set the prebackupvalidation property: Pre-backup validation for protectable objects.
*
* @param prebackupvalidation the prebackupvalidation value to set.
* @return the AzureVmWorkloadProtectableItem object itself.
*/
public AzureVmWorkloadProtectableItem withPrebackupvalidation(PreBackupValidation prebackupvalidation) {
this.prebackupvalidation = prebackupvalidation;
return this;
}
/**
* Get the isProtectable property: Indicates if item is protectable.
*
* @return the isProtectable value.
*/
public Boolean isProtectable() {
return this.isProtectable;
}
/**
* Set the isProtectable property: Indicates if item is protectable.
*
* @param isProtectable the isProtectable value to set.
* @return the AzureVmWorkloadProtectableItem object itself.
*/
public AzureVmWorkloadProtectableItem withIsProtectable(Boolean isProtectable) {
this.isProtectable = isProtectable;
return this;
}
/**
* {@inheritDoc}
*/
@Override
public AzureVmWorkloadProtectableItem withBackupManagementType(String backupManagementType) {
super.withBackupManagementType(backupManagementType);
return this;
}
/**
* {@inheritDoc}
*/
@Override
public AzureVmWorkloadProtectableItem withWorkloadType(String workloadType) {
super.withWorkloadType(workloadType);
return this;
}
/**
* {@inheritDoc}
*/
@Override
public AzureVmWorkloadProtectableItem withFriendlyName(String friendlyName) {
super.withFriendlyName(friendlyName);
return this;
}
/**
* {@inheritDoc}
*/
@Override
public AzureVmWorkloadProtectableItem withProtectionState(ProtectionStatus protectionState) {
super.withProtectionState(protectionState);
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
@Override
public void validate() {
super.validate();
if (prebackupvalidation() != null) {
prebackupvalidation().validate();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy