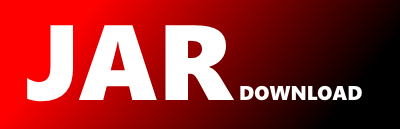
com.azure.resourcemanager.security.fluent.models.SecurityAssessmentMetadataPropertiesResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-security Show documentation
Show all versions of azure-resourcemanager-security Show documentation
This package contains Microsoft Azure SDK for Security Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. API spec for Microsoft.Security (Azure Security Center) resource provider. Package tag package-composite-v3.
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.security.fluent.models;
import com.azure.core.annotation.Fluent;
import com.azure.core.util.logging.ClientLogger;
import com.azure.json.JsonReader;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import com.azure.resourcemanager.security.models.AssessmentType;
import com.azure.resourcemanager.security.models.Categories;
import com.azure.resourcemanager.security.models.ImplementationEffort;
import com.azure.resourcemanager.security.models.SecurityAssessmentMetadataPartnerData;
import com.azure.resourcemanager.security.models.SecurityAssessmentMetadataPropertiesResponsePublishDates;
import com.azure.resourcemanager.security.models.Severity;
import com.azure.resourcemanager.security.models.Tactics;
import com.azure.resourcemanager.security.models.Techniques;
import com.azure.resourcemanager.security.models.Threats;
import com.azure.resourcemanager.security.models.UserImpact;
import java.io.IOException;
import java.util.List;
/**
* Describes properties of an assessment metadata response.
*/
@Fluent
public final class SecurityAssessmentMetadataPropertiesResponse extends SecurityAssessmentMetadataProperties {
/*
* The publishDates property.
*/
private SecurityAssessmentMetadataPropertiesResponsePublishDates publishDates;
/*
* The plannedDeprecationDate property.
*/
private String plannedDeprecationDate;
/*
* The tactics property.
*/
private List tactics;
/*
* The techniques property.
*/
private List techniques;
/*
* Azure resource ID of the policy definition that turns this assessment calculation on
*/
private String policyDefinitionId;
/**
* Creates an instance of SecurityAssessmentMetadataPropertiesResponse class.
*/
public SecurityAssessmentMetadataPropertiesResponse() {
}
/**
* Get the publishDates property: The publishDates property.
*
* @return the publishDates value.
*/
public SecurityAssessmentMetadataPropertiesResponsePublishDates publishDates() {
return this.publishDates;
}
/**
* Set the publishDates property: The publishDates property.
*
* @param publishDates the publishDates value to set.
* @return the SecurityAssessmentMetadataPropertiesResponse object itself.
*/
public SecurityAssessmentMetadataPropertiesResponse
withPublishDates(SecurityAssessmentMetadataPropertiesResponsePublishDates publishDates) {
this.publishDates = publishDates;
return this;
}
/**
* Get the plannedDeprecationDate property: The plannedDeprecationDate property.
*
* @return the plannedDeprecationDate value.
*/
public String plannedDeprecationDate() {
return this.plannedDeprecationDate;
}
/**
* Set the plannedDeprecationDate property: The plannedDeprecationDate property.
*
* @param plannedDeprecationDate the plannedDeprecationDate value to set.
* @return the SecurityAssessmentMetadataPropertiesResponse object itself.
*/
public SecurityAssessmentMetadataPropertiesResponse withPlannedDeprecationDate(String plannedDeprecationDate) {
this.plannedDeprecationDate = plannedDeprecationDate;
return this;
}
/**
* Get the tactics property: The tactics property.
*
* @return the tactics value.
*/
public List tactics() {
return this.tactics;
}
/**
* Set the tactics property: The tactics property.
*
* @param tactics the tactics value to set.
* @return the SecurityAssessmentMetadataPropertiesResponse object itself.
*/
public SecurityAssessmentMetadataPropertiesResponse withTactics(List tactics) {
this.tactics = tactics;
return this;
}
/**
* Get the techniques property: The techniques property.
*
* @return the techniques value.
*/
public List techniques() {
return this.techniques;
}
/**
* Set the techniques property: The techniques property.
*
* @param techniques the techniques value to set.
* @return the SecurityAssessmentMetadataPropertiesResponse object itself.
*/
public SecurityAssessmentMetadataPropertiesResponse withTechniques(List techniques) {
this.techniques = techniques;
return this;
}
/**
* Get the policyDefinitionId property: Azure resource ID of the policy definition that turns this assessment
* calculation on.
*
* @return the policyDefinitionId value.
*/
@Override
public String policyDefinitionId() {
return this.policyDefinitionId;
}
/**
* {@inheritDoc}
*/
@Override
public SecurityAssessmentMetadataPropertiesResponse withDisplayName(String displayName) {
super.withDisplayName(displayName);
return this;
}
/**
* {@inheritDoc}
*/
@Override
public SecurityAssessmentMetadataPropertiesResponse withDescription(String description) {
super.withDescription(description);
return this;
}
/**
* {@inheritDoc}
*/
@Override
public SecurityAssessmentMetadataPropertiesResponse withRemediationDescription(String remediationDescription) {
super.withRemediationDescription(remediationDescription);
return this;
}
/**
* {@inheritDoc}
*/
@Override
public SecurityAssessmentMetadataPropertiesResponse withCategories(List categories) {
super.withCategories(categories);
return this;
}
/**
* {@inheritDoc}
*/
@Override
public SecurityAssessmentMetadataPropertiesResponse withSeverity(Severity severity) {
super.withSeverity(severity);
return this;
}
/**
* {@inheritDoc}
*/
@Override
public SecurityAssessmentMetadataPropertiesResponse withUserImpact(UserImpact userImpact) {
super.withUserImpact(userImpact);
return this;
}
/**
* {@inheritDoc}
*/
@Override
public SecurityAssessmentMetadataPropertiesResponse
withImplementationEffort(ImplementationEffort implementationEffort) {
super.withImplementationEffort(implementationEffort);
return this;
}
/**
* {@inheritDoc}
*/
@Override
public SecurityAssessmentMetadataPropertiesResponse withThreats(List threats) {
super.withThreats(threats);
return this;
}
/**
* {@inheritDoc}
*/
@Override
public SecurityAssessmentMetadataPropertiesResponse withPreview(Boolean preview) {
super.withPreview(preview);
return this;
}
/**
* {@inheritDoc}
*/
@Override
public SecurityAssessmentMetadataPropertiesResponse withAssessmentType(AssessmentType assessmentType) {
super.withAssessmentType(assessmentType);
return this;
}
/**
* {@inheritDoc}
*/
@Override
public SecurityAssessmentMetadataPropertiesResponse
withPartnerData(SecurityAssessmentMetadataPartnerData partnerData) {
super.withPartnerData(partnerData);
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
@Override
public void validate() {
if (publishDates() != null) {
publishDates().validate();
}
if (displayName() == null) {
throw LOGGER.atError()
.log(new IllegalArgumentException(
"Missing required property displayName in model SecurityAssessmentMetadataPropertiesResponse"));
}
if (severity() == null) {
throw LOGGER.atError()
.log(new IllegalArgumentException(
"Missing required property severity in model SecurityAssessmentMetadataPropertiesResponse"));
}
if (assessmentType() == null) {
throw LOGGER.atError()
.log(new IllegalArgumentException(
"Missing required property assessmentType in model SecurityAssessmentMetadataPropertiesResponse"));
}
if (partnerData() != null) {
partnerData().validate();
}
}
private static final ClientLogger LOGGER = new ClientLogger(SecurityAssessmentMetadataPropertiesResponse.class);
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("displayName", displayName());
jsonWriter.writeStringField("severity", severity() == null ? null : severity().toString());
jsonWriter.writeStringField("assessmentType", assessmentType() == null ? null : assessmentType().toString());
jsonWriter.writeStringField("description", description());
jsonWriter.writeStringField("remediationDescription", remediationDescription());
jsonWriter.writeArrayField("categories", categories(),
(writer, element) -> writer.writeString(element == null ? null : element.toString()));
jsonWriter.writeStringField("userImpact", userImpact() == null ? null : userImpact().toString());
jsonWriter.writeStringField("implementationEffort",
implementationEffort() == null ? null : implementationEffort().toString());
jsonWriter.writeArrayField("threats", threats(),
(writer, element) -> writer.writeString(element == null ? null : element.toString()));
jsonWriter.writeBooleanField("preview", preview());
jsonWriter.writeJsonField("partnerData", partnerData());
jsonWriter.writeJsonField("publishDates", this.publishDates);
jsonWriter.writeStringField("plannedDeprecationDate", this.plannedDeprecationDate);
jsonWriter.writeArrayField("tactics", this.tactics,
(writer, element) -> writer.writeString(element == null ? null : element.toString()));
jsonWriter.writeArrayField("techniques", this.techniques,
(writer, element) -> writer.writeString(element == null ? null : element.toString()));
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of SecurityAssessmentMetadataPropertiesResponse from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of SecurityAssessmentMetadataPropertiesResponse if the JsonReader was pointing to an instance
* of it, or null if it was pointing to JSON null.
* @throws IllegalStateException If the deserialized JSON object was missing any required properties.
* @throws IOException If an error occurs while reading the SecurityAssessmentMetadataPropertiesResponse.
*/
public static SecurityAssessmentMetadataPropertiesResponse fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
SecurityAssessmentMetadataPropertiesResponse deserializedSecurityAssessmentMetadataPropertiesResponse
= new SecurityAssessmentMetadataPropertiesResponse();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("displayName".equals(fieldName)) {
deserializedSecurityAssessmentMetadataPropertiesResponse.withDisplayName(reader.getString());
} else if ("severity".equals(fieldName)) {
deserializedSecurityAssessmentMetadataPropertiesResponse
.withSeverity(Severity.fromString(reader.getString()));
} else if ("assessmentType".equals(fieldName)) {
deserializedSecurityAssessmentMetadataPropertiesResponse
.withAssessmentType(AssessmentType.fromString(reader.getString()));
} else if ("policyDefinitionId".equals(fieldName)) {
deserializedSecurityAssessmentMetadataPropertiesResponse.policyDefinitionId = reader.getString();
} else if ("description".equals(fieldName)) {
deserializedSecurityAssessmentMetadataPropertiesResponse.withDescription(reader.getString());
} else if ("remediationDescription".equals(fieldName)) {
deserializedSecurityAssessmentMetadataPropertiesResponse
.withRemediationDescription(reader.getString());
} else if ("categories".equals(fieldName)) {
List categories
= reader.readArray(reader1 -> Categories.fromString(reader1.getString()));
deserializedSecurityAssessmentMetadataPropertiesResponse.withCategories(categories);
} else if ("userImpact".equals(fieldName)) {
deserializedSecurityAssessmentMetadataPropertiesResponse
.withUserImpact(UserImpact.fromString(reader.getString()));
} else if ("implementationEffort".equals(fieldName)) {
deserializedSecurityAssessmentMetadataPropertiesResponse
.withImplementationEffort(ImplementationEffort.fromString(reader.getString()));
} else if ("threats".equals(fieldName)) {
List threats = reader.readArray(reader1 -> Threats.fromString(reader1.getString()));
deserializedSecurityAssessmentMetadataPropertiesResponse.withThreats(threats);
} else if ("preview".equals(fieldName)) {
deserializedSecurityAssessmentMetadataPropertiesResponse
.withPreview(reader.getNullable(JsonReader::getBoolean));
} else if ("partnerData".equals(fieldName)) {
deserializedSecurityAssessmentMetadataPropertiesResponse
.withPartnerData(SecurityAssessmentMetadataPartnerData.fromJson(reader));
} else if ("publishDates".equals(fieldName)) {
deserializedSecurityAssessmentMetadataPropertiesResponse.publishDates
= SecurityAssessmentMetadataPropertiesResponsePublishDates.fromJson(reader);
} else if ("plannedDeprecationDate".equals(fieldName)) {
deserializedSecurityAssessmentMetadataPropertiesResponse.plannedDeprecationDate
= reader.getString();
} else if ("tactics".equals(fieldName)) {
List tactics = reader.readArray(reader1 -> Tactics.fromString(reader1.getString()));
deserializedSecurityAssessmentMetadataPropertiesResponse.tactics = tactics;
} else if ("techniques".equals(fieldName)) {
List techniques
= reader.readArray(reader1 -> Techniques.fromString(reader1.getString()));
deserializedSecurityAssessmentMetadataPropertiesResponse.techniques = techniques;
} else {
reader.skipChildren();
}
}
return deserializedSecurityAssessmentMetadataPropertiesResponse;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy