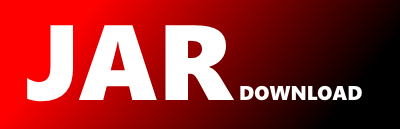
com.azure.resourcemanager.security.fluent.models.RegulatoryComplianceAssessmentProperties Maven / Gradle / Ivy
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.security.fluent.models;
import com.azure.core.annotation.Fluent;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import com.azure.resourcemanager.security.models.State;
import java.io.IOException;
/**
* Regulatory compliance assessment data.
*/
@Fluent
public final class RegulatoryComplianceAssessmentProperties
implements JsonSerializable {
/*
* The description of the regulatory compliance assessment
*/
private String description;
/*
* The expected type of assessment contained in the AssessmentDetailsLink
*/
private String assessmentType;
/*
* Link to more detailed assessment results data. The response type will be according to the assessmentType field
*/
private String assessmentDetailsLink;
/*
* Aggregative state based on the assessment's scanned resources states
*/
private State state;
/*
* The given assessment's related resources count with passed state.
*/
private Integer passedResources;
/*
* The given assessment's related resources count with failed state.
*/
private Integer failedResources;
/*
* The given assessment's related resources count with skipped state.
*/
private Integer skippedResources;
/*
* The given assessment's related resources count with unsupported state.
*/
private Integer unsupportedResources;
/**
* Creates an instance of RegulatoryComplianceAssessmentProperties class.
*/
public RegulatoryComplianceAssessmentProperties() {
}
/**
* Get the description property: The description of the regulatory compliance assessment.
*
* @return the description value.
*/
public String description() {
return this.description;
}
/**
* Get the assessmentType property: The expected type of assessment contained in the AssessmentDetailsLink.
*
* @return the assessmentType value.
*/
public String assessmentType() {
return this.assessmentType;
}
/**
* Get the assessmentDetailsLink property: Link to more detailed assessment results data. The response type will be
* according to the assessmentType field.
*
* @return the assessmentDetailsLink value.
*/
public String assessmentDetailsLink() {
return this.assessmentDetailsLink;
}
/**
* Get the state property: Aggregative state based on the assessment's scanned resources states.
*
* @return the state value.
*/
public State state() {
return this.state;
}
/**
* Set the state property: Aggregative state based on the assessment's scanned resources states.
*
* @param state the state value to set.
* @return the RegulatoryComplianceAssessmentProperties object itself.
*/
public RegulatoryComplianceAssessmentProperties withState(State state) {
this.state = state;
return this;
}
/**
* Get the passedResources property: The given assessment's related resources count with passed state.
*
* @return the passedResources value.
*/
public Integer passedResources() {
return this.passedResources;
}
/**
* Get the failedResources property: The given assessment's related resources count with failed state.
*
* @return the failedResources value.
*/
public Integer failedResources() {
return this.failedResources;
}
/**
* Get the skippedResources property: The given assessment's related resources count with skipped state.
*
* @return the skippedResources value.
*/
public Integer skippedResources() {
return this.skippedResources;
}
/**
* Get the unsupportedResources property: The given assessment's related resources count with unsupported state.
*
* @return the unsupportedResources value.
*/
public Integer unsupportedResources() {
return this.unsupportedResources;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("state", this.state == null ? null : this.state.toString());
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of RegulatoryComplianceAssessmentProperties from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of RegulatoryComplianceAssessmentProperties if the JsonReader was pointing to an instance of
* it, or null if it was pointing to JSON null.
* @throws IOException If an error occurs while reading the RegulatoryComplianceAssessmentProperties.
*/
public static RegulatoryComplianceAssessmentProperties fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
RegulatoryComplianceAssessmentProperties deserializedRegulatoryComplianceAssessmentProperties
= new RegulatoryComplianceAssessmentProperties();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("description".equals(fieldName)) {
deserializedRegulatoryComplianceAssessmentProperties.description = reader.getString();
} else if ("assessmentType".equals(fieldName)) {
deserializedRegulatoryComplianceAssessmentProperties.assessmentType = reader.getString();
} else if ("assessmentDetailsLink".equals(fieldName)) {
deserializedRegulatoryComplianceAssessmentProperties.assessmentDetailsLink = reader.getString();
} else if ("state".equals(fieldName)) {
deserializedRegulatoryComplianceAssessmentProperties.state = State.fromString(reader.getString());
} else if ("passedResources".equals(fieldName)) {
deserializedRegulatoryComplianceAssessmentProperties.passedResources
= reader.getNullable(JsonReader::getInt);
} else if ("failedResources".equals(fieldName)) {
deserializedRegulatoryComplianceAssessmentProperties.failedResources
= reader.getNullable(JsonReader::getInt);
} else if ("skippedResources".equals(fieldName)) {
deserializedRegulatoryComplianceAssessmentProperties.skippedResources
= reader.getNullable(JsonReader::getInt);
} else if ("unsupportedResources".equals(fieldName)) {
deserializedRegulatoryComplianceAssessmentProperties.unsupportedResources
= reader.getNullable(JsonReader::getInt);
} else {
reader.skipChildren();
}
}
return deserializedRegulatoryComplianceAssessmentProperties;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy