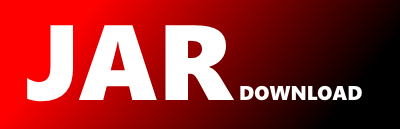
com.azure.resourcemanager.recoveryservicesbackup.models.AzureVmWorkloadProtectedItem Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-recoveryservicesbackup Show documentation
Show all versions of azure-resourcemanager-recoveryservicesbackup Show documentation
This package contains Microsoft Azure SDK for RecoveryServicesBackup Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. Open API 2.0 Specs for Azure RecoveryServices Backup service. Package tag package-2024-04.
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.recoveryservicesbackup.models;
import com.azure.core.annotation.Fluent;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonSubTypes;
import com.fasterxml.jackson.annotation.JsonTypeInfo;
import com.fasterxml.jackson.annotation.JsonTypeName;
import java.time.OffsetDateTime;
import java.util.List;
import java.util.Map;
/**
* Azure VM workload-specific protected item.
*/
@JsonTypeInfo(
use = JsonTypeInfo.Id.NAME,
include = JsonTypeInfo.As.PROPERTY,
property = "protectedItemType",
defaultImpl = AzureVmWorkloadProtectedItem.class)
@JsonTypeName("AzureVmWorkloadProtectedItem")
@JsonSubTypes({
@JsonSubTypes.Type(
name = "AzureVmWorkloadSAPAseDatabase",
value = AzureVmWorkloadSapAseDatabaseProtectedItem.class),
@JsonSubTypes.Type(
name = "AzureVmWorkloadSAPHanaDatabase",
value = AzureVmWorkloadSapHanaDatabaseProtectedItem.class),
@JsonSubTypes.Type(
name = "AzureVmWorkloadSAPHanaDBInstance",
value = AzureVmWorkloadSapHanaDBInstanceProtectedItem.class),
@JsonSubTypes.Type(name = "AzureVmWorkloadSQLDatabase", value = AzureVmWorkloadSqlDatabaseProtectedItem.class) })
@Fluent
public class AzureVmWorkloadProtectedItem extends ProtectedItem {
/*
* Friendly name of the DB represented by this backup item.
*/
@JsonProperty(value = "friendlyName", access = JsonProperty.Access.WRITE_ONLY)
private String friendlyName;
/*
* Host/Cluster Name for instance or AG
*/
@JsonProperty(value = "serverName")
private String serverName;
/*
* Parent name of the DB such as Instance or Availability Group.
*/
@JsonProperty(value = "parentName")
private String parentName;
/*
* Parent type of protected item, example: for a DB, standalone server or distributed
*/
@JsonProperty(value = "parentType")
private String parentType;
/*
* Backup status of this backup item.
*/
@JsonProperty(value = "protectionStatus", access = JsonProperty.Access.WRITE_ONLY)
private String protectionStatus;
/*
* Backup state of this backup item.
*/
@JsonProperty(value = "protectionState")
private ProtectionState protectionState;
/*
* Last backup operation status. Possible values: Healthy, Unhealthy.
*/
@JsonProperty(value = "lastBackupStatus")
private LastBackupStatus lastBackupStatus;
/*
* Timestamp of the last backup operation on this backup item.
*/
@JsonProperty(value = "lastBackupTime")
private OffsetDateTime lastBackupTime;
/*
* Error details in last backup
*/
@JsonProperty(value = "lastBackupErrorDetail")
private ErrorDetail lastBackupErrorDetail;
/*
* Data ID of the protected item.
*/
@JsonProperty(value = "protectedItemDataSourceId")
private String protectedItemDataSourceId;
/*
* Health status of the backup item, evaluated based on last heartbeat received
*/
@JsonProperty(value = "protectedItemHealthStatus")
private ProtectedItemHealthStatus protectedItemHealthStatus;
/*
* Additional information for this backup item.
*/
@JsonProperty(value = "extendedInfo")
private AzureVmWorkloadProtectedItemExtendedInfo extendedInfo;
/*
* Health details of different KPIs
*/
@JsonProperty(value = "kpisHealths")
@JsonInclude(value = JsonInclude.Include.NON_NULL, content = JsonInclude.Include.ALWAYS)
private Map kpisHealths;
/*
* List of the nodes in case of distributed container.
*/
@JsonProperty(value = "nodesList")
private List nodesList;
/**
* Creates an instance of AzureVmWorkloadProtectedItem class.
*/
public AzureVmWorkloadProtectedItem() {
}
/**
* Get the friendlyName property: Friendly name of the DB represented by this backup item.
*
* @return the friendlyName value.
*/
public String friendlyName() {
return this.friendlyName;
}
/**
* Get the serverName property: Host/Cluster Name for instance or AG.
*
* @return the serverName value.
*/
public String serverName() {
return this.serverName;
}
/**
* Set the serverName property: Host/Cluster Name for instance or AG.
*
* @param serverName the serverName value to set.
* @return the AzureVmWorkloadProtectedItem object itself.
*/
public AzureVmWorkloadProtectedItem withServerName(String serverName) {
this.serverName = serverName;
return this;
}
/**
* Get the parentName property: Parent name of the DB such as Instance or Availability Group.
*
* @return the parentName value.
*/
public String parentName() {
return this.parentName;
}
/**
* Set the parentName property: Parent name of the DB such as Instance or Availability Group.
*
* @param parentName the parentName value to set.
* @return the AzureVmWorkloadProtectedItem object itself.
*/
public AzureVmWorkloadProtectedItem withParentName(String parentName) {
this.parentName = parentName;
return this;
}
/**
* Get the parentType property: Parent type of protected item, example: for a DB, standalone server or distributed.
*
* @return the parentType value.
*/
public String parentType() {
return this.parentType;
}
/**
* Set the parentType property: Parent type of protected item, example: for a DB, standalone server or distributed.
*
* @param parentType the parentType value to set.
* @return the AzureVmWorkloadProtectedItem object itself.
*/
public AzureVmWorkloadProtectedItem withParentType(String parentType) {
this.parentType = parentType;
return this;
}
/**
* Get the protectionStatus property: Backup status of this backup item.
*
* @return the protectionStatus value.
*/
public String protectionStatus() {
return this.protectionStatus;
}
/**
* Get the protectionState property: Backup state of this backup item.
*
* @return the protectionState value.
*/
public ProtectionState protectionState() {
return this.protectionState;
}
/**
* Set the protectionState property: Backup state of this backup item.
*
* @param protectionState the protectionState value to set.
* @return the AzureVmWorkloadProtectedItem object itself.
*/
public AzureVmWorkloadProtectedItem withProtectionState(ProtectionState protectionState) {
this.protectionState = protectionState;
return this;
}
/**
* Get the lastBackupStatus property: Last backup operation status. Possible values: Healthy, Unhealthy.
*
* @return the lastBackupStatus value.
*/
public LastBackupStatus lastBackupStatus() {
return this.lastBackupStatus;
}
/**
* Set the lastBackupStatus property: Last backup operation status. Possible values: Healthy, Unhealthy.
*
* @param lastBackupStatus the lastBackupStatus value to set.
* @return the AzureVmWorkloadProtectedItem object itself.
*/
public AzureVmWorkloadProtectedItem withLastBackupStatus(LastBackupStatus lastBackupStatus) {
this.lastBackupStatus = lastBackupStatus;
return this;
}
/**
* Get the lastBackupTime property: Timestamp of the last backup operation on this backup item.
*
* @return the lastBackupTime value.
*/
public OffsetDateTime lastBackupTime() {
return this.lastBackupTime;
}
/**
* Set the lastBackupTime property: Timestamp of the last backup operation on this backup item.
*
* @param lastBackupTime the lastBackupTime value to set.
* @return the AzureVmWorkloadProtectedItem object itself.
*/
public AzureVmWorkloadProtectedItem withLastBackupTime(OffsetDateTime lastBackupTime) {
this.lastBackupTime = lastBackupTime;
return this;
}
/**
* Get the lastBackupErrorDetail property: Error details in last backup.
*
* @return the lastBackupErrorDetail value.
*/
public ErrorDetail lastBackupErrorDetail() {
return this.lastBackupErrorDetail;
}
/**
* Set the lastBackupErrorDetail property: Error details in last backup.
*
* @param lastBackupErrorDetail the lastBackupErrorDetail value to set.
* @return the AzureVmWorkloadProtectedItem object itself.
*/
public AzureVmWorkloadProtectedItem withLastBackupErrorDetail(ErrorDetail lastBackupErrorDetail) {
this.lastBackupErrorDetail = lastBackupErrorDetail;
return this;
}
/**
* Get the protectedItemDataSourceId property: Data ID of the protected item.
*
* @return the protectedItemDataSourceId value.
*/
public String protectedItemDataSourceId() {
return this.protectedItemDataSourceId;
}
/**
* Set the protectedItemDataSourceId property: Data ID of the protected item.
*
* @param protectedItemDataSourceId the protectedItemDataSourceId value to set.
* @return the AzureVmWorkloadProtectedItem object itself.
*/
public AzureVmWorkloadProtectedItem withProtectedItemDataSourceId(String protectedItemDataSourceId) {
this.protectedItemDataSourceId = protectedItemDataSourceId;
return this;
}
/**
* Get the protectedItemHealthStatus property: Health status of the backup item, evaluated based on last heartbeat
* received.
*
* @return the protectedItemHealthStatus value.
*/
public ProtectedItemHealthStatus protectedItemHealthStatus() {
return this.protectedItemHealthStatus;
}
/**
* Set the protectedItemHealthStatus property: Health status of the backup item, evaluated based on last heartbeat
* received.
*
* @param protectedItemHealthStatus the protectedItemHealthStatus value to set.
* @return the AzureVmWorkloadProtectedItem object itself.
*/
public AzureVmWorkloadProtectedItem
withProtectedItemHealthStatus(ProtectedItemHealthStatus protectedItemHealthStatus) {
this.protectedItemHealthStatus = protectedItemHealthStatus;
return this;
}
/**
* Get the extendedInfo property: Additional information for this backup item.
*
* @return the extendedInfo value.
*/
public AzureVmWorkloadProtectedItemExtendedInfo extendedInfo() {
return this.extendedInfo;
}
/**
* Set the extendedInfo property: Additional information for this backup item.
*
* @param extendedInfo the extendedInfo value to set.
* @return the AzureVmWorkloadProtectedItem object itself.
*/
public AzureVmWorkloadProtectedItem withExtendedInfo(AzureVmWorkloadProtectedItemExtendedInfo extendedInfo) {
this.extendedInfo = extendedInfo;
return this;
}
/**
* Get the kpisHealths property: Health details of different KPIs.
*
* @return the kpisHealths value.
*/
public Map kpisHealths() {
return this.kpisHealths;
}
/**
* Set the kpisHealths property: Health details of different KPIs.
*
* @param kpisHealths the kpisHealths value to set.
* @return the AzureVmWorkloadProtectedItem object itself.
*/
public AzureVmWorkloadProtectedItem withKpisHealths(Map kpisHealths) {
this.kpisHealths = kpisHealths;
return this;
}
/**
* Get the nodesList property: List of the nodes in case of distributed container.
*
* @return the nodesList value.
*/
public List nodesList() {
return this.nodesList;
}
/**
* Set the nodesList property: List of the nodes in case of distributed container.
*
* @param nodesList the nodesList value to set.
* @return the AzureVmWorkloadProtectedItem object itself.
*/
public AzureVmWorkloadProtectedItem withNodesList(List nodesList) {
this.nodesList = nodesList;
return this;
}
/**
* {@inheritDoc}
*/
@Override
public AzureVmWorkloadProtectedItem withContainerName(String containerName) {
super.withContainerName(containerName);
return this;
}
/**
* {@inheritDoc}
*/
@Override
public AzureVmWorkloadProtectedItem withSourceResourceId(String sourceResourceId) {
super.withSourceResourceId(sourceResourceId);
return this;
}
/**
* {@inheritDoc}
*/
@Override
public AzureVmWorkloadProtectedItem withPolicyId(String policyId) {
super.withPolicyId(policyId);
return this;
}
/**
* {@inheritDoc}
*/
@Override
public AzureVmWorkloadProtectedItem withLastRecoveryPoint(OffsetDateTime lastRecoveryPoint) {
super.withLastRecoveryPoint(lastRecoveryPoint);
return this;
}
/**
* {@inheritDoc}
*/
@Override
public AzureVmWorkloadProtectedItem withBackupSetName(String backupSetName) {
super.withBackupSetName(backupSetName);
return this;
}
/**
* {@inheritDoc}
*/
@Override
public AzureVmWorkloadProtectedItem withCreateMode(CreateMode createMode) {
super.withCreateMode(createMode);
return this;
}
/**
* {@inheritDoc}
*/
@Override
public AzureVmWorkloadProtectedItem withDeferredDeleteTimeInUtc(OffsetDateTime deferredDeleteTimeInUtc) {
super.withDeferredDeleteTimeInUtc(deferredDeleteTimeInUtc);
return this;
}
/**
* {@inheritDoc}
*/
@Override
public AzureVmWorkloadProtectedItem withIsScheduledForDeferredDelete(Boolean isScheduledForDeferredDelete) {
super.withIsScheduledForDeferredDelete(isScheduledForDeferredDelete);
return this;
}
/**
* {@inheritDoc}
*/
@Override
public AzureVmWorkloadProtectedItem withDeferredDeleteTimeRemaining(String deferredDeleteTimeRemaining) {
super.withDeferredDeleteTimeRemaining(deferredDeleteTimeRemaining);
return this;
}
/**
* {@inheritDoc}
*/
@Override
public AzureVmWorkloadProtectedItem withIsDeferredDeleteScheduleUpcoming(Boolean isDeferredDeleteScheduleUpcoming) {
super.withIsDeferredDeleteScheduleUpcoming(isDeferredDeleteScheduleUpcoming);
return this;
}
/**
* {@inheritDoc}
*/
@Override
public AzureVmWorkloadProtectedItem withIsRehydrate(Boolean isRehydrate) {
super.withIsRehydrate(isRehydrate);
return this;
}
/**
* {@inheritDoc}
*/
@Override
public AzureVmWorkloadProtectedItem
withResourceGuardOperationRequests(List resourceGuardOperationRequests) {
super.withResourceGuardOperationRequests(resourceGuardOperationRequests);
return this;
}
/**
* {@inheritDoc}
*/
@Override
public AzureVmWorkloadProtectedItem withIsArchiveEnabled(Boolean isArchiveEnabled) {
super.withIsArchiveEnabled(isArchiveEnabled);
return this;
}
/**
* {@inheritDoc}
*/
@Override
public AzureVmWorkloadProtectedItem withPolicyName(String policyName) {
super.withPolicyName(policyName);
return this;
}
/**
* {@inheritDoc}
*/
@Override
public AzureVmWorkloadProtectedItem withSoftDeleteRetentionPeriod(Integer softDeleteRetentionPeriod) {
super.withSoftDeleteRetentionPeriod(softDeleteRetentionPeriod);
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
@Override
public void validate() {
super.validate();
if (lastBackupErrorDetail() != null) {
lastBackupErrorDetail().validate();
}
if (extendedInfo() != null) {
extendedInfo().validate();
}
if (kpisHealths() != null) {
kpisHealths().values().forEach(e -> {
if (e != null) {
e.validate();
}
});
}
if (nodesList() != null) {
nodesList().forEach(e -> e.validate());
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy