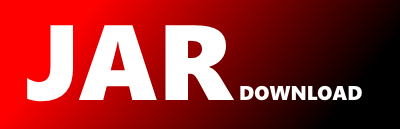
com.azure.resourcemanager.security.fluent.models.HealthReportProperties Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-security Show documentation
Show all versions of azure-resourcemanager-security Show documentation
This package contains Microsoft Azure SDK for Security Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. API spec for Microsoft.Security (Azure Security Center) resource provider. Package tag package-composite-v3.
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.security.fluent.models;
import com.azure.core.annotation.Fluent;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import com.azure.resourcemanager.security.models.EnvironmentDetails;
import com.azure.resourcemanager.security.models.HealthDataClassification;
import com.azure.resourcemanager.security.models.Issue;
import com.azure.resourcemanager.security.models.ResourceDetailsAutoGenerated;
import com.azure.resourcemanager.security.models.StatusAutoGenerated;
import java.io.IOException;
import java.util.List;
import java.util.Map;
/**
* Describes properties of the health report.
*/
@Fluent
public final class HealthReportProperties implements JsonSerializable {
/*
* The resource details of the health report
*/
private ResourceDetailsAutoGenerated resourceDetails;
/*
* The environment details of the resource
*/
private EnvironmentDetails environmentDetails;
/*
* The classification of the health report
*/
private HealthDataClassification healthDataClassification;
/*
* The status of the health report
*/
private StatusAutoGenerated status;
/*
* The affected defenders plans by unhealthy report
*/
private List affectedDefendersPlans;
/*
* The affected defenders sub plans by unhealthy report
*/
private List affectedDefendersSubPlans;
/*
* Additional data for the given health report, this field can include more details on the resource and the health
* scenario.
*/
private Map reportAdditionalData;
/*
* A collection of the issues in the report
*/
private List issues;
/**
* Creates an instance of HealthReportProperties class.
*/
public HealthReportProperties() {
}
/**
* Get the resourceDetails property: The resource details of the health report.
*
* @return the resourceDetails value.
*/
public ResourceDetailsAutoGenerated resourceDetails() {
return this.resourceDetails;
}
/**
* Set the resourceDetails property: The resource details of the health report.
*
* @param resourceDetails the resourceDetails value to set.
* @return the HealthReportProperties object itself.
*/
public HealthReportProperties withResourceDetails(ResourceDetailsAutoGenerated resourceDetails) {
this.resourceDetails = resourceDetails;
return this;
}
/**
* Get the environmentDetails property: The environment details of the resource.
*
* @return the environmentDetails value.
*/
public EnvironmentDetails environmentDetails() {
return this.environmentDetails;
}
/**
* Set the environmentDetails property: The environment details of the resource.
*
* @param environmentDetails the environmentDetails value to set.
* @return the HealthReportProperties object itself.
*/
public HealthReportProperties withEnvironmentDetails(EnvironmentDetails environmentDetails) {
this.environmentDetails = environmentDetails;
return this;
}
/**
* Get the healthDataClassification property: The classification of the health report.
*
* @return the healthDataClassification value.
*/
public HealthDataClassification healthDataClassification() {
return this.healthDataClassification;
}
/**
* Set the healthDataClassification property: The classification of the health report.
*
* @param healthDataClassification the healthDataClassification value to set.
* @return the HealthReportProperties object itself.
*/
public HealthReportProperties withHealthDataClassification(HealthDataClassification healthDataClassification) {
this.healthDataClassification = healthDataClassification;
return this;
}
/**
* Get the status property: The status of the health report.
*
* @return the status value.
*/
public StatusAutoGenerated status() {
return this.status;
}
/**
* Set the status property: The status of the health report.
*
* @param status the status value to set.
* @return the HealthReportProperties object itself.
*/
public HealthReportProperties withStatus(StatusAutoGenerated status) {
this.status = status;
return this;
}
/**
* Get the affectedDefendersPlans property: The affected defenders plans by unhealthy report.
*
* @return the affectedDefendersPlans value.
*/
public List affectedDefendersPlans() {
return this.affectedDefendersPlans;
}
/**
* Set the affectedDefendersPlans property: The affected defenders plans by unhealthy report.
*
* @param affectedDefendersPlans the affectedDefendersPlans value to set.
* @return the HealthReportProperties object itself.
*/
public HealthReportProperties withAffectedDefendersPlans(List affectedDefendersPlans) {
this.affectedDefendersPlans = affectedDefendersPlans;
return this;
}
/**
* Get the affectedDefendersSubPlans property: The affected defenders sub plans by unhealthy report.
*
* @return the affectedDefendersSubPlans value.
*/
public List affectedDefendersSubPlans() {
return this.affectedDefendersSubPlans;
}
/**
* Set the affectedDefendersSubPlans property: The affected defenders sub plans by unhealthy report.
*
* @param affectedDefendersSubPlans the affectedDefendersSubPlans value to set.
* @return the HealthReportProperties object itself.
*/
public HealthReportProperties withAffectedDefendersSubPlans(List affectedDefendersSubPlans) {
this.affectedDefendersSubPlans = affectedDefendersSubPlans;
return this;
}
/**
* Get the reportAdditionalData property: Additional data for the given health report, this field can include more
* details on the resource and the health scenario.
*
* @return the reportAdditionalData value.
*/
public Map reportAdditionalData() {
return this.reportAdditionalData;
}
/**
* Get the issues property: A collection of the issues in the report.
*
* @return the issues value.
*/
public List issues() {
return this.issues;
}
/**
* Set the issues property: A collection of the issues in the report.
*
* @param issues the issues value to set.
* @return the HealthReportProperties object itself.
*/
public HealthReportProperties withIssues(List issues) {
this.issues = issues;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (resourceDetails() != null) {
resourceDetails().validate();
}
if (environmentDetails() != null) {
environmentDetails().validate();
}
if (healthDataClassification() != null) {
healthDataClassification().validate();
}
if (status() != null) {
status().validate();
}
if (issues() != null) {
issues().forEach(e -> e.validate());
}
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeJsonField("resourceDetails", this.resourceDetails);
jsonWriter.writeJsonField("environmentDetails", this.environmentDetails);
jsonWriter.writeJsonField("healthDataClassification", this.healthDataClassification);
jsonWriter.writeJsonField("status", this.status);
jsonWriter.writeArrayField("affectedDefendersPlans", this.affectedDefendersPlans,
(writer, element) -> writer.writeString(element));
jsonWriter.writeArrayField("affectedDefendersSubPlans", this.affectedDefendersSubPlans,
(writer, element) -> writer.writeString(element));
jsonWriter.writeArrayField("issues", this.issues, (writer, element) -> writer.writeJson(element));
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of HealthReportProperties from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of HealthReportProperties if the JsonReader was pointing to an instance of it, or null if it
* was pointing to JSON null.
* @throws IOException If an error occurs while reading the HealthReportProperties.
*/
public static HealthReportProperties fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
HealthReportProperties deserializedHealthReportProperties = new HealthReportProperties();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("resourceDetails".equals(fieldName)) {
deserializedHealthReportProperties.resourceDetails = ResourceDetailsAutoGenerated.fromJson(reader);
} else if ("environmentDetails".equals(fieldName)) {
deserializedHealthReportProperties.environmentDetails = EnvironmentDetails.fromJson(reader);
} else if ("healthDataClassification".equals(fieldName)) {
deserializedHealthReportProperties.healthDataClassification
= HealthDataClassification.fromJson(reader);
} else if ("status".equals(fieldName)) {
deserializedHealthReportProperties.status = StatusAutoGenerated.fromJson(reader);
} else if ("affectedDefendersPlans".equals(fieldName)) {
List affectedDefendersPlans = reader.readArray(reader1 -> reader1.getString());
deserializedHealthReportProperties.affectedDefendersPlans = affectedDefendersPlans;
} else if ("affectedDefendersSubPlans".equals(fieldName)) {
List affectedDefendersSubPlans = reader.readArray(reader1 -> reader1.getString());
deserializedHealthReportProperties.affectedDefendersSubPlans = affectedDefendersSubPlans;
} else if ("reportAdditionalData".equals(fieldName)) {
Map reportAdditionalData = reader.readMap(reader1 -> reader1.getString());
deserializedHealthReportProperties.reportAdditionalData = reportAdditionalData;
} else if ("issues".equals(fieldName)) {
List issues = reader.readArray(reader1 -> Issue.fromJson(reader1));
deserializedHealthReportProperties.issues = issues;
} else {
reader.skipChildren();
}
}
return deserializedHealthReportProperties;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy