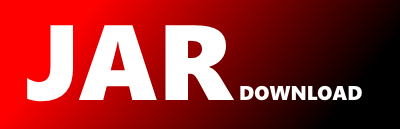
com.azure.resourcemanager.security.fluent.models.SecurityAssessmentMetadataProperties Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-security Show documentation
Show all versions of azure-resourcemanager-security Show documentation
This package contains Microsoft Azure SDK for Security Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. API spec for Microsoft.Security (Azure Security Center) resource provider. Package tag package-composite-v3.
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.security.fluent.models;
import com.azure.core.annotation.Fluent;
import com.azure.core.util.logging.ClientLogger;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import com.azure.resourcemanager.security.models.AssessmentType;
import com.azure.resourcemanager.security.models.Categories;
import com.azure.resourcemanager.security.models.ImplementationEffort;
import com.azure.resourcemanager.security.models.SecurityAssessmentMetadataPartnerData;
import com.azure.resourcemanager.security.models.Severity;
import com.azure.resourcemanager.security.models.Threats;
import com.azure.resourcemanager.security.models.UserImpact;
import java.io.IOException;
import java.util.List;
/**
* Describes properties of an assessment metadata.
*/
@Fluent
public class SecurityAssessmentMetadataProperties implements JsonSerializable {
/*
* User friendly display name of the assessment
*/
private String displayName;
/*
* Azure resource ID of the policy definition that turns this assessment calculation on
*/
private String policyDefinitionId;
/*
* Human readable description of the assessment
*/
private String description;
/*
* Human readable description of what you should do to mitigate this security issue
*/
private String remediationDescription;
/*
* The categories property.
*/
private List categories;
/*
* The severity level of the assessment
*/
private Severity severity;
/*
* The user impact of the assessment
*/
private UserImpact userImpact;
/*
* The implementation effort required to remediate this assessment
*/
private ImplementationEffort implementationEffort;
/*
* The threats property.
*/
private List threats;
/*
* True if this assessment is in preview release status
*/
private Boolean preview;
/*
* BuiltIn if the assessment based on built-in Azure Policy definition, Custom if the assessment based on custom
* Azure Policy definition
*/
private AssessmentType assessmentType;
/*
* Describes the partner that created the assessment
*/
private SecurityAssessmentMetadataPartnerData partnerData;
/**
* Creates an instance of SecurityAssessmentMetadataProperties class.
*/
public SecurityAssessmentMetadataProperties() {
}
/**
* Get the displayName property: User friendly display name of the assessment.
*
* @return the displayName value.
*/
public String displayName() {
return this.displayName;
}
/**
* Set the displayName property: User friendly display name of the assessment.
*
* @param displayName the displayName value to set.
* @return the SecurityAssessmentMetadataProperties object itself.
*/
public SecurityAssessmentMetadataProperties withDisplayName(String displayName) {
this.displayName = displayName;
return this;
}
/**
* Get the policyDefinitionId property: Azure resource ID of the policy definition that turns this assessment
* calculation on.
*
* @return the policyDefinitionId value.
*/
public String policyDefinitionId() {
return this.policyDefinitionId;
}
/**
* Set the policyDefinitionId property: Azure resource ID of the policy definition that turns this assessment
* calculation on.
*
* @param policyDefinitionId the policyDefinitionId value to set.
* @return the SecurityAssessmentMetadataProperties object itself.
*/
SecurityAssessmentMetadataProperties withPolicyDefinitionId(String policyDefinitionId) {
this.policyDefinitionId = policyDefinitionId;
return this;
}
/**
* Get the description property: Human readable description of the assessment.
*
* @return the description value.
*/
public String description() {
return this.description;
}
/**
* Set the description property: Human readable description of the assessment.
*
* @param description the description value to set.
* @return the SecurityAssessmentMetadataProperties object itself.
*/
public SecurityAssessmentMetadataProperties withDescription(String description) {
this.description = description;
return this;
}
/**
* Get the remediationDescription property: Human readable description of what you should do to mitigate this
* security issue.
*
* @return the remediationDescription value.
*/
public String remediationDescription() {
return this.remediationDescription;
}
/**
* Set the remediationDescription property: Human readable description of what you should do to mitigate this
* security issue.
*
* @param remediationDescription the remediationDescription value to set.
* @return the SecurityAssessmentMetadataProperties object itself.
*/
public SecurityAssessmentMetadataProperties withRemediationDescription(String remediationDescription) {
this.remediationDescription = remediationDescription;
return this;
}
/**
* Get the categories property: The categories property.
*
* @return the categories value.
*/
public List categories() {
return this.categories;
}
/**
* Set the categories property: The categories property.
*
* @param categories the categories value to set.
* @return the SecurityAssessmentMetadataProperties object itself.
*/
public SecurityAssessmentMetadataProperties withCategories(List categories) {
this.categories = categories;
return this;
}
/**
* Get the severity property: The severity level of the assessment.
*
* @return the severity value.
*/
public Severity severity() {
return this.severity;
}
/**
* Set the severity property: The severity level of the assessment.
*
* @param severity the severity value to set.
* @return the SecurityAssessmentMetadataProperties object itself.
*/
public SecurityAssessmentMetadataProperties withSeverity(Severity severity) {
this.severity = severity;
return this;
}
/**
* Get the userImpact property: The user impact of the assessment.
*
* @return the userImpact value.
*/
public UserImpact userImpact() {
return this.userImpact;
}
/**
* Set the userImpact property: The user impact of the assessment.
*
* @param userImpact the userImpact value to set.
* @return the SecurityAssessmentMetadataProperties object itself.
*/
public SecurityAssessmentMetadataProperties withUserImpact(UserImpact userImpact) {
this.userImpact = userImpact;
return this;
}
/**
* Get the implementationEffort property: The implementation effort required to remediate this assessment.
*
* @return the implementationEffort value.
*/
public ImplementationEffort implementationEffort() {
return this.implementationEffort;
}
/**
* Set the implementationEffort property: The implementation effort required to remediate this assessment.
*
* @param implementationEffort the implementationEffort value to set.
* @return the SecurityAssessmentMetadataProperties object itself.
*/
public SecurityAssessmentMetadataProperties withImplementationEffort(ImplementationEffort implementationEffort) {
this.implementationEffort = implementationEffort;
return this;
}
/**
* Get the threats property: The threats property.
*
* @return the threats value.
*/
public List threats() {
return this.threats;
}
/**
* Set the threats property: The threats property.
*
* @param threats the threats value to set.
* @return the SecurityAssessmentMetadataProperties object itself.
*/
public SecurityAssessmentMetadataProperties withThreats(List threats) {
this.threats = threats;
return this;
}
/**
* Get the preview property: True if this assessment is in preview release status.
*
* @return the preview value.
*/
public Boolean preview() {
return this.preview;
}
/**
* Set the preview property: True if this assessment is in preview release status.
*
* @param preview the preview value to set.
* @return the SecurityAssessmentMetadataProperties object itself.
*/
public SecurityAssessmentMetadataProperties withPreview(Boolean preview) {
this.preview = preview;
return this;
}
/**
* Get the assessmentType property: BuiltIn if the assessment based on built-in Azure Policy definition, Custom if
* the assessment based on custom Azure Policy definition.
*
* @return the assessmentType value.
*/
public AssessmentType assessmentType() {
return this.assessmentType;
}
/**
* Set the assessmentType property: BuiltIn if the assessment based on built-in Azure Policy definition, Custom if
* the assessment based on custom Azure Policy definition.
*
* @param assessmentType the assessmentType value to set.
* @return the SecurityAssessmentMetadataProperties object itself.
*/
public SecurityAssessmentMetadataProperties withAssessmentType(AssessmentType assessmentType) {
this.assessmentType = assessmentType;
return this;
}
/**
* Get the partnerData property: Describes the partner that created the assessment.
*
* @return the partnerData value.
*/
public SecurityAssessmentMetadataPartnerData partnerData() {
return this.partnerData;
}
/**
* Set the partnerData property: Describes the partner that created the assessment.
*
* @param partnerData the partnerData value to set.
* @return the SecurityAssessmentMetadataProperties object itself.
*/
public SecurityAssessmentMetadataProperties withPartnerData(SecurityAssessmentMetadataPartnerData partnerData) {
this.partnerData = partnerData;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (displayName() == null) {
throw LOGGER.atError()
.log(new IllegalArgumentException(
"Missing required property displayName in model SecurityAssessmentMetadataProperties"));
}
if (severity() == null) {
throw LOGGER.atError()
.log(new IllegalArgumentException(
"Missing required property severity in model SecurityAssessmentMetadataProperties"));
}
if (assessmentType() == null) {
throw LOGGER.atError()
.log(new IllegalArgumentException(
"Missing required property assessmentType in model SecurityAssessmentMetadataProperties"));
}
if (partnerData() != null) {
partnerData().validate();
}
}
private static final ClientLogger LOGGER = new ClientLogger(SecurityAssessmentMetadataProperties.class);
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("displayName", this.displayName);
jsonWriter.writeStringField("severity", this.severity == null ? null : this.severity.toString());
jsonWriter.writeStringField("assessmentType",
this.assessmentType == null ? null : this.assessmentType.toString());
jsonWriter.writeStringField("description", this.description);
jsonWriter.writeStringField("remediationDescription", this.remediationDescription);
jsonWriter.writeArrayField("categories", this.categories,
(writer, element) -> writer.writeString(element == null ? null : element.toString()));
jsonWriter.writeStringField("userImpact", this.userImpact == null ? null : this.userImpact.toString());
jsonWriter.writeStringField("implementationEffort",
this.implementationEffort == null ? null : this.implementationEffort.toString());
jsonWriter.writeArrayField("threats", this.threats,
(writer, element) -> writer.writeString(element == null ? null : element.toString()));
jsonWriter.writeBooleanField("preview", this.preview);
jsonWriter.writeJsonField("partnerData", this.partnerData);
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of SecurityAssessmentMetadataProperties from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of SecurityAssessmentMetadataProperties if the JsonReader was pointing to an instance of it,
* or null if it was pointing to JSON null.
* @throws IllegalStateException If the deserialized JSON object was missing any required properties.
* @throws IOException If an error occurs while reading the SecurityAssessmentMetadataProperties.
*/
public static SecurityAssessmentMetadataProperties fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
SecurityAssessmentMetadataProperties deserializedSecurityAssessmentMetadataProperties
= new SecurityAssessmentMetadataProperties();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("displayName".equals(fieldName)) {
deserializedSecurityAssessmentMetadataProperties.displayName = reader.getString();
} else if ("severity".equals(fieldName)) {
deserializedSecurityAssessmentMetadataProperties.severity = Severity.fromString(reader.getString());
} else if ("assessmentType".equals(fieldName)) {
deserializedSecurityAssessmentMetadataProperties.assessmentType
= AssessmentType.fromString(reader.getString());
} else if ("policyDefinitionId".equals(fieldName)) {
deserializedSecurityAssessmentMetadataProperties.policyDefinitionId = reader.getString();
} else if ("description".equals(fieldName)) {
deserializedSecurityAssessmentMetadataProperties.description = reader.getString();
} else if ("remediationDescription".equals(fieldName)) {
deserializedSecurityAssessmentMetadataProperties.remediationDescription = reader.getString();
} else if ("categories".equals(fieldName)) {
List categories
= reader.readArray(reader1 -> Categories.fromString(reader1.getString()));
deserializedSecurityAssessmentMetadataProperties.categories = categories;
} else if ("userImpact".equals(fieldName)) {
deserializedSecurityAssessmentMetadataProperties.userImpact
= UserImpact.fromString(reader.getString());
} else if ("implementationEffort".equals(fieldName)) {
deserializedSecurityAssessmentMetadataProperties.implementationEffort
= ImplementationEffort.fromString(reader.getString());
} else if ("threats".equals(fieldName)) {
List threats = reader.readArray(reader1 -> Threats.fromString(reader1.getString()));
deserializedSecurityAssessmentMetadataProperties.threats = threats;
} else if ("preview".equals(fieldName)) {
deserializedSecurityAssessmentMetadataProperties.preview
= reader.getNullable(JsonReader::getBoolean);
} else if ("partnerData".equals(fieldName)) {
deserializedSecurityAssessmentMetadataProperties.partnerData
= SecurityAssessmentMetadataPartnerData.fromJson(reader);
} else {
reader.skipChildren();
}
}
return deserializedSecurityAssessmentMetadataProperties;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy