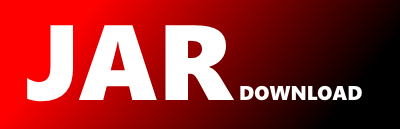
com.azure.resourcemanager.security.fluent.models.IoTSecurityAggregatedRecommendationProperties Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-security Show documentation
Show all versions of azure-resourcemanager-security Show documentation
This package contains Microsoft Azure SDK for Security Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. API spec for Microsoft.Security (Azure Security Center) resource provider. Package tag package-composite-v3.
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.security.fluent.models;
import com.azure.core.annotation.Fluent;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import com.azure.resourcemanager.security.models.ReportedSeverity;
import java.io.IOException;
/**
* IoT Security solution aggregated recommendation information.
*/
@Fluent
public final class IoTSecurityAggregatedRecommendationProperties
implements JsonSerializable {
/*
* Name of the recommendation.
*/
private String recommendationName;
/*
* Display name of the recommendation type.
*/
private String recommendationDisplayName;
/*
* Description of the suspected vulnerability and meaning.
*/
private String description;
/*
* Recommendation-type GUID.
*/
private String recommendationTypeId;
/*
* Name of the organization that made the recommendation.
*/
private String detectedBy;
/*
* Recommended steps for remediation
*/
private String remediationSteps;
/*
* Assessed recommendation severity.
*/
private ReportedSeverity reportedSeverity;
/*
* Number of healthy devices within the IoT Security solution.
*/
private Long healthyDevices;
/*
* Number of unhealthy devices within the IoT Security solution.
*/
private Long unhealthyDeviceCount;
/*
* Log analytics query for getting the list of affected devices/alerts.
*/
private String logAnalyticsQuery;
/**
* Creates an instance of IoTSecurityAggregatedRecommendationProperties class.
*/
public IoTSecurityAggregatedRecommendationProperties() {
}
/**
* Get the recommendationName property: Name of the recommendation.
*
* @return the recommendationName value.
*/
public String recommendationName() {
return this.recommendationName;
}
/**
* Set the recommendationName property: Name of the recommendation.
*
* @param recommendationName the recommendationName value to set.
* @return the IoTSecurityAggregatedRecommendationProperties object itself.
*/
public IoTSecurityAggregatedRecommendationProperties withRecommendationName(String recommendationName) {
this.recommendationName = recommendationName;
return this;
}
/**
* Get the recommendationDisplayName property: Display name of the recommendation type.
*
* @return the recommendationDisplayName value.
*/
public String recommendationDisplayName() {
return this.recommendationDisplayName;
}
/**
* Get the description property: Description of the suspected vulnerability and meaning.
*
* @return the description value.
*/
public String description() {
return this.description;
}
/**
* Get the recommendationTypeId property: Recommendation-type GUID.
*
* @return the recommendationTypeId value.
*/
public String recommendationTypeId() {
return this.recommendationTypeId;
}
/**
* Get the detectedBy property: Name of the organization that made the recommendation.
*
* @return the detectedBy value.
*/
public String detectedBy() {
return this.detectedBy;
}
/**
* Get the remediationSteps property: Recommended steps for remediation.
*
* @return the remediationSteps value.
*/
public String remediationSteps() {
return this.remediationSteps;
}
/**
* Get the reportedSeverity property: Assessed recommendation severity.
*
* @return the reportedSeverity value.
*/
public ReportedSeverity reportedSeverity() {
return this.reportedSeverity;
}
/**
* Get the healthyDevices property: Number of healthy devices within the IoT Security solution.
*
* @return the healthyDevices value.
*/
public Long healthyDevices() {
return this.healthyDevices;
}
/**
* Get the unhealthyDeviceCount property: Number of unhealthy devices within the IoT Security solution.
*
* @return the unhealthyDeviceCount value.
*/
public Long unhealthyDeviceCount() {
return this.unhealthyDeviceCount;
}
/**
* Get the logAnalyticsQuery property: Log analytics query for getting the list of affected devices/alerts.
*
* @return the logAnalyticsQuery value.
*/
public String logAnalyticsQuery() {
return this.logAnalyticsQuery;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("recommendationName", this.recommendationName);
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of IoTSecurityAggregatedRecommendationProperties from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of IoTSecurityAggregatedRecommendationProperties if the JsonReader was pointing to an
* instance of it, or null if it was pointing to JSON null.
* @throws IOException If an error occurs while reading the IoTSecurityAggregatedRecommendationProperties.
*/
public static IoTSecurityAggregatedRecommendationProperties fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
IoTSecurityAggregatedRecommendationProperties deserializedIoTSecurityAggregatedRecommendationProperties
= new IoTSecurityAggregatedRecommendationProperties();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("recommendationName".equals(fieldName)) {
deserializedIoTSecurityAggregatedRecommendationProperties.recommendationName = reader.getString();
} else if ("recommendationDisplayName".equals(fieldName)) {
deserializedIoTSecurityAggregatedRecommendationProperties.recommendationDisplayName
= reader.getString();
} else if ("description".equals(fieldName)) {
deserializedIoTSecurityAggregatedRecommendationProperties.description = reader.getString();
} else if ("recommendationTypeId".equals(fieldName)) {
deserializedIoTSecurityAggregatedRecommendationProperties.recommendationTypeId = reader.getString();
} else if ("detectedBy".equals(fieldName)) {
deserializedIoTSecurityAggregatedRecommendationProperties.detectedBy = reader.getString();
} else if ("remediationSteps".equals(fieldName)) {
deserializedIoTSecurityAggregatedRecommendationProperties.remediationSteps = reader.getString();
} else if ("reportedSeverity".equals(fieldName)) {
deserializedIoTSecurityAggregatedRecommendationProperties.reportedSeverity
= ReportedSeverity.fromString(reader.getString());
} else if ("healthyDevices".equals(fieldName)) {
deserializedIoTSecurityAggregatedRecommendationProperties.healthyDevices
= reader.getNullable(JsonReader::getLong);
} else if ("unhealthyDeviceCount".equals(fieldName)) {
deserializedIoTSecurityAggregatedRecommendationProperties.unhealthyDeviceCount
= reader.getNullable(JsonReader::getLong);
} else if ("logAnalyticsQuery".equals(fieldName)) {
deserializedIoTSecurityAggregatedRecommendationProperties.logAnalyticsQuery = reader.getString();
} else {
reader.skipChildren();
}
}
return deserializedIoTSecurityAggregatedRecommendationProperties;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy