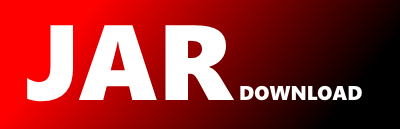
com.azure.resourcemanager.recoveryservicesbackup.models.AzureWorkloadRestoreRequest Maven / Gradle / Ivy
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.recoveryservicesbackup.models;
import com.azure.core.annotation.Fluent;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonSubTypes;
import com.fasterxml.jackson.annotation.JsonTypeInfo;
import com.fasterxml.jackson.annotation.JsonTypeName;
import java.util.Map;
/**
* AzureWorkload-specific restore.
*/
@JsonTypeInfo(
use = JsonTypeInfo.Id.NAME,
include = JsonTypeInfo.As.PROPERTY,
property = "objectType",
defaultImpl = AzureWorkloadRestoreRequest.class)
@JsonTypeName("AzureWorkloadRestoreRequest")
@JsonSubTypes({
@JsonSubTypes.Type(
name = "AzureWorkloadPointInTimeRestoreRequest",
value = AzureWorkloadPointInTimeRestoreRequest.class),
@JsonSubTypes.Type(name = "AzureWorkloadSAPHanaRestoreRequest", value = AzureWorkloadSapHanaRestoreRequest.class),
@JsonSubTypes.Type(name = "AzureWorkloadSQLRestoreRequest", value = AzureWorkloadSqlRestoreRequest.class) })
@Fluent
public class AzureWorkloadRestoreRequest extends RestoreRequest {
/*
* Type of this recovery.
*/
@JsonProperty(value = "recoveryType")
private RecoveryType recoveryType;
/*
* Fully qualified ARM ID of the VM on which workload that was running is being recovered.
*/
@JsonProperty(value = "sourceResourceId")
private String sourceResourceId;
/*
* Workload specific property bag.
*/
@JsonProperty(value = "propertyBag")
@JsonInclude(value = JsonInclude.Include.NON_NULL, content = JsonInclude.Include.ALWAYS)
private Map propertyBag;
/*
* Details of target database
*/
@JsonProperty(value = "targetInfo")
private TargetRestoreInfo targetInfo;
/*
* Defines whether the current recovery mode is file restore or database restore
*/
@JsonProperty(value = "recoveryMode")
private RecoveryMode recoveryMode;
/*
* Defines the Resource group of the Target VM
*/
@JsonProperty(value = "targetResourceGroupName")
private String targetResourceGroupName;
/*
* User Assigned managed identity details
* Currently used for snapshot.
*/
@JsonProperty(value = "userAssignedManagedIdentityDetails")
private UserAssignedManagedIdentityDetails userAssignedManagedIdentityDetails;
/*
* Additional details for snapshot recovery
* Currently used for snapshot for SAP Hana.
*/
@JsonProperty(value = "snapshotRestoreParameters")
private SnapshotRestoreParameters snapshotRestoreParameters;
/*
* This is the complete ARM Id of the target VM
* For e.g. /subscriptions/{subId}/resourcegroups/{rg}/provider/Microsoft.Compute/virtualmachines/{vm}
*/
@JsonProperty(value = "targetVirtualMachineId")
private String targetVirtualMachineId;
/**
* Creates an instance of AzureWorkloadRestoreRequest class.
*/
public AzureWorkloadRestoreRequest() {
}
/**
* Get the recoveryType property: Type of this recovery.
*
* @return the recoveryType value.
*/
public RecoveryType recoveryType() {
return this.recoveryType;
}
/**
* Set the recoveryType property: Type of this recovery.
*
* @param recoveryType the recoveryType value to set.
* @return the AzureWorkloadRestoreRequest object itself.
*/
public AzureWorkloadRestoreRequest withRecoveryType(RecoveryType recoveryType) {
this.recoveryType = recoveryType;
return this;
}
/**
* Get the sourceResourceId property: Fully qualified ARM ID of the VM on which workload that was running is being
* recovered.
*
* @return the sourceResourceId value.
*/
public String sourceResourceId() {
return this.sourceResourceId;
}
/**
* Set the sourceResourceId property: Fully qualified ARM ID of the VM on which workload that was running is being
* recovered.
*
* @param sourceResourceId the sourceResourceId value to set.
* @return the AzureWorkloadRestoreRequest object itself.
*/
public AzureWorkloadRestoreRequest withSourceResourceId(String sourceResourceId) {
this.sourceResourceId = sourceResourceId;
return this;
}
/**
* Get the propertyBag property: Workload specific property bag.
*
* @return the propertyBag value.
*/
public Map propertyBag() {
return this.propertyBag;
}
/**
* Set the propertyBag property: Workload specific property bag.
*
* @param propertyBag the propertyBag value to set.
* @return the AzureWorkloadRestoreRequest object itself.
*/
public AzureWorkloadRestoreRequest withPropertyBag(Map propertyBag) {
this.propertyBag = propertyBag;
return this;
}
/**
* Get the targetInfo property: Details of target database.
*
* @return the targetInfo value.
*/
public TargetRestoreInfo targetInfo() {
return this.targetInfo;
}
/**
* Set the targetInfo property: Details of target database.
*
* @param targetInfo the targetInfo value to set.
* @return the AzureWorkloadRestoreRequest object itself.
*/
public AzureWorkloadRestoreRequest withTargetInfo(TargetRestoreInfo targetInfo) {
this.targetInfo = targetInfo;
return this;
}
/**
* Get the recoveryMode property: Defines whether the current recovery mode is file restore or database restore.
*
* @return the recoveryMode value.
*/
public RecoveryMode recoveryMode() {
return this.recoveryMode;
}
/**
* Set the recoveryMode property: Defines whether the current recovery mode is file restore or database restore.
*
* @param recoveryMode the recoveryMode value to set.
* @return the AzureWorkloadRestoreRequest object itself.
*/
public AzureWorkloadRestoreRequest withRecoveryMode(RecoveryMode recoveryMode) {
this.recoveryMode = recoveryMode;
return this;
}
/**
* Get the targetResourceGroupName property: Defines the Resource group of the Target VM.
*
* @return the targetResourceGroupName value.
*/
public String targetResourceGroupName() {
return this.targetResourceGroupName;
}
/**
* Set the targetResourceGroupName property: Defines the Resource group of the Target VM.
*
* @param targetResourceGroupName the targetResourceGroupName value to set.
* @return the AzureWorkloadRestoreRequest object itself.
*/
public AzureWorkloadRestoreRequest withTargetResourceGroupName(String targetResourceGroupName) {
this.targetResourceGroupName = targetResourceGroupName;
return this;
}
/**
* Get the userAssignedManagedIdentityDetails property: User Assigned managed identity details
* Currently used for snapshot.
*
* @return the userAssignedManagedIdentityDetails value.
*/
public UserAssignedManagedIdentityDetails userAssignedManagedIdentityDetails() {
return this.userAssignedManagedIdentityDetails;
}
/**
* Set the userAssignedManagedIdentityDetails property: User Assigned managed identity details
* Currently used for snapshot.
*
* @param userAssignedManagedIdentityDetails the userAssignedManagedIdentityDetails value to set.
* @return the AzureWorkloadRestoreRequest object itself.
*/
public AzureWorkloadRestoreRequest
withUserAssignedManagedIdentityDetails(UserAssignedManagedIdentityDetails userAssignedManagedIdentityDetails) {
this.userAssignedManagedIdentityDetails = userAssignedManagedIdentityDetails;
return this;
}
/**
* Get the snapshotRestoreParameters property: Additional details for snapshot recovery
* Currently used for snapshot for SAP Hana.
*
* @return the snapshotRestoreParameters value.
*/
public SnapshotRestoreParameters snapshotRestoreParameters() {
return this.snapshotRestoreParameters;
}
/**
* Set the snapshotRestoreParameters property: Additional details for snapshot recovery
* Currently used for snapshot for SAP Hana.
*
* @param snapshotRestoreParameters the snapshotRestoreParameters value to set.
* @return the AzureWorkloadRestoreRequest object itself.
*/
public AzureWorkloadRestoreRequest
withSnapshotRestoreParameters(SnapshotRestoreParameters snapshotRestoreParameters) {
this.snapshotRestoreParameters = snapshotRestoreParameters;
return this;
}
/**
* Get the targetVirtualMachineId property: This is the complete ARM Id of the target VM
* For e.g. /subscriptions/{subId}/resourcegroups/{rg}/provider/Microsoft.Compute/virtualmachines/{vm}.
*
* @return the targetVirtualMachineId value.
*/
public String targetVirtualMachineId() {
return this.targetVirtualMachineId;
}
/**
* Set the targetVirtualMachineId property: This is the complete ARM Id of the target VM
* For e.g. /subscriptions/{subId}/resourcegroups/{rg}/provider/Microsoft.Compute/virtualmachines/{vm}.
*
* @param targetVirtualMachineId the targetVirtualMachineId value to set.
* @return the AzureWorkloadRestoreRequest object itself.
*/
public AzureWorkloadRestoreRequest withTargetVirtualMachineId(String targetVirtualMachineId) {
this.targetVirtualMachineId = targetVirtualMachineId;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
@Override
public void validate() {
super.validate();
if (targetInfo() != null) {
targetInfo().validate();
}
if (userAssignedManagedIdentityDetails() != null) {
userAssignedManagedIdentityDetails().validate();
}
if (snapshotRestoreParameters() != null) {
snapshotRestoreParameters().validate();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy