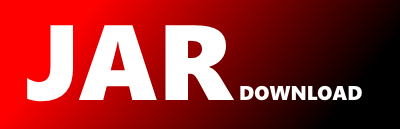
com.azure.resourcemanager.security.fluent.models.AlertProperties Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-security Show documentation
Show all versions of azure-resourcemanager-security Show documentation
This package contains Microsoft Azure SDK for Security Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. API spec for Microsoft.Security (Azure Security Center) resource provider. Package tag package-composite-v3.
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.security.fluent.models;
import com.azure.core.annotation.Fluent;
import com.azure.core.util.CoreUtils;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import com.azure.resourcemanager.security.models.AlertEntity;
import com.azure.resourcemanager.security.models.AlertPropertiesSupportingEvidence;
import com.azure.resourcemanager.security.models.AlertSeverity;
import com.azure.resourcemanager.security.models.AlertStatus;
import com.azure.resourcemanager.security.models.Intent;
import com.azure.resourcemanager.security.models.ResourceIdentifier;
import java.io.IOException;
import java.time.OffsetDateTime;
import java.util.List;
import java.util.Map;
/**
* describes security alert properties.
*/
@Fluent
public final class AlertProperties implements JsonSerializable {
/*
* Schema version.
*/
private String version;
/*
* Unique identifier for the detection logic (all alert instances from the same detection logic will have the same
* alertType).
*/
private String alertType;
/*
* Unique identifier for the alert.
*/
private String systemAlertId;
/*
* The name of Azure Security Center pricing tier which powering this alert. Learn more:
* https://docs.microsoft.com/en-us/azure/security-center/security-center-pricing
*/
private String productComponentName;
/*
* The display name of the alert.
*/
private String alertDisplayName;
/*
* Description of the suspicious activity that was detected.
*/
private String description;
/*
* The risk level of the threat that was detected. Learn more:
* https://docs.microsoft.com/en-us/azure/security-center/security-center-alerts-overview#how-are-alerts-classified.
*/
private AlertSeverity severity;
/*
* The kill chain related intent behind the alert. For list of supported values, and explanations of Azure Security
* Center's supported kill chain intents.
*/
private Intent intent;
/*
* The UTC time of the first event or activity included in the alert in ISO8601 format.
*/
private OffsetDateTime startTimeUtc;
/*
* The UTC time of the last event or activity included in the alert in ISO8601 format.
*/
private OffsetDateTime endTimeUtc;
/*
* The resource identifiers that can be used to direct the alert to the right product exposure group (tenant,
* workspace, subscription etc.). There can be multiple identifiers of different type per alert.
*/
private List resourceIdentifiers;
/*
* Manual action items to take to remediate the alert.
*/
private List remediationSteps;
/*
* The name of the vendor that raises the alert.
*/
private String vendorName;
/*
* The life cycle status of the alert.
*/
private AlertStatus status;
/*
* Links related to the alert
*/
private List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy