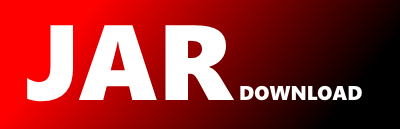
com.azure.resourcemanager.recoveryservicesbackup.models.BackupEngineExtendedInfo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-recoveryservicesbackup Show documentation
Show all versions of azure-resourcemanager-recoveryservicesbackup Show documentation
This package contains Microsoft Azure SDK for RecoveryServicesBackup Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. Open API 2.0 Specs for Azure RecoveryServices Backup service. Package tag package-2024-04.
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.recoveryservicesbackup.models;
import com.azure.core.annotation.Fluent;
import com.fasterxml.jackson.annotation.JsonProperty;
import java.time.OffsetDateTime;
/**
* Additional information on backup engine.
*/
@Fluent
public final class BackupEngineExtendedInfo {
/*
* Database name of backup engine.
*/
@JsonProperty(value = "databaseName")
private String databaseName;
/*
* Number of protected items in the backup engine.
*/
@JsonProperty(value = "protectedItemsCount")
private Integer protectedItemsCount;
/*
* Number of protected servers in the backup engine.
*/
@JsonProperty(value = "protectedServersCount")
private Integer protectedServersCount;
/*
* Number of disks in the backup engine.
*/
@JsonProperty(value = "diskCount")
private Integer diskCount;
/*
* Disk space used in the backup engine.
*/
@JsonProperty(value = "usedDiskSpace")
private Double usedDiskSpace;
/*
* Disk space currently available in the backup engine.
*/
@JsonProperty(value = "availableDiskSpace")
private Double availableDiskSpace;
/*
* Last refresh time in the backup engine.
*/
@JsonProperty(value = "refreshedAt")
private OffsetDateTime refreshedAt;
/*
* Protected instances in the backup engine.
*/
@JsonProperty(value = "azureProtectedInstances")
private Integer azureProtectedInstances;
/**
* Creates an instance of BackupEngineExtendedInfo class.
*/
public BackupEngineExtendedInfo() {
}
/**
* Get the databaseName property: Database name of backup engine.
*
* @return the databaseName value.
*/
public String databaseName() {
return this.databaseName;
}
/**
* Set the databaseName property: Database name of backup engine.
*
* @param databaseName the databaseName value to set.
* @return the BackupEngineExtendedInfo object itself.
*/
public BackupEngineExtendedInfo withDatabaseName(String databaseName) {
this.databaseName = databaseName;
return this;
}
/**
* Get the protectedItemsCount property: Number of protected items in the backup engine.
*
* @return the protectedItemsCount value.
*/
public Integer protectedItemsCount() {
return this.protectedItemsCount;
}
/**
* Set the protectedItemsCount property: Number of protected items in the backup engine.
*
* @param protectedItemsCount the protectedItemsCount value to set.
* @return the BackupEngineExtendedInfo object itself.
*/
public BackupEngineExtendedInfo withProtectedItemsCount(Integer protectedItemsCount) {
this.protectedItemsCount = protectedItemsCount;
return this;
}
/**
* Get the protectedServersCount property: Number of protected servers in the backup engine.
*
* @return the protectedServersCount value.
*/
public Integer protectedServersCount() {
return this.protectedServersCount;
}
/**
* Set the protectedServersCount property: Number of protected servers in the backup engine.
*
* @param protectedServersCount the protectedServersCount value to set.
* @return the BackupEngineExtendedInfo object itself.
*/
public BackupEngineExtendedInfo withProtectedServersCount(Integer protectedServersCount) {
this.protectedServersCount = protectedServersCount;
return this;
}
/**
* Get the diskCount property: Number of disks in the backup engine.
*
* @return the diskCount value.
*/
public Integer diskCount() {
return this.diskCount;
}
/**
* Set the diskCount property: Number of disks in the backup engine.
*
* @param diskCount the diskCount value to set.
* @return the BackupEngineExtendedInfo object itself.
*/
public BackupEngineExtendedInfo withDiskCount(Integer diskCount) {
this.diskCount = diskCount;
return this;
}
/**
* Get the usedDiskSpace property: Disk space used in the backup engine.
*
* @return the usedDiskSpace value.
*/
public Double usedDiskSpace() {
return this.usedDiskSpace;
}
/**
* Set the usedDiskSpace property: Disk space used in the backup engine.
*
* @param usedDiskSpace the usedDiskSpace value to set.
* @return the BackupEngineExtendedInfo object itself.
*/
public BackupEngineExtendedInfo withUsedDiskSpace(Double usedDiskSpace) {
this.usedDiskSpace = usedDiskSpace;
return this;
}
/**
* Get the availableDiskSpace property: Disk space currently available in the backup engine.
*
* @return the availableDiskSpace value.
*/
public Double availableDiskSpace() {
return this.availableDiskSpace;
}
/**
* Set the availableDiskSpace property: Disk space currently available in the backup engine.
*
* @param availableDiskSpace the availableDiskSpace value to set.
* @return the BackupEngineExtendedInfo object itself.
*/
public BackupEngineExtendedInfo withAvailableDiskSpace(Double availableDiskSpace) {
this.availableDiskSpace = availableDiskSpace;
return this;
}
/**
* Get the refreshedAt property: Last refresh time in the backup engine.
*
* @return the refreshedAt value.
*/
public OffsetDateTime refreshedAt() {
return this.refreshedAt;
}
/**
* Set the refreshedAt property: Last refresh time in the backup engine.
*
* @param refreshedAt the refreshedAt value to set.
* @return the BackupEngineExtendedInfo object itself.
*/
public BackupEngineExtendedInfo withRefreshedAt(OffsetDateTime refreshedAt) {
this.refreshedAt = refreshedAt;
return this;
}
/**
* Get the azureProtectedInstances property: Protected instances in the backup engine.
*
* @return the azureProtectedInstances value.
*/
public Integer azureProtectedInstances() {
return this.azureProtectedInstances;
}
/**
* Set the azureProtectedInstances property: Protected instances in the backup engine.
*
* @param azureProtectedInstances the azureProtectedInstances value to set.
* @return the BackupEngineExtendedInfo object itself.
*/
public BackupEngineExtendedInfo withAzureProtectedInstances(Integer azureProtectedInstances) {
this.azureProtectedInstances = azureProtectedInstances;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy