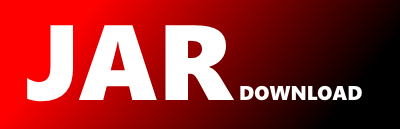
com.azure.resourcemanager.security.fluent.models.IoTSecurityAggregatedAlertProperties Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-security Show documentation
Show all versions of azure-resourcemanager-security Show documentation
This package contains Microsoft Azure SDK for Security Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. API spec for Microsoft.Security (Azure Security Center) resource provider. Package tag package-composite-v3.
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.security.fluent.models;
import com.azure.core.annotation.Immutable;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import com.azure.resourcemanager.security.models.IoTSecurityAggregatedAlertPropertiesTopDevicesListItem;
import com.azure.resourcemanager.security.models.ReportedSeverity;
import java.io.IOException;
import java.time.LocalDate;
import java.util.List;
/**
* IoT Security solution aggregated alert details.
*/
@Immutable
public final class IoTSecurityAggregatedAlertProperties
implements JsonSerializable {
/*
* Name of the alert type.
*/
private String alertType;
/*
* Display name of the alert type.
*/
private String alertDisplayName;
/*
* Date of detection.
*/
private LocalDate aggregatedDateUtc;
/*
* Name of the organization that raised the alert.
*/
private String vendorName;
/*
* Assessed alert severity.
*/
private ReportedSeverity reportedSeverity;
/*
* Recommended steps for remediation.
*/
private String remediationSteps;
/*
* Description of the suspected vulnerability and meaning.
*/
private String description;
/*
* Number of alerts occurrences within the aggregated time window.
*/
private Long count;
/*
* Azure resource ID of the resource that received the alerts.
*/
private String effectedResourceType;
/*
* The type of the alerted resource (Azure, Non-Azure).
*/
private String systemSource;
/*
* IoT Security solution alert response.
*/
private String actionTaken;
/*
* Log analytics query for getting the list of affected devices/alerts.
*/
private String logAnalyticsQuery;
/*
* 10 devices with the highest number of occurrences of this alert type, on this day.
*/
private List topDevicesList;
/**
* Creates an instance of IoTSecurityAggregatedAlertProperties class.
*/
public IoTSecurityAggregatedAlertProperties() {
}
/**
* Get the alertType property: Name of the alert type.
*
* @return the alertType value.
*/
public String alertType() {
return this.alertType;
}
/**
* Get the alertDisplayName property: Display name of the alert type.
*
* @return the alertDisplayName value.
*/
public String alertDisplayName() {
return this.alertDisplayName;
}
/**
* Get the aggregatedDateUtc property: Date of detection.
*
* @return the aggregatedDateUtc value.
*/
public LocalDate aggregatedDateUtc() {
return this.aggregatedDateUtc;
}
/**
* Get the vendorName property: Name of the organization that raised the alert.
*
* @return the vendorName value.
*/
public String vendorName() {
return this.vendorName;
}
/**
* Get the reportedSeverity property: Assessed alert severity.
*
* @return the reportedSeverity value.
*/
public ReportedSeverity reportedSeverity() {
return this.reportedSeverity;
}
/**
* Get the remediationSteps property: Recommended steps for remediation.
*
* @return the remediationSteps value.
*/
public String remediationSteps() {
return this.remediationSteps;
}
/**
* Get the description property: Description of the suspected vulnerability and meaning.
*
* @return the description value.
*/
public String description() {
return this.description;
}
/**
* Get the count property: Number of alerts occurrences within the aggregated time window.
*
* @return the count value.
*/
public Long count() {
return this.count;
}
/**
* Get the effectedResourceType property: Azure resource ID of the resource that received the alerts.
*
* @return the effectedResourceType value.
*/
public String effectedResourceType() {
return this.effectedResourceType;
}
/**
* Get the systemSource property: The type of the alerted resource (Azure, Non-Azure).
*
* @return the systemSource value.
*/
public String systemSource() {
return this.systemSource;
}
/**
* Get the actionTaken property: IoT Security solution alert response.
*
* @return the actionTaken value.
*/
public String actionTaken() {
return this.actionTaken;
}
/**
* Get the logAnalyticsQuery property: Log analytics query for getting the list of affected devices/alerts.
*
* @return the logAnalyticsQuery value.
*/
public String logAnalyticsQuery() {
return this.logAnalyticsQuery;
}
/**
* Get the topDevicesList property: 10 devices with the highest number of occurrences of this alert type, on this
* day.
*
* @return the topDevicesList value.
*/
public List topDevicesList() {
return this.topDevicesList;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (topDevicesList() != null) {
topDevicesList().forEach(e -> e.validate());
}
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of IoTSecurityAggregatedAlertProperties from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of IoTSecurityAggregatedAlertProperties if the JsonReader was pointing to an instance of it,
* or null if it was pointing to JSON null.
* @throws IOException If an error occurs while reading the IoTSecurityAggregatedAlertProperties.
*/
public static IoTSecurityAggregatedAlertProperties fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
IoTSecurityAggregatedAlertProperties deserializedIoTSecurityAggregatedAlertProperties
= new IoTSecurityAggregatedAlertProperties();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("alertType".equals(fieldName)) {
deserializedIoTSecurityAggregatedAlertProperties.alertType = reader.getString();
} else if ("alertDisplayName".equals(fieldName)) {
deserializedIoTSecurityAggregatedAlertProperties.alertDisplayName = reader.getString();
} else if ("aggregatedDateUtc".equals(fieldName)) {
deserializedIoTSecurityAggregatedAlertProperties.aggregatedDateUtc
= reader.getNullable(nonNullReader -> LocalDate.parse(nonNullReader.getString()));
} else if ("vendorName".equals(fieldName)) {
deserializedIoTSecurityAggregatedAlertProperties.vendorName = reader.getString();
} else if ("reportedSeverity".equals(fieldName)) {
deserializedIoTSecurityAggregatedAlertProperties.reportedSeverity
= ReportedSeverity.fromString(reader.getString());
} else if ("remediationSteps".equals(fieldName)) {
deserializedIoTSecurityAggregatedAlertProperties.remediationSteps = reader.getString();
} else if ("description".equals(fieldName)) {
deserializedIoTSecurityAggregatedAlertProperties.description = reader.getString();
} else if ("count".equals(fieldName)) {
deserializedIoTSecurityAggregatedAlertProperties.count = reader.getNullable(JsonReader::getLong);
} else if ("effectedResourceType".equals(fieldName)) {
deserializedIoTSecurityAggregatedAlertProperties.effectedResourceType = reader.getString();
} else if ("systemSource".equals(fieldName)) {
deserializedIoTSecurityAggregatedAlertProperties.systemSource = reader.getString();
} else if ("actionTaken".equals(fieldName)) {
deserializedIoTSecurityAggregatedAlertProperties.actionTaken = reader.getString();
} else if ("logAnalyticsQuery".equals(fieldName)) {
deserializedIoTSecurityAggregatedAlertProperties.logAnalyticsQuery = reader.getString();
} else if ("topDevicesList".equals(fieldName)) {
List topDevicesList = reader
.readArray(reader1 -> IoTSecurityAggregatedAlertPropertiesTopDevicesListItem.fromJson(reader1));
deserializedIoTSecurityAggregatedAlertProperties.topDevicesList = topDevicesList;
} else {
reader.skipChildren();
}
}
return deserializedIoTSecurityAggregatedAlertProperties;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy