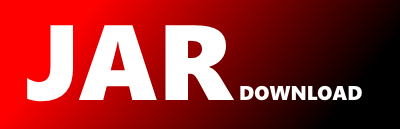
com.azure.resourcemanager.security.fluent.models.IoTSecuritySolutionAnalyticsModelProperties Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-security Show documentation
Show all versions of azure-resourcemanager-security Show documentation
This package contains Microsoft Azure SDK for Security Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. API spec for Microsoft.Security (Azure Security Center) resource provider. Package tag package-composite-v3.
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.security.fluent.models;
import com.azure.core.annotation.Fluent;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import com.azure.resourcemanager.security.models.IoTSecurityAlertedDevice;
import com.azure.resourcemanager.security.models.IoTSecurityDeviceAlert;
import com.azure.resourcemanager.security.models.IoTSecurityDeviceRecommendation;
import com.azure.resourcemanager.security.models.IoTSecuritySolutionAnalyticsModelPropertiesDevicesMetricsItem;
import com.azure.resourcemanager.security.models.IoTSeverityMetrics;
import java.io.IOException;
import java.util.List;
/**
* Security analytics properties of your IoT Security solution.
*/
@Fluent
public final class IoTSecuritySolutionAnalyticsModelProperties
implements JsonSerializable {
/*
* Security analytics of your IoT Security solution.
*/
private IoTSeverityMetrics metrics;
/*
* Number of unhealthy devices within your IoT Security solution.
*/
private Long unhealthyDeviceCount;
/*
* List of device metrics by the aggregation date.
*/
private List devicesMetrics;
/*
* List of the 3 devices with the most alerts.
*/
private List topAlertedDevices;
/*
* List of the 3 most prevalent device alerts.
*/
private List mostPrevalentDeviceAlerts;
/*
* List of the 3 most prevalent device recommendations.
*/
private List mostPrevalentDeviceRecommendations;
/**
* Creates an instance of IoTSecuritySolutionAnalyticsModelProperties class.
*/
public IoTSecuritySolutionAnalyticsModelProperties() {
}
/**
* Get the metrics property: Security analytics of your IoT Security solution.
*
* @return the metrics value.
*/
public IoTSeverityMetrics metrics() {
return this.metrics;
}
/**
* Get the unhealthyDeviceCount property: Number of unhealthy devices within your IoT Security solution.
*
* @return the unhealthyDeviceCount value.
*/
public Long unhealthyDeviceCount() {
return this.unhealthyDeviceCount;
}
/**
* Get the devicesMetrics property: List of device metrics by the aggregation date.
*
* @return the devicesMetrics value.
*/
public List devicesMetrics() {
return this.devicesMetrics;
}
/**
* Get the topAlertedDevices property: List of the 3 devices with the most alerts.
*
* @return the topAlertedDevices value.
*/
public List topAlertedDevices() {
return this.topAlertedDevices;
}
/**
* Set the topAlertedDevices property: List of the 3 devices with the most alerts.
*
* @param topAlertedDevices the topAlertedDevices value to set.
* @return the IoTSecuritySolutionAnalyticsModelProperties object itself.
*/
public IoTSecuritySolutionAnalyticsModelProperties
withTopAlertedDevices(List topAlertedDevices) {
this.topAlertedDevices = topAlertedDevices;
return this;
}
/**
* Get the mostPrevalentDeviceAlerts property: List of the 3 most prevalent device alerts.
*
* @return the mostPrevalentDeviceAlerts value.
*/
public List mostPrevalentDeviceAlerts() {
return this.mostPrevalentDeviceAlerts;
}
/**
* Set the mostPrevalentDeviceAlerts property: List of the 3 most prevalent device alerts.
*
* @param mostPrevalentDeviceAlerts the mostPrevalentDeviceAlerts value to set.
* @return the IoTSecuritySolutionAnalyticsModelProperties object itself.
*/
public IoTSecuritySolutionAnalyticsModelProperties
withMostPrevalentDeviceAlerts(List mostPrevalentDeviceAlerts) {
this.mostPrevalentDeviceAlerts = mostPrevalentDeviceAlerts;
return this;
}
/**
* Get the mostPrevalentDeviceRecommendations property: List of the 3 most prevalent device recommendations.
*
* @return the mostPrevalentDeviceRecommendations value.
*/
public List mostPrevalentDeviceRecommendations() {
return this.mostPrevalentDeviceRecommendations;
}
/**
* Set the mostPrevalentDeviceRecommendations property: List of the 3 most prevalent device recommendations.
*
* @param mostPrevalentDeviceRecommendations the mostPrevalentDeviceRecommendations value to set.
* @return the IoTSecuritySolutionAnalyticsModelProperties object itself.
*/
public IoTSecuritySolutionAnalyticsModelProperties withMostPrevalentDeviceRecommendations(
List mostPrevalentDeviceRecommendations) {
this.mostPrevalentDeviceRecommendations = mostPrevalentDeviceRecommendations;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (metrics() != null) {
metrics().validate();
}
if (devicesMetrics() != null) {
devicesMetrics().forEach(e -> e.validate());
}
if (topAlertedDevices() != null) {
topAlertedDevices().forEach(e -> e.validate());
}
if (mostPrevalentDeviceAlerts() != null) {
mostPrevalentDeviceAlerts().forEach(e -> e.validate());
}
if (mostPrevalentDeviceRecommendations() != null) {
mostPrevalentDeviceRecommendations().forEach(e -> e.validate());
}
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeArrayField("topAlertedDevices", this.topAlertedDevices,
(writer, element) -> writer.writeJson(element));
jsonWriter.writeArrayField("mostPrevalentDeviceAlerts", this.mostPrevalentDeviceAlerts,
(writer, element) -> writer.writeJson(element));
jsonWriter.writeArrayField("mostPrevalentDeviceRecommendations", this.mostPrevalentDeviceRecommendations,
(writer, element) -> writer.writeJson(element));
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of IoTSecuritySolutionAnalyticsModelProperties from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of IoTSecuritySolutionAnalyticsModelProperties if the JsonReader was pointing to an instance
* of it, or null if it was pointing to JSON null.
* @throws IOException If an error occurs while reading the IoTSecuritySolutionAnalyticsModelProperties.
*/
public static IoTSecuritySolutionAnalyticsModelProperties fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
IoTSecuritySolutionAnalyticsModelProperties deserializedIoTSecuritySolutionAnalyticsModelProperties
= new IoTSecuritySolutionAnalyticsModelProperties();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("metrics".equals(fieldName)) {
deserializedIoTSecuritySolutionAnalyticsModelProperties.metrics
= IoTSeverityMetrics.fromJson(reader);
} else if ("unhealthyDeviceCount".equals(fieldName)) {
deserializedIoTSecuritySolutionAnalyticsModelProperties.unhealthyDeviceCount
= reader.getNullable(JsonReader::getLong);
} else if ("devicesMetrics".equals(fieldName)) {
List devicesMetrics
= reader.readArray(
reader1 -> IoTSecuritySolutionAnalyticsModelPropertiesDevicesMetricsItem.fromJson(reader1));
deserializedIoTSecuritySolutionAnalyticsModelProperties.devicesMetrics = devicesMetrics;
} else if ("topAlertedDevices".equals(fieldName)) {
List topAlertedDevices
= reader.readArray(reader1 -> IoTSecurityAlertedDevice.fromJson(reader1));
deserializedIoTSecuritySolutionAnalyticsModelProperties.topAlertedDevices = topAlertedDevices;
} else if ("mostPrevalentDeviceAlerts".equals(fieldName)) {
List mostPrevalentDeviceAlerts
= reader.readArray(reader1 -> IoTSecurityDeviceAlert.fromJson(reader1));
deserializedIoTSecuritySolutionAnalyticsModelProperties.mostPrevalentDeviceAlerts
= mostPrevalentDeviceAlerts;
} else if ("mostPrevalentDeviceRecommendations".equals(fieldName)) {
List mostPrevalentDeviceRecommendations
= reader.readArray(reader1 -> IoTSecurityDeviceRecommendation.fromJson(reader1));
deserializedIoTSecuritySolutionAnalyticsModelProperties.mostPrevalentDeviceRecommendations
= mostPrevalentDeviceRecommendations;
} else {
reader.skipChildren();
}
}
return deserializedIoTSecuritySolutionAnalyticsModelProperties;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy