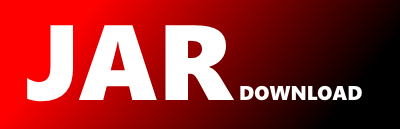
com.azure.resourcemanager.search.fluent.models.SearchServiceProperties Maven / Gradle / Ivy
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.search.fluent.models;
import com.azure.core.annotation.Fluent;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import com.azure.resourcemanager.search.models.DataPlaneAuthOptions;
import com.azure.resourcemanager.search.models.EncryptionWithCmk;
import com.azure.resourcemanager.search.models.HostingMode;
import com.azure.resourcemanager.search.models.NetworkRuleSet;
import com.azure.resourcemanager.search.models.ProvisioningState;
import com.azure.resourcemanager.search.models.PublicNetworkAccess;
import com.azure.resourcemanager.search.models.SearchSemanticSearch;
import com.azure.resourcemanager.search.models.SearchServiceStatus;
import java.io.IOException;
import java.util.List;
/**
* Properties of the search service.
*/
@Fluent
public final class SearchServiceProperties implements JsonSerializable {
/*
* The number of replicas in the search service. If specified, it must be a value between 1 and 12 inclusive for
* standard SKUs or between 1 and 3 inclusive for basic SKU.
*/
private Integer replicaCount;
/*
* The number of partitions in the search service; if specified, it can be 1, 2, 3, 4, 6, or 12. Values greater than
* 1 are only valid for standard SKUs. For 'standard3' services with hostingMode set to 'highDensity', the allowed
* values are between 1 and 3.
*/
private Integer partitionCount;
/*
* Applicable only for the standard3 SKU. You can set this property to enable up to 3 high density partitions that
* allow up to 1000 indexes, which is much higher than the maximum indexes allowed for any other SKU. For the
* standard3 SKU, the value is either 'default' or 'highDensity'. For all other SKUs, this value must be 'default'.
*/
private HostingMode hostingMode;
/*
* This value can be set to 'enabled' to avoid breaking changes on existing customer resources and templates. If set
* to 'disabled', traffic over public interface is not allowed, and private endpoint connections would be the
* exclusive access method.
*/
private PublicNetworkAccess publicNetworkAccess;
/*
* The status of the search service. Possible values include: 'running': The search service is running and no
* provisioning operations are underway. 'provisioning': The search service is being provisioned or scaled up or
* down. 'deleting': The search service is being deleted. 'degraded': The search service is degraded. This can occur
* when the underlying search units are not healthy. The search service is most likely operational, but performance
* might be slow and some requests might be dropped. 'disabled': The search service is disabled. In this state, the
* service will reject all API requests. 'error': The search service is in an error state. If your service is in the
* degraded, disabled, or error states, Microsoft is actively investigating the underlying issue. Dedicated services
* in these states are still chargeable based on the number of search units provisioned.
*/
private SearchServiceStatus status;
/*
* The details of the search service status.
*/
private String statusDetails;
/*
* The state of the last provisioning operation performed on the search service. Provisioning is an intermediate
* state that occurs while service capacity is being established. After capacity is set up, provisioningState
* changes to either 'succeeded' or 'failed'. Client applications can poll provisioning status (the recommended
* polling interval is from 30 seconds to one minute) by using the Get Search Service operation to see when an
* operation is completed. If you are using the free service, this value tends to come back as 'succeeded' directly
* in the call to Create search service. This is because the free service uses capacity that is already set up.
*/
private ProvisioningState provisioningState;
/*
* Network-specific rules that determine how the search service may be reached.
*/
private NetworkRuleSet networkRuleSet;
/*
* Specifies any policy regarding encryption of resources (such as indexes) using customer manager keys within a
* search service.
*/
private EncryptionWithCmk encryptionWithCmk;
/*
* When set to true, calls to the search service will not be permitted to utilize API keys for authentication. This
* cannot be set to true if 'dataPlaneAuthOptions' are defined.
*/
private Boolean disableLocalAuth;
/*
* Defines the options for how the data plane API of a search service authenticates requests. This cannot be set if
* 'disableLocalAuth' is set to true.
*/
private DataPlaneAuthOptions authOptions;
/*
* The list of private endpoint connections to the search service.
*/
private List privateEndpointConnections;
/*
* Sets options that control the availability of semantic search. This configuration is only possible for certain
* search SKUs in certain locations.
*/
private SearchSemanticSearch semanticSearch;
/*
* The list of shared private link resources managed by the search service.
*/
private List sharedPrivateLinkResources;
/**
* Creates an instance of SearchServiceProperties class.
*/
public SearchServiceProperties() {
}
/**
* Get the replicaCount property: The number of replicas in the search service. If specified, it must be a value
* between 1 and 12 inclusive for standard SKUs or between 1 and 3 inclusive for basic SKU.
*
* @return the replicaCount value.
*/
public Integer replicaCount() {
return this.replicaCount;
}
/**
* Set the replicaCount property: The number of replicas in the search service. If specified, it must be a value
* between 1 and 12 inclusive for standard SKUs or between 1 and 3 inclusive for basic SKU.
*
* @param replicaCount the replicaCount value to set.
* @return the SearchServiceProperties object itself.
*/
public SearchServiceProperties withReplicaCount(Integer replicaCount) {
this.replicaCount = replicaCount;
return this;
}
/**
* Get the partitionCount property: The number of partitions in the search service; if specified, it can be 1, 2, 3,
* 4, 6, or 12. Values greater than 1 are only valid for standard SKUs. For 'standard3' services with hostingMode
* set to 'highDensity', the allowed values are between 1 and 3.
*
* @return the partitionCount value.
*/
public Integer partitionCount() {
return this.partitionCount;
}
/**
* Set the partitionCount property: The number of partitions in the search service; if specified, it can be 1, 2, 3,
* 4, 6, or 12. Values greater than 1 are only valid for standard SKUs. For 'standard3' services with hostingMode
* set to 'highDensity', the allowed values are between 1 and 3.
*
* @param partitionCount the partitionCount value to set.
* @return the SearchServiceProperties object itself.
*/
public SearchServiceProperties withPartitionCount(Integer partitionCount) {
this.partitionCount = partitionCount;
return this;
}
/**
* Get the hostingMode property: Applicable only for the standard3 SKU. You can set this property to enable up to 3
* high density partitions that allow up to 1000 indexes, which is much higher than the maximum indexes allowed for
* any other SKU. For the standard3 SKU, the value is either 'default' or 'highDensity'. For all other SKUs, this
* value must be 'default'.
*
* @return the hostingMode value.
*/
public HostingMode hostingMode() {
return this.hostingMode;
}
/**
* Set the hostingMode property: Applicable only for the standard3 SKU. You can set this property to enable up to 3
* high density partitions that allow up to 1000 indexes, which is much higher than the maximum indexes allowed for
* any other SKU. For the standard3 SKU, the value is either 'default' or 'highDensity'. For all other SKUs, this
* value must be 'default'.
*
* @param hostingMode the hostingMode value to set.
* @return the SearchServiceProperties object itself.
*/
public SearchServiceProperties withHostingMode(HostingMode hostingMode) {
this.hostingMode = hostingMode;
return this;
}
/**
* Get the publicNetworkAccess property: This value can be set to 'enabled' to avoid breaking changes on existing
* customer resources and templates. If set to 'disabled', traffic over public interface is not allowed, and private
* endpoint connections would be the exclusive access method.
*
* @return the publicNetworkAccess value.
*/
public PublicNetworkAccess publicNetworkAccess() {
return this.publicNetworkAccess;
}
/**
* Set the publicNetworkAccess property: This value can be set to 'enabled' to avoid breaking changes on existing
* customer resources and templates. If set to 'disabled', traffic over public interface is not allowed, and private
* endpoint connections would be the exclusive access method.
*
* @param publicNetworkAccess the publicNetworkAccess value to set.
* @return the SearchServiceProperties object itself.
*/
public SearchServiceProperties withPublicNetworkAccess(PublicNetworkAccess publicNetworkAccess) {
this.publicNetworkAccess = publicNetworkAccess;
return this;
}
/**
* Get the status property: The status of the search service. Possible values include: 'running': The search service
* is running and no provisioning operations are underway. 'provisioning': The search service is being provisioned
* or scaled up or down. 'deleting': The search service is being deleted. 'degraded': The search service is
* degraded. This can occur when the underlying search units are not healthy. The search service is most likely
* operational, but performance might be slow and some requests might be dropped. 'disabled': The search service is
* disabled. In this state, the service will reject all API requests. 'error': The search service is in an error
* state. If your service is in the degraded, disabled, or error states, Microsoft is actively investigating the
* underlying issue. Dedicated services in these states are still chargeable based on the number of search units
* provisioned.
*
* @return the status value.
*/
public SearchServiceStatus status() {
return this.status;
}
/**
* Get the statusDetails property: The details of the search service status.
*
* @return the statusDetails value.
*/
public String statusDetails() {
return this.statusDetails;
}
/**
* Get the provisioningState property: The state of the last provisioning operation performed on the search service.
* Provisioning is an intermediate state that occurs while service capacity is being established. After capacity is
* set up, provisioningState changes to either 'succeeded' or 'failed'. Client applications can poll provisioning
* status (the recommended polling interval is from 30 seconds to one minute) by using the Get Search Service
* operation to see when an operation is completed. If you are using the free service, this value tends to come back
* as 'succeeded' directly in the call to Create search service. This is because the free service uses capacity that
* is already set up.
*
* @return the provisioningState value.
*/
public ProvisioningState provisioningState() {
return this.provisioningState;
}
/**
* Get the networkRuleSet property: Network-specific rules that determine how the search service may be reached.
*
* @return the networkRuleSet value.
*/
public NetworkRuleSet networkRuleSet() {
return this.networkRuleSet;
}
/**
* Set the networkRuleSet property: Network-specific rules that determine how the search service may be reached.
*
* @param networkRuleSet the networkRuleSet value to set.
* @return the SearchServiceProperties object itself.
*/
public SearchServiceProperties withNetworkRuleSet(NetworkRuleSet networkRuleSet) {
this.networkRuleSet = networkRuleSet;
return this;
}
/**
* Get the encryptionWithCmk property: Specifies any policy regarding encryption of resources (such as indexes)
* using customer manager keys within a search service.
*
* @return the encryptionWithCmk value.
*/
public EncryptionWithCmk encryptionWithCmk() {
return this.encryptionWithCmk;
}
/**
* Set the encryptionWithCmk property: Specifies any policy regarding encryption of resources (such as indexes)
* using customer manager keys within a search service.
*
* @param encryptionWithCmk the encryptionWithCmk value to set.
* @return the SearchServiceProperties object itself.
*/
public SearchServiceProperties withEncryptionWithCmk(EncryptionWithCmk encryptionWithCmk) {
this.encryptionWithCmk = encryptionWithCmk;
return this;
}
/**
* Get the disableLocalAuth property: When set to true, calls to the search service will not be permitted to utilize
* API keys for authentication. This cannot be set to true if 'dataPlaneAuthOptions' are defined.
*
* @return the disableLocalAuth value.
*/
public Boolean disableLocalAuth() {
return this.disableLocalAuth;
}
/**
* Set the disableLocalAuth property: When set to true, calls to the search service will not be permitted to utilize
* API keys for authentication. This cannot be set to true if 'dataPlaneAuthOptions' are defined.
*
* @param disableLocalAuth the disableLocalAuth value to set.
* @return the SearchServiceProperties object itself.
*/
public SearchServiceProperties withDisableLocalAuth(Boolean disableLocalAuth) {
this.disableLocalAuth = disableLocalAuth;
return this;
}
/**
* Get the authOptions property: Defines the options for how the data plane API of a search service authenticates
* requests. This cannot be set if 'disableLocalAuth' is set to true.
*
* @return the authOptions value.
*/
public DataPlaneAuthOptions authOptions() {
return this.authOptions;
}
/**
* Set the authOptions property: Defines the options for how the data plane API of a search service authenticates
* requests. This cannot be set if 'disableLocalAuth' is set to true.
*
* @param authOptions the authOptions value to set.
* @return the SearchServiceProperties object itself.
*/
public SearchServiceProperties withAuthOptions(DataPlaneAuthOptions authOptions) {
this.authOptions = authOptions;
return this;
}
/**
* Get the privateEndpointConnections property: The list of private endpoint connections to the search service.
*
* @return the privateEndpointConnections value.
*/
public List privateEndpointConnections() {
return this.privateEndpointConnections;
}
/**
* Get the semanticSearch property: Sets options that control the availability of semantic search. This
* configuration is only possible for certain search SKUs in certain locations.
*
* @return the semanticSearch value.
*/
public SearchSemanticSearch semanticSearch() {
return this.semanticSearch;
}
/**
* Set the semanticSearch property: Sets options that control the availability of semantic search. This
* configuration is only possible for certain search SKUs in certain locations.
*
* @param semanticSearch the semanticSearch value to set.
* @return the SearchServiceProperties object itself.
*/
public SearchServiceProperties withSemanticSearch(SearchSemanticSearch semanticSearch) {
this.semanticSearch = semanticSearch;
return this;
}
/**
* Get the sharedPrivateLinkResources property: The list of shared private link resources managed by the search
* service.
*
* @return the sharedPrivateLinkResources value.
*/
public List sharedPrivateLinkResources() {
return this.sharedPrivateLinkResources;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (networkRuleSet() != null) {
networkRuleSet().validate();
}
if (encryptionWithCmk() != null) {
encryptionWithCmk().validate();
}
if (authOptions() != null) {
authOptions().validate();
}
if (privateEndpointConnections() != null) {
privateEndpointConnections().forEach(e -> e.validate());
}
if (sharedPrivateLinkResources() != null) {
sharedPrivateLinkResources().forEach(e -> e.validate());
}
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeNumberField("replicaCount", this.replicaCount);
jsonWriter.writeNumberField("partitionCount", this.partitionCount);
jsonWriter.writeStringField("hostingMode", this.hostingMode == null ? null : this.hostingMode.toString());
jsonWriter.writeStringField("publicNetworkAccess",
this.publicNetworkAccess == null ? null : this.publicNetworkAccess.toString());
jsonWriter.writeJsonField("networkRuleSet", this.networkRuleSet);
jsonWriter.writeJsonField("encryptionWithCmk", this.encryptionWithCmk);
jsonWriter.writeBooleanField("disableLocalAuth", this.disableLocalAuth);
jsonWriter.writeJsonField("authOptions", this.authOptions);
jsonWriter.writeStringField("semanticSearch",
this.semanticSearch == null ? null : this.semanticSearch.toString());
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of SearchServiceProperties from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of SearchServiceProperties if the JsonReader was pointing to an instance of it, or null if it
* was pointing to JSON null.
* @throws IOException If an error occurs while reading the SearchServiceProperties.
*/
public static SearchServiceProperties fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
SearchServiceProperties deserializedSearchServiceProperties = new SearchServiceProperties();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("replicaCount".equals(fieldName)) {
deserializedSearchServiceProperties.replicaCount = reader.getNullable(JsonReader::getInt);
} else if ("partitionCount".equals(fieldName)) {
deserializedSearchServiceProperties.partitionCount = reader.getNullable(JsonReader::getInt);
} else if ("hostingMode".equals(fieldName)) {
deserializedSearchServiceProperties.hostingMode = HostingMode.fromString(reader.getString());
} else if ("publicNetworkAccess".equals(fieldName)) {
deserializedSearchServiceProperties.publicNetworkAccess
= PublicNetworkAccess.fromString(reader.getString());
} else if ("status".equals(fieldName)) {
deserializedSearchServiceProperties.status = SearchServiceStatus.fromString(reader.getString());
} else if ("statusDetails".equals(fieldName)) {
deserializedSearchServiceProperties.statusDetails = reader.getString();
} else if ("provisioningState".equals(fieldName)) {
deserializedSearchServiceProperties.provisioningState
= ProvisioningState.fromString(reader.getString());
} else if ("networkRuleSet".equals(fieldName)) {
deserializedSearchServiceProperties.networkRuleSet = NetworkRuleSet.fromJson(reader);
} else if ("encryptionWithCmk".equals(fieldName)) {
deserializedSearchServiceProperties.encryptionWithCmk = EncryptionWithCmk.fromJson(reader);
} else if ("disableLocalAuth".equals(fieldName)) {
deserializedSearchServiceProperties.disableLocalAuth = reader.getNullable(JsonReader::getBoolean);
} else if ("authOptions".equals(fieldName)) {
deserializedSearchServiceProperties.authOptions = DataPlaneAuthOptions.fromJson(reader);
} else if ("privateEndpointConnections".equals(fieldName)) {
List privateEndpointConnections
= reader.readArray(reader1 -> PrivateEndpointConnectionInner.fromJson(reader1));
deserializedSearchServiceProperties.privateEndpointConnections = privateEndpointConnections;
} else if ("semanticSearch".equals(fieldName)) {
deserializedSearchServiceProperties.semanticSearch
= SearchSemanticSearch.fromString(reader.getString());
} else if ("sharedPrivateLinkResources".equals(fieldName)) {
List sharedPrivateLinkResources
= reader.readArray(reader1 -> SharedPrivateLinkResourceInner.fromJson(reader1));
deserializedSearchServiceProperties.sharedPrivateLinkResources = sharedPrivateLinkResources;
} else {
reader.skipChildren();
}
}
return deserializedSearchServiceProperties;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy