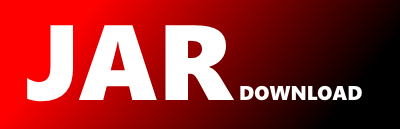
com.azure.resourcemanager.security.fluent.models.GovernanceRuleInner Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-security Show documentation
Show all versions of azure-resourcemanager-security Show documentation
This package contains Microsoft Azure SDK for Security Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. API spec for Microsoft.Security (Azure Security Center) resource provider. Package tag package-composite-v3.
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.security.fluent.models;
import com.azure.core.annotation.Fluent;
import com.azure.core.management.ProxyResource;
import com.azure.json.JsonReader;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import com.azure.resourcemanager.security.models.GovernanceRuleEmailNotification;
import com.azure.resourcemanager.security.models.GovernanceRuleMetadata;
import com.azure.resourcemanager.security.models.GovernanceRuleOwnerSource;
import com.azure.resourcemanager.security.models.GovernanceRuleSourceResourceType;
import com.azure.resourcemanager.security.models.GovernanceRuleType;
import java.io.IOException;
import java.util.List;
/**
* Governance rule over a given scope.
*/
@Fluent
public final class GovernanceRuleInner extends ProxyResource {
/*
* Properties of a governance rule
*/
private GovernanceRuleProperties innerProperties;
/*
* The type of the resource.
*/
private String type;
/*
* The name of the resource.
*/
private String name;
/*
* Fully qualified resource Id for the resource.
*/
private String id;
/**
* Creates an instance of GovernanceRuleInner class.
*/
public GovernanceRuleInner() {
}
/**
* Get the innerProperties property: Properties of a governance rule.
*
* @return the innerProperties value.
*/
private GovernanceRuleProperties innerProperties() {
return this.innerProperties;
}
/**
* Get the type property: The type of the resource.
*
* @return the type value.
*/
@Override
public String type() {
return this.type;
}
/**
* Get the name property: The name of the resource.
*
* @return the name value.
*/
@Override
public String name() {
return this.name;
}
/**
* Get the id property: Fully qualified resource Id for the resource.
*
* @return the id value.
*/
@Override
public String id() {
return this.id;
}
/**
* Get the tenantId property: The tenantId (GUID).
*
* @return the tenantId value.
*/
public String tenantId() {
return this.innerProperties() == null ? null : this.innerProperties().tenantId();
}
/**
* Get the displayName property: Display name of the governance rule.
*
* @return the displayName value.
*/
public String displayName() {
return this.innerProperties() == null ? null : this.innerProperties().displayName();
}
/**
* Set the displayName property: Display name of the governance rule.
*
* @param displayName the displayName value to set.
* @return the GovernanceRuleInner object itself.
*/
public GovernanceRuleInner withDisplayName(String displayName) {
if (this.innerProperties() == null) {
this.innerProperties = new GovernanceRuleProperties();
}
this.innerProperties().withDisplayName(displayName);
return this;
}
/**
* Get the description property: Description of the governance rule.
*
* @return the description value.
*/
public String description() {
return this.innerProperties() == null ? null : this.innerProperties().description();
}
/**
* Set the description property: Description of the governance rule.
*
* @param description the description value to set.
* @return the GovernanceRuleInner object itself.
*/
public GovernanceRuleInner withDescription(String description) {
if (this.innerProperties() == null) {
this.innerProperties = new GovernanceRuleProperties();
}
this.innerProperties().withDescription(description);
return this;
}
/**
* Get the remediationTimeframe property: Governance rule remediation timeframe - this is the time that will affect
* on the grace-period duration e.g. 7.00:00:00 - means 7 days.
*
* @return the remediationTimeframe value.
*/
public String remediationTimeframe() {
return this.innerProperties() == null ? null : this.innerProperties().remediationTimeframe();
}
/**
* Set the remediationTimeframe property: Governance rule remediation timeframe - this is the time that will affect
* on the grace-period duration e.g. 7.00:00:00 - means 7 days.
*
* @param remediationTimeframe the remediationTimeframe value to set.
* @return the GovernanceRuleInner object itself.
*/
public GovernanceRuleInner withRemediationTimeframe(String remediationTimeframe) {
if (this.innerProperties() == null) {
this.innerProperties = new GovernanceRuleProperties();
}
this.innerProperties().withRemediationTimeframe(remediationTimeframe);
return this;
}
/**
* Get the isGracePeriod property: Defines whether there is a grace period on the governance rule.
*
* @return the isGracePeriod value.
*/
public Boolean isGracePeriod() {
return this.innerProperties() == null ? null : this.innerProperties().isGracePeriod();
}
/**
* Set the isGracePeriod property: Defines whether there is a grace period on the governance rule.
*
* @param isGracePeriod the isGracePeriod value to set.
* @return the GovernanceRuleInner object itself.
*/
public GovernanceRuleInner withIsGracePeriod(Boolean isGracePeriod) {
if (this.innerProperties() == null) {
this.innerProperties = new GovernanceRuleProperties();
}
this.innerProperties().withIsGracePeriod(isGracePeriod);
return this;
}
/**
* Get the rulePriority property: The governance rule priority, priority to the lower number. Rules with the same
* priority on the same scope will not be allowed.
*
* @return the rulePriority value.
*/
public Integer rulePriority() {
return this.innerProperties() == null ? null : this.innerProperties().rulePriority();
}
/**
* Set the rulePriority property: The governance rule priority, priority to the lower number. Rules with the same
* priority on the same scope will not be allowed.
*
* @param rulePriority the rulePriority value to set.
* @return the GovernanceRuleInner object itself.
*/
public GovernanceRuleInner withRulePriority(Integer rulePriority) {
if (this.innerProperties() == null) {
this.innerProperties = new GovernanceRuleProperties();
}
this.innerProperties().withRulePriority(rulePriority);
return this;
}
/**
* Get the isDisabled property: Defines whether the rule is active/inactive.
*
* @return the isDisabled value.
*/
public Boolean isDisabled() {
return this.innerProperties() == null ? null : this.innerProperties().isDisabled();
}
/**
* Set the isDisabled property: Defines whether the rule is active/inactive.
*
* @param isDisabled the isDisabled value to set.
* @return the GovernanceRuleInner object itself.
*/
public GovernanceRuleInner withIsDisabled(Boolean isDisabled) {
if (this.innerProperties() == null) {
this.innerProperties = new GovernanceRuleProperties();
}
this.innerProperties().withIsDisabled(isDisabled);
return this;
}
/**
* Get the ruleType property: The rule type of the governance rule, defines the source of the rule e.g. Integrated.
*
* @return the ruleType value.
*/
public GovernanceRuleType ruleType() {
return this.innerProperties() == null ? null : this.innerProperties().ruleType();
}
/**
* Set the ruleType property: The rule type of the governance rule, defines the source of the rule e.g. Integrated.
*
* @param ruleType the ruleType value to set.
* @return the GovernanceRuleInner object itself.
*/
public GovernanceRuleInner withRuleType(GovernanceRuleType ruleType) {
if (this.innerProperties() == null) {
this.innerProperties = new GovernanceRuleProperties();
}
this.innerProperties().withRuleType(ruleType);
return this;
}
/**
* Get the sourceResourceType property: The governance rule source, what the rule affects, e.g. Assessments.
*
* @return the sourceResourceType value.
*/
public GovernanceRuleSourceResourceType sourceResourceType() {
return this.innerProperties() == null ? null : this.innerProperties().sourceResourceType();
}
/**
* Set the sourceResourceType property: The governance rule source, what the rule affects, e.g. Assessments.
*
* @param sourceResourceType the sourceResourceType value to set.
* @return the GovernanceRuleInner object itself.
*/
public GovernanceRuleInner withSourceResourceType(GovernanceRuleSourceResourceType sourceResourceType) {
if (this.innerProperties() == null) {
this.innerProperties = new GovernanceRuleProperties();
}
this.innerProperties().withSourceResourceType(sourceResourceType);
return this;
}
/**
* Get the excludedScopes property: Excluded scopes, filter out the descendants of the scope (on management scopes).
*
* @return the excludedScopes value.
*/
public List excludedScopes() {
return this.innerProperties() == null ? null : this.innerProperties().excludedScopes();
}
/**
* Set the excludedScopes property: Excluded scopes, filter out the descendants of the scope (on management scopes).
*
* @param excludedScopes the excludedScopes value to set.
* @return the GovernanceRuleInner object itself.
*/
public GovernanceRuleInner withExcludedScopes(List excludedScopes) {
if (this.innerProperties() == null) {
this.innerProperties = new GovernanceRuleProperties();
}
this.innerProperties().withExcludedScopes(excludedScopes);
return this;
}
/**
* Get the conditionSets property: The governance rule conditionSets - see examples.
*
* @return the conditionSets value.
*/
public List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy