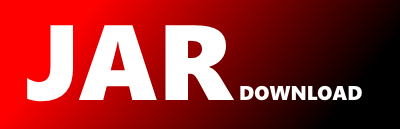
com.azure.resourcemanager.recoveryservicesbackup.models.PreValidateEnableBackupRequest Maven / Gradle / Ivy
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.recoveryservicesbackup.models;
import com.azure.core.annotation.Fluent;
import com.fasterxml.jackson.annotation.JsonProperty;
/**
* Contract to validate if backup can be enabled on the given resource in a given vault and given configuration.
* It will validate followings
* 1. Vault capacity
* 2. VM is already protected
* 3. Any VM related configuration passed in properties.
*/
@Fluent
public final class PreValidateEnableBackupRequest {
/*
* ProtectedItem Type- VM, SqlDataBase, AzureFileShare etc
*/
@JsonProperty(value = "resourceType")
private DataSourceType resourceType;
/*
* ARM Virtual Machine Id
*/
@JsonProperty(value = "resourceId")
private String resourceId;
/*
* ARM id of the Recovery Services Vault
*/
@JsonProperty(value = "vaultId")
private String vaultId;
/*
* Configuration of VM if any needs to be validated like OS type etc
*/
@JsonProperty(value = "properties")
private String properties;
/**
* Creates an instance of PreValidateEnableBackupRequest class.
*/
public PreValidateEnableBackupRequest() {
}
/**
* Get the resourceType property: ProtectedItem Type- VM, SqlDataBase, AzureFileShare etc.
*
* @return the resourceType value.
*/
public DataSourceType resourceType() {
return this.resourceType;
}
/**
* Set the resourceType property: ProtectedItem Type- VM, SqlDataBase, AzureFileShare etc.
*
* @param resourceType the resourceType value to set.
* @return the PreValidateEnableBackupRequest object itself.
*/
public PreValidateEnableBackupRequest withResourceType(DataSourceType resourceType) {
this.resourceType = resourceType;
return this;
}
/**
* Get the resourceId property: ARM Virtual Machine Id.
*
* @return the resourceId value.
*/
public String resourceId() {
return this.resourceId;
}
/**
* Set the resourceId property: ARM Virtual Machine Id.
*
* @param resourceId the resourceId value to set.
* @return the PreValidateEnableBackupRequest object itself.
*/
public PreValidateEnableBackupRequest withResourceId(String resourceId) {
this.resourceId = resourceId;
return this;
}
/**
* Get the vaultId property: ARM id of the Recovery Services Vault.
*
* @return the vaultId value.
*/
public String vaultId() {
return this.vaultId;
}
/**
* Set the vaultId property: ARM id of the Recovery Services Vault.
*
* @param vaultId the vaultId value to set.
* @return the PreValidateEnableBackupRequest object itself.
*/
public PreValidateEnableBackupRequest withVaultId(String vaultId) {
this.vaultId = vaultId;
return this;
}
/**
* Get the properties property: Configuration of VM if any needs to be validated like OS type etc.
*
* @return the properties value.
*/
public String properties() {
return this.properties;
}
/**
* Set the properties property: Configuration of VM if any needs to be validated like OS type etc.
*
* @param properties the properties value to set.
* @return the PreValidateEnableBackupRequest object itself.
*/
public PreValidateEnableBackupRequest withProperties(String properties) {
this.properties = properties;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy