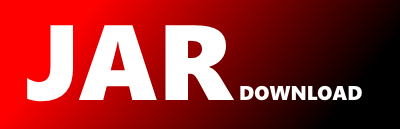
com.azure.resourcemanager.recoveryservicesbackup.models.DpmProtectedItemExtendedInfo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-recoveryservicesbackup Show documentation
Show all versions of azure-resourcemanager-recoveryservicesbackup Show documentation
This package contains Microsoft Azure SDK for RecoveryServicesBackup Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. Open API 2.0 Specs for Azure RecoveryServices Backup service. Package tag package-2024-04.
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.recoveryservicesbackup.models;
import com.azure.core.annotation.Fluent;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import java.time.OffsetDateTime;
import java.util.Map;
/**
* Additional information of DPM Protected item.
*/
@Fluent
public final class DpmProtectedItemExtendedInfo {
/*
* Attribute to provide information on various DBs.
*/
@JsonProperty(value = "protectableObjectLoadPath")
@JsonInclude(value = JsonInclude.Include.NON_NULL, content = JsonInclude.Include.ALWAYS)
private Map protectableObjectLoadPath;
/*
* To check if backup item is disk protected.
*/
@JsonProperty(value = "protected")
private Boolean protectedProperty;
/*
* To check if backup item is cloud protected.
*/
@JsonProperty(value = "isPresentOnCloud")
private Boolean isPresentOnCloud;
/*
* Last backup status information on backup item.
*/
@JsonProperty(value = "lastBackupStatus")
private String lastBackupStatus;
/*
* Last refresh time on backup item.
*/
@JsonProperty(value = "lastRefreshedAt")
private OffsetDateTime lastRefreshedAt;
/*
* Oldest cloud recovery point time.
*/
@JsonProperty(value = "oldestRecoveryPoint")
private OffsetDateTime oldestRecoveryPoint;
/*
* cloud recovery point count.
*/
@JsonProperty(value = "recoveryPointCount")
private Integer recoveryPointCount;
/*
* Oldest disk recovery point time.
*/
@JsonProperty(value = "onPremiseOldestRecoveryPoint")
private OffsetDateTime onPremiseOldestRecoveryPoint;
/*
* latest disk recovery point time.
*/
@JsonProperty(value = "onPremiseLatestRecoveryPoint")
private OffsetDateTime onPremiseLatestRecoveryPoint;
/*
* disk recovery point count.
*/
@JsonProperty(value = "onPremiseRecoveryPointCount")
private Integer onPremiseRecoveryPointCount;
/*
* To check if backup item is collocated.
*/
@JsonProperty(value = "isCollocated")
private Boolean isCollocated;
/*
* Protection group name of the backup item.
*/
@JsonProperty(value = "protectionGroupName")
private String protectionGroupName;
/*
* Used Disk storage in bytes.
*/
@JsonProperty(value = "diskStorageUsedInBytes")
private String diskStorageUsedInBytes;
/*
* total Disk storage in bytes.
*/
@JsonProperty(value = "totalDiskStorageSizeInBytes")
private String totalDiskStorageSizeInBytes;
/**
* Creates an instance of DpmProtectedItemExtendedInfo class.
*/
public DpmProtectedItemExtendedInfo() {
}
/**
* Get the protectableObjectLoadPath property: Attribute to provide information on various DBs.
*
* @return the protectableObjectLoadPath value.
*/
public Map protectableObjectLoadPath() {
return this.protectableObjectLoadPath;
}
/**
* Set the protectableObjectLoadPath property: Attribute to provide information on various DBs.
*
* @param protectableObjectLoadPath the protectableObjectLoadPath value to set.
* @return the DpmProtectedItemExtendedInfo object itself.
*/
public DpmProtectedItemExtendedInfo withProtectableObjectLoadPath(Map protectableObjectLoadPath) {
this.protectableObjectLoadPath = protectableObjectLoadPath;
return this;
}
/**
* Get the protectedProperty property: To check if backup item is disk protected.
*
* @return the protectedProperty value.
*/
public Boolean protectedProperty() {
return this.protectedProperty;
}
/**
* Set the protectedProperty property: To check if backup item is disk protected.
*
* @param protectedProperty the protectedProperty value to set.
* @return the DpmProtectedItemExtendedInfo object itself.
*/
public DpmProtectedItemExtendedInfo withProtectedProperty(Boolean protectedProperty) {
this.protectedProperty = protectedProperty;
return this;
}
/**
* Get the isPresentOnCloud property: To check if backup item is cloud protected.
*
* @return the isPresentOnCloud value.
*/
public Boolean isPresentOnCloud() {
return this.isPresentOnCloud;
}
/**
* Set the isPresentOnCloud property: To check if backup item is cloud protected.
*
* @param isPresentOnCloud the isPresentOnCloud value to set.
* @return the DpmProtectedItemExtendedInfo object itself.
*/
public DpmProtectedItemExtendedInfo withIsPresentOnCloud(Boolean isPresentOnCloud) {
this.isPresentOnCloud = isPresentOnCloud;
return this;
}
/**
* Get the lastBackupStatus property: Last backup status information on backup item.
*
* @return the lastBackupStatus value.
*/
public String lastBackupStatus() {
return this.lastBackupStatus;
}
/**
* Set the lastBackupStatus property: Last backup status information on backup item.
*
* @param lastBackupStatus the lastBackupStatus value to set.
* @return the DpmProtectedItemExtendedInfo object itself.
*/
public DpmProtectedItemExtendedInfo withLastBackupStatus(String lastBackupStatus) {
this.lastBackupStatus = lastBackupStatus;
return this;
}
/**
* Get the lastRefreshedAt property: Last refresh time on backup item.
*
* @return the lastRefreshedAt value.
*/
public OffsetDateTime lastRefreshedAt() {
return this.lastRefreshedAt;
}
/**
* Set the lastRefreshedAt property: Last refresh time on backup item.
*
* @param lastRefreshedAt the lastRefreshedAt value to set.
* @return the DpmProtectedItemExtendedInfo object itself.
*/
public DpmProtectedItemExtendedInfo withLastRefreshedAt(OffsetDateTime lastRefreshedAt) {
this.lastRefreshedAt = lastRefreshedAt;
return this;
}
/**
* Get the oldestRecoveryPoint property: Oldest cloud recovery point time.
*
* @return the oldestRecoveryPoint value.
*/
public OffsetDateTime oldestRecoveryPoint() {
return this.oldestRecoveryPoint;
}
/**
* Set the oldestRecoveryPoint property: Oldest cloud recovery point time.
*
* @param oldestRecoveryPoint the oldestRecoveryPoint value to set.
* @return the DpmProtectedItemExtendedInfo object itself.
*/
public DpmProtectedItemExtendedInfo withOldestRecoveryPoint(OffsetDateTime oldestRecoveryPoint) {
this.oldestRecoveryPoint = oldestRecoveryPoint;
return this;
}
/**
* Get the recoveryPointCount property: cloud recovery point count.
*
* @return the recoveryPointCount value.
*/
public Integer recoveryPointCount() {
return this.recoveryPointCount;
}
/**
* Set the recoveryPointCount property: cloud recovery point count.
*
* @param recoveryPointCount the recoveryPointCount value to set.
* @return the DpmProtectedItemExtendedInfo object itself.
*/
public DpmProtectedItemExtendedInfo withRecoveryPointCount(Integer recoveryPointCount) {
this.recoveryPointCount = recoveryPointCount;
return this;
}
/**
* Get the onPremiseOldestRecoveryPoint property: Oldest disk recovery point time.
*
* @return the onPremiseOldestRecoveryPoint value.
*/
public OffsetDateTime onPremiseOldestRecoveryPoint() {
return this.onPremiseOldestRecoveryPoint;
}
/**
* Set the onPremiseOldestRecoveryPoint property: Oldest disk recovery point time.
*
* @param onPremiseOldestRecoveryPoint the onPremiseOldestRecoveryPoint value to set.
* @return the DpmProtectedItemExtendedInfo object itself.
*/
public DpmProtectedItemExtendedInfo withOnPremiseOldestRecoveryPoint(OffsetDateTime onPremiseOldestRecoveryPoint) {
this.onPremiseOldestRecoveryPoint = onPremiseOldestRecoveryPoint;
return this;
}
/**
* Get the onPremiseLatestRecoveryPoint property: latest disk recovery point time.
*
* @return the onPremiseLatestRecoveryPoint value.
*/
public OffsetDateTime onPremiseLatestRecoveryPoint() {
return this.onPremiseLatestRecoveryPoint;
}
/**
* Set the onPremiseLatestRecoveryPoint property: latest disk recovery point time.
*
* @param onPremiseLatestRecoveryPoint the onPremiseLatestRecoveryPoint value to set.
* @return the DpmProtectedItemExtendedInfo object itself.
*/
public DpmProtectedItemExtendedInfo withOnPremiseLatestRecoveryPoint(OffsetDateTime onPremiseLatestRecoveryPoint) {
this.onPremiseLatestRecoveryPoint = onPremiseLatestRecoveryPoint;
return this;
}
/**
* Get the onPremiseRecoveryPointCount property: disk recovery point count.
*
* @return the onPremiseRecoveryPointCount value.
*/
public Integer onPremiseRecoveryPointCount() {
return this.onPremiseRecoveryPointCount;
}
/**
* Set the onPremiseRecoveryPointCount property: disk recovery point count.
*
* @param onPremiseRecoveryPointCount the onPremiseRecoveryPointCount value to set.
* @return the DpmProtectedItemExtendedInfo object itself.
*/
public DpmProtectedItemExtendedInfo withOnPremiseRecoveryPointCount(Integer onPremiseRecoveryPointCount) {
this.onPremiseRecoveryPointCount = onPremiseRecoveryPointCount;
return this;
}
/**
* Get the isCollocated property: To check if backup item is collocated.
*
* @return the isCollocated value.
*/
public Boolean isCollocated() {
return this.isCollocated;
}
/**
* Set the isCollocated property: To check if backup item is collocated.
*
* @param isCollocated the isCollocated value to set.
* @return the DpmProtectedItemExtendedInfo object itself.
*/
public DpmProtectedItemExtendedInfo withIsCollocated(Boolean isCollocated) {
this.isCollocated = isCollocated;
return this;
}
/**
* Get the protectionGroupName property: Protection group name of the backup item.
*
* @return the protectionGroupName value.
*/
public String protectionGroupName() {
return this.protectionGroupName;
}
/**
* Set the protectionGroupName property: Protection group name of the backup item.
*
* @param protectionGroupName the protectionGroupName value to set.
* @return the DpmProtectedItemExtendedInfo object itself.
*/
public DpmProtectedItemExtendedInfo withProtectionGroupName(String protectionGroupName) {
this.protectionGroupName = protectionGroupName;
return this;
}
/**
* Get the diskStorageUsedInBytes property: Used Disk storage in bytes.
*
* @return the diskStorageUsedInBytes value.
*/
public String diskStorageUsedInBytes() {
return this.diskStorageUsedInBytes;
}
/**
* Set the diskStorageUsedInBytes property: Used Disk storage in bytes.
*
* @param diskStorageUsedInBytes the diskStorageUsedInBytes value to set.
* @return the DpmProtectedItemExtendedInfo object itself.
*/
public DpmProtectedItemExtendedInfo withDiskStorageUsedInBytes(String diskStorageUsedInBytes) {
this.diskStorageUsedInBytes = diskStorageUsedInBytes;
return this;
}
/**
* Get the totalDiskStorageSizeInBytes property: total Disk storage in bytes.
*
* @return the totalDiskStorageSizeInBytes value.
*/
public String totalDiskStorageSizeInBytes() {
return this.totalDiskStorageSizeInBytes;
}
/**
* Set the totalDiskStorageSizeInBytes property: total Disk storage in bytes.
*
* @param totalDiskStorageSizeInBytes the totalDiskStorageSizeInBytes value to set.
* @return the DpmProtectedItemExtendedInfo object itself.
*/
public DpmProtectedItemExtendedInfo withTotalDiskStorageSizeInBytes(String totalDiskStorageSizeInBytes) {
this.totalDiskStorageSizeInBytes = totalDiskStorageSizeInBytes;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy