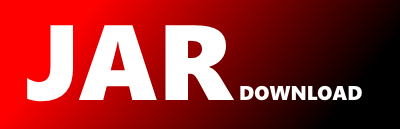
com.azure.resourcemanager.security.fluent.models.SecurityConnectorProperties Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-security Show documentation
Show all versions of azure-resourcemanager-security Show documentation
This package contains Microsoft Azure SDK for Security Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. API spec for Microsoft.Security (Azure Security Center) resource provider. Package tag package-composite-v3.
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.security.fluent.models;
import com.azure.core.annotation.Fluent;
import com.azure.core.util.CoreUtils;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import com.azure.resourcemanager.security.models.CloudName;
import com.azure.resourcemanager.security.models.CloudOffering;
import com.azure.resourcemanager.security.models.EnvironmentData;
import java.io.IOException;
import java.time.OffsetDateTime;
import java.util.List;
/**
* A set of properties that defines the security connector configuration.
*/
@Fluent
public final class SecurityConnectorProperties implements JsonSerializable {
/*
* The multi cloud resource identifier (account id in case of AWS connector, project number in case of GCP
* connector).
*/
private String hierarchyIdentifier;
/*
* The date on which the trial period will end, if applicable. Trial period exists for 30 days after upgrading to
* payed offerings.
*/
private OffsetDateTime hierarchyIdentifierTrialEndDate;
/*
* The multi cloud resource's cloud name.
*/
private CloudName environmentName;
/*
* A collection of offerings for the security connector.
*/
private List offerings;
/*
* The security connector environment data.
*/
private EnvironmentData environmentData;
/**
* Creates an instance of SecurityConnectorProperties class.
*/
public SecurityConnectorProperties() {
}
/**
* Get the hierarchyIdentifier property: The multi cloud resource identifier (account id in case of AWS connector,
* project number in case of GCP connector).
*
* @return the hierarchyIdentifier value.
*/
public String hierarchyIdentifier() {
return this.hierarchyIdentifier;
}
/**
* Set the hierarchyIdentifier property: The multi cloud resource identifier (account id in case of AWS connector,
* project number in case of GCP connector).
*
* @param hierarchyIdentifier the hierarchyIdentifier value to set.
* @return the SecurityConnectorProperties object itself.
*/
public SecurityConnectorProperties withHierarchyIdentifier(String hierarchyIdentifier) {
this.hierarchyIdentifier = hierarchyIdentifier;
return this;
}
/**
* Get the hierarchyIdentifierTrialEndDate property: The date on which the trial period will end, if applicable.
* Trial period exists for 30 days after upgrading to payed offerings.
*
* @return the hierarchyIdentifierTrialEndDate value.
*/
public OffsetDateTime hierarchyIdentifierTrialEndDate() {
return this.hierarchyIdentifierTrialEndDate;
}
/**
* Get the environmentName property: The multi cloud resource's cloud name.
*
* @return the environmentName value.
*/
public CloudName environmentName() {
return this.environmentName;
}
/**
* Set the environmentName property: The multi cloud resource's cloud name.
*
* @param environmentName the environmentName value to set.
* @return the SecurityConnectorProperties object itself.
*/
public SecurityConnectorProperties withEnvironmentName(CloudName environmentName) {
this.environmentName = environmentName;
return this;
}
/**
* Get the offerings property: A collection of offerings for the security connector.
*
* @return the offerings value.
*/
public List offerings() {
return this.offerings;
}
/**
* Set the offerings property: A collection of offerings for the security connector.
*
* @param offerings the offerings value to set.
* @return the SecurityConnectorProperties object itself.
*/
public SecurityConnectorProperties withOfferings(List offerings) {
this.offerings = offerings;
return this;
}
/**
* Get the environmentData property: The security connector environment data.
*
* @return the environmentData value.
*/
public EnvironmentData environmentData() {
return this.environmentData;
}
/**
* Set the environmentData property: The security connector environment data.
*
* @param environmentData the environmentData value to set.
* @return the SecurityConnectorProperties object itself.
*/
public SecurityConnectorProperties withEnvironmentData(EnvironmentData environmentData) {
this.environmentData = environmentData;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (offerings() != null) {
offerings().forEach(e -> e.validate());
}
if (environmentData() != null) {
environmentData().validate();
}
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("hierarchyIdentifier", this.hierarchyIdentifier);
jsonWriter.writeStringField("environmentName",
this.environmentName == null ? null : this.environmentName.toString());
jsonWriter.writeArrayField("offerings", this.offerings, (writer, element) -> writer.writeJson(element));
jsonWriter.writeJsonField("environmentData", this.environmentData);
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of SecurityConnectorProperties from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of SecurityConnectorProperties if the JsonReader was pointing to an instance of it, or null
* if it was pointing to JSON null.
* @throws IOException If an error occurs while reading the SecurityConnectorProperties.
*/
public static SecurityConnectorProperties fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
SecurityConnectorProperties deserializedSecurityConnectorProperties = new SecurityConnectorProperties();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("hierarchyIdentifier".equals(fieldName)) {
deserializedSecurityConnectorProperties.hierarchyIdentifier = reader.getString();
} else if ("hierarchyIdentifierTrialEndDate".equals(fieldName)) {
deserializedSecurityConnectorProperties.hierarchyIdentifierTrialEndDate = reader
.getNullable(nonNullReader -> CoreUtils.parseBestOffsetDateTime(nonNullReader.getString()));
} else if ("environmentName".equals(fieldName)) {
deserializedSecurityConnectorProperties.environmentName = CloudName.fromString(reader.getString());
} else if ("offerings".equals(fieldName)) {
List offerings = reader.readArray(reader1 -> CloudOffering.fromJson(reader1));
deserializedSecurityConnectorProperties.offerings = offerings;
} else if ("environmentData".equals(fieldName)) {
deserializedSecurityConnectorProperties.environmentData = EnvironmentData.fromJson(reader);
} else {
reader.skipChildren();
}
}
return deserializedSecurityConnectorProperties;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy