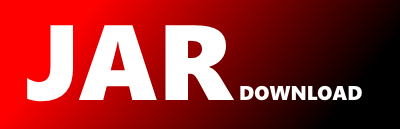
com.azure.resourcemanager.security.fluent.models.SecuritySubAssessmentProperties Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-security Show documentation
Show all versions of azure-resourcemanager-security Show documentation
This package contains Microsoft Azure SDK for Security Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. API spec for Microsoft.Security (Azure Security Center) resource provider. Package tag package-composite-v3.
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.security.fluent.models;
import com.azure.core.annotation.Fluent;
import com.azure.core.util.CoreUtils;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import com.azure.resourcemanager.security.models.AdditionalData;
import com.azure.resourcemanager.security.models.ResourceDetails;
import com.azure.resourcemanager.security.models.SubAssessmentStatus;
import java.io.IOException;
import java.time.OffsetDateTime;
/**
* Describes properties of an sub-assessment.
*/
@Fluent
public final class SecuritySubAssessmentProperties implements JsonSerializable {
/*
* Vulnerability ID
*/
private String id;
/*
* User friendly display name of the sub-assessment
*/
private String displayName;
/*
* Status of the sub-assessment
*/
private SubAssessmentStatus status;
/*
* Information on how to remediate this sub-assessment
*/
private String remediation;
/*
* Description of the impact of this sub-assessment
*/
private String impact;
/*
* Category of the sub-assessment
*/
private String category;
/*
* Human readable description of the assessment status
*/
private String description;
/*
* The date and time the sub-assessment was generated
*/
private OffsetDateTime timeGenerated;
/*
* Details of the resource that was assessed
*/
private ResourceDetails resourceDetails;
/*
* Details of the sub-assessment
*/
private AdditionalData additionalData;
/**
* Creates an instance of SecuritySubAssessmentProperties class.
*/
public SecuritySubAssessmentProperties() {
}
/**
* Get the id property: Vulnerability ID.
*
* @return the id value.
*/
public String id() {
return this.id;
}
/**
* Get the displayName property: User friendly display name of the sub-assessment.
*
* @return the displayName value.
*/
public String displayName() {
return this.displayName;
}
/**
* Get the status property: Status of the sub-assessment.
*
* @return the status value.
*/
public SubAssessmentStatus status() {
return this.status;
}
/**
* Set the status property: Status of the sub-assessment.
*
* @param status the status value to set.
* @return the SecuritySubAssessmentProperties object itself.
*/
public SecuritySubAssessmentProperties withStatus(SubAssessmentStatus status) {
this.status = status;
return this;
}
/**
* Get the remediation property: Information on how to remediate this sub-assessment.
*
* @return the remediation value.
*/
public String remediation() {
return this.remediation;
}
/**
* Get the impact property: Description of the impact of this sub-assessment.
*
* @return the impact value.
*/
public String impact() {
return this.impact;
}
/**
* Get the category property: Category of the sub-assessment.
*
* @return the category value.
*/
public String category() {
return this.category;
}
/**
* Get the description property: Human readable description of the assessment status.
*
* @return the description value.
*/
public String description() {
return this.description;
}
/**
* Get the timeGenerated property: The date and time the sub-assessment was generated.
*
* @return the timeGenerated value.
*/
public OffsetDateTime timeGenerated() {
return this.timeGenerated;
}
/**
* Get the resourceDetails property: Details of the resource that was assessed.
*
* @return the resourceDetails value.
*/
public ResourceDetails resourceDetails() {
return this.resourceDetails;
}
/**
* Set the resourceDetails property: Details of the resource that was assessed.
*
* @param resourceDetails the resourceDetails value to set.
* @return the SecuritySubAssessmentProperties object itself.
*/
public SecuritySubAssessmentProperties withResourceDetails(ResourceDetails resourceDetails) {
this.resourceDetails = resourceDetails;
return this;
}
/**
* Get the additionalData property: Details of the sub-assessment.
*
* @return the additionalData value.
*/
public AdditionalData additionalData() {
return this.additionalData;
}
/**
* Set the additionalData property: Details of the sub-assessment.
*
* @param additionalData the additionalData value to set.
* @return the SecuritySubAssessmentProperties object itself.
*/
public SecuritySubAssessmentProperties withAdditionalData(AdditionalData additionalData) {
this.additionalData = additionalData;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (status() != null) {
status().validate();
}
if (resourceDetails() != null) {
resourceDetails().validate();
}
if (additionalData() != null) {
additionalData().validate();
}
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeJsonField("status", this.status);
jsonWriter.writeJsonField("resourceDetails", this.resourceDetails);
jsonWriter.writeJsonField("additionalData", this.additionalData);
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of SecuritySubAssessmentProperties from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of SecuritySubAssessmentProperties if the JsonReader was pointing to an instance of it, or
* null if it was pointing to JSON null.
* @throws IOException If an error occurs while reading the SecuritySubAssessmentProperties.
*/
public static SecuritySubAssessmentProperties fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
SecuritySubAssessmentProperties deserializedSecuritySubAssessmentProperties
= new SecuritySubAssessmentProperties();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("id".equals(fieldName)) {
deserializedSecuritySubAssessmentProperties.id = reader.getString();
} else if ("displayName".equals(fieldName)) {
deserializedSecuritySubAssessmentProperties.displayName = reader.getString();
} else if ("status".equals(fieldName)) {
deserializedSecuritySubAssessmentProperties.status = SubAssessmentStatus.fromJson(reader);
} else if ("remediation".equals(fieldName)) {
deserializedSecuritySubAssessmentProperties.remediation = reader.getString();
} else if ("impact".equals(fieldName)) {
deserializedSecuritySubAssessmentProperties.impact = reader.getString();
} else if ("category".equals(fieldName)) {
deserializedSecuritySubAssessmentProperties.category = reader.getString();
} else if ("description".equals(fieldName)) {
deserializedSecuritySubAssessmentProperties.description = reader.getString();
} else if ("timeGenerated".equals(fieldName)) {
deserializedSecuritySubAssessmentProperties.timeGenerated = reader
.getNullable(nonNullReader -> CoreUtils.parseBestOffsetDateTime(nonNullReader.getString()));
} else if ("resourceDetails".equals(fieldName)) {
deserializedSecuritySubAssessmentProperties.resourceDetails = ResourceDetails.fromJson(reader);
} else if ("additionalData".equals(fieldName)) {
deserializedSecuritySubAssessmentProperties.additionalData = AdditionalData.fromJson(reader);
} else {
reader.skipChildren();
}
}
return deserializedSecuritySubAssessmentProperties;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy