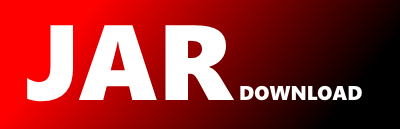
com.azure.resourcemanager.recoveryservicesbackup.models.BackupResourceVaultConfig Maven / Gradle / Ivy
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.recoveryservicesbackup.models;
import com.azure.core.annotation.Fluent;
import com.fasterxml.jackson.annotation.JsonProperty;
import java.util.List;
/**
* Backup resource vault config details.
*/
@Fluent
public final class BackupResourceVaultConfig {
/*
* Storage type.
*/
@JsonProperty(value = "storageModelType")
private StorageType storageModelType;
/*
* Storage type.
*/
@JsonProperty(value = "storageType")
private StorageType storageType;
/*
* Locked or Unlocked. Once a machine is registered against a resource, the storageTypeState is always Locked.
*/
@JsonProperty(value = "storageTypeState")
private StorageTypeState storageTypeState;
/*
* Enabled or Disabled.
*/
@JsonProperty(value = "enhancedSecurityState")
private EnhancedSecurityState enhancedSecurityState;
/*
* Soft Delete feature state
*/
@JsonProperty(value = "softDeleteFeatureState")
private SoftDeleteFeatureState softDeleteFeatureState;
/*
* Soft delete retention period in days
*/
@JsonProperty(value = "softDeleteRetentionPeriodInDays")
private Integer softDeleteRetentionPeriodInDays;
/*
* ResourceGuard Operation Requests
*/
@JsonProperty(value = "resourceGuardOperationRequests")
private List resourceGuardOperationRequests;
/*
* This flag is no longer in use. Please use 'softDeleteFeatureState' to set the soft delete state for the vault
*/
@JsonProperty(value = "isSoftDeleteFeatureStateEditable")
private Boolean isSoftDeleteFeatureStateEditable;
/**
* Creates an instance of BackupResourceVaultConfig class.
*/
public BackupResourceVaultConfig() {
}
/**
* Get the storageModelType property: Storage type.
*
* @return the storageModelType value.
*/
public StorageType storageModelType() {
return this.storageModelType;
}
/**
* Set the storageModelType property: Storage type.
*
* @param storageModelType the storageModelType value to set.
* @return the BackupResourceVaultConfig object itself.
*/
public BackupResourceVaultConfig withStorageModelType(StorageType storageModelType) {
this.storageModelType = storageModelType;
return this;
}
/**
* Get the storageType property: Storage type.
*
* @return the storageType value.
*/
public StorageType storageType() {
return this.storageType;
}
/**
* Set the storageType property: Storage type.
*
* @param storageType the storageType value to set.
* @return the BackupResourceVaultConfig object itself.
*/
public BackupResourceVaultConfig withStorageType(StorageType storageType) {
this.storageType = storageType;
return this;
}
/**
* Get the storageTypeState property: Locked or Unlocked. Once a machine is registered against a resource, the
* storageTypeState is always Locked.
*
* @return the storageTypeState value.
*/
public StorageTypeState storageTypeState() {
return this.storageTypeState;
}
/**
* Set the storageTypeState property: Locked or Unlocked. Once a machine is registered against a resource, the
* storageTypeState is always Locked.
*
* @param storageTypeState the storageTypeState value to set.
* @return the BackupResourceVaultConfig object itself.
*/
public BackupResourceVaultConfig withStorageTypeState(StorageTypeState storageTypeState) {
this.storageTypeState = storageTypeState;
return this;
}
/**
* Get the enhancedSecurityState property: Enabled or Disabled.
*
* @return the enhancedSecurityState value.
*/
public EnhancedSecurityState enhancedSecurityState() {
return this.enhancedSecurityState;
}
/**
* Set the enhancedSecurityState property: Enabled or Disabled.
*
* @param enhancedSecurityState the enhancedSecurityState value to set.
* @return the BackupResourceVaultConfig object itself.
*/
public BackupResourceVaultConfig withEnhancedSecurityState(EnhancedSecurityState enhancedSecurityState) {
this.enhancedSecurityState = enhancedSecurityState;
return this;
}
/**
* Get the softDeleteFeatureState property: Soft Delete feature state.
*
* @return the softDeleteFeatureState value.
*/
public SoftDeleteFeatureState softDeleteFeatureState() {
return this.softDeleteFeatureState;
}
/**
* Set the softDeleteFeatureState property: Soft Delete feature state.
*
* @param softDeleteFeatureState the softDeleteFeatureState value to set.
* @return the BackupResourceVaultConfig object itself.
*/
public BackupResourceVaultConfig withSoftDeleteFeatureState(SoftDeleteFeatureState softDeleteFeatureState) {
this.softDeleteFeatureState = softDeleteFeatureState;
return this;
}
/**
* Get the softDeleteRetentionPeriodInDays property: Soft delete retention period in days.
*
* @return the softDeleteRetentionPeriodInDays value.
*/
public Integer softDeleteRetentionPeriodInDays() {
return this.softDeleteRetentionPeriodInDays;
}
/**
* Set the softDeleteRetentionPeriodInDays property: Soft delete retention period in days.
*
* @param softDeleteRetentionPeriodInDays the softDeleteRetentionPeriodInDays value to set.
* @return the BackupResourceVaultConfig object itself.
*/
public BackupResourceVaultConfig withSoftDeleteRetentionPeriodInDays(Integer softDeleteRetentionPeriodInDays) {
this.softDeleteRetentionPeriodInDays = softDeleteRetentionPeriodInDays;
return this;
}
/**
* Get the resourceGuardOperationRequests property: ResourceGuard Operation Requests.
*
* @return the resourceGuardOperationRequests value.
*/
public List resourceGuardOperationRequests() {
return this.resourceGuardOperationRequests;
}
/**
* Set the resourceGuardOperationRequests property: ResourceGuard Operation Requests.
*
* @param resourceGuardOperationRequests the resourceGuardOperationRequests value to set.
* @return the BackupResourceVaultConfig object itself.
*/
public BackupResourceVaultConfig withResourceGuardOperationRequests(List resourceGuardOperationRequests) {
this.resourceGuardOperationRequests = resourceGuardOperationRequests;
return this;
}
/**
* Get the isSoftDeleteFeatureStateEditable property: This flag is no longer in use. Please use
* 'softDeleteFeatureState' to set the soft delete state for the vault.
*
* @return the isSoftDeleteFeatureStateEditable value.
*/
public Boolean isSoftDeleteFeatureStateEditable() {
return this.isSoftDeleteFeatureStateEditable;
}
/**
* Set the isSoftDeleteFeatureStateEditable property: This flag is no longer in use. Please use
* 'softDeleteFeatureState' to set the soft delete state for the vault.
*
* @param isSoftDeleteFeatureStateEditable the isSoftDeleteFeatureStateEditable value to set.
* @return the BackupResourceVaultConfig object itself.
*/
public BackupResourceVaultConfig withIsSoftDeleteFeatureStateEditable(Boolean isSoftDeleteFeatureStateEditable) {
this.isSoftDeleteFeatureStateEditable = isSoftDeleteFeatureStateEditable;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy